Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / CharacterHit.cs / 1 / CharacterHit.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: The CharacterHit structure represents information about a character hit // within a glyph run - the index of the first character that got hit and the information // about leading or trailing edge. // // See spec at [....]/sites/Avalon/Specs/Glyph%20Run%20hit%20testing%20and%20caret%20placement%20API.htm#CharacterHit // // // History: // 11/30/2004 : [....] - Created // //--------------------------------------------------------------------------- #region Using directives using System; #endregion namespace System.Windows.Media.TextFormatting { ////// The CharacterHit structure represents information about a character hit within a glyph run /// - the index of the first character that got hit and the information about leading or trailing edge. /// public struct CharacterHit : IEquatable{ /// /// Constructs a new CharacterHit structure. /// /// Index of the first character that got hit. /// In case of leading edge this value is 0. /// In case of trailing edge this value is the number of codepoints until the next valid caret position. public CharacterHit(int firstCharacterIndex, int trailingLength) { _firstCharacterIndex = firstCharacterIndex; _trailingLength = trailingLength; } ////// Index of the first character that got hit. /// public int FirstCharacterIndex { get { return _firstCharacterIndex; } } ////// In case of leading edge this value is 0. /// In case of trailing edge this value is the number of codepoints until the next valid caret position. /// public int TrailingLength { get { return _trailingLength; } } ////// Checks whether two character hit objects are equal. /// /// First object to compare. /// Second object to compare. ///Returns true when the values of FirstCharacterIndex and TrailingLength are equal for both objects, /// and false otherwise. public static bool operator==(CharacterHit left, CharacterHit right) { return left._firstCharacterIndex == right._firstCharacterIndex && left._trailingLength == right._trailingLength; } ////// Checks whether two character hit objects are not equal. /// /// First object to compare. /// Second object to compare. ///Returns false when the values of FirstCharacterIndex and TrailingLength are equal for both objects, /// and true otherwise. public static bool operator!=(CharacterHit left, CharacterHit right) { return !(left == right); } ////// Checks whether an object is equal to another character hit object. /// /// CharacterHit object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public bool Equals(CharacterHit obj) { return this == obj; } ////// Checks whether an object is equal to another character hit object. /// /// CharacterHit object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public override bool Equals(object obj) { if (!(obj is CharacterHit)) return false; return this == (CharacterHit)obj; } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { return _firstCharacterIndex.GetHashCode() ^ _trailingLength.GetHashCode(); } private int _firstCharacterIndex; private int _trailingLength; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
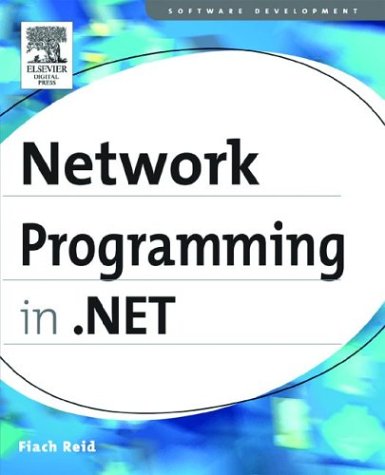
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WmlPageAdapter.cs
- UnsafeMethods.cs
- FormViewDeletedEventArgs.cs
- ArrangedElementCollection.cs
- ConnectionPoolManager.cs
- ChildChangedEventArgs.cs
- AllMembershipCondition.cs
- DataIdProcessor.cs
- ObjectStateFormatter.cs
- HandledMouseEvent.cs
- PeerNameRecordCollection.cs
- TextPointerBase.cs
- CookieParameter.cs
- Int64KeyFrameCollection.cs
- ControlAdapter.cs
- JsonFormatGeneratorStatics.cs
- OdbcHandle.cs
- ServiceObjectContainer.cs
- TrustManager.cs
- TextElement.cs
- SpellerInterop.cs
- SetIterators.cs
- ListQueryResults.cs
- ErrorsHelper.cs
- RewritingValidator.cs
- BypassElement.cs
- DesignerToolboxInfo.cs
- ApplicationGesture.cs
- ShellProvider.cs
- TextRangeSerialization.cs
- Int64Animation.cs
- HtmlTable.cs
- XmlSchemaComplexType.cs
- FigureHelper.cs
- XmlILCommand.cs
- AtlasWeb.Designer.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- RestHandlerFactory.cs
- UnitySerializationHolder.cs
- AddInEnvironment.cs
- MergeFilterQuery.cs
- FileDataSource.cs
- Line.cs
- TemplateControlCodeDomTreeGenerator.cs
- SimpleWebHandlerParser.cs
- CodeBlockBuilder.cs
- PropertyRecord.cs
- TypeHelpers.cs
- SafeHandle.cs
- ImageListImage.cs
- MediaElementAutomationPeer.cs
- DoWorkEventArgs.cs
- RepeatBehaviorConverter.cs
- BaseAsyncResult.cs
- SqlDataSourceQueryConverter.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- DesignerTransactionCloseEvent.cs
- PropertyEntry.cs
- HtmlWindow.cs
- TrackingMemoryStream.cs
- PointCollectionValueSerializer.cs
- DiagnosticTraceSource.cs
- DiscoveryDocumentSearchPattern.cs
- SourceFileBuildProvider.cs
- PipelineModuleStepContainer.cs
- COM2ICategorizePropertiesHandler.cs
- SymmetricAlgorithm.cs
- Pair.cs
- SubMenuStyle.cs
- HtmlUtf8RawTextWriter.cs
- SoapSchemaExporter.cs
- GenericIdentity.cs
- EncryptionUtility.cs
- CalendarDateRangeChangingEventArgs.cs
- TypefaceMap.cs
- ItemsControlAutomationPeer.cs
- MulticastNotSupportedException.cs
- AutoGeneratedField.cs
- VirtualPath.cs
- SqlExpressionNullability.cs
- CodeArrayIndexerExpression.cs
- CapabilitiesPattern.cs
- SqlNotificationRequest.cs
- Win32.cs
- CodeConstructor.cs
- OleDbError.cs
- FixedSOMTableCell.cs
- KeyboardEventArgs.cs
- codemethodreferenceexpression.cs
- ContentElement.cs
- EntityDataSourceDesigner.cs
- XPathAncestorIterator.cs
- ReadOnlyCollection.cs
- DataGridViewCellCancelEventArgs.cs
- SafeRightsManagementPubHandle.cs
- UnsafeNativeMethodsCLR.cs
- Update.cs
- TrackingCondition.cs
- XmlDataCollection.cs
- ElementProxy.cs