Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Packaging / XpsResource.cs / 1 / XpsResource.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation All rights reserved. Module Name: XpsResource.cs Abstract: This file contains the definition and implementation for the XpsResource class. This class acts as the base class for all resources that can be added to a Xps package. Author: [....] ([....]) 1-November-2004 Revision History: Brian Adleberg ([....] ) 12-July-2005 Reach -> Xps --*/ using System; using System.Collections.Generic; using System.IO; using System.IO.Packaging; namespace System.Windows.Xps.Packaging { ////// Base class for all Xps Resources /// ///part is null. public class XpsResource : XpsPartBase, INode, IDisposable { #region Constructors internal XpsResource( XpsManager xpsManager, INode parent, PackagePart part ) : base(xpsManager) { if (null == part) { throw new ArgumentNullException("part"); } this.Uri = part.Uri; _parentNode = parent; _metroPart = part; _partEditor = new PartEditor(_metroPart); } #endregion Constructors #region Public methods ////// This method retrieves the relative Uri for this resource /// based on a supplied absolute resource. /// /// /// Absolute Uri used for conversion. /// ////// A Uri to this resource relative to the supplied resource. /// public Uri RelativeUri( Uri inUri ) { if( inUri == null ) { throw new ArgumentNullException("inUri"); } return new Uri(XpsManager.MakeRelativePath(this.Uri, inUri), UriKind.Relative); } ////// This method retrieves a reference to the Stream that can /// be used to read and/or write data to/from this resource /// within the Metro package. /// ////// A reference to a writable/readable stream. /// public virtual Stream GetStream( ) { return _partEditor.DataStream; } ////// This method commits all changes for this resource /// public void Commit( ) { CommitInternal(); } ////// This method closes this resource part and frees all /// associated memory. /// internal override void CommitInternal() { if (_partEditor != null) { _partEditor.Close(); _partEditor = null; _metroPart = null; _parentNode = null; } } #endregion Public methods #region Private data private INode _parentNode; private PackagePart _metroPart; private PartEditor _partEditor; #endregion Private data #region INode implementation void INode.Flush( ) { if( _partEditor != null ) { // // Flush the part editor // _partEditor.Flush(); } } void INode.CommitInternal() { CommitInternal(); } PackagePart INode.GetPart( ) { return _metroPart; } #endregion INode implementation #region IDisposable implementation void IDisposable.Dispose() { if (_partEditor != null) { _partEditor.Close(); } } #endregion IDisposable implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
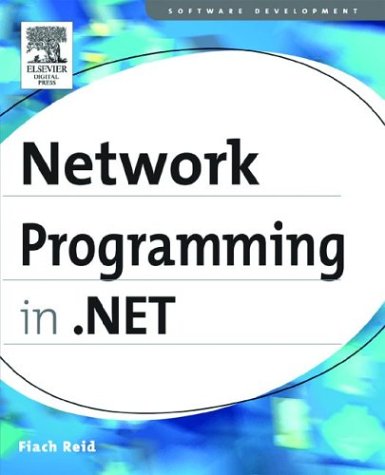
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIPropertyMetadata.cs
- AdapterUtil.cs
- DispatcherHooks.cs
- ChtmlImageAdapter.cs
- CodeMemberProperty.cs
- WmlObjectListAdapter.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- GridViewColumn.cs
- LostFocusEventManager.cs
- KeyboardNavigation.cs
- BindingOperations.cs
- NodeFunctions.cs
- DefaultHttpHandler.cs
- PrefixQName.cs
- QuaternionAnimation.cs
- ValueQuery.cs
- NativeCompoundFileAPIs.cs
- HMACSHA512.cs
- AuthenticationSection.cs
- ScriptingRoleServiceSection.cs
- MultipartIdentifier.cs
- X509Chain.cs
- DataGridViewImageCell.cs
- Oid.cs
- SystemIPAddressInformation.cs
- AnnotationAuthorChangedEventArgs.cs
- ContentElement.cs
- PathSegment.cs
- ConfigurationLockCollection.cs
- UIPermission.cs
- ParsedAttributeCollection.cs
- ShutDownListener.cs
- SystemIcmpV4Statistics.cs
- BadImageFormatException.cs
- BufferModesCollection.cs
- ProfilePropertyMetadata.cs
- ScrollContentPresenter.cs
- ResXDataNode.cs
- NamespaceEmitter.cs
- RequestContext.cs
- SecurityIdentifierConverter.cs
- ConnectionManagementElementCollection.cs
- ContentPlaceHolderDesigner.cs
- Color.cs
- SqlXmlStorage.cs
- ThemeableAttribute.cs
- InkCanvasInnerCanvas.cs
- XomlCompilerParameters.cs
- ToolStripButton.cs
- DelegatingStream.cs
- OracleTimeSpan.cs
- ArgumentOutOfRangeException.cs
- Pair.cs
- NetMsmqBindingCollectionElement.cs
- CallSiteBinder.cs
- DataGridHelper.cs
- XmlSignatureProperties.cs
- GestureRecognitionResult.cs
- MulticastNotSupportedException.cs
- JsonFormatReaderGenerator.cs
- AssociatedControlConverter.cs
- UTF7Encoding.cs
- WebCategoryAttribute.cs
- SaveFileDialogDesigner.cs
- ParentUndoUnit.cs
- DataGridColumnCollection.cs
- ObjectAnimationUsingKeyFrames.cs
- XmlSerializerVersionAttribute.cs
- IRCollection.cs
- TransformProviderWrapper.cs
- FloaterParagraph.cs
- RoutedEventConverter.cs
- MetricEntry.cs
- SystemIPAddressInformation.cs
- Base64Decoder.cs
- BoundColumn.cs
- MetadataCache.cs
- SwitchElementsCollection.cs
- XhtmlTextWriter.cs
- ObjectDisposedException.cs
- StylusCaptureWithinProperty.cs
- MemoryStream.cs
- ParamArrayAttribute.cs
- StreamingContext.cs
- StructuredCompositeActivityDesigner.cs
- OutputCacheModule.cs
- ByteStreamMessage.cs
- SoapConverter.cs
- XPathDocumentNavigator.cs
- NullableConverter.cs
- ExceptionRoutedEventArgs.cs
- WebPartDisplayMode.cs
- SettingsBindableAttribute.cs
- FilteredSchemaElementLookUpTable.cs
- PropertyEmitterBase.cs
- Config.cs
- TitleStyle.cs
- ParseElementCollection.cs
- DataReceivedEventArgs.cs
- TextTabProperties.cs