Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / objectquery_tresulttype.cs / 1305376 / objectquery_tresulttype.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Text.RegularExpressions; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Globalization; using System.Diagnostics; using System.Data; using System.Data.Common; using System.Data.Common.EntitySql; using System.Data.Common.Utils; using System.Data.Mapping; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; using System.Data.Common.CommandTrees.Internal; using System.Data.Objects.DataClasses; using System.Data.Objects.ELinq; using System.Data.Objects.Internal; using System.Data.Common.QueryCache; using System.Data.Entity; using System.Data.EntityClient; using System.Linq; using System.Linq.Expressions; using System.Reflection; namespace System.Data.Objects { ////// This class implements strongly-typed queries at the object-layer through /// Entity SQL text and query-building helper methods. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public partial class ObjectQuery: ObjectQuery, IEnumerable , IQueryable , IOrderedQueryable , IListSource { internal ObjectQuery(ObjectQueryState queryState) : base(queryState) { } #region Public Methods /// /// This method allows explicit query evaluation with a specified merge /// option which will override the merge option property. /// /// /// The MergeOption to use when executing the query. /// ////// An enumerable for the ObjectQuery results. /// public new ObjectResultExecute(MergeOption mergeOption) { EntityUtil.CheckArgumentMergeOption(mergeOption); return this.GetResults(mergeOption); } /// /// Adds a path to the set of navigation property span paths included in the results of this query /// /// The new span path ///A new ObjectQuery that includes the specified span path public ObjectQueryInclude(string path) { EntityUtil.CheckStringArgument(path, "path"); return new ObjectQuery (this.QueryState.Include(this, path)); } #endregion #region IEnumerable implementation /// /// These methods are the "executors" for the query. They can be called /// directly, or indirectly (by foreach'ing through the query, for example). /// IEnumeratorIEnumerable .GetEnumerator() { ObjectResult disposableEnumerable = this.GetResults(null); try { IEnumerator result = disposableEnumerable.GetEnumerator(); return result; } catch { // if there is a problem creating the enumerator, we should dispose // the enumerable (if there is no problem, the enumerator will take // care of the dispose) disposableEnumerable.Dispose(); throw; } } #endregion #region ObjectQuery Overrides internal override IEnumerator GetEnumeratorInternal() { return ((IEnumerable )this).GetEnumerator(); } internal override IList GetIListSourceListInternal() { return ((IListSource)this.GetResults(null)).GetList(); } internal override ObjectResult ExecuteInternal(MergeOption mergeOption) { return this.GetResults(mergeOption); } /// /// Retrieves the LINQ expression that backs this ObjectQuery for external consumption. /// It is important that the work to wrap the expression in an appropriate MergeAs call /// takes place in this method and NOT in ObjectQueryState.TryGetExpression which allows /// the unmodified expression (that does not include the MergeOption-preserving MergeAs call) /// to be retrieved and processed by the ELinq ExpressionConverter. /// ////// The LINQ expression for this ObjectQuery, wrapped in a MergeOption-preserving call /// to the MergeAs method if the ObjectQuery.MergeOption property has been set. /// internal override Expression GetExpression() { // If this ObjectQuery is not backed by a LINQ Expression (it is an ESQL query), // then create a ConstantExpression that uses this ObjectQuery as its value. Expression retExpr; if (!this.QueryState.TryGetExpression(out retExpr)) { retExpr = Expression.Constant(this); } Type objectQueryType = typeof(ObjectQuery); if (this.QueryState.UserSpecifiedMergeOption.HasValue) { MethodInfo mergeAsMethod = objectQueryType.GetMethod("MergeAs", BindingFlags.Instance | BindingFlags.NonPublic); Debug.Assert(mergeAsMethod != null, "Could not retrieve ObjectQuery .MergeAs method using reflection?"); retExpr = TypeSystem.EnsureType(retExpr, objectQueryType); retExpr = Expression.Call(retExpr, mergeAsMethod, Expression.Constant(this.QueryState.UserSpecifiedMergeOption.Value)); } if (null != this.QueryState.Span) { MethodInfo includeSpanMethod = objectQueryType.GetMethod("IncludeSpan", BindingFlags.Instance | BindingFlags.NonPublic); Debug.Assert(includeSpanMethod != null, "Could not retrieve ObjectQuery .IncludeSpan method using reflection?"); retExpr = TypeSystem.EnsureType(retExpr, objectQueryType); retExpr = Expression.Call(retExpr, includeSpanMethod, Expression.Constant(this.QueryState.Span)); } return retExpr; } // Intended for use only in the MethodCallExpression produced for inline queries. internal ObjectQuery MergeAs(MergeOption mergeOption) { throw EntityUtil.InvalidOperation(Strings.ELinq_MethodNotDirectlyCallable); } // Intended for use only in the MethodCallExpression produced for inline queries. internal ObjectQuery IncludeSpan(Span span) { throw EntityUtil.InvalidOperation(Strings.ELinq_MethodNotDirectlyCallable); } #endregion #region Private Methods private ObjectResult GetResults(MergeOption? forMergeOption) { this.QueryState.ObjectContext.EnsureConnection(); try { ObjectQueryExecutionPlan execPlan = this.QueryState.GetExecutionPlan(forMergeOption); return execPlan.Execute (this.QueryState.ObjectContext, this.QueryState.Parameters); } catch { this.QueryState.ObjectContext.ReleaseConnection(); throw; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Text.RegularExpressions; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Globalization; using System.Diagnostics; using System.Data; using System.Data.Common; using System.Data.Common.EntitySql; using System.Data.Common.Utils; using System.Data.Mapping; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; using System.Data.Common.CommandTrees.Internal; using System.Data.Objects.DataClasses; using System.Data.Objects.ELinq; using System.Data.Objects.Internal; using System.Data.Common.QueryCache; using System.Data.Entity; using System.Data.EntityClient; using System.Linq; using System.Linq.Expressions; using System.Reflection; namespace System.Data.Objects { ////// This class implements strongly-typed queries at the object-layer through /// Entity SQL text and query-building helper methods. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public partial class ObjectQuery: ObjectQuery, IEnumerable , IQueryable , IOrderedQueryable , IListSource { internal ObjectQuery(ObjectQueryState queryState) : base(queryState) { } #region Public Methods /// /// This method allows explicit query evaluation with a specified merge /// option which will override the merge option property. /// /// /// The MergeOption to use when executing the query. /// ////// An enumerable for the ObjectQuery results. /// public new ObjectResultExecute(MergeOption mergeOption) { EntityUtil.CheckArgumentMergeOption(mergeOption); return this.GetResults(mergeOption); } /// /// Adds a path to the set of navigation property span paths included in the results of this query /// /// The new span path ///A new ObjectQuery that includes the specified span path public ObjectQueryInclude(string path) { EntityUtil.CheckStringArgument(path, "path"); return new ObjectQuery (this.QueryState.Include(this, path)); } #endregion #region IEnumerable implementation /// /// These methods are the "executors" for the query. They can be called /// directly, or indirectly (by foreach'ing through the query, for example). /// IEnumeratorIEnumerable .GetEnumerator() { ObjectResult disposableEnumerable = this.GetResults(null); try { IEnumerator result = disposableEnumerable.GetEnumerator(); return result; } catch { // if there is a problem creating the enumerator, we should dispose // the enumerable (if there is no problem, the enumerator will take // care of the dispose) disposableEnumerable.Dispose(); throw; } } #endregion #region ObjectQuery Overrides internal override IEnumerator GetEnumeratorInternal() { return ((IEnumerable )this).GetEnumerator(); } internal override IList GetIListSourceListInternal() { return ((IListSource)this.GetResults(null)).GetList(); } internal override ObjectResult ExecuteInternal(MergeOption mergeOption) { return this.GetResults(mergeOption); } /// /// Retrieves the LINQ expression that backs this ObjectQuery for external consumption. /// It is important that the work to wrap the expression in an appropriate MergeAs call /// takes place in this method and NOT in ObjectQueryState.TryGetExpression which allows /// the unmodified expression (that does not include the MergeOption-preserving MergeAs call) /// to be retrieved and processed by the ELinq ExpressionConverter. /// ////// The LINQ expression for this ObjectQuery, wrapped in a MergeOption-preserving call /// to the MergeAs method if the ObjectQuery.MergeOption property has been set. /// internal