Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Shell / TaskbarItemInfo.cs / 1305600 / TaskbarItemInfo.cs
namespace System.Windows.Shell { using System; using System.Windows; using System.Windows.Media; using MS.Internal; ////// The values of TaskbarItemInfo's ProgressState dependency property. /// public enum TaskbarItemProgressState { None, Indeterminate, Normal, Error, Paused, } ////// This class manages Window's interaction with the Taskbar features added in Windows 7. /// public sealed class TaskbarItemInfo : Freezable { protected override Freezable CreateInstanceCore() { return new TaskbarItemInfo(); } #region Dependency Properties and support methods. ////// ProgressState Dependency Property /// public static readonly DependencyProperty ProgressStateProperty = DependencyProperty.Register( "ProgressState", typeof(TaskbarItemProgressState), typeof(TaskbarItemInfo), new PropertyMetadata( TaskbarItemProgressState.None, (d, e) => ((TaskbarItemInfo)d).NotifyDependencyPropertyChanged(e), (d, baseValue) => ((TaskbarItemInfo)d).CoerceProgressState((TaskbarItemProgressState)baseValue))); ////// Gets or sets the ProgressState property. This dependency property /// indicates the type of progress bar to display for the Window on the taskbar. /// public TaskbarItemProgressState ProgressState { get { return (TaskbarItemProgressState)GetValue(ProgressStateProperty); } set { SetValue(ProgressStateProperty, value); } } private TaskbarItemProgressState CoerceProgressState(TaskbarItemProgressState value) { switch (value) { case TaskbarItemProgressState.Error: case TaskbarItemProgressState.Indeterminate: case TaskbarItemProgressState.None: case TaskbarItemProgressState.Normal: case TaskbarItemProgressState.Paused: break; default: // Convert bad data into no-progress bar. value = TaskbarItemProgressState.None; break; } return value; } ////// ProgressValue Dependency Property /// public static readonly DependencyProperty ProgressValueProperty = DependencyProperty.Register( "ProgressValue", typeof(double), typeof(TaskbarItemInfo), new PropertyMetadata( 0d, (d, e) => ((TaskbarItemInfo)d).NotifyDependencyPropertyChanged(e), (d, baseValue) => CoerceProgressValue((double)baseValue))); ////// Gets or sets the ProgressValue property. This dependency property /// indicates the value of the progress bar for the Window in the taskbar. /// public double ProgressValue { get { return (double)GetValue(ProgressValueProperty); } set { SetValue(ProgressValueProperty, value); } } private static double CoerceProgressValue(double progressValue) { if (double.IsNaN(progressValue)) { progressValue = 0; } else { progressValue = Math.Max(progressValue, 0); progressValue = Math.Min(1, progressValue); } return progressValue; } ////// Overlay Dependency Property /// public static readonly DependencyProperty OverlayProperty = DependencyProperty.Register( "Overlay", typeof(ImageSource), typeof(TaskbarItemInfo), new PropertyMetadata( null, (d, e) => ((TaskbarItemInfo)d).NotifyDependencyPropertyChanged(e))); ////// Gets or sets the Overlay property. This dependency property /// indicates the overlay that is used to indicate status for the Window in the taskbar. /// public ImageSource Overlay { get { return (ImageSource)GetValue(OverlayProperty); } set { SetValue(OverlayProperty, value); } } ////// Description Dependency Property /// public static readonly DependencyProperty DescriptionProperty = DependencyProperty.Register( "Description", typeof(string), typeof(TaskbarItemInfo), new PropertyMetadata( string.Empty, (d, e) => ((TaskbarItemInfo)d).NotifyDependencyPropertyChanged(e))); ////// Gets or sets the Description property. This dependency property /// indicates the tooltip to display on the thumbnail for this window. /// public string Description { get { return (string)GetValue(DescriptionProperty); } set { SetValue(DescriptionProperty, value); } } ////// ThumbnailClipMargin Dependency Property /// public static readonly DependencyProperty ThumbnailClipMarginProperty = DependencyProperty.Register( "ThumbnailClipMargin", typeof(Thickness), typeof(TaskbarItemInfo), new