Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / BamlVersionHeader.cs / 1 / BamlVersionHeader.cs
/****************************************************************************\ * * File: BamlVersionHeader.cs * * Copyright (C) 2005 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.IO; using System.Globalization; using System.Diagnostics; using MS.Internal.IO.Packaging.CompoundFile; #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { internal class BamlVersionHeader { // The current BAML record version. This is incremented whenever // the BAML format changes // Baml Format Breaking Changes should change this. internal static readonly VersionPair BamlWriterVersion; static BamlVersionHeader() { // Initialize the Version number this way so that it can be // seen in the Lutz Reflector. BamlWriterVersion = new VersionPair(0, 96); } public BamlVersionHeader() { _bamlVersion = new FormatVersion("MSBAML", BamlWriterVersion); } public FormatVersion BamlVersion { get { return _bamlVersion; } #if !PBTCOMPILER set { _bamlVersion = value; } #endif } // This is used by Async loading to measure if the whole record is present static public int BinarySerializationSize { get { // Unicode "MSBAML" = 12 // + 4 bytes length header = 12 + 4 = 16 // + 3*(16bit MinorVer + 16bit MajorVer) = 16+(3*(2+2))= 28 // For product stability the size of this data structure // shouldn't change anyway. return 28; } } #if !PBTCOMPILER internal void LoadVersion(BinaryReader bamlBinaryReader) { #if DEBUG long posStart = bamlBinaryReader.BaseStream.Position; #endif BamlVersion = FormatVersion.LoadFromStream(bamlBinaryReader.BaseStream); #if DEBUG long posEnd = bamlBinaryReader.BaseStream.Position; Debug.Assert((posEnd-posStart) == BamlVersionHeader.BinarySerializationSize, "Incorrect Baml Version Header Size"); #endif // We're assuming that only major versions are significant for compatibility, // so if we have a major version in the file that is higher than that in // the code, we can't read it. if (BamlVersion.ReaderVersion != BamlWriterVersion) { throw new InvalidOperationException(SR.Get(SRID.ParserBamlVersion, (BamlVersion.ReaderVersion.Major.ToString(CultureInfo.CurrentCulture) + "." + BamlVersion.ReaderVersion.Minor.ToString(CultureInfo.CurrentCulture)), (BamlWriterVersion.Major.ToString(CultureInfo.CurrentCulture) + "." + BamlWriterVersion.Minor.ToString(CultureInfo.CurrentCulture)))); } } #endif internal void WriteVersion(BinaryWriter bamlBinaryWriter) { #if DEBUG long posStart = bamlBinaryWriter.BaseStream.Position; #endif BamlVersion.SaveToStream(bamlBinaryWriter.BaseStream); #if DEBUG long posEnd = bamlBinaryWriter.BaseStream.Position; if(-1 == posStart) { long length = bamlBinaryWriter.BaseStream.Length; Debug.Assert(length == BamlVersionHeader.BinarySerializationSize, "Incorrect Baml Version Header Size"); } else { Debug.Assert((posEnd-posStart) == BamlVersionHeader.BinarySerializationSize, "Incorrect Baml Version Header Size"); } #endif } FormatVersion _bamlVersion; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
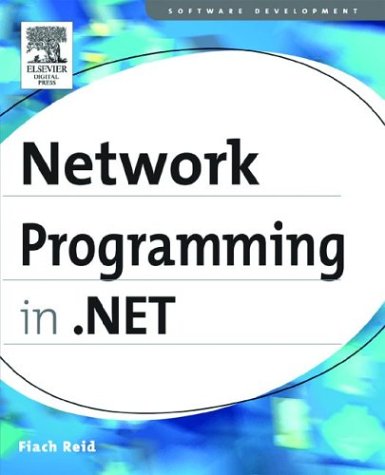
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuilderInfo.cs
- SelectionUIHandler.cs
- EncryptedKey.cs
- TypeDefinition.cs
- SkipQueryOptionExpression.cs
- SafeTokenHandle.cs
- XamlPointCollectionSerializer.cs
- BufferedGraphics.cs
- BaseValidatorDesigner.cs
- BindToObject.cs
- XmlTextEncoder.cs
- MessageFault.cs
- TableItemStyle.cs
- _TransmitFileOverlappedAsyncResult.cs
- PipeStream.cs
- TrackingServices.cs
- TextEmbeddedObject.cs
- BindingNavigator.cs
- cookiecontainer.cs
- WebAdminConfigurationHelper.cs
- MD5Cng.cs
- PasswordRecovery.cs
- EncryptedHeader.cs
- Attributes.cs
- XmlQualifiedNameTest.cs
- RunInstallerAttribute.cs
- OleDbConnection.cs
- AlignmentXValidation.cs
- XmlBoundElement.cs
- HtmlTernaryTree.cs
- IdentifierService.cs
- SemanticResultKey.cs
- CompilerErrorCollection.cs
- ControllableStoryboardAction.cs
- InstanceLockTracking.cs
- MSHTMLHostUtil.cs
- QilIterator.cs
- SmiSettersStream.cs
- NamedObject.cs
- ConstraintStruct.cs
- SqlServer2KCompatibilityCheck.cs
- StringUtil.cs
- HScrollProperties.cs
- ChildTable.cs
- SemanticTag.cs
- FieldNameLookup.cs
- FontSource.cs
- ClaimTypeElementCollection.cs
- FieldAccessException.cs
- BuildResult.cs
- EventItfInfo.cs
- SqlUnionizer.cs
- MessageBox.cs
- MimeParameterWriter.cs
- ReceiveContent.cs
- DataBindingCollection.cs
- AsymmetricSignatureFormatter.cs
- TypedTableBaseExtensions.cs
- TransformedBitmap.cs
- TrackingWorkflowEventArgs.cs
- Listen.cs
- HttpPostServerProtocol.cs
- ValidationHelpers.cs
- PassportAuthentication.cs
- EntityCommand.cs
- StreamGeometry.cs
- TaskFactory.cs
- TextBox.cs
- SchemaContext.cs
- MonthCalendar.cs
- PageStatePersister.cs
- XmlSchemaInferenceException.cs
- XAMLParseException.cs
- CompilerInfo.cs
- SafeRightsManagementEnvironmentHandle.cs
- ConfigurationElement.cs
- SessionStateSection.cs
- PriorityBinding.cs
- PropertyTab.cs
- ListViewInsertedEventArgs.cs
- MemberMaps.cs
- FieldBuilder.cs
- BlobPersonalizationState.cs
- ExpandSegmentCollection.cs
- WorkflowDataContext.cs
- HttpStreamMessage.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- ComponentDispatcher.cs
- EpmCustomContentSerializer.cs
- ComponentDispatcherThread.cs
- DebugHandleTracker.cs
- MouseButton.cs
- FormViewDeleteEventArgs.cs
- MobileControlsSectionHelper.cs
- DataViewSettingCollection.cs
- AssemblyInfo.cs
- ProcessManager.cs
- KnownTypes.cs
- FindSimilarActivitiesVerb.cs
- EntityFunctions.cs