Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / RequestedSignatureDialog.cs / 1 / RequestedSignatureDialog.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // RequestedSignatureDialog . // // History: // 05/24/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Windows.Forms; using System.Windows.TrustUI; using System.Security; // for SecurityCritical, etc using MS.Internal.PresentationUI; namespace MS.Internal.Documents { internal sealed partial class RequestedSignatureDialog : DialogBaseForm { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Constructor /// internal RequestedSignatureDialog(DocumentSignatureManager docSigManager) { if (docSigManager != null) { //Init private fields _documentSignatureManager = docSigManager; } else { throw new ArgumentNullException("docSigManager"); } // Initialize the "Must Sign By:" field _dateTimePicker.MinDate = DateTime.Now; _dateTimePicker.Value = DateTime.Now.AddDays(10); } #endregion Constructors #region Private Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- ////// oKButton_Click /// ////// Critical - Calls a critical method DocumentSignatureManager::OnAddRequestSignature. /// TreatAsSafe - The data we are passing into the critical call is strictly user supplied text /// directly from the UI (i.e. user typed it), and does not have any affect on /// the actual signing of the document. It is supplemental data that is added to /// the signature and is signed along with the document. Since the user can add /// any arbitrary text they want here and since we are constructing the class that /// is passed locally, this should be fine. /// [SecurityCritical, SecurityTreatAsSafe] private void _addButton_Click(object sender, EventArgs e) { //Check to see this the input is valid if (ValidateUserData()) { //Create SignatureResource to pass back to DocumentSignatureManager SignatureResources sigResources = new SignatureResources(); //Get the user data. sigResources._subjectName = _requestedSignerNameTextBox.Text; sigResources._reason = _intentComboBox.Text; sigResources._location = _requestedLocationTextBox.Text; //Add the SignatureDefinition. _documentSignatureManager.OnAddRequestSignature(sigResources,_dateTimePicker.Value); //Close the Add Request dialog Close(); } else { System.Windows.MessageBox.Show( SR.Get(SRID.DigitalSignatureWarnErrorReadOnlyInputError), SR.Get(SRID.DigitalSignatureWarnErrorSigningErrorTitle), System.Windows.MessageBoxButton.OK, System.Windows.MessageBoxImage.Exclamation ); } } ////// ValidateUserData. Check the user input is valid. /// private bool ValidateUserData() { bool rtnvalue = false; //Remove extra white space. string requestSignerName = _requestedSignerNameTextBox.Text.Trim(); string intentComboBoxText = _intentComboBox.Text.Trim(); //Do the text/combo contain any text? if (!String.IsNullOrEmpty(requestSignerName) && !String.IsNullOrEmpty(intentComboBoxText)) { rtnvalue = true; } return rtnvalue; } #endregion Private Methods #region Private Fields //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ private DocumentSignatureManager _documentSignatureManager; #endregion Private Fields #region Protected Methods //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// ApplyResources /// protected override void ApplyResources() { base.ApplyResources(); //Get localized strings. _addButton.Text = SR.Get(SRID.RequestSignatureDialogAdd); _cancelButton.Text = SR.Get(SRID.RequestSignatureDialogCancel); _requestSignerNameLabel.Text = SR.Get(SRID.RequestSignatureDialogRequestSignerNameLabel); _intentLabel.Text = SR.Get(SRID.RequestSignatureDialogIntentLabel); _requestLocationLabel.Text = SR.Get(SRID.RequestSignatureDialogLocationLabel); _signatureAppliedByDateLabel.Text = SR.Get(SRID.RequestSignatureDialogSignatureAppliedByDateLabel); Text = SR.Get(SRID.RequestSignatureDialogTitle); //Load the Intent/Reason combo _intentComboBox.Items.Add(SR.Get(SRID.DigSigIntentString1)); _intentComboBox.Items.Add(SR.Get(SRID.DigSigIntentString2)); _intentComboBox.Items.Add(SR.Get(SRID.DigSigIntentString3)); _intentComboBox.Items.Add(SR.Get(SRID.DigSigIntentString4)); _intentComboBox.Items.Add(SR.Get(SRID.DigSigIntentString5)); _intentComboBox.Items.Add(SR.Get(SRID.DigSigIntentString6)); } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
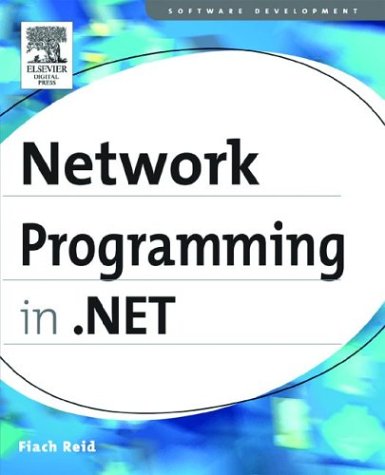
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LabelAutomationPeer.cs
- AutomationEvent.cs
- tabpagecollectioneditor.cs
- HtmlInputCheckBox.cs
- DetailsViewRow.cs
- OneToOneMappingSerializer.cs
- NamedPipeTransportSecurity.cs
- HttpRawResponse.cs
- COM2ExtendedUITypeEditor.cs
- CompensateDesigner.cs
- BamlLocalizableResource.cs
- AnonymousIdentificationSection.cs
- SelectionHighlightInfo.cs
- SoapSchemaImporter.cs
- StyleTypedPropertyAttribute.cs
- ObjectContextServiceProvider.cs
- LayoutEditorPart.cs
- WebPartHelpVerb.cs
- UserControl.cs
- InvokeMethodActivity.cs
- BuildProviderCollection.cs
- EntityContainerRelationshipSet.cs
- ReadOnlyHierarchicalDataSourceView.cs
- LineServicesRun.cs
- EntityRecordInfo.cs
- TitleStyle.cs
- Msmq4SubqueuePoisonHandler.cs
- InvalidDataException.cs
- DateTimeValueSerializerContext.cs
- VirtualPathProvider.cs
- PauseStoryboard.cs
- GeneralTransform3DTo2DTo3D.cs
- ListItemConverter.cs
- InternalBufferOverflowException.cs
- WebPartDeleteVerb.cs
- SqlBulkCopyColumnMapping.cs
- AddInProcess.cs
- SqlClientPermission.cs
- IdentityModelDictionary.cs
- DefaultPropertyAttribute.cs
- DetailsViewRow.cs
- BitmapFrameDecode.cs
- ApplicationSecurityInfo.cs
- DefaultValueAttribute.cs
- ColumnWidthChangingEvent.cs
- GenericWebPart.cs
- EmulateRecognizeCompletedEventArgs.cs
- WorkflowPersistenceContext.cs
- Stackframe.cs
- ParagraphVisual.cs
- ToolStripPanel.cs
- ServiceDefaults.cs
- DbDataSourceEnumerator.cs
- MultiView.cs
- Version.cs
- MetadataArtifactLoaderFile.cs
- CqlIdentifiers.cs
- StorageInfo.cs
- MSG.cs
- MouseButtonEventArgs.cs
- MailDefinition.cs
- BitmapEffectOutputConnector.cs
- BitStream.cs
- XmlSerializerAssemblyAttribute.cs
- ASCIIEncoding.cs
- AxisAngleRotation3D.cs
- Selection.cs
- CancellationToken.cs
- TypeSystem.cs
- StringCollectionEditor.cs
- FunctionDetailsReader.cs
- CodeExpressionCollection.cs
- RepeaterItemEventArgs.cs
- CLSCompliantAttribute.cs
- OdbcFactory.cs
- InfiniteTimeSpanConverter.cs
- HttpHandlerAction.cs
- AddInIpcChannel.cs
- NoClickablePointException.cs
- StyleSheetRefUrlEditor.cs
- XMLDiffLoader.cs
- TableLayoutStyleCollection.cs
- XmlAttributes.cs
- XmlTypeAttribute.cs
- ParamArrayAttribute.cs
- TextPenaltyModule.cs
- FileSecurity.cs
- KeyNotFoundException.cs
- Model3D.cs
- GenericPrincipal.cs
- ExpressionBindingCollection.cs
- ToolStripComboBox.cs
- CompilerGlobalScopeAttribute.cs
- Context.cs
- SchemaMerger.cs
- UIElementIsland.cs
- XmlValidatingReader.cs
- DrawingGroup.cs
- BooleanToVisibilityConverter.cs
- ParameterToken.cs