PropertyMetadata( default(Thickness), (d, e) => ((TaskbarItemInfo)d).NotifyDependencyPropertyChanged(e), (d, baseValue) => ((TaskbarItemInfo)d).CoerceThumbnailClipMargin((Thickness)baseValue))); ////// Gets or sets the ThumbnailClipMargin property. This dependency property /// indicates the border of the Window to clip when displayed in the taskbar thumbnail preview. /// public Thickness ThumbnailClipMargin { get { return (Thickness)GetValue(ThumbnailClipMarginProperty); } set { SetValue(ThumbnailClipMarginProperty, value); } } private Thickness CoerceThumbnailClipMargin(Thickness margin) { // Any negative/NaN/Infinity margins we'll treat as nil. if (!margin.IsValid(false, false, false, false)) { return new Thickness(); } return margin; } ////// ThumbButtonInfos Dependency Property /// public static readonly DependencyProperty ThumbButtonInfosProperty = DependencyProperty.Register( "ThumbButtonInfos", typeof(ThumbButtonInfoCollection), typeof(TaskbarItemInfo), new PropertyMetadata( new FreezableDefaultValueFactory(ThumbButtonInfoCollection.Empty), (d, e) => ((TaskbarItemInfo)d).NotifyDependencyPropertyChanged(e))); ////// Gets the ThumbButtonInfos property. This dependency property /// indicates the collection of command buttons to be displayed in the taskbar item thumbnail for the window. /// public ThumbButtonInfoCollection ThumbButtonInfos { get { return (ThumbButtonInfoCollection)GetValue(ThumbButtonInfosProperty); } set { SetValue(ThumbButtonInfosProperty, value); } } #endregion private void NotifyDependencyPropertyChanged(DependencyPropertyChangedEventArgs e) { DependencyPropertyChangedEventHandler handler = PropertyChanged; if (handler != null) { handler(this, e); } } // Used by Window to receive notifications about sub-property changes. internal event DependencyPropertyChangedEventHandler PropertyChanged; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
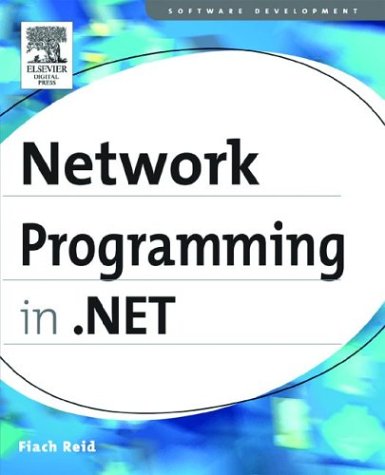
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnknownBitmapEncoder.cs
- XPathAncestorIterator.cs
- AppManager.cs
- RemotingAttributes.cs
- ImageBrush.cs
- FieldDescriptor.cs
- TextTrailingWordEllipsis.cs
- ObjectSecurity.cs
- TextEditorDragDrop.cs
- EpmCustomContentWriterNodeData.cs
- EUCJPEncoding.cs
- HandlerBase.cs
- SafeThemeHandle.cs
- ContactManager.cs
- Form.cs
- EventLogger.cs
- TextTreeDeleteContentUndoUnit.cs
- TableLayoutSettings.cs
- ServiceChannelProxy.cs
- WebBrowserSiteBase.cs
- GZipStream.cs
- ToolStripPanelSelectionGlyph.cs
- Emitter.cs
- XmlSchemaSequence.cs
- WebBrowsableAttribute.cs
- ListChunk.cs
- MediaEntryAttribute.cs
- PassportAuthentication.cs
- SqlDataSourceCommandEventArgs.cs
- PageFunction.cs
- WebPartDeleteVerb.cs
- ViewManager.cs
- Utilities.cs
- DateTimeOffsetStorage.cs
- BitFlagsGenerator.cs
- IxmlLineInfo.cs
- PolicyUtility.cs
- XamlGridLengthSerializer.cs
- RepeatBehavior.cs
- FileLogRecordStream.cs
- PersonalizableTypeEntry.cs
- StreamAsIStream.cs
- SecuritySessionFilter.cs
- StorageAssociationSetMapping.cs
- SQLMoneyStorage.cs
- XmlDocumentType.cs
- StringFunctions.cs
- ClientProxyGenerator.cs
- EventMappingSettingsCollection.cs
- ConnectionProviderAttribute.cs
- DataSourceView.cs
- AdornerHitTestResult.cs
- AsyncInvokeOperation.cs
- Configuration.cs
- DataGridViewButtonCell.cs
- CustomAttribute.cs
- URLAttribute.cs
- ByteRangeDownloader.cs
- IpcManager.cs
- MethodExpr.cs
- PointAnimation.cs
- WorkflowPrinting.cs
- DateTimePicker.cs
- ImpersonationContext.cs
- Pen.cs
- DerivedKeySecurityToken.cs
- MemberAccessException.cs
- XmlLinkedNode.cs
- Cloud.cs
- XmlDomTextWriter.cs
- PeerResolver.cs
- BinaryObjectWriter.cs
- GPRECT.cs
- PropertyGridView.cs
- XmlSchemaAttributeGroupRef.cs
- WebControl.cs
- Missing.cs
- NameSpaceEvent.cs
- TableLayoutPanel.cs
- HttpDebugHandler.cs
- SafeThreadHandle.cs
- Util.cs
- HtmlElement.cs
- EntityDataSourceSelectingEventArgs.cs
- SafeRegistryKey.cs
- LinkArea.cs
- ZipIOExtraField.cs
- Select.cs
- WmpBitmapDecoder.cs
- RemotingException.cs
- SourceFileInfo.cs
- ImageCreator.cs
- UserControlBuildProvider.cs
- EventRecordWrittenEventArgs.cs
- IsolatedStorageFileStream.cs
- CodeMemberField.cs
- VoiceChangeEventArgs.cs
- UdpSocket.cs
- Control.cs
- StopStoryboard.cs