Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Principal / WindowsImpersonationContext.cs / 1305376 / WindowsImpersonationContext.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // WindowsImpersonationContext.cs // // Representation of an impersonation context. // namespace System.Security.Principal { using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; #if FEATURE_CORRUPTING_EXCEPTIONS using System.Runtime.ExceptionServices; #endif // FEATURE_CORRUPTING_EXCEPTIONS using System.Security.Permissions; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public class WindowsImpersonationContext : IDisposable { [System.Security.SecurityCritical /*auto-generated*/] private SafeTokenHandle m_safeTokenHandle = SafeTokenHandle.InvalidHandle; private WindowsIdentity m_wi; private FrameSecurityDescriptor m_fsd; [System.Security.SecurityCritical] // auto-generated private WindowsImpersonationContext () {} [System.Security.SecurityCritical] // auto-generated [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] internal WindowsImpersonationContext (SafeTokenHandle safeTokenHandle, WindowsIdentity wi, bool isImpersonating, FrameSecurityDescriptor fsd) { if (safeTokenHandle.IsInvalid) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidImpersonationToken")); Contract.EndContractBlock(); if (isImpersonating) { if (!Win32Native.DuplicateHandle(Win32Native.GetCurrentProcess(), safeTokenHandle, Win32Native.GetCurrentProcess(), ref m_safeTokenHandle, 0, true, Win32Native.DUPLICATE_SAME_ACCESS)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); m_wi = wi; } m_fsd = fsd; } // Revert to previous impersonation (the only public method). [System.Security.SecuritySafeCritical] // auto-generated [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] public void Undo () { int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); if (hr < 0) Environment.FailFast(Win32Native.GetMessage(hr)); } else { hr = Win32.RevertToSelf(); if (hr < 0) Environment.FailFast(Win32Native.GetMessage(hr)); hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); } WindowsIdentity.UpdateThreadWI(m_wi); if (m_fsd != null) m_fsd.SetTokenHandles(null, null); } // Non-throwing version that does not new any exception objects. To be called when reliability matters [System.Security.SecurityCritical] // auto-generated [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] #if FEATURE_CORRUPTING_EXCEPTIONS [HandleProcessCorruptedStateExceptions] // #endif // FEATURE_CORRUPTING_EXCEPTIONS internal bool UndoNoThrow() { bool bRet = false; try{ int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); if (hr < 0) Environment.FailFast(Win32Native.GetMessage(hr)); } else { hr = Win32.RevertToSelf(); if (hr >= 0) { hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); } else { Environment.FailFast(Win32Native.GetMessage(hr)); } } bRet = (hr >= 0); if (m_fsd != null) m_fsd.SetTokenHandles(null,null); } catch { bRet = false; } return bRet; } // // IDisposable interface. // [System.Security.SecuritySafeCritical] // auto-generated [ComVisible(false)] protected virtual void Dispose(bool disposing) { if (disposing) { if (m_safeTokenHandle != null && !m_safeTokenHandle.IsClosed) { Undo(); m_safeTokenHandle.Dispose(); } } } [System.Security.SecuritySafeCritical] // auto-generated [ComVisible(false)] public void Dispose () { Dispose(true); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // WindowsImpersonationContext.cs // // Representation of an impersonation context. // namespace System.Security.Principal { using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; #if FEATURE_CORRUPTING_EXCEPTIONS using System.Runtime.ExceptionServices; #endif // FEATURE_CORRUPTING_EXCEPTIONS using System.Security.Permissions; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public class WindowsImpersonationContext : IDisposable { [System.Security.SecurityCritical /*auto-generated*/] private SafeTokenHandle m_safeTokenHandle = SafeTokenHandle.InvalidHandle; private WindowsIdentity m_wi; private FrameSecurityDescriptor m_fsd; [System.Security.SecurityCritical] // auto-generated private WindowsImpersonationContext () {} [System.Security.SecurityCritical] // auto-generated [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] internal WindowsImpersonationContext (SafeTokenHandle safeTokenHandle, WindowsIdentity wi, bool isImpersonating, FrameSecurityDescriptor fsd) { if (safeTokenHandle.IsInvalid) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidImpersonationToken")); Contract.EndContractBlock(); if (isImpersonating) { if (!Win32Native.DuplicateHandle(Win32Native.GetCurrentProcess(), safeTokenHandle, Win32Native.GetCurrentProcess(), ref m_safeTokenHandle, 0, true, Win32Native.DUPLICATE_SAME_ACCESS)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); m_wi = wi; } m_fsd = fsd; } // Revert to previous impersonation (the only public method). [System.Security.SecuritySafeCritical] // auto-generated [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] public void Undo () { int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); if (hr < 0) Environment.FailFast(Win32Native.GetMessage(hr)); } else { hr = Win32.RevertToSelf(); if (hr < 0) Environment.FailFast(Win32Native.GetMessage(hr)); hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); } WindowsIdentity.UpdateThreadWI(m_wi); if (m_fsd != null) m_fsd.SetTokenHandles(null, null); } // Non-throwing version that does not new any exception objects. To be called when reliability matters [System.Security.SecurityCritical] // auto-generated [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] #if FEATURE_CORRUPTING_EXCEPTIONS [HandleProcessCorruptedStateExceptions] // #endif // FEATURE_CORRUPTING_EXCEPTIONS internal bool UndoNoThrow() { bool bRet = false; try{ int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); if (hr < 0) Environment.FailFast(Win32Native.GetMessage(hr)); } else { hr = Win32.RevertToSelf(); if (hr >= 0) { hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); } else { Environment.FailFast(Win32Native.GetMessage(hr)); } } bRet = (hr >= 0); if (m_fsd != null) m_fsd.SetTokenHandles(null,null); } catch { bRet = false; } return bRet; } // // IDisposable interface. // [System.Security.SecuritySafeCritical] // auto-generated [ComVisible(false)] protected virtual void Dispose(bool disposing) { if (disposing) { if (m_safeTokenHandle != null && !m_safeTokenHandle.IsClosed) { Undo(); m_safeTokenHandle.Dispose(); } } } [System.Security.SecuritySafeCritical] // auto-generated [ComVisible(false)] public void Dispose () { Dispose(true); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
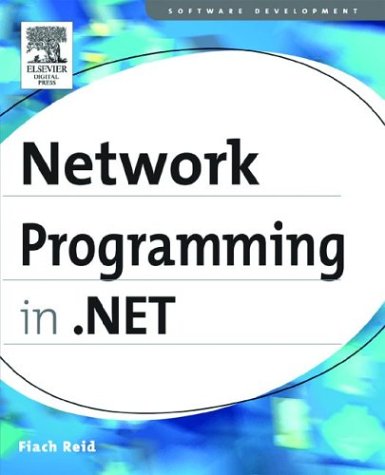
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymmetricKeyWrap.cs
- DataTableReaderListener.cs
- ReadOnlyHierarchicalDataSource.cs
- InteropBitmapSource.cs
- SiteMapPathDesigner.cs
- ReferentialConstraint.cs
- AutomationElement.cs
- ElementUtil.cs
- ZipIOCentralDirectoryFileHeader.cs
- BeginStoryboard.cs
- Point3DCollectionConverter.cs
- ExplicitDiscriminatorMap.cs
- ConstructorBuilder.cs
- Connection.cs
- documentsequencetextpointer.cs
- RestHandlerFactory.cs
- SubtreeProcessor.cs
- WebFormsRootDesigner.cs
- CqlLexer.cs
- FlowchartDesignerCommands.cs
- ArrayWithOffset.cs
- TabRenderer.cs
- StdValidatorsAndConverters.cs
- SerializeAbsoluteContext.cs
- Tuple.cs
- EnumerableRowCollectionExtensions.cs
- StreamMarshaler.cs
- SqlLiftIndependentRowExpressions.cs
- ByeOperationAsyncResult.cs
- TextTreeUndo.cs
- PerformanceCounterManager.cs
- GlobalizationSection.cs
- DriveInfo.cs
- XmlSchemaValidationException.cs
- PersonalizablePropertyEntry.cs
- ToolTipService.cs
- SessionMode.cs
- SqlReferenceCollection.cs
- CssStyleCollection.cs
- SR.cs
- TabRenderer.cs
- ToolStripSplitButton.cs
- Opcode.cs
- AdministrationHelpers.cs
- RegexBoyerMoore.cs
- SecurityDescriptor.cs
- SystemFonts.cs
- SchemaObjectWriter.cs
- SymbolType.cs
- Triplet.cs
- BuildProviderCollection.cs
- ReaderWriterLockSlim.cs
- _SafeNetHandles.cs
- OletxTransactionManager.cs
- SettingsPropertyValue.cs
- EdmSchemaAttribute.cs
- BitStream.cs
- Attributes.cs
- DataGridViewColumnConverter.cs
- CapacityStreamGeometryContext.cs
- OdbcDataReader.cs
- TypeHelpers.cs
- ConfigXmlComment.cs
- FloaterParaClient.cs
- RangeEnumerable.cs
- PersistenceException.cs
- WebPartChrome.cs
- TextEditorDragDrop.cs
- XsdValidatingReader.cs
- Parser.cs
- MailAddress.cs
- BinaryObjectWriter.cs
- InternalBufferOverflowException.cs
- COSERVERINFO.cs
- embossbitmapeffect.cs
- ToolStripRenderEventArgs.cs
- Attachment.cs
- QilPatternVisitor.cs
- CounterSampleCalculator.cs
- InternalPermissions.cs
- DbMetaDataFactory.cs
- PostBackTrigger.cs
- WizardForm.cs
- SimpleTextLine.cs
- TemplateBindingExpressionConverter.cs
- TwoPhaseCommit.cs
- ItemsControlAutomationPeer.cs
- SqlBuilder.cs
- StrokeRenderer.cs
- TrimSurroundingWhitespaceAttribute.cs
- JournalNavigationScope.cs
- SiteMapSection.cs
- TextEditorTables.cs
- OleDbDataReader.cs
- MenuItemStyle.cs
- ResolveRequestResponseAsyncResult.cs
- DownloadProgressEventArgs.cs
- BitmapImage.cs
- InvokePatternIdentifiers.cs
- safex509handles.cs