Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PageScaling.cs / 1 / PageScaling.cs
/*++ Copyright (C) 2003 Microsoft Corporation All rights reserved. Module Name: PageScaling.cs Abstract: Definition and implementation of this public feature/parameter related types. Author: [....] ([....]) 7/24/2003 --*/ using System; using System.IO; using System.Xml; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Text; using System.Printing; using MS.Internal.Printing.Configuration; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages namespace MS.Internal.Printing.Configuration { ////// Represents a scaling option. /// internal class ScalingOption: PrintCapabilityOption { #region Constructors internal ScalingOption(PrintCapabilityFeature ownerFeature) : base(ownerFeature) { _value = PageScaling.Unspecified; // We use negative index value to indicate that the custom option's // ParameterRef element hasn't been parsed and accepted yet. _scaleWIndex = _scaleHIndex = _squareScaleIndex = -1; } #endregion Constructors #region Public Properties ////// Gets the page scaling option's value. /// public PageScaling Value { get { return _value; } } ////// Gets the ///object that specifies the custom scaling option's scaling percentage capability in the width direction. /// /// For non-custom scaling options, this property's value is always null. /// public ScalingScaleWidthCapability CustomScaleWidth { get { if (_scaleWIndex < 0) return null; #if _DEBUG Trace.Assert(Value == PageScaling.Custom, "THIS SHOULD NOT HAPPEN: non-Custom scaling option has ScaleWidth"); #endif return (ScalingScaleWidthCapability)(this.OwnerFeature.OwnerPrintCap._pcLocalParamDefs[_scaleWIndex]); } } ////// Gets the ///object that specifies the custom scaling option's scaling percentage capability in the height direction. /// /// For non-custom scaling options, this property's value is always null. /// public ScalingScaleHeightCapability CustomScaleHeight { get { if (_scaleHIndex < 0) return null; #if _DEBUG Trace.Assert(Value == PageScaling.Custom, "THIS SHOULD NOT HAPPEN: non-Custom scaling option has ScaleHeight"); #endif return (ScalingScaleHeightCapability)(this.OwnerFeature.OwnerPrintCap._pcLocalParamDefs[_scaleHIndex]); } } public ScalingSquareScaleCapability CustomSquareScale { get { if (_squareScaleIndex < 0) return null; return (ScalingSquareScaleCapability)(this.OwnerFeature.OwnerPrintCap._pcLocalParamDefs[_squareScaleIndex]); } } #endregion Public Properties #region Public Methods ////// Converts the scaling option to human-readable string. /// ///A string that represents this scaling option. public override string ToString() { return Value.ToString() + ": " + ((CustomScaleWidth != null) ? CustomScaleWidth.ToString() : "null") + " x " + ((CustomScaleHeight != null) ? CustomScaleHeight.ToString() : "null") + " x " + ((CustomSquareScale != null) ? CustomSquareScale.ToString() : "null"); } #endregion Public Methods #region Internal Fields internal PageScaling _value; internal int _scaleWIndex, _scaleHIndex, _squareScaleIndex; #endregion Internal Fields } ////// Represents page scaling capability. /// internal class PageScalingCapability : PrintCapabilityFeature { #region Constructors internal PageScalingCapability(InternalPrintCapabilities ownerPrintCap) : base(ownerPrintCap) { } #endregion Constructors #region Public Properties ////// Gets the collection object that represents page scaling options supported by the device. /// public CollectionScalingOptions { get { return _scalingOptions; } } #endregion Public Properties #region Internal Methods internal static PrintCapabilityFeature NewFeatureCallback(InternalPrintCapabilities printCap) { PageScalingCapability cap = new PageScalingCapability(printCap); cap._scalingOptions = new Collection (); return cap; } internal override sealed bool AddOptionCallback(PrintCapabilityOption baseOption) { bool