Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / InputReport.cs / 1305600 / InputReport.cs
using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; using MS.Win32; using System.Windows; namespace System.Windows.Input { ////// The InputReport is an abstract base class for all input that is /// reported to the InputManager. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// [FriendAccessAllowed] internal abstract class InputReport { ////// Constructs ad instance of the InputReport class. /// /// /// The type of input that is being reported. /// /// /// The type of input that is being reported. /// /// /// The mode in which the input is being reported. /// /// /// The time when the input occured. /// ////// This handles critical data in the form of PresentationSource but there are demands on the /// critical data /// [SecurityCritical, SecurityTreatAsSafe] protected InputReport(PresentationSource inputSource, InputType type, InputMode mode, int timestamp) { if (inputSource == null) throw new ArgumentNullException("inputSource"); Validate_InputType( type ); Validate_InputMode( mode ); _inputSource= new SecurityCriticalData(inputSource); _type = type; _mode = mode; _timestamp = timestamp; } /// /// Read-only access to the type of input source that reported input. /// ////// Critical: This element is treated as critical and is not ok to expose. /// A link demand exists but that in itself is not adequate to safeguard this. /// The critical exists to expose users. /// public PresentationSource InputSource { [SecurityCritical] get { return _inputSource.Value; } } ////// Read-only access to the type of input that was reported. /// public InputType Type {get {return _type;}} ////// Read-only access to the mode in which the input was reported. /// public InputMode Mode {get {return _mode;}} ////// Read-only access to the time when the input occured. /// public int Timestamp {get {return _timestamp;}} ////// There is a proscription against using Enum.IsDefined(). (it is slow) /// so we write these PRIVATE validate routines instead. /// private void Validate_InputMode( InputMode mode ) { switch( mode ) { case InputMode.Foreground: case InputMode.Sink: break; default: throw new System.ComponentModel.InvalidEnumArgumentException("mode", (int)mode, typeof(InputMode)); } } ////// There is a proscription against using Enum.IsDefined(). (it is slow) /// so we write these PRIVATE validate routines instead. /// private void Validate_InputType( InputType type ) { switch( type ) { case InputType.Keyboard: case InputType.Mouse: case InputType.Stylus: case InputType.Hid: case InputType.Text: case InputType.Command: break; default: throw new System.ComponentModel.InvalidEnumArgumentException("type", (int)type, typeof(InputType)); } } private SecurityCriticalData_inputSource; private InputType _type; private InputMode _mode; private int _timestamp; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
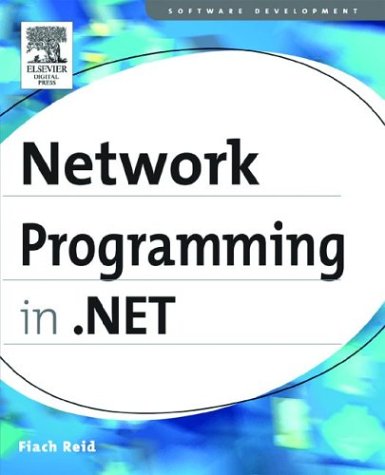
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListItemCollection.cs
- SiteMapDataSourceDesigner.cs
- FixedDocumentPaginator.cs
- FileSystemInfo.cs
- updatecommandorderer.cs
- BitmapSource.cs
- AuthenticateEventArgs.cs
- XamlSerializerUtil.cs
- TemplateComponentConnector.cs
- XmlSchemaComplexContentRestriction.cs
- IISUnsafeMethods.cs
- StaticExtension.cs
- GeometryValueSerializer.cs
- OperationContractAttribute.cs
- InternalBase.cs
- TableParagraph.cs
- Mutex.cs
- UserUseLicenseDictionaryLoader.cs
- TypeDelegator.cs
- SplitterDesigner.cs
- IdentityHolder.cs
- Translator.cs
- BlurBitmapEffect.cs
- AuthorizationRule.cs
- LayoutTable.cs
- BindingSourceDesigner.cs
- Ops.cs
- NamedPipeTransportSecurity.cs
- XMLSchema.cs
- SecurityKeyIdentifier.cs
- TextInfo.cs
- SplitContainer.cs
- ColorAnimationUsingKeyFrames.cs
- LookupNode.cs
- ConditionBrowserDialog.cs
- SystemIcons.cs
- XmlCustomFormatter.cs
- WindowPatternIdentifiers.cs
- newinstructionaction.cs
- WebBrowsableAttribute.cs
- FixedSOMPageElement.cs
- TemplateControlCodeDomTreeGenerator.cs
- ReadOnlyNameValueCollection.cs
- FormatException.cs
- ContentPlaceHolderDesigner.cs
- DataColumnPropertyDescriptor.cs
- BitmapVisualManager.cs
- WpfKnownMemberInvoker.cs
- AnimationClock.cs
- QuaternionKeyFrameCollection.cs
- cryptoapiTransform.cs
- XmlSchemaImport.cs
- contentDescriptor.cs
- ServiceContractListItem.cs
- EdmPropertyAttribute.cs
- ProvidersHelper.cs
- EncoderFallback.cs
- TextEffect.cs
- CollectionViewGroupInternal.cs
- InvalidCastException.cs
- columnmapkeybuilder.cs
- BindingMAnagerBase.cs
- JsonServiceDocumentSerializer.cs
- FixedSOMTableCell.cs
- FlowDecisionDesigner.xaml.cs
- SqlGatherProducedAliases.cs
- ObjectConverter.cs
- GraphicsContainer.cs
- XmlSchemaDocumentation.cs
- BitmapImage.cs
- __FastResourceComparer.cs
- PageRequestManager.cs
- ExtenderControl.cs
- URLAttribute.cs
- _ChunkParse.cs
- ObfuscateAssemblyAttribute.cs
- ConfigurationSettings.cs
- FileDialog.cs
- NativeMethods.cs
- PostBackOptions.cs
- KeyPressEvent.cs
- DBConnection.cs
- SessionEndingCancelEventArgs.cs
- TextDecorationCollectionConverter.cs
- DataKeyArray.cs
- SwitchElementsCollection.cs
- BamlTreeMap.cs
- SettingsPropertyCollection.cs
- BitmapScalingModeValidation.cs
- NumericUpDownAcceleration.cs
- WebPartZoneCollection.cs
- BuildDependencySet.cs
- DATA_BLOB.cs
- MediaTimeline.cs
- AVElementHelper.cs
- Int16Storage.cs
- ReadOnlyCollectionBase.cs
- ResourceDictionary.cs
- EventMap.cs
- EventHandlersStore.cs