Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / MemberDescriptor.cs / 1 / MemberDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel.Design; using System.Diagnostics; using System.Reflection; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Runtime.InteropServices.ComVisible(true)] public abstract class MemberDescriptor { private string name; private string displayName; private int nameHash; private AttributeCollection attributeCollection; private Attribute[] attributes; private Attribute[] originalAttributes; private bool attributesFiltered = false; private bool attributesFilled = false; private int metadataVersion; private string category; private string description; ////// Declares an array of attributes for a member and defines /// the properties and methods that give you access to the attributes in the array. /// All attributes must derive from ///. /// /// protected MemberDescriptor(string name) : this(name, null) { } ////// Initializes a new instance of the ///class with the specified and no /// attributes. /// /// protected MemberDescriptor(string name, Attribute[] attributes) { try { if (name == null || name.Length == 0) { throw new ArgumentException(SR.GetString(SR.InvalidMemberName)); } this.name = name; this.displayName = name; this.nameHash = name.GetHashCode(); if (attributes != null) { this.attributes = attributes; attributesFiltered = false; } this.originalAttributes = this.attributes; } catch (Exception t) { Debug.Fail(t.ToString()); throw t; } } ////// Initializes a new instance of the ///class with the specified /// and /// array. /// /// protected MemberDescriptor(MemberDescriptor descr) { this.name = descr.Name; this.displayName = this.name; this.nameHash = name.GetHashCode(); this.attributes = new Attribute[descr.Attributes.Count]; descr.Attributes.CopyTo(this.attributes, 0); attributesFiltered = true; this.originalAttributes = this.attributes; } ////// Initializes a new instance of the ///class with the specified . /// /// protected MemberDescriptor(MemberDescriptor oldMemberDescriptor, Attribute[] newAttributes) { this.name = oldMemberDescriptor.Name; this.displayName = oldMemberDescriptor.DisplayName; this.nameHash = name.GetHashCode(); ArrayList newArray = new ArrayList(); if (oldMemberDescriptor.Attributes.Count != 0) { foreach (object o in oldMemberDescriptor.Attributes) { newArray.Add(o); } } if (newAttributes != null) { foreach (object o in newAttributes) { newArray.Add(o); } } this.attributes = new Attribute[ newArray.Count ]; newArray.CopyTo( this.attributes, 0); attributesFiltered = false; this.originalAttributes = this.attributes; } ////// Initializes a new instance of the ///class with the name in the specified /// and the attributes /// in both the old and the array. /// /// protected virtual Attribute[] AttributeArray { get { CheckAttributesValid(); FilterAttributesIfNeeded(); return attributes; } set { lock(this) { attributes = value; originalAttributes = value; attributesFiltered = false; attributeCollection = null; } } } ////// Gets or sets an array of /// attributes. /// ////// public virtual AttributeCollection Attributes { get { CheckAttributesValid(); if (attributeCollection == null) { attributeCollection = CreateAttributeCollection(); } return attributeCollection; } } ////// Gets the collection of attributes for this member. /// ////// public virtual string Category { get { if (category == null) { category = ((CategoryAttribute)Attributes[typeof(CategoryAttribute)]).Category; } return category; } } ////// Gets the name of the category that the /// member /// belongs to, as specified in the ///. /// /// public virtual string Description { get { if (description == null) { description = ((DescriptionAttribute) Attributes[typeof(DescriptionAttribute)]).Description; } return description; } } ////// Gets the description of /// the member as specified in the ///. /// /// public virtual bool IsBrowsable { get { return((BrowsableAttribute)Attributes[typeof(BrowsableAttribute)]).Browsable; } } ////// Gets a value indicating whether the member is browsable as specified in the /// ///. /// /// public virtual string Name { get { if (name == null) { return ""; } return name; } } ////// Gets the /// name of the member. /// ////// protected virtual int NameHashCode { get { return nameHash; } } ////// Gets the hash /// code for the name of the member as specified in ///. /// /// public virtual bool DesignTimeOnly { get { return(DesignOnlyAttribute.Yes.Equals(Attributes[typeof(DesignOnlyAttribute)])); } } ////// Determines whether this member should be set only at /// design time as specified in the ///. /// /// public virtual string DisplayName { get { DisplayNameAttribute displayNameAttr = Attributes[typeof(DisplayNameAttribute)] as DisplayNameAttribute; if (displayNameAttr == null || displayNameAttr.IsDefaultAttribute()) { return displayName; } return displayNameAttr.DisplayName; } } ////// Gets the name that can be displayed in a window like a /// properties window. /// ////// Called each time we access the attribtes on /// this member descriptor to give deriving classes /// a chance to change them on the fly. /// private void CheckAttributesValid() { if (attributesFiltered) { if (metadataVersion != TypeDescriptor.MetadataVersion) { attributesFilled = false; attributesFiltered = false; attributeCollection = null; } } } ////// /// protected virtual AttributeCollection CreateAttributeCollection() { return new AttributeCollection(AttributeArray); } ////// Creates a collection of attributes using the /// array of attributes that you passed to the constructor. /// ////// public override bool Equals(object obj) { if (this == obj) { return true; } if (obj == null) { return false; } if (obj.GetType() != GetType()) { return false; } MemberDescriptor mdObj = (MemberDescriptor)obj; FilterAttributesIfNeeded(); mdObj.FilterAttributesIfNeeded(); if (mdObj.nameHash != nameHash) { return false; } if ((mdObj.category == null) != (category == null) || (category != null && !mdObj.category.Equals(category))) { return false; } if ((mdObj.description == null) != (description == null) || (description != null && !mdObj.category.Equals(description))) { return false; } if ((mdObj.attributes == null) != (attributes == null)) { return false; } bool sameAttrs = true; if (attributes != null) { if (attributes.Length != mdObj.attributes.Length) { return false; } for (int i = 0; i < attributes.Length; i++) { if (!attributes[i].Equals(mdObj.attributes[i])) { sameAttrs = false; break; } } } return sameAttrs; } ////// Compares this instance to the specified ///to see if they are equivalent. /// NOTE: If you make a change here, you likely need to change GetHashCode() as well. /// /// protected virtual void FillAttributes(IList attributeList) { if (originalAttributes != null) { foreach (Attribute attr in originalAttributes) { attributeList.Add(attr); } } } private void FilterAttributesIfNeeded() { if (!attributesFiltered) { IList list; if (!attributesFilled) { list = new ArrayList(); try { FillAttributes(list); } catch (Exception e) { Debug.Fail(name + ">>" + e.ToString()); } } else { list = new ArrayList(attributes); } Hashtable hash = new Hashtable(list.Count); foreach (Attribute attr in list) { hash[attr.TypeId] = attr; } Attribute[] newAttributes = new Attribute[hash.Values.Count]; hash.Values.CopyTo(newAttributes, 0); lock(this) { attributes = newAttributes; attributesFiltered = true; attributesFilled = true; metadataVersion = TypeDescriptor.MetadataVersion; } } } ////// In an inheriting class, adds the attributes of the inheriting class to the /// specified list of attributes in the parent class. For duplicate attributes, /// the last one added to the list will be kept. /// ////// protected static MethodInfo FindMethod(Type componentClass, string name, Type[] args, Type returnType) { return FindMethod(componentClass, name, args, returnType, true); } ////// Finds the given method through reflection. This method only looks for public methods. /// ////// protected static MethodInfo FindMethod(Type componentClass, string name, Type[] args, Type returnType, bool publicOnly) { MethodInfo result = null; if (publicOnly) { result = componentClass.GetMethod(name, args); } else { result = componentClass.GetMethod(name, BindingFlags.Instance | BindingFlags.Static | BindingFlags.Public | BindingFlags.NonPublic, null, args, null); } if (result != null && result.ReturnType != returnType) { result = null; } return result; } ////// Finds the given method through reflection. /// ////// Try to keep this reasonable in sync with Equals(). Specifically, /// if A.Equals(B) returns true, A & B should have the same hash code. /// public override int GetHashCode() { return nameHash; } ////// This method returns the object that should be used during invocation of members. /// Normally the return value will be the same as the instance passed in. If /// someone associated another object with this instance, or if the instance is a /// custom type descriptor, GetInvocationTarget may return a different value. /// protected virtual object GetInvocationTarget(Type type, object instance) { if (type == null) { throw new ArgumentNullException("type"); } if (instance == null) { throw new ArgumentNullException("instance"); } return TypeDescriptor.GetAssociation(type, instance); } ////// protected static ISite GetSite(object component) { if (!(component is IComponent)) { return null; } return((IComponent)component).Site; } ////// Gets a component site /// for the given component. /// ////// [Obsolete("This method has been deprecated. Use GetInvocationTarget instead. http://go.microsoft.com/fwlink/?linkid=14202")] protected static object GetInvokee(Type componentClass, object component) { if (componentClass == null) { throw new ArgumentNullException("componentClass"); } if (component == null) { throw new ArgumentNullException("component"); } return TypeDescriptor.GetAssociation(componentClass, component); } } }/// Gets /// the component /// that a method should be invoked on. /// ///
Link Menu
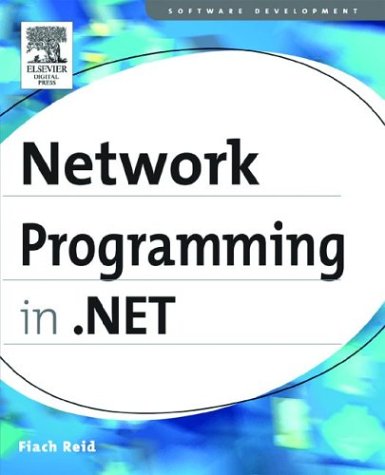
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SiteMapDataSourceView.cs
- PersonalizationProviderCollection.cs
- MetadataItem_Static.cs
- PropertyInfo.cs
- TypedTableBase.cs
- EntitySetBase.cs
- RegexRunnerFactory.cs
- ClientOptions.cs
- Rectangle.cs
- BulletedList.cs
- serverconfig.cs
- ValidationEventArgs.cs
- PackWebRequest.cs
- AsyncDataRequest.cs
- MessageDecoder.cs
- ClonableStack.cs
- EntityUtil.cs
- OdbcTransaction.cs
- EntityCollectionChangedParams.cs
- EndEvent.cs
- SpotLight.cs
- CriticalHandle.cs
- WindowsGrip.cs
- DataViewListener.cs
- MeasureItemEvent.cs
- DataViewListener.cs
- BitArray.cs
- CustomAssemblyResolver.cs
- WebServiceEnumData.cs
- ManagedFilter.cs
- DataListItemEventArgs.cs
- ScriptRegistrationManager.cs
- GACIdentityPermission.cs
- XPathNavigatorReader.cs
- HMAC.cs
- ObjectContext.cs
- Tag.cs
- SQLBinary.cs
- DataControlPagerLinkButton.cs
- ToolZoneDesigner.cs
- TemplateBindingExpressionConverter.cs
- RelatedCurrencyManager.cs
- NamedPermissionSet.cs
- VisualTreeUtils.cs
- EntityAdapter.cs
- GeneralTransformGroup.cs
- DBCSCodePageEncoding.cs
- OverrideMode.cs
- HeaderedContentControl.cs
- DataControlFieldCollection.cs
- ModifierKeysValueSerializer.cs
- DuplicateWaitObjectException.cs
- CheckBox.cs
- StringKeyFrameCollection.cs
- BooleanExpr.cs
- RawKeyboardInputReport.cs
- FillRuleValidation.cs
- EventLogInternal.cs
- HttpWriter.cs
- HtmlTextArea.cs
- EditorPartChrome.cs
- LoginViewDesigner.cs
- WebResourceAttribute.cs
- TextMetrics.cs
- DrawToolTipEventArgs.cs
- Model3D.cs
- TypeToStringValueConverter.cs
- Decorator.cs
- XmlAttributeOverrides.cs
- HttpValueCollection.cs
- SHA256.cs
- StopStoryboard.cs
- ReadOnlyDataSourceView.cs
- ModuleConfigurationInfo.cs
- SignatureHelper.cs
- TrackingDataItemValue.cs
- AdCreatedEventArgs.cs
- Stackframe.cs
- MemberBinding.cs
- DBDataPermissionAttribute.cs
- GlobalDataBindingHandler.cs
- TemplateBamlRecordReader.cs
- LocationInfo.cs
- ErasingStroke.cs
- ZipPackagePart.cs
- AnnotationComponentManager.cs
- DocumentViewerBase.cs
- CodeAttributeArgumentCollection.cs
- OwnerDrawPropertyBag.cs
- StringUtil.cs
- baseshape.cs
- UnwrappedTypesXmlSerializerManager.cs
- DataGridViewUtilities.cs
- PixelFormat.cs
- HoistedLocals.cs
- SmtpLoginAuthenticationModule.cs
- ToolStripArrowRenderEventArgs.cs
- TextRunCacheImp.cs
- CodeTypeParameter.cs
- WebPartEventArgs.cs