Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / Behavior / designeractionbehavior.cs / 1 / designeractionbehavior.cs
namespace System.Windows.Forms.Design.Behavior { using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Windows.Forms.Design; using System.Diagnostics.CodeAnalysis; ////// /// This is the Behavior that represents DesignerActions for a particular /// control. The DesignerActionBehavior is responsible for responding to the /// MouseDown message and either 1) selecting the control and changing the /// DesignerActionGlyph's image or 2) building up a chrome menu /// and requesting it to be shown. /// Also, this Behavior acts as a proxy between "clicked" context menu /// items and the actual DesignerActions that they represent. /// internal sealed class DesignerActionBehavior : Behavior { private IComponent relatedComponent;//The component we are bound to private DesignerActionUI parentUI;//ptr to the parenting UI, used for showing menus and setting selection private DesignerActionListCollection actionLists;//all the shortcuts! private IServiceProvider serviceProvider; // we need to cache the service provider here to be able to create the panel with the proper arguments private bool ignoreNextMouseUp = false; ////// /// Constructor that calls base and caches off the action lists. /// internal DesignerActionBehavior(IServiceProvider serviceProvider, IComponent relatedComponent, DesignerActionListCollection actionLists ,DesignerActionUI parentUI) { this.actionLists = actionLists; this.serviceProvider = serviceProvider; this.relatedComponent = relatedComponent; this.parentUI = parentUI; } ////// /// Returns the collection of DesignerActionLists this Behavior is managing. /// These will be dynamically updated (some can be removed, new ones can be /// added, etc...). /// internal DesignerActionListCollection ActionLists { get { return actionLists; } set { actionLists = value; } } ////// /// Returns the parenting UI (a DesignerActionUI) /// internal DesignerActionUI ParentUI { get { return parentUI; } } ////// /// Returns the Component that this glyph is attached to. /// internal IComponent RelatedComponent { get { return relatedComponent; } } ////// /// Hides the designer action panel UI. /// internal void HideUI() { ParentUI.HideDesignerActionPanel(); } internal DesignerActionPanel CreateDesignerActionPanel(IComponent relatedComponent) { // BUILD AND SHOW THE CHROME UI DesignerActionListCollection lists = new DesignerActionListCollection(); lists.AddRange(ActionLists); DesignerActionPanel dap = new DesignerActionPanel(serviceProvider); dap.UpdateTasks(lists, new DesignerActionListCollection(), SR.GetString(SR.DesignerActionPanel_DefaultPanelTitle, relatedComponent.GetType().Name), null); return dap; } ////// /// Shows the designer action panel UI associated with this glyph. /// internal void ShowUI(Glyph g) { DesignerActionGlyph glyph = g as DesignerActionGlyph; if (glyph == null) { Debug.Fail("Why are we trying to 'showui' on a glyph that's not a DesignerActionGlyph?"); return; } DesignerActionPanel dap = CreateDesignerActionPanel(RelatedComponent); ParentUI.ShowDesignerActionPanel(RelatedComponent, dap, glyph); } internal bool IgnoreNextMouseUp { [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] set { ignoreNextMouseUp = value; } } public override bool OnMouseDoubleClick(Glyph g, MouseButtons button, Point mouseLoc) { ignoreNextMouseUp = true; return true; } public override bool OnMouseDown(Glyph g, MouseButtons button, Point