Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / NavigationHelper.cs / 1 / NavigationHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // NavigationHelper is an internal utility class for Mongoose to deal // with Uri navigations. // // History: // 11/10/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Security; using System.Windows.Interop; using System.Windows.TrustUI; using MS.Internal; using MS.Internal.PresentationUI; namespace MS.Internal.Documents.Application { ////// Helper class for handling browser navigations. /// internal static class NavigationHelper { ////////// Invokes a navigation to a new document ///// ////// Critical: /// - uses delegate from framework /// - uses Document.Uri /// [SecurityCritical] internal static void NavigateToDocument(Document document) { Trace.SafeWrite( Trace.File, "Attempting to navigate to new document {0}.", document.Uri); Invariant.Assert( _navigate != null, "Navigation object has not been instantiated."); Invariant.Assert( _navigate.Value != null, "Navigation delegate has not been assigned."); Invariant.Assert( document != null, "Target document has not been assigned."); _navigate.Value(new SecurityCriticalData(document.Uri)); } ///// ///// Invokes a top-level browserNavigation action to the specified Uri. ///// ////////// Critical: ///// - causes a navigation action to occur to an external uri ///// - passes the Uri to another method ///// [SecurityCritical] internal static void NavigateToExternalUri(Uri uri) { Trace.SafeWrite( Trace.File, "Attempting to navigate to external Uri {0}.", uri); Invariant.Assert( _navigate != null, "Navigation object has not been instantiated."); Invariant.Assert( _navigate.Value != null, "Navigation delegate has not been assigned."); Invariant.Assert( uri != null, "Target uri has not been assigned."); _navigate.Value(new SecurityCriticalData(uri)); } /// /// A delegate that will navigate the root browser window. /// ////// Critical: /// - sets _navigate which should only be BrowserInteropHelper.Navigate /// ////// If we are going to add more functionality a IBrowserService interface /// of some type should be defined and set vs many delegates. /// [FriendAccessAllowed] internal static NavigateDelegate Navigate { [SecurityCritical] get { if (_navigate != null) { return _navigate.Value; } return null; } [SecurityCritical] set { _navigate = new SecurityCriticalDataClass(value); } } internal delegate void NavigateDelegate(SecurityCriticalData uri); private static SecurityCriticalDataClass _navigate; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
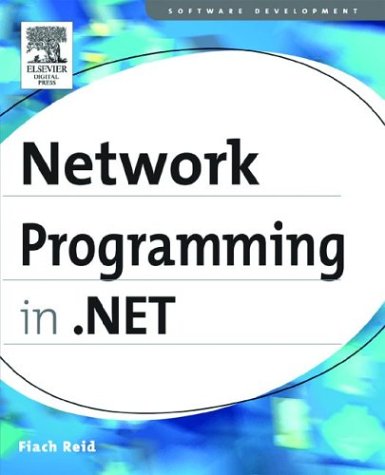
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthorizationRule.cs
- TextBoxAutomationPeer.cs
- DataRowComparer.cs
- HttpAsyncResult.cs
- ZipIOExtraFieldPaddingElement.cs
- SyndicationSerializer.cs
- ProviderIncompatibleException.cs
- SortedDictionary.cs
- Label.cs
- TransactedBatchingElement.cs
- HttpGetClientProtocol.cs
- SqlDataSourceView.cs
- FontNamesConverter.cs
- HandlerElement.cs
- CustomLineCap.cs
- ThumbAutomationPeer.cs
- mda.cs
- ByteFacetDescriptionElement.cs
- UserNamePasswordValidator.cs
- BufferedGraphics.cs
- AssemblyAttributesGoHere.cs
- NativeMethods.cs
- LayoutTableCell.cs
- TrueReadOnlyCollection.cs
- RunInstallerAttribute.cs
- ImageIndexConverter.cs
- DropSource.cs
- DataSourceViewSchemaConverter.cs
- XmlSchemaSimpleTypeUnion.cs
- CachedRequestParams.cs
- HyperLinkStyle.cs
- EntityKeyElement.cs
- FixedSOMPageConstructor.cs
- XmlTextEncoder.cs
- ConvertTextFrag.cs
- BaseResourcesBuildProvider.cs
- XmlArrayItemAttribute.cs
- Point3D.cs
- UIElementIsland.cs
- EntityCollectionChangedParams.cs
- DiscoveryServerProtocol.cs
- XsdCachingReader.cs
- ExtendedPropertyDescriptor.cs
- SystemIPGlobalStatistics.cs
- BoundingRectTracker.cs
- SqlStream.cs
- PassportPrincipal.cs
- RequestBringIntoViewEventArgs.cs
- ResourcesGenerator.cs
- ReflectTypeDescriptionProvider.cs
- XmlText.cs
- ArrangedElement.cs
- DataObject.cs
- InvalidWMPVersionException.cs
- Propagator.cs
- BamlVersionHeader.cs
- SqlParameterizer.cs
- SafeThemeHandle.cs
- DesignerActionHeaderItem.cs
- FormViewModeEventArgs.cs
- isolationinterop.cs
- WindowsAuthenticationEventArgs.cs
- HttpListenerContext.cs
- TypeUsageBuilder.cs
- RoleManagerModule.cs
- ObjRef.cs
- CalendarDesigner.cs
- QueryUtil.cs
- WindowsSolidBrush.cs
- ActivityCompletionCallbackWrapper.cs
- SweepDirectionValidation.cs
- Thread.cs
- SqlCacheDependencySection.cs
- DiagnosticTrace.cs
- AuthenticationSection.cs
- WebRequestModuleElement.cs
- GuidelineCollection.cs
- FixedSOMTableCell.cs
- GPPOINTF.cs
- DataGridViewRowEventArgs.cs
- ToolStripStatusLabel.cs
- DecodeHelper.cs
- PropertyTabAttribute.cs
- ArgumentValue.cs
- CheckBoxDesigner.cs
- LogEntryUtils.cs
- DataGridViewTextBoxCell.cs
- InheritanceRules.cs
- basenumberconverter.cs
- ComponentEditorForm.cs
- KerberosSecurityTokenProvider.cs
- ObjectQuery_EntitySqlExtensions.cs
- RequestResizeEvent.cs
- ProcessHostMapPath.cs
- Quaternion.cs
- AttributeProviderAttribute.cs
- QuaternionRotation3D.cs
- XamlReaderHelper.cs
- EntityViewGenerationConstants.cs
- ProfilePropertyMetadata.cs