Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / EventManager.cs / 2 / EventManager.cs
using System; using System.Diagnostics; using MS.Internal.PresentationCore; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Allows management of RoutedEvents /// ///public static class EventManager { #region External API /// /// Registers a ////// with the given parameters /// /// /// ///must be /// unique within the /// (super class types not considered when talking about /// uniqueness) and cannot be null /// must be a /// type of delegate and cannot be null /// must be any /// object type and cannot be null /// /// /// NOTE: Caller must be the static constructor of the /// - /// enforced by stack walk /// /// /// /// /// /// /// /// /// /// /// /// /// The new registered ////// public static RoutedEvent RegisterRoutedEvent( string name, RoutingStrategy routingStrategy, Type handlerType, Type ownerType) { if (name == null) { throw new ArgumentNullException("name"); } if (routingStrategy != RoutingStrategy.Tunnel && routingStrategy != RoutingStrategy.Bubble && routingStrategy != RoutingStrategy.Direct) { throw new System.ComponentModel.InvalidEnumArgumentException("routingStrategy", (int)routingStrategy, typeof(RoutingStrategy)); } if (handlerType == null) { throw new ArgumentNullException("handlerType"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } if (GlobalEventManager.GetRoutedEventFromName(name, ownerType, false) != null) { throw new ArgumentException(SR.Get(SRID.DuplicateEventName, name, ownerType)); } return GlobalEventManager.RegisterRoutedEvent(name, routingStrategy, handlerType, ownerType); } /// /// See overloaded method for details /// ////// handledEventsToo defaults to false /// See overloaded method for details /// /// /// /// ///public static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler) { // HandledEventToo defaults to false // Call forwarded RegisterClassHandler(classType, routedEvent, handler, false); } /// /// Add a routed event handler to all instances of a /// particular type inclusive of its sub-class types /// ////// The handlers added thus are also known as /// an class handlers /// /// Target object type on which the handler will be invoked /// when the RoutedEvent is raised /// /// ////// /// /// Class handlers are invoked before the /// instance handlers. Also see /// /// Sub-class class handlers are invoked before /// the super-class class handlers /// /// /// Input parameters classType, /// and handler cannot be null /// handledEventsToo input parameter when false means /// that listener does not care about already handled events. /// Hence the handler will not be invoked on the target if /// the RoutedEvent has already been /// /// handledEventsToo input parameter when true means /// that the listener wants to hear about all events even if /// they have already been handled. Hence the handler will /// be invoked irrespective of the event being /// /// for which the handler /// is attached /// /// /// The handler that will be invoked on the target object /// when the RoutedEvent is raised /// /// /// Flag indicating whether or not the listener wants to /// hear about events that have already been handled /// /// public static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { if (classType == null) { throw new ArgumentNullException("classType"); } if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!typeof(UIElement).IsAssignableFrom(classType) && !typeof(ContentElement).IsAssignableFrom(classType) && !typeof(UIElement3D).IsAssignableFrom(classType)) { throw new ArgumentException(SR.Get(SRID.ClassTypeIllegal)); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } GlobalEventManager.RegisterClassHandler(classType, routedEvent, handler, handledEventsToo); } /// /// Returns ///s /// that have been registered so far /// /// Also see /// ////// /// /// /// NOTE: There may be more /// s registered later /// /// The ///s /// that have been registered so far /// public static RoutedEvent[] GetRoutedEvents() { return GlobalEventManager.GetRoutedEvents(); } /// /// Finds ///s for the /// given /// /// More specifically finds /// /// ///s starting /// on the /// and looking at its super class types /// /// /// If no matches are found, this method returns null /// to start /// search with and follow through to super class types /// /// /// Matching ///s /// public static RoutedEvent[] GetRoutedEventsForOwner(Type ownerType) { if (ownerType == null) { throw new ArgumentNullException("ownerType"); } return GlobalEventManager.GetRoutedEventsForOwner(ownerType); } /// /// Finds a ///with a /// matching /// and /// /// More specifically finds a /// /// ///with a matching /// starting /// on the /// and looking at its super class types /// /// /// If no matches are found, this method returns null /// to be matched /// /// /// to start /// search with and follow through to super class types /// /// /// Matching [FriendAccessAllowed] internal static RoutedEvent GetRoutedEventFromName(string name, Type ownerType) { if (name == null) { throw new ArgumentNullException("name"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } return GlobalEventManager.GetRoutedEventFromName(name, ownerType, true); } #endregion ExternalAPI } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
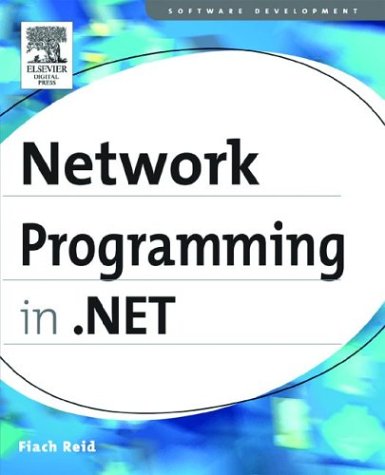
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IncrementalCompileAnalyzer.cs
- ListItemParagraph.cs
- TogglePatternIdentifiers.cs
- DesigntimeLicenseContext.cs
- HashStream.cs
- TextSelection.cs
- ResourceCategoryAttribute.cs
- DefaultTextStore.cs
- CommandDevice.cs
- TextTreeRootNode.cs
- RequestCacheManager.cs
- Table.cs
- XPathNodeInfoAtom.cs
- SqlStream.cs
- externdll.cs
- RangeValidator.cs
- XPathParser.cs
- XmlTextAttribute.cs
- XmlSchemaSimpleTypeUnion.cs
- ProcessHostServerConfig.cs
- storepermission.cs
- NonVisualControlAttribute.cs
- NestPullup.cs
- OletxTransactionManager.cs
- GotoExpression.cs
- FloatSumAggregationOperator.cs
- OrthographicCamera.cs
- securitymgrsite.cs
- ConfigXmlElement.cs
- XmlCompatibilityReader.cs
- DrawingBrush.cs
- SafeProcessHandle.cs
- FilteredSchemaElementLookUpTable.cs
- PropertyGeneratedEventArgs.cs
- FixedTextPointer.cs
- securitycriticaldata.cs
- Predicate.cs
- RelationshipEnd.cs
- ManagementException.cs
- Tool.cs
- ProtocolsConfiguration.cs
- FormViewPageEventArgs.cs
- FlowThrottle.cs
- RepeaterCommandEventArgs.cs
- FormsAuthenticationUser.cs
- ZipFileInfoCollection.cs
- HebrewCalendar.cs
- RelatedImageListAttribute.cs
- xamlnodes.cs
- ProfileEventArgs.cs
- SingleObjectCollection.cs
- StrokeSerializer.cs
- ProviderSettingsCollection.cs
- MaskInputRejectedEventArgs.cs
- SmiGettersStream.cs
- CodeMethodMap.cs
- WinFormsSecurity.cs
- XmlCharCheckingWriter.cs
- GridViewPageEventArgs.cs
- AuthenticationService.cs
- SqlBulkCopyColumnMappingCollection.cs
- ToolStripOverflowButton.cs
- HebrewNumber.cs
- SafeTokenHandle.cs
- CssClassPropertyAttribute.cs
- XmlSchemaGroupRef.cs
- Point3DAnimationUsingKeyFrames.cs
- ValueConversionAttribute.cs
- WorkflowServiceHost.cs
- CacheSection.cs
- ByteStreamGeometryContext.cs
- DictionaryBase.cs
- InfoCardSymmetricAlgorithm.cs
- SqlParameter.cs
- CheckBoxRenderer.cs
- CompiledQueryCacheKey.cs
- COM2PropertyDescriptor.cs
- remotingproxy.cs
- TogglePattern.cs
- ExpressionBuilder.cs
- MsmqIntegrationChannelFactory.cs
- SuppressMergeCheckAttribute.cs
- EffectiveValueEntry.cs
- FunctionQuery.cs
- SecurityTokenValidationException.cs
- SByte.cs
- TripleDESCryptoServiceProvider.cs
- RegexBoyerMoore.cs
- ContentPlaceHolder.cs
- SerialPort.cs
- SmiSettersStream.cs
- securitycriticaldataformultiplegetandset.cs
- ColumnMap.cs
- SQLMembershipProvider.cs
- TemplateInstanceAttribute.cs
- TemplateLookupAction.cs
- DictionaryKeyPropertyAttribute.cs
- MexHttpsBindingElement.cs
- SqlProfileProvider.cs
- SocketAddress.cs