Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Internal / Ink / Native.cs / 1 / Native.cs
using System; using System.Security; using System.Runtime.InteropServices; namespace MS.Internal.Ink { internal static class Native { ////// Initialize the constants /// ////// Critical: Critical as this code invokes Marshal.SizeOf which uses LinkDemand for UnmanagedCode permission. /// TreatAsSafe: The method doesn't take any user inputs. It only pre-computes the size of our internal types. /// [SecurityCritical, SecurityTreatAsSafe] static Native() { // NOTICE-2005/10/14-WAYNEZEN, // Make sure those lengths are indepentent from the 32bit or 64bit platform. Otherwise it could // break the ISF format. SizeOfInt = (uint)Marshal.SizeOf(typeof(int)); SizeOfUInt = (uint)Marshal.SizeOf(typeof(uint)); SizeOfUShort = (uint)Marshal.SizeOf(typeof(ushort)); SizeOfByte = (uint)Marshal.SizeOf(typeof(byte)); SizeOfFloat = (uint)Marshal.SizeOf(typeof(float)); SizeOfDouble = (uint)Marshal.SizeOf(typeof(double)); SizeOfGuid = (uint)Marshal.SizeOf(typeof(Guid)); SizeOfDecimal = (uint)Marshal.SizeOf(typeof(decimal)); } internal static readonly uint SizeOfInt; // Size of an int internal static readonly uint SizeOfUInt; // Size of an unsigned int internal static readonly uint SizeOfUShort; // Size of an unsigned short internal static readonly uint SizeOfByte; // Size of a byte internal static readonly uint SizeOfFloat; // Size of a float internal static readonly uint SizeOfDouble; // Size of a double internal static readonly uint SizeOfGuid; // Size of a GUID internal static readonly uint SizeOfDecimal; // Size of a VB-style Decimal internal const int BitsPerByte = 8; // number of bits in a byte internal const int BitsPerShort = 16; // number of bits in one short - 2 bytes internal const int BitsPerInt = 32; // number of bits in one integer - 4 bytes internal const int BitsPerLong = 64; // number of bits in one long - 8 bytes // since casting from floats have mantisaa components, // casts from float to int are not constrained by // Int32.MaxValue, but by the maximum float value // whose mantissa component is still within range // of an integer. Anything larger will cause an overflow. internal const int MaxFloatToIntValue = 2147483584 - 1; // 2.14748e+009 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security; using System.Runtime.InteropServices; namespace MS.Internal.Ink { internal static class Native { ////// Initialize the constants /// ////// Critical: Critical as this code invokes Marshal.SizeOf which uses LinkDemand for UnmanagedCode permission. /// TreatAsSafe: The method doesn't take any user inputs. It only pre-computes the size of our internal types. /// [SecurityCritical, SecurityTreatAsSafe] static Native() { // NOTICE-2005/10/14-WAYNEZEN, // Make sure those lengths are indepentent from the 32bit or 64bit platform. Otherwise it could // break the ISF format. SizeOfInt = (uint)Marshal.SizeOf(typeof(int)); SizeOfUInt = (uint)Marshal.SizeOf(typeof(uint)); SizeOfUShort = (uint)Marshal.SizeOf(typeof(ushort)); SizeOfByte = (uint)Marshal.SizeOf(typeof(byte)); SizeOfFloat = (uint)Marshal.SizeOf(typeof(float)); SizeOfDouble = (uint)Marshal.SizeOf(typeof(double)); SizeOfGuid = (uint)Marshal.SizeOf(typeof(Guid)); SizeOfDecimal = (uint)Marshal.SizeOf(typeof(decimal)); } internal static readonly uint SizeOfInt; // Size of an int internal static readonly uint SizeOfUInt; // Size of an unsigned int internal static