Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintSystemExceptions / PrintSystemException.cs / 1 / PrintSystemException.cs
/*++ Copyright (C) 2002 - 2003 Microsoft Corporation All rights reserved. Module Name: PrintSystemException.cs Abstract: Print System exception objects declaration. Author: [....] June 3rd 2003 Revision History: --*/ using System; using System.Collections; using System.Runtime.InteropServices; using System.Text; using System.Security; //SecurityCritical using System.Collections.ObjectModel; using System.Collections.Generic; using MS.Internal.PrintWin32Thunk.Win32ApiThunk; namespace System.Printing { abstract internal class PrinterHResult { ////// /// public static int HResultFromWin32( int win32ErrorCode ) { return (win32ErrorCode <= 0) ? win32ErrorCode : (int)((win32ErrorCode & unchecked((int)0x80000000)) | ((int)Facility.Win32 << 16) | unchecked((int)0x80000000)); } ////// /// public static int HResultCode( int errorCode ) { return ((errorCode) & 0xFFFF); } ////// /// public static Facility HResultFacility( int errorCode ) { return (Facility)(((errorCode) >> 16) & 0x1fff); } public enum Error { PrintSystemGenericError = (int)1801, //ERROR_INVALID_PRINTER_NAME, PrintingCancelledGenericError = (int)1223, //ERROR_CANCELLED, }; public enum Facility { Win32 = 7, }; } ////// Print System exception object. /// ///[System.Serializable] public class PrintSystemException : SystemException { /// /// PrintSystemException constructor. /// ////// Default message: Print System exception. /// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintSystemException( ) : base(GetMessageFromResource("PrintSystemException.Generic")) { base.HResult = PrinterHResult.HResultFromWin32((int)PrinterHResult.Error.PrintSystemGenericError); } ////// PrintSystemException constructor. /// /// /// Message that describes the current exception. Must be localized. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintSystemException( String message ) : base(GetMessageFromResource(PrinterHResult.HResultFromWin32((int)PrinterHResult.Error.PrintSystemGenericError), message)) { base.HResult = PrinterHResult.HResultFromWin32((int)PrinterHResult.Error.PrintSystemGenericError); } ////// PrintSystemException constructor. /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintSystemException( String message, Exception innerException ) : base (GetMessageFromResource(PrinterHResult.HResultFromWin32((int)PrinterHResult.Error.PrintSystemGenericError), message), innerException) { base.HResult = PrinterHResult.HResultFromWin32((int)PrinterHResult.Error.PrintSystemGenericError); } ////// Sets the SerializationInfo with information about the exception. /// ////// Inherited from Exception. /// /// Holds the serialized object data about the exception being thrown. /// The contextual information about the source or destination. ////// Critical: calls Exception.GetObjectData which LinkDemands /// PublicOK: a demand exists here /// [SecurityCritical] [System.Security.Permissions.SecurityPermissionAttribute( System.Security.Permissions.SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) { base.GetObjectData(info, context); } ////// /// public PrintSystemException( int errorCode, String message ) : base(GetMessageFromResource(errorCode,message)) { base.HResult = errorCode; } ////// /// public PrintSystemException( int errorCode, String message, String printerMessage ) : base(GetMessageFromResource(errorCode,message) + printerMessage) { base.HResult = errorCode; } ////// /// public PrintSystemException( int errorCode, String message, Exception innerException ) : base(GetMessageFromResource(errorCode,message), innerException) { base.HResult = errorCode; } ////// Initializes a new instance of the PrintQueueException class with serialized data. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected PrintSystemException( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) : base(info, context) { } ////// Loads the resource string for a given resource key. /// /// Resource key. private static String GetMessageFromResource( String resourceKey ) { return printResourceManager.GetString(resourceKey, System.Threading.Thread.CurrentThread.CurrentUICulture); } ////// Loads the resource string for a Win32 error and /// formats an error message given a resource key. /// /// Win32 error code. /// Resource key. private static String GetMessageFromResource( int errorCode, String resourceKey ) { String exceptionMessage = null; String resourceString = printResourceManager.GetString(resourceKey, System.Threading.Thread.CurrentThread.CurrentUICulture); if (PrinterHResult.HResultFacility(errorCode) == PrinterHResult.Facility.Win32) { exceptionMessage = String.Format(System.Threading.Thread.CurrentThread.CurrentUICulture, resourceString, GetFormattedWin32Error(PrinterHResult.HResultCode(errorCode))); } else { exceptionMessage = String.Format(System.Threading.Thread.CurrentThread.CurrentUICulture, resourceString, errorCode); } return exceptionMessage; } ////// /// ////// Critical - calls Win32 FormatMessage API /// TreatAsSafe - FormatMessage is innocuous by nature. /// [System.Security.SecurityCritical, System.Security.SecurityTreatAsSafe] private static String GetFormattedWin32Error( int win32Error ) { StringBuilder win32ErrorMessage = new StringBuilder(defaultWin32ErrorMessageLength); int length = NativeMethodsForPrintExceptions.InvokeFormatMessage(FormatMessageFromSystem, IntPtr.Zero, win32Error, 0, win32ErrorMessage, win32ErrorMessage.Capacity, IntPtr.Zero); int error = Marshal.GetLastWin32Error(); return win32ErrorMessage.ToString(); } ////// /// static PrintSystemException( ) { printResourceManager = new System.Resources.ResourceManager("System.Printing", (typeof(PrintSystemException)).Assembly); } ////// /// static System.Resources.ResourceManager printResourceManager; const int defaultWin32ErrorMessageLength = 256; const int FormatMessageFromSystem = unchecked((int)0x00001000); }; ////// PrintQueueException exception object. /// Exceptions of type PrintQueueException are thrown when operating on a /// ///PrintQueue object. ///[System.Serializable] public class PrintQueueException : PrintSystemException { /// /// PrintQueueException constructor. /// ////// Default message: An exception occurred while creating the PrintQueue object. Win32 error: {0} /// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintQueueException( ): base("PrintSystemException.PrintQueue.Generic") { this.printerName = null; } ////// PrintQueueException constructor. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// /// /// Message that describes the current exception. Must be localized. /// public PrintQueueException( String message ) : base (message) { this.printerName = null; } ////// PrintQueueException constructor. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// public PrintQueueException( String message, Exception innerException ): base(message, innerException) { this.printerName = null; } ////// Printer name property. The name represents the name identifier of /// the PrintQueue object that was running the code when the exception was thrown. /// public String PrinterName { get { return printerName; } } ////// Sets the SerializationInfo with information about the exception. /// ////// Inherited from Exception. /// /// Holds the serialized object data about the exception being thrown. /// The contextual information about the source or destination. ////// SecurityOK - This demand was added to satisfy FxCop rule where override methods should demand /// same permissions as base methods /// [System.Security.Permissions.SecurityPermissionAttribute( System.Security.Permissions.SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) { if (info != null) { info.AddValue("PrinterName", printerName); } base.GetObjectData(info, context); } ////// /// public PrintQueueException( int errorCode, String message, String printerName ) : base(errorCode, message) { this.printerName = printerName; base.HResult = errorCode; } ////// /// public PrintQueueException( int errorCode, String message, String printerName, String printerMessage ) : base(errorCode, message, printerMessage) { this.printerName = printerName; base.HResult = errorCode; } ////// /// public PrintQueueException( int errorCode, String message, String printerName, Exception innerException ) : base(errorCode, message, innerException) { this.printerName = printerName; base.HResult = errorCode; } ////// Initializes a new instance of the PrintQueueException class with serialized data. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected PrintQueueException( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) : base(info, context) { this.printerName = (String)(info.GetValue("PrinterName", typeof(System.String))); } private String printerName; }; ////// PrintServerException constructor. /// Exceptions of type PrintServerException are thrown when operating on a /// ///PrintServer object. ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// [System.Serializable] public class PrintServerException : PrintSystemException { ////// PrintQueueException constructor. /// ////// Default message: An exception occurred while creating the PrintServer object. Win32 error is: {0} /// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintServerException( ): base ("PrintSystemException.PrintServer.Generic") { this.serverName = null; } ////// PrintServerException constructor. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// /// /// Message that describes the current exception. Must be localized. /// public PrintServerException( String message ): base(message) { this.serverName = null; } ////// PrintServerException constructor. