Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / EventLogger.cs / 1 / EventLogger.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Install { using System; using System.Diagnostics; using System.Globalization; using System.ComponentModel; internal static class EventLogger { const int MaxEventLogLength = 32768; static MsiStyleLogWriter msiStyleLogWriter = null; static EventLogger() { if (!EventLog.SourceExists(ServiceModelInstallStrings.EventLogSource)) { EventLog.CreateEventSource(ServiceModelInstallStrings.EventLogSource, ServiceModelInstallStrings.EventLogName); } } internal static void InitializeMsiStyleLogging() { EventLogger.msiStyleLogWriter = MsiStyleLogWriter.CreateWriter(); } internal static void TerminateMsiStyleLogging() { if (EventLogger.msiStyleLogWriter != null) { DateTime now = DateTime.Now; EventLogger.msiStyleLogWriter.WriteRaw(SR.GetString(SR.MsiStyleLogTerminator, now.ToShortDateString(), now.ToString("HH:mm:ss", CultureInfo.CurrentCulture))); } EventLogger.msiStyleLogWriter = null; } internal static void LogException(Exception e) { EventLogger.LogToConsole(SR.GetString(SR.Error, e.Message)); EventLogger.WriteMsiStyleLogEntry(e.ToString()); EventLogger.WriteLogEntry(e.ToString(), EventLogEntryType.Error); } internal static void LogError(string message) { EventLogger.LogToConsole(message); EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.Error, message)); EventLogger.WriteLogEntry(message, EventLogEntryType.Error); } internal static void LogInformation(string message, bool displayToConsole) { if (displayToConsole) { EventLogger.LogToConsole(message); } EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.Information, message)); EventLogger.WriteLogEntry(message, EventLogEntryType.Information); } internal static void LogToConsole(string message) { Console.WriteLine(); Console.WriteLine(message); } internal static void LogWarning(string message, bool displayToConsole) { if (displayToConsole) { EventLogger.LogToConsole(message); } EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.Warning, message)); EventLogger.WriteLogEntry(message, EventLogEntryType.Warning); } internal static void WriteLogEntry(string message, EventLogEntryType entryType) { try { EventLog.WriteEntry(ServiceModelInstallStrings.EventLogSource, message.Trim(), entryType); } catch (ArgumentException e) { // Issues related to message length EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.ErrorWritingEventLogEntry, e.ToString())); } catch (InvalidOperationException e) { // Registry access issues EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.ErrorWritingEventLogEntry, e.ToString())); } catch (Win32Exception e) { // Other OS reported issues EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.ErrorWritingEventLogEntry, e.ToString())); } } internal static void WriteMsiStyleLogEntry(string message) { if (EventLogger.msiStyleLogWriter != null) { EventLogger.msiStyleLogWriter.WriteEntry(message); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
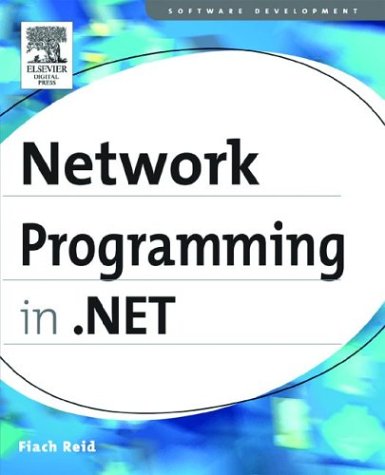
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BStrWrapper.cs
- WindowExtensionMethods.cs
- RuleSettings.cs
- Tag.cs
- ISessionStateStore.cs
- GlyphCache.cs
- ItemsChangedEventArgs.cs
- CompModSwitches.cs
- AnnotationService.cs
- PenLineCapValidation.cs
- StrongNameUtility.cs
- SolidBrush.cs
- DataGridViewCellStateChangedEventArgs.cs
- SafeLibraryHandle.cs
- DataColumnChangeEvent.cs
- EdmFunctionAttribute.cs
- TextBoxView.cs
- DataGridColumnCollection.cs
- XamlWrappingReader.cs
- MethodBody.cs
- FullTextLine.cs
- X509CertificateValidator.cs
- SQLSingle.cs
- BamlResourceDeserializer.cs
- PromptStyle.cs
- ScriptMethodAttribute.cs
- TextParagraphProperties.cs
- ReadOnlyObservableCollection.cs
- Cursors.cs
- ScaleTransform.cs
- XmlSchemaInclude.cs
- KeysConverter.cs
- ReadOnlyDataSourceView.cs
- SqlConnectionString.cs
- _UriTypeConverter.cs
- DesignTimeParseData.cs
- ContentValidator.cs
- GridViewCellAutomationPeer.cs
- UserControl.cs
- HtmlLink.cs
- CustomAssemblyResolver.cs
- AssociatedControlConverter.cs
- WmlControlAdapter.cs
- ChtmlTextWriter.cs
- EntityDataSourceStatementEditor.cs
- QueryReaderSettings.cs
- PropertyOverridesTypeEditor.cs
- BinaryReader.cs
- AsymmetricCryptoHandle.cs
- MSG.cs
- XmlTextReaderImpl.cs
- TemplateInstanceAttribute.cs
- SafeBitVector32.cs
- EditBehavior.cs
- Maps.cs
- StrokeNodeData.cs
- StreamInfo.cs
- CopyNamespacesAction.cs
- ProviderConnectionPointCollection.cs
- StructuredCompositeActivityDesigner.cs
- TabItem.cs
- KeyProperty.cs
- XmlSubtreeReader.cs
- UIAgentRequest.cs
- MenuItemBindingCollection.cs
- XmlSchemaGroupRef.cs
- JournalNavigationScope.cs
- InvokeProviderWrapper.cs
- InheritanceRules.cs
- _DomainName.cs
- ParallelTimeline.cs
- xml.cs
- ConfigurationManagerHelper.cs
- ValidationHelpers.cs
- ModelUIElement3D.cs
- QilNode.cs
- WebSysDisplayNameAttribute.cs
- FormatStringEditor.cs
- DesignRelation.cs
- IssuanceLicense.cs
- ReferentialConstraint.cs
- ComponentManagerBroker.cs
- ChildrenQuery.cs
- CorrelationValidator.cs
- HtmlContainerControl.cs
- InputReportEventArgs.cs
- ListViewTableCell.cs
- XhtmlConformanceSection.cs
- UInt32Converter.cs
- Variant.cs
- TextDpi.cs
- ConfigurationManagerInternalFactory.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- TypeExtensions.cs
- CodeSubDirectory.cs
- Literal.cs
- UnknownExceptionActionHelper.cs
- EDesignUtil.cs
- Aes.cs
- PanelStyle.cs