readonly uint SizeOfUShort; // Size of an unsigned short internal static readonly uint SizeOfByte; // Size of a byte internal static readonly uint SizeOfFloat; // Size of a float internal static readonly uint SizeOfDouble; // Size of a double internal static readonly uint SizeOfGuid; // Size of a GUID internal static readonly uint SizeOfDecimal; // Size of a VB-style Decimal internal const int BitsPerByte = 8; // number of bits in a byte internal const int BitsPerShort = 16; // number of bits in one short - 2 bytes internal const int BitsPerInt = 32; // number of bits in one integer - 4 bytes internal const int BitsPerLong = 64; // number of bits in one long - 8 bytes // since casting from floats have mantisaa components, // casts from float to int are not constrained by // Int32.MaxValue, but by the maximum float value // whose mantissa component is still within range // of an integer. Anything larger will cause an overflow. internal const int MaxFloatToIntValue = 2147483584 - 1; // 2.14748e+009 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
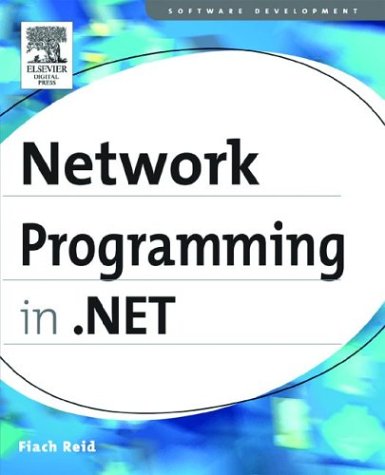
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseGesture.cs
- DataBinder.cs
- SmiEventSink.cs
- CachedPathData.cs
- TimeZone.cs
- XmlDataDocument.cs
- LineSegment.cs
- SchemaNames.cs
- HashStream.cs
- ProviderCommandInfoUtils.cs
- Byte.cs
- MetadataSerializer.cs
- FontDriver.cs
- SecurityDocument.cs
- XamlReader.cs
- DBPropSet.cs
- MetadataItem_Static.cs
- __Error.cs
- AuthenticationConfig.cs
- _CommandStream.cs
- CodeStatement.cs
- DesignerPerfEventProvider.cs
- ReflectionHelper.cs
- Form.cs
- ThreadInterruptedException.cs
- GridViewRowCollection.cs
- BevelBitmapEffect.cs
- FactoryGenerator.cs
- DataSourceXmlSerializationAttribute.cs
- ByteAnimationBase.cs
- PointAnimation.cs
- List.cs
- MultipleViewPatternIdentifiers.cs
- future.cs
- TableLayoutSettingsTypeConverter.cs
- CachedBitmap.cs
- MsmqIntegrationProcessProtocolHandler.cs
- SmtpAuthenticationManager.cs
- MatrixValueSerializer.cs
- NetDataContractSerializer.cs
- ItemCollection.cs
- listitem.cs
- TransactionInterop.cs
- WmpBitmapEncoder.cs
- PostBackTrigger.cs
- XmlSchemaAttributeGroupRef.cs
- TextBounds.cs
- CollectionConverter.cs
- SqlUtils.cs
- IndependentAnimationStorage.cs
- WebPartRestoreVerb.cs
- DecimalAnimationBase.cs
- AmbiguousMatchException.cs
- _Win32.cs
- SystemIcmpV4Statistics.cs
- FileDataSourceCache.cs
- RadioButtonStandardAdapter.cs
- WebBrowserEvent.cs
- Module.cs
- PeerCollaborationPermission.cs
- TransformCollection.cs
- WindowsPrincipal.cs
- HMACSHA1.cs
- keycontainerpermission.cs
- XmlWellformedWriter.cs
- GeneratedView.cs
- ExpressionNode.cs
- StateRuntime.cs
- ParallelSeparator.xaml.cs
- CustomBindingElementCollection.cs
- ImageBrush.cs
- OpacityConverter.cs
- ContainerUtilities.cs
- SiteIdentityPermission.cs
- XmlSchemaAnyAttribute.cs
- DebugViewWriter.cs
- DescendantBaseQuery.cs
- PassportAuthentication.cs
- StackSpiller.Bindings.cs
- SqlTypeSystemProvider.cs
- AlphabeticalEnumConverter.cs
- StrokeCollection.cs
- MulticastOption.cs
- AssemblySettingAttributes.cs
- Label.cs
- JsonMessageEncoderFactory.cs
- FlowStep.cs
- DiscoveryMessageSequenceGenerator.cs
- MediaTimeline.cs
- HttpHandlersSection.cs
- HMACSHA384.cs
- CollectionBase.cs
- XmlValidatingReaderImpl.cs
- VisualState.cs
- UshortList2.cs
- AuthenticationException.cs
- TransformCollection.cs
- QilReference.cs
- CodeIdentifiers.cs
- ResourceDisplayNameAttribute.cs