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// public PrintServerException( String message, Exception innerException ): base(message, innerException) { this.serverName = null; } ////// Server name property. The name represents the name identifier of /// the PrintServer object that was running the code when the exception was thrown. /// public String ServerName { get { return serverName; } } ////// Sets the SerializationInfo with information about the exception. /// ////// Inherited from Exception. /// /// Holds the serialized object data about the exception being thrown. /// The contextual information about the source or destination. ////// SecurityOK - This demand was added to satisfy FxCop rule where override methods should demand /// same permissions as base methods /// [System.Security.Permissions.SecurityPermissionAttribute( System.Security.Permissions.SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) { if (info != null) { info.AddValue("ServerName", serverName); } base.GetObjectData(info, context); } ////// /// public PrintServerException( int errorCode, String message, String serverName ): base(errorCode, message) { this.serverName = serverName; base.HResult = errorCode; } ////// /// public PrintServerException( int errorCode, String message, String serverName, Exception innerException ): base(errorCode, message, innerException) { this.serverName = serverName; base.HResult = errorCode; } ////// Initializes a new instance of the PrintServerException class with serialized data. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected PrintServerException( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ): base(info, context) { this.serverName = (String)(info.GetValue("ServerName", typeof(String))); } private String serverName; }; ////// PrintCommitAttributesException constructor. /// Exceptions of type PrintCommitAttributesException are thrown /// when Commit method of any of the Print System objects that implement the method. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// [System.Serializable] public class PrintCommitAttributesException : PrintSystemException { ////// PrintCommitAttributesException constructor. /// ////// Default message: Print System exception. /// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintCommitAttributesException( ): base("PrintSystemException.CommitPrintSystemAttributesException") { committedAttributes = new Collection(); failedAttributes = new Collection (); printObjectName = null; } /// /// PrintCommitAttributesException constructor. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// /// /// Message that describes the current exception. Must be localized. /// public PrintCommitAttributesException( String message ): base(message) { printObjectName = null; committedAttributes = new Collection(); failedAttributes = new Collection (); } /// /// PrintCommitAttributesException constructor. /// ////// Default error code: ERROR_INVALID_PRINTER_NAME. /// public PrintCommitAttributesException( String message, Exception innerException ): base(message, innerException) { printObjectName = null; committedAttributes = new Collection(); failedAttributes = new Collection (); } /// /// PrintSystemObject name property. The name represents the name identifier of /// the PrintSystemObject object that was running the code when the exception was thrown. /// public String PrintObjectName { get { return printObjectName; } } ////// Sets the SerializationInfo with information about the exception. /// ////// Inherited from Exception. /// /// Holds the serialized object data about the exception being thrown. /// The contextual information about the source or destination. ////// SecurityOK - This demand was added to satisfy FxCop rule where override methods should demand /// same permissions as base methods /// [System.Security.Permissions.SecurityPermissionAttribute( System.Security.Permissions.SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) { if (info != null) { info.AddValue("CommittedAttributes", committedAttributes); info.AddValue("FailedAttributes", failedAttributes); info.AddValue("ObjectName", printObjectName); } base.GetObjectData(info, context); } ////// /// public PrintCommitAttributesException( int errorCode, CollectionattributesSuccessList, Collection attributesFailList ): base(errorCode, "PrintSystemException.CommitPrintSystemAttributesException") { this.committedAttributes = attributesSuccessList; this.failedAttributes = attributesFailList; this.printObjectName = null; } /// /// /// public PrintCommitAttributesException( int errorCode, String message, CollectionattributesSuccessList, Collection attributesFailList, String objectName ) : base(errorCode,message) { this.committedAttributes = attributesSuccessList; this.failedAttributes = attributesFailList; this.printObjectName = null; } /// /// /// public CollectionCommittedAttributesCollection { get { return committedAttributes; } } /// /// /// public CollectionFailedAttributesCollection { get { return failedAttributes; } } /// /// Initializes a new instance of the PrintCommitAttributesException class with serialized data. