Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Host / DesignSurfaceServiceContainer.cs / 1 / DesignSurfaceServiceContainer.cs
using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; namespace System.ComponentModel.Design { ////// A service container that supports "fixed" services. Fixed /// services cannot be removed. /// internal sealed class DesignSurfaceServiceContainer : ServiceContainer { private Hashtable _fixedServices; ////// We always add ourselves as a service. /// internal DesignSurfaceServiceContainer(IServiceProvider parentProvider) : base(parentProvider) { AddFixedService(typeof(DesignSurfaceServiceContainer), this); } ////// Removes the given service type from the service container. /// internal void AddFixedService(Type serviceType, object serviceInstance) { AddService(serviceType, serviceInstance); if (_fixedServices == null) { _fixedServices = new Hashtable(); } _fixedServices[serviceType] = serviceType; } ////// Removes a previously added fixed service. /// internal void RemoveFixedService(Type serviceType) { if (_fixedServices != null) { _fixedServices.Remove(serviceType); } RemoveService(serviceType); } ////// Removes the given service type from the service container. Throws /// an exception if the service is fixed. /// public override void RemoveService(Type serviceType, bool promote) { if (serviceType != null && _fixedServices != null && _fixedServices.ContainsKey(serviceType)) { throw new InvalidOperationException(SR.GetString(SR.DesignSurfaceServiceIsFixed, serviceType.Name)); } base.RemoveService(serviceType, promote); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
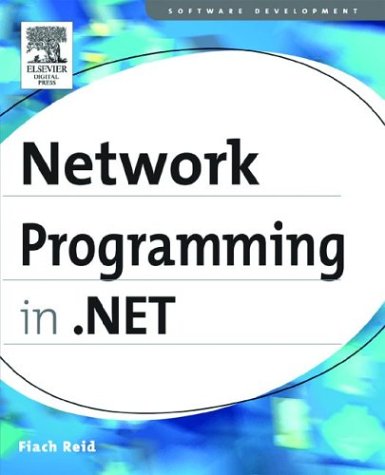
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CssClassPropertyAttribute.cs
- TextEditorLists.cs
- HttpStreams.cs
- safesecurityhelperavalon.cs
- SystemFonts.cs
- SerializationSectionGroup.cs
- CorrelationManager.cs
- StreamWithDictionary.cs
- InsufficientMemoryException.cs
- ClientEventManager.cs
- Substitution.cs
- WindowProviderWrapper.cs
- ItemContainerGenerator.cs
- BlurBitmapEffect.cs
- BaseCollection.cs
- ModelFunctionTypeElement.cs
- ErrorProvider.cs
- ToolBarButtonClickEvent.cs
- HtmlWindowCollection.cs
- WebPartDescriptionCollection.cs
- CngUIPolicy.cs
- MembershipUser.cs
- EdmToObjectNamespaceMap.cs
- ReflectTypeDescriptionProvider.cs
- BCLDebug.cs
- ExtractedStateEntry.cs
- glyphs.cs
- SizeKeyFrameCollection.cs
- HebrewNumber.cs
- ItemsControlAutomationPeer.cs
- AddInBase.cs
- WindowsListBox.cs
- Visitor.cs
- IApplicationTrustManager.cs
- recordstate.cs
- DataGridViewColumn.cs
- DomainUpDown.cs
- XmlCodeExporter.cs
- Clock.cs
- PDBReader.cs
- HostedTransportConfigurationManager.cs
- ListItemConverter.cs
- SuppressMergeCheckAttribute.cs
- GetWinFXPath.cs
- DataGridTextColumn.cs
- TabControlCancelEvent.cs
- OracleMonthSpan.cs
- _SslState.cs
- CultureInfoConverter.cs
- EntryPointNotFoundException.cs
- DrawingAttributeSerializer.cs
- Calendar.cs
- XPathSingletonIterator.cs
- _Win32.cs
- StdValidatorsAndConverters.cs
- TransactionContextValidator.cs
- MessageSmuggler.cs
- BaseCodeDomTreeGenerator.cs
- MenuItem.cs
- ResourceAttributes.cs
- figurelength.cs
- MessageFormatterConverter.cs
- EdgeModeValidation.cs
- BufferedResponseStream.cs
- XmlSchemaCollection.cs
- ListItemConverter.cs
- GuidelineCollection.cs
- XmlSchemaSimpleContentRestriction.cs
- X509CertificateInitiatorClientCredential.cs
- StrokeCollectionDefaultValueFactory.cs
- ViewDesigner.cs
- WindowsListView.cs
- Speller.cs
- InlineUIContainer.cs
- ISAPIWorkerRequest.cs
- Model3D.cs
- StringInfo.cs
- HtmlInputSubmit.cs
- ResourceContainer.cs
- SQLUtility.cs
- InkCanvasAutomationPeer.cs
- Metafile.cs
- DistinctQueryOperator.cs
- HttpStreamXmlDictionaryReader.cs
- PackageDigitalSignature.cs
- XsdDateTime.cs
- WebPartUtil.cs
- KeyedQueue.cs
- _UriTypeConverter.cs
- TreeNodeBindingCollection.cs
- WebGetAttribute.cs
- Setter.cs
- ThreadAbortException.cs
- DeclarativeCatalogPartDesigner.cs
- PrtCap_Base.cs
- EndPoint.cs
- ServiceHttpModule.cs
- DateTimeConstantAttribute.cs
- ClientRolePrincipal.cs
- EnumConverter.cs