mouseLoc) { // we take the msg return (!ParentUI.IsDesignerActionPanelVisible); } ////// /// In response to a MouseUp, we will either 1) select the Glyph /// and control if not selected, or 2) Build up our context menu /// representing our DesignerActions and show it. /// public override bool OnMouseUp(Glyph g, MouseButtons button) { if (button != MouseButtons.Left || ParentUI == null) { return true; } bool returnValue = true; if(ParentUI.IsDesignerActionPanelVisible) { HideUI(); } else if(!ignoreNextMouseUp) { if(serviceProvider != null) { ISelectionService selectionService = (ISelectionService)serviceProvider.GetService(typeof(ISelectionService)); if(selectionService != null) { if(selectionService.PrimarySelection != RelatedComponent) { ListcomponentList = new List (); componentList.Add(RelatedComponent); selectionService.SetSelectedComponents(componentList, SelectionTypes.Primary); } } } ShowUI(g); } else { returnValue = false; } ignoreNextMouseUp = false; return returnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
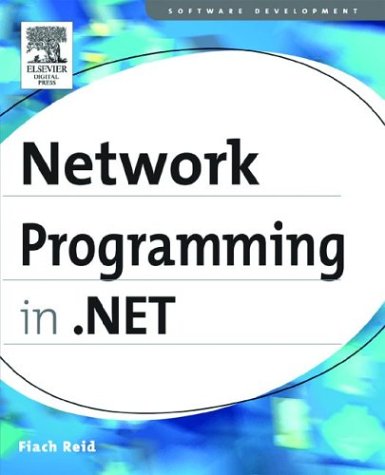
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OperationBehaviorAttribute.cs
- PlatformNotSupportedException.cs
- TypeGeneratedEventArgs.cs
- Selector.cs
- FutureFactory.cs
- File.cs
- ProjectedWrapper.cs
- HttpHandlersSection.cs
- XPathNodePointer.cs
- OdbcException.cs
- ServiceNameCollection.cs
- SynchronizedInputHelper.cs
- InkSerializer.cs
- UIElementCollection.cs
- CompositionDesigner.cs
- TypeSystemProvider.cs
- CommandHelpers.cs
- _StreamFramer.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- XmlComment.cs
- PersonalizationStateInfoCollection.cs
- GroupItem.cs
- PtsHelper.cs
- IFormattable.cs
- MergePropertyDescriptor.cs
- FormClosingEvent.cs
- DebuggerAttributes.cs
- IntellisenseTextBox.designer.cs
- PreDigestedSignedInfo.cs
- SqlUtil.cs
- Codec.cs
- SafeBitVector32.cs
- SafePEFileHandle.cs
- StylusButtonCollection.cs
- ValidationSummary.cs
- LinkedResource.cs
- SchemaType.cs
- CodeDomConfigurationHandler.cs
- PropertiesTab.cs
- MSG.cs
- PlatformNotSupportedException.cs
- ControlFilterExpression.cs
- AssemblyAssociatedContentFileAttribute.cs
- SecurityProtocolCorrelationState.cs
- KeyboardNavigation.cs
- DictionaryEntry.cs
- DesignerActionList.cs
- ParameterCollection.cs
- Viewport3DVisual.cs
- GeometryGroup.cs
- Command.cs
- LinkTarget.cs
- jithelpers.cs
- AddDataControlFieldDialog.cs
- NeutralResourcesLanguageAttribute.cs
- GridViewCommandEventArgs.cs
- NameNode.cs
- BinaryNode.cs
- LinqTreeNodeEvaluator.cs
- Label.cs
- AVElementHelper.cs
- CurrentTimeZone.cs
- QilFactory.cs
- CustomCategoryAttribute.cs
- DateTimeParse.cs
- Input.cs
- _ListenerAsyncResult.cs
- ExtendedProtectionPolicyTypeConverter.cs
- TextEditorThreadLocalStore.cs
- ListViewItemSelectionChangedEvent.cs
- Padding.cs
- GZipDecoder.cs
- HtmlImage.cs
- ByteAnimationUsingKeyFrames.cs
- BindingContext.cs
- shaper.cs
- StructuralType.cs
- CollaborationHelperFunctions.cs
- HttpModuleAction.cs
- SessionParameter.cs
- UnsafeNativeMethodsPenimc.cs
- GeometryGroup.cs
- SafeViewOfFileHandle.cs
- MembershipValidatePasswordEventArgs.cs
- WebPartConnectionsDisconnectVerb.cs
- InvokeHandlers.cs
- LazyTextWriterCreator.cs
- SQLResource.cs
- QEncodedStream.cs
- VirtualPathUtility.cs
- IsolatedStorageFileStream.cs
- FixedSOMSemanticBox.cs
- SqlGatherConsumedAliases.cs
- SettingsPropertyNotFoundException.cs
- RequestUriProcessor.cs
- Psha1DerivedKeyGenerator.cs
- RegionIterator.cs
- xdrvalidator.cs
- Command.cs
- SqlWriter.cs