Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Data / DesignerDataConnection.cs / 1 / DesignerDataConnection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Data { using System; using System.Collections; using System.Windows.Forms; ////// A data connection represents a single connection to a particular /// database or data source in the design tool or in an application /// config file. /// /// A DesignerDataConnection object may also be passed to other APIs /// to get access to services such as database schema information or /// the QueryBuilder host dialog. /// public sealed class DesignerDataConnection { private string _connectionString; private bool _isConfigured; private string _name; private string _providerName; ////// Creates a new instance of a DesignerDataConnection representing a /// database connection stored by a host environment or located in an /// application config file. /// This constructor is used to create non-configured connections. /// public DesignerDataConnection(string name, string providerName, string connectionString) : this(name, providerName, connectionString, false) { } ////// Creates a new instance of a DesignerDataConnection representing a /// database connection stored by a host environment or located in an /// application config file. /// This constructor is used to create both configured and /// non-configured connections. /// public DesignerDataConnection(string name, string providerName, string connectionString, bool isConfigured) { _name = name; _providerName = providerName; _connectionString = connectionString; _isConfigured = isConfigured; } ////// The connection string value for the connection. /// public string ConnectionString { get { return _connectionString; } } ////// Returns true if the connection is configured in the /// application-level configuration file (web.config), false /// otherwise. /// public bool IsConfigured { get { return _isConfigured; } } ////// The name associated with this connection in the design tool. Typically /// this is used to represent the connection in user interface. /// If this is a configured connection (IsConfigured=true) then this is /// the name of the connection defined in the public string Name { get { return _name; } } ////// section of the application web.config. /// /// The name of the ADO.NET managed provider used to access data from this /// connection. /// public string ProviderName { get { return _providerName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
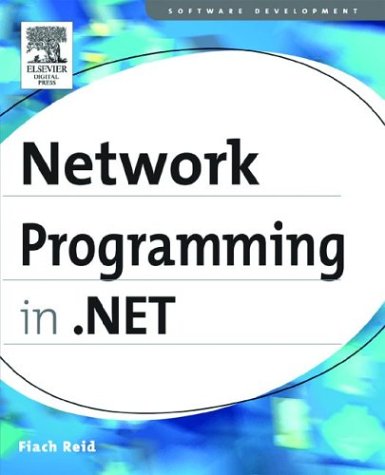
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArrayHelper.cs
- SequentialWorkflowRootDesigner.cs
- HttpHandlersSection.cs
- SettingsContext.cs
- TimestampInformation.cs
- ServiceOperationParameter.cs
- SmtpLoginAuthenticationModule.cs
- ScopeElementCollection.cs
- HexParser.cs
- ScrollEvent.cs
- EntityDataSourceView.cs
- InkCanvasAutomationPeer.cs
- WindowsFormsDesignerOptionService.cs
- FixedSOMPage.cs
- Maps.cs
- FactoryId.cs
- Animatable.cs
- ObjectViewQueryResultData.cs
- DrawListViewSubItemEventArgs.cs
- RoutedEventArgs.cs
- IIS7WorkerRequest.cs
- WindowsSidIdentity.cs
- Symbol.cs
- Module.cs
- CodePageUtils.cs
- SendingRequestEventArgs.cs
- RightsController.cs
- TrayIconDesigner.cs
- ClientTargetCollection.cs
- MenuCommands.cs
- ScrollEvent.cs
- MdImport.cs
- SortableBindingList.cs
- TextElement.cs
- UshortList2.cs
- StorageEntitySetMapping.cs
- TextOutput.cs
- UnknownWrapper.cs
- DeploymentSection.cs
- MouseGestureValueSerializer.cs
- QueryableFilterRepeater.cs
- SetIterators.cs
- MsdtcWrapper.cs
- _BasicClient.cs
- CodeMethodInvokeExpression.cs
- TreeViewItemAutomationPeer.cs
- InstanceStoreQueryResult.cs
- TransformerConfigurationWizardBase.cs
- ForwardPositionQuery.cs
- LayoutSettings.cs
- NonClientArea.cs
- SmtpLoginAuthenticationModule.cs
- SchemaDeclBase.cs
- RectAnimationClockResource.cs
- WmlControlAdapter.cs
- SequenceRangeCollection.cs
- HierarchicalDataBoundControl.cs
- XmlWriter.cs
- GrammarBuilderDictation.cs
- QilSortKey.cs
- TypedDataSourceCodeGenerator.cs
- DotExpr.cs
- AddInDeploymentState.cs
- DesignerAutoFormatCollection.cs
- DataIdProcessor.cs
- RadioButton.cs
- SystemWebCachingSectionGroup.cs
- GraphicsPathIterator.cs
- KeyNotFoundException.cs
- SoapFault.cs
- HuffCodec.cs
- _Semaphore.cs
- CompareInfo.cs
- DesignerVerb.cs
- PartialClassGenerationTaskInternal.cs
- StronglyTypedResourceBuilder.cs
- PictureBox.cs
- DataServiceQueryOfT.cs
- SessionStateItemCollection.cs
- InstanceNameConverter.cs
- ComplexObject.cs
- CurrentChangedEventManager.cs
- WindowsProgressbar.cs
- ToolStripEditorManager.cs
- SoapUnknownHeader.cs
- TracingConnectionListener.cs
- DLinqTableProvider.cs
- QueueProcessor.cs
- ToolStripTextBox.cs
- HtmlImage.cs
- CapabilitiesSection.cs
- StringKeyFrameCollection.cs
- TaskFactory.cs
- MemberPath.cs
- DateTimeFormatInfo.cs
- ErrorFormatterPage.cs
- SortFieldComparer.cs
- HttpHandlerAction.cs
- SqlDependencyUtils.cs
- XmlSchemaResource.cs