Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / CodeDOM / CodeIterationStatement.cs / 1 / CodeIterationStatement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using Microsoft.Win32; using System.Collections; using System.Runtime.InteropServices; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeIterationStatement : CodeStatement { private CodeStatement initStatement; private CodeExpression testExpression; private CodeStatement incrementStatement; private CodeStatementCollection statements = new CodeStatementCollection(); ////// Represents a simple for loop. /// ////// public CodeIterationStatement() { } ////// Initializes a new instance of ///. /// /// public CodeIterationStatement(CodeStatement initStatement, CodeExpression testExpression, CodeStatement incrementStatement, params CodeStatement[] statements) { InitStatement = initStatement; TestExpression = testExpression; IncrementStatement = incrementStatement; Statements.AddRange(statements); } ////// Initializes a new instance of ///. /// /// public CodeStatement InitStatement { get { return initStatement; } set { initStatement = value; } } ////// Gets or sets /// the loop initialization statement. /// ////// public CodeExpression TestExpression { get { return testExpression; } set { testExpression = value; } } ////// Gets or sets /// the expression to test for. /// ////// public CodeStatement IncrementStatement { get { return incrementStatement; } set { incrementStatement = value; } } ////// Gets or sets /// the per loop cycle increment statement. /// ////// public CodeStatementCollection Statements { get { return statements; } } } }/// Gets or sets /// the statements to be executed within the loop. /// ///
Link Menu
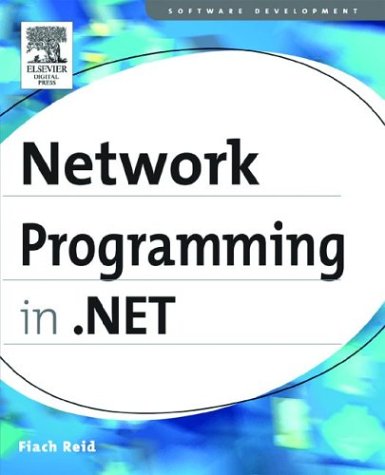
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlMappingCollection.cs
- RegexNode.cs
- NestedContainer.cs
- ProtocolReflector.cs
- AdRotatorDesigner.cs
- CriticalFinalizerObject.cs
- NavigationFailedEventArgs.cs
- XhtmlConformanceSection.cs
- InputLanguageCollection.cs
- IListConverters.cs
- HttpListenerException.cs
- Storyboard.cs
- ControlCollection.cs
- AttachmentService.cs
- DecoderBestFitFallback.cs
- PageEventArgs.cs
- ManagementPath.cs
- Types.cs
- WinFormsSpinner.cs
- ControlEvent.cs
- ProxyHelper.cs
- PageAdapter.cs
- TextParagraph.cs
- PersonalizationState.cs
- ArgumentFixer.cs
- ControlOperationInvoker.cs
- SemanticResolver.cs
- InsufficientMemoryException.cs
- TableRow.cs
- BatchServiceHost.cs
- InlinedAggregationOperatorEnumerator.cs
- PreservationFileWriter.cs
- SystemGatewayIPAddressInformation.cs
- _ConnectionGroup.cs
- XhtmlBasicValidationSummaryAdapter.cs
- OleCmdHelper.cs
- ColorKeyFrameCollection.cs
- WindowsGraphicsCacheManager.cs
- LowerCaseStringConverter.cs
- ElementProxy.cs
- FormsAuthenticationEventArgs.cs
- TileModeValidation.cs
- StrongNameKeyPair.cs
- TTSEngineTypes.cs
- BasicHttpMessageSecurityElement.cs
- RunWorkerCompletedEventArgs.cs
- objectquery_tresulttype.cs
- TypeResolvingOptions.cs
- ModelProperty.cs
- XPathScanner.cs
- Math.cs
- JsonStringDataContract.cs
- XmlLoader.cs
- EntityDataSourceQueryBuilder.cs
- FormatConvertedBitmap.cs
- CompareValidator.cs
- TextCompositionManager.cs
- DataGridViewToolTip.cs
- KeyboardEventArgs.cs
- WorkflowOperationErrorHandler.cs
- SelectionRange.cs
- SchemaImporterExtensionsSection.cs
- GridViewCommandEventArgs.cs
- GridViewColumn.cs
- SBCSCodePageEncoding.cs
- XmlDomTextWriter.cs
- Socket.cs
- RtfNavigator.cs
- XmlAttributeAttribute.cs
- WSSecurityPolicy.cs
- _Rfc2616CacheValidators.cs
- SourceLocation.cs
- SystemIcmpV4Statistics.cs
- _IPv6Address.cs
- ProcessModuleCollection.cs
- HasCopySemanticsAttribute.cs
- LocatorManager.cs
- CompilationSection.cs
- dtdvalidator.cs
- CapabilitiesUse.cs
- _Win32.cs
- IndicShape.cs
- RootBrowserWindowProxy.cs
- AuthenticodeSignatureInformation.cs
- DataGridViewColumnCollection.cs
- NamespaceTable.cs
- TypeConverter.cs
- WindowsEditBoxRange.cs
- AllMembershipCondition.cs
- GridViewEditEventArgs.cs
- JapaneseCalendar.cs
- ValueConversionAttribute.cs
- PersistenceProvider.cs
- String.cs
- Transform3DCollection.cs
- ExtensibleClassFactory.cs
- ISAPIRuntime.cs
- DynamicFilter.cs
- TypeDelegator.cs
- InfiniteTimeSpanConverter.cs