override Expression GetExpression() { // If this ObjectQuery is not backed by a LINQ Expression (it is an ESQL query), // then create a ConstantExpression that uses this ObjectQuery as its value. Expression retExpr; if (!this.QueryState.TryGetExpression(out retExpr)) { retExpr = Expression.Constant(this); } Type objectQueryType = typeof(ObjectQuery); if (this.QueryState.UserSpecifiedMergeOption.HasValue) { MethodInfo mergeAsMethod = objectQueryType.GetMethod("MergeAs", BindingFlags.Instance | BindingFlags.NonPublic); Debug.Assert(mergeAsMethod != null, "Could not retrieve ObjectQuery .MergeAs method using reflection?"); retExpr = TypeSystem.EnsureType(retExpr, objectQueryType); retExpr = Expression.Call(retExpr, mergeAsMethod, Expression.Constant(this.QueryState.UserSpecifiedMergeOption.Value)); } if (null != this.QueryState.Span) { MethodInfo includeSpanMethod = objectQueryType.GetMethod("IncludeSpan", BindingFlags.Instance | BindingFlags.NonPublic); Debug.Assert(includeSpanMethod != null, "Could not retrieve ObjectQuery .IncludeSpan method using reflection?"); retExpr = TypeSystem.EnsureType(retExpr, objectQueryType); retExpr = Expression.Call(retExpr, includeSpanMethod, Expression.Constant(this.QueryState.Span)); } return retExpr; } // Intended for use only in the MethodCallExpression produced for inline queries. internal ObjectQuery MergeAs(MergeOption mergeOption) { throw EntityUtil.InvalidOperation(Strings.ELinq_MethodNotDirectlyCallable); } // Intended for use only in the MethodCallExpression produced for inline queries. internal ObjectQuery IncludeSpan(Span span) { throw EntityUtil.InvalidOperation(Strings.ELinq_MethodNotDirectlyCallable); } #endregion #region Private Methods private ObjectResult GetResults(MergeOption? forMergeOption) { this.QueryState.ObjectContext.EnsureConnection(); try { ObjectQueryExecutionPlan execPlan = this.QueryState.GetExecutionPlan(forMergeOption); return execPlan.Execute (this.QueryState.ObjectContext, this.QueryState.Parameters); } catch { this.QueryState.ObjectContext.ReleaseConnection(); throw; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
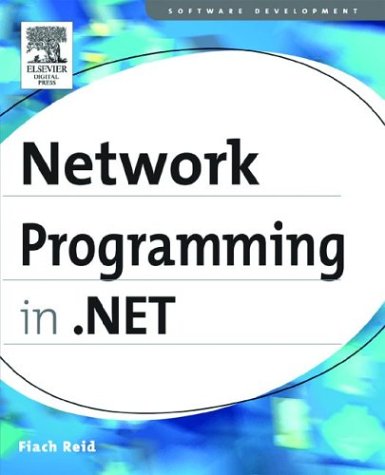
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Validator.cs
- BitmapEffectInput.cs
- ActivityExecutorOperation.cs
- Pens.cs
- NoPersistProperty.cs
- AttributeParameterInfo.cs
- TextElementEnumerator.cs
- CommunicationObjectFaultedException.cs
- QilTernary.cs
- ITreeGenerator.cs
- URLIdentityPermission.cs
- DLinqTableProvider.cs
- LinkLabelLinkClickedEvent.cs
- Size3D.cs
- WebPartDisplayModeEventArgs.cs
- UrlPath.cs
- WebPartDescriptionCollection.cs
- ComponentResourceKeyConverter.cs
- FontNamesConverter.cs
- ResourceDescriptionAttribute.cs
- Evidence.cs
- safex509handles.cs
- Hyperlink.cs
- RequestNavigateEventArgs.cs
- IntegerValidator.cs
- DigitalSignature.cs
- DataGridViewTextBoxCell.cs
- RuntimeConfigLKG.cs
- WebPartsPersonalizationAuthorization.cs
- CompiledAction.cs
- StatusBarItemAutomationPeer.cs
- ThrowHelper.cs
- InputLanguageSource.cs
- PeerCustomResolverElement.cs
- XmlILStorageConverter.cs
- PageThemeCodeDomTreeGenerator.cs
- ToolStripRenderer.cs
- TdsEnums.cs
- CompositeControl.cs
- dataobject.cs
- ProgressiveCrcCalculatingStream.cs
- ProcessHost.cs
- ClientSponsor.cs
- SqlInternalConnectionSmi.cs
- SByte.cs
- EntityException.cs
- EntityCollectionChangedParams.cs
- StoryFragments.cs
- HwndSourceParameters.cs
- NotifyInputEventArgs.cs
- ListManagerBindingsCollection.cs
- TrackingRecord.cs
- FollowerQueueCreator.cs
- HiddenFieldPageStatePersister.cs
- Journaling.cs
- webeventbuffer.cs
- XsltLoader.cs
- WmiInstallComponent.cs
- File.cs
- Debug.cs
- Window.cs
- TimeSpanValidatorAttribute.cs
- ExcCanonicalXml.cs
- AutoResizedEvent.cs
- WindowsIdentity.cs
- AsmxEndpointPickerExtension.cs
- LineMetrics.cs
- XhtmlCssHandler.cs
- IssuanceLicense.cs
- WebUtil.cs
- LayoutUtils.cs
- TextProviderWrapper.cs
- HtmlGenericControl.cs
- EventRoute.cs
- ChildrenQuery.cs
- ADMembershipProvider.cs
- WebPartDisplayModeCancelEventArgs.cs
- MenuItemStyle.cs
- FileUtil.cs
- ReadOnlyDictionary.cs
- CultureInfoConverter.cs
- RuleEngine.cs
- DbConnectionInternal.cs
- RowSpanVector.cs
- ImageDrawing.cs
- CodeTypeConstructor.cs
- MexBindingBindingCollectionElement.cs
- SchemaInfo.cs
- AnimationClock.cs
- TrustLevelCollection.cs
- SrgsSubset.cs
- EnumConverter.cs
- Compress.cs
- ControlCachePolicy.cs
- ComponentResourceKey.cs
- DES.cs
- SmtpNetworkElement.cs
- MenuCommandsChangedEventArgs.cs
- DataGridColumnHeadersPresenter.cs
- Page.cs