complete = false; ScalingOption option = baseOption as ScalingOption; if (option._optionName != null) { int enumValue = PrintSchemaMapper.SchemaNameToEnumValueWithArray( PrintSchemaTags.Keywords.PageScalingKeys.ScalingNames, PrintSchemaTags.Keywords.PageScalingKeys.ScalingEnums, option._optionName); if (enumValue > 0) { option._value = (PageScaling)enumValue; } } if (option.Value != PageScaling.Unspecified) { if (option.Value == PageScaling.None) { // Non-custom scaling option doesn't need any ParameterRefs complete = true; } else if (option.Value == PageScaling.Custom) { // Custom scaling option must have the 2 scale ParameterRefs if ((option._scaleWIndex >= 0) && (option._scaleHIndex >= 0)) { complete = true; } else { // Need to reset the local parameter required flag since we are // ignoring the custom scaling option if (option._scaleWIndex >= 0) { option.OwnerFeature.OwnerPrintCap.SetLocalParameterDefAsRequired(option._scaleWIndex, false); } if (option._scaleHIndex >= 0) { option.OwnerFeature.OwnerPrintCap.SetLocalParameterDefAsRequired(option._scaleHIndex, false); } } } else if (option.Value == PageScaling.CustomSquare) { // Custom square scaling option must have the scale ParameterRef if (option._squareScaleIndex >= 0) { complete = true; } else { // Need to reset the local parameter required flag since we are // ignoring the custom scaling option if (option._squareScaleIndex >= 0) { option.OwnerFeature.OwnerPrintCap.SetLocalParameterDefAsRequired(option._squareScaleIndex, false); } } } } if (complete) { this.ScalingOptions.Add(option); } return complete; } internal override sealed void AddSubFeatureCallback(PrintCapabilityFeature subFeature) { // PageScaling has no sub features. return; } internal override sealed bool FeaturePropCallback(PrintCapabilityFeature feature, XmlPrintCapReader reader) { // no feature property to handle return false; } internal override sealed PrintCapabilityOption NewOptionCallback(PrintCapabilityFeature baseFeature) { ScalingOption newOption = new ScalingOption(baseFeature); return newOption; } internal override sealed void OptionAttrCallback(PrintCapabilityOption baseOption, XmlPrintCapReader reader) { // no option attribute to handle return; } /// XML is not well-formed. internal override sealed bool OptionPropCallback(PrintCapabilityOption baseOption, XmlPrintCapReader reader) { bool handled = false; ScalingOption option = baseOption as ScalingOption; if (reader.CurrentElementNodeType == PrintSchemaNodeTypes.ScoredProperty) { handled = true; string sPropertyName = reader.CurrentElementNameAttrValue; if ( (sPropertyName == PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleWidth) || (sPropertyName == PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleHeight) || (sPropertyName == PrintSchemaTags.Keywords.PageScalingKeys.CustomSquareScale) ) { // custom or custom square scaling properties try { string paramRefName = reader.GetCurrentPropertyParamRefNameWithException(); if ( (sPropertyName == PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleWidth) && (paramRefName == PrintSchemaTags.Keywords.ParameterDefs.PageScalingScaleWidth) ) { option._scaleWIndex = (int)PrintSchemaLocalParameterDefs.PageScalingScaleWidth; // Mark the local parameter-def as required option.OwnerFeature.OwnerPrintCap.SetLocalParameterDefAsRequired(option._scaleWIndex, true); } else if ( (sPropertyName == PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleHeight) && (paramRefName == PrintSchemaTags.Keywords.ParameterDefs.PageScalingScaleHeight) ) { option._scaleHIndex = (int)PrintSchemaLocalParameterDefs.PageScalingScaleHeight; // Mark the local parameter-def as required option.OwnerFeature.OwnerPrintCap.SetLocalParameterDefAsRequired(option._scaleHIndex, true); } else if ( (sPropertyName == PrintSchemaTags.Keywords.PageScalingKeys.CustomSquareScale) && (paramRefName == PrintSchemaTags.Keywords.ParameterDefs.PageSquareScalingScale) ) { option._squareScaleIndex = (int)PrintSchemaLocalParameterDefs.PageSquareScalingScale; // Mark the local parameter-def as required option.OwnerFeature.OwnerPrintCap.SetLocalParameterDefAsRequired(option._squareScaleIndex, true); } else { #if _DEBUG Trace.WriteLine("-Warning- skip unknown ParameterRef " + paramRefName + "' at line number " + reader._xmlReader.LineNumber + ", line position " + reader._xmlReader.LinePosition); #endif } } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else { handled = false; #if _DEBUG Trace.WriteLine("-Warning- skip unknown ScoredProperty '" + reader.CurrentElementNameAttrValue + "' at line " + reader._xmlReader.LineNumber + ", position " + reader._xmlReader.LinePosition); #endif } } return handled; } #endregion Internal Methods #region Internal Properties internal override sealed bool IsValid { get { return (this.ScalingOptions.Count > 0); } } internal override sealed string FeatureName { get { return PrintSchemaTags.Keywords.PageScalingKeys.Self; } } internal override sealed bool HasSubFeature { get { return false; } } #endregion Internal Properties #region Internal Fields internal Collection_scalingOptions; #endregion Internal Fields } /// /// Represents page scaling setting. /// internal class PageScalingSetting : PrintTicketFeature { #region Constructors ////// Constructs a new page scaling setting object. /// internal PageScalingSetting(InternalPrintTicket ownerPrintTicket) : base(ownerPrintTicket) { this._featureName = PrintSchemaTags.Keywords.PageScalingKeys.Self; this._propertyMaps = new PTPropertyMapEntry[] { new PTPropertyMapEntry(this, PrintSchemaTags.Framework.OptionNameProperty, PTPropValueTypes.EnumStringValue, PrintSchemaTags.Keywords.PageScalingKeys.ScalingNames, PrintSchemaTags.Keywords.PageScalingKeys.ScalingEnums), new PTPropertyMapEntry(this, PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleWidth, PTPropValueTypes.IntParamRefValue, PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleWidth, PrintSchemaTags.Keywords.ParameterDefs.PageScalingScaleWidth), new PTPropertyMapEntry(this, PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleHeight, PTPropValueTypes.IntParamRefValue, PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleHeight, PrintSchemaTags.Keywords.ParameterDefs.PageScalingScaleHeight), new PTPropertyMapEntry(this, PrintSchemaTags.Keywords.PageScalingKeys.CustomSquareScale, PTPropValueTypes.IntParamRefValue, PrintSchemaTags.Keywords.PageScalingKeys.CustomSquareScale, PrintSchemaTags.Keywords.ParameterDefs.PageSquareScalingScale), }; } #endregion Constructors #region Public Properties ////// Gets the value of the page scaling setting. /// ////// If the setting is not specified yet, the return value will be /// public PageScaling Value { get { return (PageScaling)this[PrintSchemaTags.Framework.OptionNameProperty]; } } ///. /// /// Gets the custom page scaling setting's scaling percentage in the width direction. /// ////// If current page scaling setting is not public int CustomScaleWidth { get { if (this.Value != PageScaling.Custom) return PrintSchema.UnspecifiedIntValue; return this[PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleWidth]; } } ///, /// the return value will be . /// /// Gets the custom page scaling setting's scaling percentage in the height direction. /// ////// If current page scaling setting is not public int CustomScaleHeight { get { if (this.Value != PageScaling.Custom) return PrintSchema.UnspecifiedIntValue; return this[PrintSchemaTags.Keywords.PageScalingKeys.CustomScaleHeight]; } } ///, /// the return value will be . /// /// Gets the custom square page scaling setting's scaling percentage. /// ////// If current page scaling setting is not public int CustomSquareScale { get { if (this.Value != PageScaling.CustomSquare) return