Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / Accessible.cs / 1305600 / Accessible.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Wraps some of IAccessible to support Focus and top-level window creates // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Automation; using System; using Accessibility; using System.Text; using System.Diagnostics; using MS.Win32; // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace MS.Internal.Automation { internal class Accessible { internal Accessible(IAccessible acc, object child) { Debug.Assert(acc != null, "null IAccessible"); _acc = acc; _child = child; } // Create an Accessible object; may return null internal static Accessible Create( IntPtr hwnd, int idObject, int idChild ) { IAccessible acc = null; object child = null; if( UnsafeNativeMethods.AccessibleObjectFromEvent( hwnd, idObject, idChild, ref acc, ref child ) != 0 /*S_OK*/ || acc == null ) return null; // Per SDK must use the ppacc and pvarChild from AccessibleObjectFromEvent // to access information about this UI element. return new Accessible( acc, child ); } internal int State { get { try { return (int)_acc.get_accState(_child); } catch (Exception e) { if (IsCriticalMSAAException(e)) // PRESHARP will flag this as a warning 56503/6503: Property get methods should not throw exceptions // Since this Property is internal, we CAN throw an exception #pragma warning suppress 6503 throw; return UnsafeNativeMethods.STATE_SYSTEM_UNAVAILABLE; } } } internal IntPtr Window { get { if (_hwnd == IntPtr.Zero) { try { if (UnsafeNativeMethods.WindowFromAccessibleObject(_acc, ref _hwnd) != 0/*S_OK*/) { _hwnd = IntPtr.Zero; } } catch( Exception e ) { if (IsCriticalMSAAException(e)) // PRESHARP will flag this as a warning 56503/6503: Property get methods should not throw exceptions // Since this Property is internal, we CAN throw an exception #pragma warning suppress 6503 throw; _hwnd = IntPtr.Zero; } } return _hwnd; } } // miscellaneous functions used with Accessible internal static bool CompareClass(IntPtr hwnd, string szClass) { return ProxyManager.GetClassName(NativeMethods.HWND.Cast(hwnd)) == szClass; } internal static bool IsComboDropdown(IntPtr hwnd) { return CompareClass(hwnd, "ComboLBox"); } internal static bool IsStatic(IntPtr hwnd) { return CompareClass(hwnd, "Static"); } private bool IsCriticalMSAAException(Exception e) { // Some OLEACC proxies produce out-of-memory for non-critical reasons: // notably, the treeview proxy will raise this if the target HWND no longer exists, // GetWindowThreadProcessID fails and it therefore won't be able to allocate shared // memory in the target process, so it incorrectly assumes OOM. // Some Native impls (media player) return E_POINTER, which COM Interop translates // into NullRefException; need to ignore those too. // should ignore those return !(e is OutOfMemoryException) && !(e is NullReferenceException) && Misc.IsCriticalException(e); } private IntPtr _hwnd; private IAccessible _acc; private Object _child; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
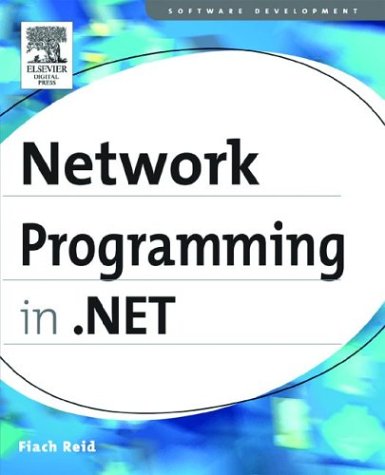
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapMetadataEnumerator.cs
- XmlSchemaSimpleType.cs
- securitymgrsite.cs
- TabRenderer.cs
- webclient.cs
- DateTimeParse.cs
- TransformerInfo.cs
- RuleAction.cs
- XPathNodePointer.cs
- ControlType.cs
- Activator.cs
- SQLConvert.cs
- TimeSpanValidatorAttribute.cs
- VectorAnimationBase.cs
- WCFBuildProvider.cs
- DbExpressionVisitor_TResultType.cs
- ListViewSortEventArgs.cs
- InvalidateEvent.cs
- SecurityTokenException.cs
- XmlLanguage.cs
- oledbmetadatacolumnnames.cs
- sqlpipe.cs
- TransformGroup.cs
- LocalizabilityAttribute.cs
- SizeChangedEventArgs.cs
- PlatformNotSupportedException.cs
- LockedAssemblyCache.cs
- RawAppCommandInputReport.cs
- NativeMethods.cs
- Label.cs
- ApplicationProxyInternal.cs
- DesignerActionMethodItem.cs
- GenericWebPart.cs
- MatrixTransform3D.cs
- SetterBase.cs
- HotSpot.cs
- ComponentResourceKey.cs
- ButtonAutomationPeer.cs
- ObjectDataSourceWizardForm.cs
- HtmlImage.cs
- DesignerPerfEventProvider.cs
- GeometryGroup.cs
- LocalizabilityAttribute.cs
- XmlNodeReader.cs
- SafeProcessHandle.cs
- Button.cs
- SHA512Cng.cs
- FontWeight.cs
- NonBatchDirectoryCompiler.cs
- WebPartEditorCancelVerb.cs
- OrderByExpression.cs
- HttpWebRequest.cs
- EdgeModeValidation.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- DatagridviewDisplayedBandsData.cs
- configsystem.cs
- EncryptedXml.cs
- HierarchicalDataSourceControl.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- ExpressionBindings.cs
- ResourceDescriptionAttribute.cs
- EntitySetBaseCollection.cs
- Fonts.cs
- Matrix.cs
- HtmlInputCheckBox.cs
- VisualStyleElement.cs
- XamlStyleSerializer.cs
- X509Chain.cs
- DynamicResourceExtension.cs
- SynchronousReceiveElement.cs
- FlowLayout.cs
- OdbcConnectionPoolProviderInfo.cs
- RepeatButtonAutomationPeer.cs
- ValidationErrorCollection.cs
- ResourceDictionary.cs
- TableLayoutSettings.cs
- ValidatedControlConverter.cs
- InputMethod.cs
- FieldToken.cs
- RuntimeConfig.cs
- MenuItemBinding.cs
- XmlAttributeOverrides.cs
- WindowsListViewItemCheckBox.cs
- FunctionQuery.cs
- StateDesignerConnector.cs
- Certificate.cs
- RoutingTable.cs
- CustomAttributeBuilder.cs
- MenuScrollingVisibilityConverter.cs
- ToolStripScrollButton.cs
- Rotation3DAnimationBase.cs
- OciEnlistContext.cs
- TripleDES.cs
- Transform.cs
- SmiRequestExecutor.cs
- MethodBody.cs
- HtmlElementCollection.cs
- XdrBuilder.cs
- HttpCacheVaryByContentEncodings.cs
- DataTablePropertyDescriptor.cs