Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Compilation / RouteValueExpressionBuilder.cs / 1305376 / RouteValueExpressionBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.CodeDom; using System.ComponentModel; using System.Configuration; using System.Diagnostics; using System.Web.UI; using System.Web.Routing; using System.Collections.Generic; [ExpressionPrefix("Routes")] [ExpressionEditor("System.Web.UI.Design.RouteValueExpressionEditor, " + AssemblyRef.SystemDesign)] public class RouteValueExpressionBuilder : ExpressionBuilder { public override bool SupportsEvaluate { get { return true; } } public override CodeExpression GetCodeExpression(BoundPropertyEntry entry, object parsedData, ExpressionBuilderContext context) { return new CodeMethodInvokeExpression( new CodeTypeReferenceExpression(this.GetType()), "GetRouteValue", new CodePropertyReferenceExpression(new CodeThisReferenceExpression(), "Page"), new CodePrimitiveExpression(entry.Expression.Trim()), new CodeTypeOfExpression(new CodeTypeReference(entry.ControlType)), new CodePrimitiveExpression(entry.Name) ); } public override object EvaluateExpression(object target, BoundPropertyEntry entry, object parsedData, ExpressionBuilderContext context) { // Target should always be a control Control control = target as Control; if (control == null) return null; return GetRouteValue(context.TemplateControl.Page, entry.Expression.Trim(), entry.ControlType, entry.Name); } internal static object ConvertRouteValue(object value, Type controlType, string propertyName) { // Try to do any type converting necessary for the property set if (controlType != null && !String.IsNullOrEmpty(propertyName)) { PropertyDescriptor propDesc = TypeDescriptor.GetProperties(controlType)[propertyName]; if (propDesc != null) { if (propDesc.PropertyType != typeof(string)) { TypeConverter converter = propDesc.Converter; if (converter.CanConvertFrom(typeof(string))) { return converter.ConvertFrom(value); } } } } return value; } // Format will be <%$ RouteValue: Key %>, controlType,propertyName are used to figure out what typeconverter to use public static object GetRouteValue(Page page, string key, Type controlType, string propertyName) { if (page == null || String.IsNullOrEmpty(key) || page.RouteData == null) { return null; } return ConvertRouteValue(page.RouteData.Values[key], controlType, propertyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
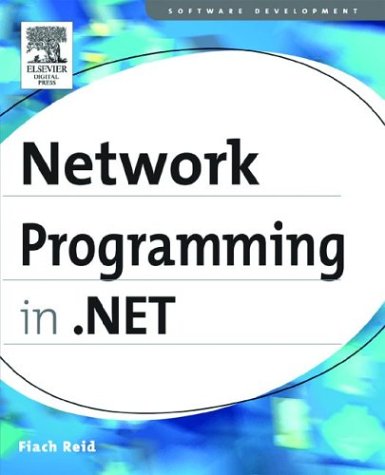
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateKeyConverter.cs
- ClientScriptManager.cs
- MemberRelationshipService.cs
- TextRangeBase.cs
- NetTcpSecurityElement.cs
- RuntimeEnvironment.cs
- LabelLiteral.cs
- TimeoutValidationAttribute.cs
- UInt16Converter.cs
- SubordinateTransaction.cs
- OdbcConnectionHandle.cs
- UrlMappingsModule.cs
- XmlCDATASection.cs
- RegionIterator.cs
- KeyValueSerializer.cs
- HtmlTableCell.cs
- RootBrowserWindowAutomationPeer.cs
- Rect3DConverter.cs
- PrintDialogException.cs
- dataprotectionpermission.cs
- OleDbInfoMessageEvent.cs
- ReadWriteObjectLock.cs
- InlineCollection.cs
- DelimitedListTraceListener.cs
- SpellerError.cs
- XPathAncestorQuery.cs
- WindowClosedEventArgs.cs
- DesignerTransactionCloseEvent.cs
- SystemDiagnosticsSection.cs
- WindowsComboBox.cs
- UInt16.cs
- DataGridViewTextBoxColumn.cs
- AuthenticationManager.cs
- DbModificationClause.cs
- CatalogZoneBase.cs
- ParentUndoUnit.cs
- TrustManager.cs
- NumberFormatter.cs
- SqlCacheDependencySection.cs
- CodeGroup.cs
- SettingsSection.cs
- DataServiceRequest.cs
- MenuItemCollection.cs
- HtmlEncodedRawTextWriter.cs
- PathNode.cs
- SafeSystemMetrics.cs
- PathTooLongException.cs
- ResourceReader.cs
- Normalization.cs
- SqlMethods.cs
- SmtpSection.cs
- HandlerBase.cs
- ProtectedProviderSettings.cs
- IpcClientManager.cs
- DatagridviewDisplayedBandsData.cs
- DrawingContextDrawingContextWalker.cs
- ConsoleTraceListener.cs
- EncoderReplacementFallback.cs
- MessageBox.cs
- DesignerVerb.cs
- NativeCompoundFileAPIs.cs
- DataExpression.cs
- FileUtil.cs
- FileChangeNotifier.cs
- SoapSchemaMember.cs
- NegotiateStream.cs
- ConnectionManagementElementCollection.cs
- HttpModuleActionCollection.cs
- QilDataSource.cs
- DescendantBaseQuery.cs
- DSACryptoServiceProvider.cs
- QueryHandler.cs
- AbstractSvcMapFileLoader.cs
- CustomPopupPlacement.cs
- Link.cs
- HtmlProps.cs
- ContentHostHelper.cs
- HexParser.cs
- EtwProvider.cs
- DataGridItem.cs
- SrgsSemanticInterpretationTag.cs
- WebPartAuthorizationEventArgs.cs
- ManagementEventArgs.cs
- DataGridCellEditEndingEventArgs.cs
- EntityTransaction.cs
- ListViewContainer.cs
- ExtenderControl.cs
- DbgUtil.cs
- RegexGroup.cs
- UpdateManifestForBrowserApplication.cs
- DesignerAdapterAttribute.cs
- WindowsScrollBar.cs
- ConfigurationStrings.cs
- RoleManagerSection.cs
- Viewport2DVisual3D.cs
- FailedToStartupUIException.cs
- KeyedCollection.cs
- PeerPresenceInfo.cs
- TreeNodeStyleCollection.cs
- XamlPathDataSerializer.cs