PrintSchema.UnspecifiedIntValue; return this[PrintSchemaTags.Keywords.PageScalingKeys.CustomSquareScale]; } } #endregion Public Properties #region Public Methods ///, /// the return value will be . /// /// Sets page scaling setting to custom square scaling. /// /// custom square scaling percentage ////// "squareScale" is not non-negative value. /// public void SetCustomSquareScaling(int squareScale) { // Scaling percentage value must be non-negative. We do allow negative scaling offset values. if (squareScale < 0) { throw new ArgumentOutOfRangeException("squareScale", PTUtility.GetTextFromResource("ArgumentException.NonNegativeValue")); } // Remove all XML content related to PageScaling feature // ClearSetting(); this[PrintSchemaTags.Framework.OptionNameProperty] = (int)PageScaling.CustomSquare; this[PrintSchemaTags.Keywords.PageScalingKeys.CustomSquareScale] = squareScale; } ////// Converts the page scaling setting to human-readable string. /// ///A string that represents this page scaling setting. public override string ToString() { if ((Value != PageScaling.Custom) || (Value != PageScaling.CustomSquare)) { return Value.ToString(); } else { return Value.ToString() + ", ScaleWidth=" + CustomScaleWidth.ToString(CultureInfo.CurrentCulture) + ", ScaleHeight=" + CustomScaleHeight.ToString(CultureInfo.CurrentCulture) + ", SquareScale=" + CustomSquareScale.ToString(CultureInfo.CurrentCulture); } } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
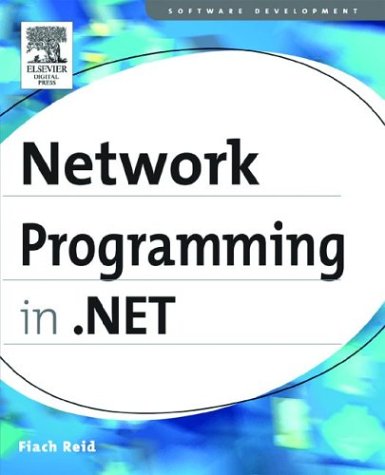
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Label.cs
- ListViewInsertEventArgs.cs
- UIElementAutomationPeer.cs
- SqlConnectionFactory.cs
- WebSysDisplayNameAttribute.cs
- GlobalizationAssembly.cs
- ReadOnlyMetadataCollection.cs
- AppSettingsSection.cs
- NominalTypeEliminator.cs
- LogicalExpr.cs
- ResourceDictionaryCollection.cs
- DesignerActionListCollection.cs
- RoutingTable.cs
- TypeForwardedToAttribute.cs
- EncoderParameter.cs
- TcpTransportManager.cs
- KeyboardEventArgs.cs
- CodeAccessSecurityEngine.cs
- GridSplitter.cs
- VisualBrush.cs
- KeyPressEvent.cs
- DateTimeOffset.cs
- AmbientLight.cs
- ThreadStartException.cs
- ErrorTableItemStyle.cs
- XPathScanner.cs
- _StreamFramer.cs
- SHA384Managed.cs
- WindowsToolbarAsMenu.cs
- ToolStripSeparatorRenderEventArgs.cs
- WebEncodingValidatorAttribute.cs
- StandardToolWindows.cs
- Globals.cs
- XmlWrappingWriter.cs
- ObjectStorage.cs
- Timer.cs
- grammarelement.cs
- InkCanvasAutomationPeer.cs
- WindowsIdentity.cs
- TypeDescriptor.cs
- ReferenceSchema.cs
- SqlDataReaderSmi.cs
- ContentFileHelper.cs
- ThreadAttributes.cs
- XmlHelper.cs
- PathFigure.cs
- TdsParserStateObject.cs
- ButtonColumn.cs
- DictionaryManager.cs
- DriveInfo.cs
- TextureBrush.cs
- MaskInputRejectedEventArgs.cs
- DbFunctionCommandTree.cs
- MenuEventArgs.cs
- ErrorProvider.cs
- MarkupObject.cs
- DesignColumnCollection.cs
- AggregateNode.cs
- StringFunctions.cs
- ClientScriptManagerWrapper.cs
- IteratorDescriptor.cs
- SHA1Managed.cs
- TerminatorSinks.cs
- MSHTMLHost.cs
- XmlTypeAttribute.cs
- HWStack.cs
- ScrollViewerAutomationPeer.cs
- EventLogPermission.cs
- OperandQuery.cs
- ResourcePermissionBase.cs
- TaiwanLunisolarCalendar.cs
- RequiredFieldValidator.cs
- IntSecurity.cs
- ProcessHostConfigUtils.cs
- WsdlInspector.cs
- Int32Converter.cs
- Shape.cs
- JpegBitmapEncoder.cs
- ColumnWidthChangedEvent.cs
- NaturalLanguageHyphenator.cs
- EntityContainerEntitySetDefiningQuery.cs
- TrailingSpaceComparer.cs
- SerialErrors.cs
- DataKeyArray.cs
- PolyBezierSegment.cs
- EntityContainer.cs
- HandlerFactoryCache.cs
- ArithmeticLiteral.cs
- UriWriter.cs
- DetailsViewInsertEventArgs.cs
- WarningException.cs
- DocumentAutomationPeer.cs
- EntityConnectionStringBuilderItem.cs
- FixedFindEngine.cs
- VarRefManager.cs
- MarkupCompilePass2.cs
- Int64Converter.cs
- IUnknownConstantAttribute.cs
- MenuItemStyleCollection.cs
- WindowsPrincipal.cs