Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / X509Certificates / TimestampInformation.cs / 1305376 / TimestampInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the timestamp applied to a manifest's Authenticode signature /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class TimestampInformation { private CapiNative.AlgorithmId m_hashAlgorithmId; private DateTime m_timestamp; private X509Chain m_timestampChain; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_timestamper; //// [System.Security.SecurityCritical] internal TimestampInformation(X509Native.AXL_AUTHENTICODE_TIMESTAMPER_INFO timestamper) { m_hashAlgorithmId = timestamper.algHash; m_verificationResult = (SignatureVerificationResult)timestamper.dwError; ulong filetime = ((ulong)((uint)timestamper.ftTimestamp.dwHighDateTime) << 32) | (ulong)((uint)timestamper.ftTimestamp.dwLowDateTime); m_timestamp = DateTime.FromFileTimeUtc((long)filetime); if (timestamper.pChainContext != IntPtr.Zero) { m_timestampChain = new X509Chain(timestamper.pChainContext); } } internal TimestampInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid, "error != SignatureVerificationResult.Valid"); m_verificationResult = error; } ///// /// Hash algorithm the timestamp signature was calculated with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the timestamp /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// Is the signature of the timestamp valid /// public bool IsValid { get { // Timestamp signatures are valid only if they were created by a trusted chain return VerificationResult == SignatureVerificationResult.Valid || VerificationResult == SignatureVerificationResult.CertificateNotExplicitlyTrusted; } } ////// Chain of certificates used to verify the timestamp /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_timestampChain; } } ////// Certificate that signed the timestamp /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_timestamper == null && SignatureChain != null) { m_timestamper = SignatureChain.ChainElements[0].Certificate; } return m_timestamper; } } ////// When the timestamp was applied, expressed in local time /// public DateTime Timestamp { get { return m_timestamp.ToLocalTime(); } } ////// Result of verifying the timestamp signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the timestamp applied to a manifest's Authenticode signature /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class TimestampInformation { private CapiNative.AlgorithmId m_hashAlgorithmId; private DateTime m_timestamp; private X509Chain m_timestampChain; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_timestamper; //// [System.Security.SecurityCritical] internal TimestampInformation(X509Native.AXL_AUTHENTICODE_TIMESTAMPER_INFO timestamper) { m_hashAlgorithmId = timestamper.algHash; m_verificationResult = (SignatureVerificationResult)timestamper.dwError; ulong filetime = ((ulong)((uint)timestamper.ftTimestamp.dwHighDateTime) << 32) | (ulong)((uint)timestamper.ftTimestamp.dwLowDateTime); m_timestamp = DateTime.FromFileTimeUtc((long)filetime); if (timestamper.pChainContext != IntPtr.Zero) { m_timestampChain = new X509Chain(timestamper.pChainContext); } } internal TimestampInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid, "error != SignatureVerificationResult.Valid"); m_verificationResult = error; } ///// /// Hash algorithm the timestamp signature was calculated with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the timestamp /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// Is the signature of the timestamp valid /// public bool IsValid { get { // Timestamp signatures are valid only if they were created by a trusted chain return VerificationResult == SignatureVerificationResult.Valid || VerificationResult == SignatureVerificationResult.CertificateNotExplicitlyTrusted; } } ////// Chain of certificates used to verify the timestamp /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_timestampChain; } } ////// Certificate that signed the timestamp /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_timestamper == null && SignatureChain != null) { m_timestamper = SignatureChain.ChainElements[0].Certificate; } return m_timestamper; } } ////// When the timestamp was applied, expressed in local time /// public DateTime Timestamp { get { return m_timestamp.ToLocalTime(); } } ////// Result of verifying the timestamp signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
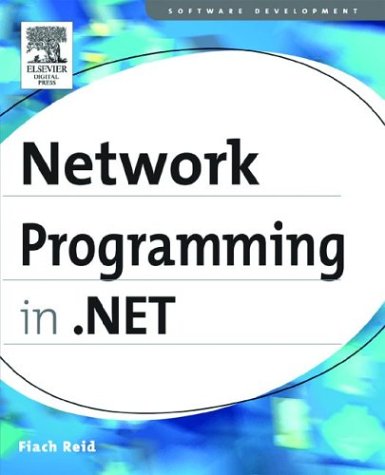
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlConvert.cs
- ClientRuntimeConfig.cs
- Registry.cs
- ConnectionProviderAttribute.cs
- CustomLineCap.cs
- ConnectionStringsExpressionBuilder.cs
- PointLight.cs
- ConnectionPoolRegistry.cs
- ArrayExtension.cs
- PropertyChangedEventArgs.cs
- KnownBoxes.cs
- basevalidator.cs
- safesecurityhelperavalon.cs
- Models.cs
- AlternationConverter.cs
- Property.cs
- RemotingSurrogateSelector.cs
- ExpressionEditor.cs
- WinEventTracker.cs
- StringBuilder.cs
- ConfigurationSectionHelper.cs
- Highlights.cs
- SignatureToken.cs
- HttpRequestWrapper.cs
- ResourceKey.cs
- HandlerMappingMemo.cs
- ProfileParameter.cs
- QilList.cs
- PrintPageEvent.cs
- SmiGettersStream.cs
- ZoomPercentageConverter.cs
- log.cs
- SessionStateSection.cs
- SafeSecurityHandles.cs
- StaticFileHandler.cs
- ImageDrawing.cs
- RuleProcessor.cs
- DebugView.cs
- ReadOnlyHierarchicalDataSource.cs
- FindRequestContext.cs
- TextContainerChangedEventArgs.cs
- X509ChainElement.cs
- GridViewColumnHeaderAutomationPeer.cs
- RootBuilder.cs
- BuildResultCache.cs
- Attributes.cs
- PrintPreviewControl.cs
- ExitEventArgs.cs
- FieldBuilder.cs
- AssemblyUtil.cs
- AmbientEnvironment.cs
- documentation.cs
- DropSourceBehavior.cs
- HierarchicalDataTemplate.cs
- FrameworkContentElement.cs
- DataGridViewAccessibleObject.cs
- CopyOfAction.cs
- DataGridBoolColumn.cs
- infer.cs
- TypeNameConverter.cs
- HttpRawResponse.cs
- DbDataRecord.cs
- BinaryFormatter.cs
- ListView.cs
- HtmlControlPersistable.cs
- CodeDOMProvider.cs
- RuntimeIdentifierPropertyAttribute.cs
- DataObjectMethodAttribute.cs
- SecurityTokenProvider.cs
- XpsFixedPageReaderWriter.cs
- PeerCollaboration.cs
- ObjectSecurity.cs
- ComponentGlyph.cs
- HttpDictionary.cs
- TableHeaderCell.cs
- ApplicationSecurityManager.cs
- ConnectionPointCookie.cs
- TableHeaderCell.cs
- AuthenticationManager.cs
- SqlDataReader.cs
- QilExpression.cs
- DetailsView.cs
- LambdaCompiler.ControlFlow.cs
- XmlMemberMapping.cs
- D3DImage.cs
- EnumType.cs
- HtmlTitle.cs
- AsynchronousChannelMergeEnumerator.cs
- WebPageTraceListener.cs
- Registry.cs
- AttributeXamlType.cs
- ZipQueryOperator.cs
- ParameterBuilder.cs
- DataContractSerializerSection.cs
- DeadCharTextComposition.cs
- WebPartZoneAutoFormat.cs
- OleDbEnumerator.cs
- ConnectionManagementElement.cs
- AsyncDataRequest.cs
- TemplateNodeContextMenu.cs