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected PrintCommitAttributesException( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ): base(info, context) { committedAttributes = (Collection)(info.GetValue("CommittedAttributes", committedAttributes.GetType())); failedAttributes = (Collection )(info.GetValue("FailedAttributes", failedAttributes.GetType())); printObjectName = (String)(info.GetValue("ObjectName", printObjectName.GetType())); } Collection committedAttributes; Collection failedAttributes; String printObjectName; }; /// /// PrintJobException exception object. /// Exceptions of type PrintJobException submitting a print job to a PrintQueue /// ///PrintQueue object. ///[System.Serializable] public class PrintJobException : PrintSystemException { /// /// PrintJobException constructor. /// public PrintJobException( ) { this.jobId = 0; this.printQueueName = null; this.jobContainer = null; } ////// PrintJobException constructor. /// /// /// Message that describes the current exception. Must be localized. /// public PrintJobException( String message ): base(message) { this.jobId = 0; this.printQueueName = null; this.jobContainer = null; } ////// PrintJobException constructor. /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// public PrintJobException( String message, Exception innerException ) : base(message, innerException) { this.jobId = 0; this.printQueueName = null; this.jobContainer = null; } ////// Job identifier /// public int JobId { get { return jobId; } } ////// Job name /// public String JobName { get { return jobContainer; } } ////// Printer name /// public String PrintQueueName { get { return printQueueName; } } ////// Sets the SerializationInfo with information about the exception. /// ////// Inherited from Exception. /// /// Holds the serialized object data about the exception being thrown. /// The contextual information about the source or destination. ////// SecurityOK - This demand was added to satisfy FxCop rule where override methods should demand /// same permissions as base methods /// [System.Security.Permissions.SecurityPermissionAttribute( System.Security.Permissions.SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) { if( info != null ) { info.AddValue("JobId", jobId ); } base.GetObjectData(info, context); } ////// PrintJobException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// public PrintJobException( int errorCode, String message ) : base(errorCode, (message)) { this.jobId = 0; this.printQueueName = null; this.jobContainer = null; } ////// PrintJobException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// /// /// Job identifier /// /// /// Job name /// /// /// Printer name /// public PrintJobException( int errorCode, String message, String printQueueName, String jobName, int jobId ) : base(errorCode, (message)) { this.printQueueName = printQueueName; this.jobContainer = jobName; this.jobId = jobId; } ////// PrintJobException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// /// /// Job identifier /// /// /// Job name /// /// /// Printer name /// /// /// The exception instance that caused the current exception. /// public PrintJobException( int errorCode, String message, String printQueueName, String jobName, int jobId, Exception innerException ) : base(errorCode, (message), innerException) { this.printQueueName = printQueueName; this.jobContainer = jobName; this.jobId = jobId; } ////// PrintJobException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// public PrintJobException( int errorCode, String message, Exception innerException ) : base(errorCode, message, innerException) { this.jobId = 0; this.printQueueName = null; this.jobContainer = null; } ////// Initializes a new instance of the PrintSystemException class with serialized data. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected PrintJobException( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) : base(info, context) { this.jobId = (int)(info.GetValue("JobId", typeof(int))); } int jobId; String printQueueName; String jobContainer; }; ////// PrintingCanceledException exception object. /// Exceptions of type PrintingCanceledException are thrown when the user cancels /// a printing operation. /// ///[System.Serializable] public class PrintingCanceledException : PrintJobException { /// /// PrintingCanceledException constructor. /// ////// Default message: Printing has been cancelled. Win32 error is {0} /// Default error code: ERROR_CANCELLED. /// public PrintingCanceledException( ) : base(PrinterHResult.HResultFromWin32((int)PrinterHResult.Error.PrintingCancelledGenericError), "PrintSystemException.PrintingCancelled.Generic") { } ////// PrintingCanceledException constructor. /// /// /// Message that describes the current exception. Must be localized. /// public PrintingCanceledException( String message ) : base(message) { } ////// PrintingCanceledException constructor. /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// public PrintingCanceledException( String message, Exception innerException ) : base(message, innerException) { } ////// PrintingCanceledException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// public PrintingCanceledException( int errorCode, String message ) : base(errorCode, message) { } ////// PrintingCanceledException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// /// /// The exception instance that caused the current exception. /// public PrintingCanceledException( int errorCode, String message, Exception innerException ) : base(errorCode, message, innerException) { } ////// PrintingCanceledException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// /// /// Printer name /// /// /// Job name /// /// /// Job identifier /// public PrintingCanceledException( int errorCode, String message, String printQueueName, String jobName, int jobId ) : base(errorCode, message, printQueueName, jobName, jobId) { } ////// PrintingCanceledException constructor. /// /// /// HRESULT error code /// /// /// Message that describes the current exception. Must be localized. /// /// /// Printer name /// /// /// Job name /// /// /// Job identifier /// /// /// The exception instance that caused the current exception. /// public PrintingCanceledException( int errorCode, String message, String printQueueName, String jobName, int jobId, Exception innerException ) : base(errorCode, message, printQueueName, jobName, jobId, innerException) { } ////// Initializes a new instance of the PrintingCanceledException class with serialized data. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected PrintingCanceledException( System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context ) : base(info, context) { } }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
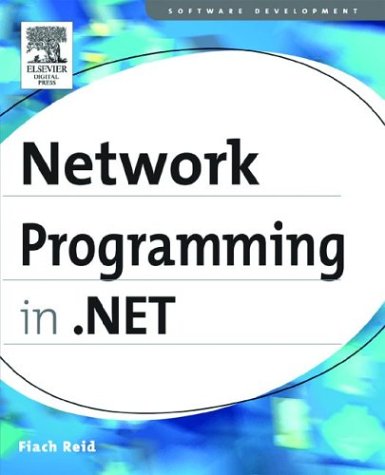
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateBoldEvent.cs
- CompiledQueryCacheEntry.cs
- ComponentEvent.cs
- CultureTableRecord.cs
- ProfilePropertySettings.cs
- XamlPathDataSerializer.cs
- ObjectManager.cs
- CryptoConfig.cs
- dsa.cs
- XmlWhitespace.cs
- TcpPortSharing.cs
- WorkflowPrinting.cs
- ClientBuildManager.cs
- ButtonBaseDesigner.cs
- BuiltInExpr.cs
- ReflectTypeDescriptionProvider.cs
- ValidationEventArgs.cs
- OperationCanceledException.cs
- FunctionDetailsReader.cs
- DataServiceBehavior.cs
- WmlPanelAdapter.cs
- OdbcInfoMessageEvent.cs
- XsltOutput.cs
- KnownBoxes.cs
- PersonalizablePropertyEntry.cs
- HexParser.cs
- CodeArrayIndexerExpression.cs
- DNS.cs
- ItemsControl.cs
- QueryStringParameter.cs
- ListViewItemCollectionEditor.cs
- DrawingBrush.cs
- XPathNodeIterator.cs
- ApplicationSecurityInfo.cs
- NonClientArea.cs
- ColorTranslator.cs
- recordstate.cs
- XmlUrlResolver.cs
- RegexNode.cs
- ExpressionNormalizer.cs
- HtmlCalendarAdapter.cs
- WorkflowShape.cs
- FixedElement.cs
- Utils.cs
- WinFormsUtils.cs
- DataServiceResponse.cs
- UnsafeNativeMethods.cs
- PropertyValue.cs
- DivideByZeroException.cs
- RoleManagerEventArgs.cs
- GCHandleCookieTable.cs
- ContractHandle.cs
- ScriptingSectionGroup.cs
- BigInt.cs
- HtmlTableCell.cs
- UidManager.cs
- Pens.cs
- NodeFunctions.cs
- ClientSponsor.cs
- BuildProviderAppliesToAttribute.cs
- XmlSchemaSimpleContentExtension.cs
- URLString.cs
- X509ClientCertificateAuthenticationElement.cs
- SingleSelectRootGridEntry.cs
- FlowDocumentFormatter.cs
- FlatButtonAppearance.cs
- Icon.cs
- OracleConnectionStringBuilder.cs
- Compiler.cs
- DocumentXmlWriter.cs
- RenderingBiasValidation.cs
- ProcessHostMapPath.cs
- SchemaElementLookUpTableEnumerator.cs
- SafeLibraryHandle.cs
- ColorConvertedBitmap.cs
- ScriptBehaviorDescriptor.cs
- Parsers.cs
- RoleGroup.cs
- ListBoxAutomationPeer.cs
- NameValuePermission.cs
- XmlSchemaSimpleType.cs
- XmlWellformedWriter.cs
- TextTrailingCharacterEllipsis.cs
- AttachedPropertyBrowsableAttribute.cs
- SHA256.cs
- AnimationClockResource.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- XmlEventCache.cs
- PreviewPrintController.cs
- AnnouncementService.cs
- InvokePattern.cs
- MethodExpression.cs
- ObsoleteAttribute.cs
- KeyValueSerializer.cs
- ColorAnimation.cs
- InheritanceAttribute.cs
- BamlBinaryReader.cs
- EventItfInfo.cs
- EnumerableRowCollectionExtensions.cs
- PermissionRequestEvidence.cs