Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / Workflow / Activities / Design / ServiceContractListItem.cs / 1305376 / ServiceContractListItem.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Workflow.Activities.Design { using System; using System.Collections.Generic; using System.ComponentModel; using System.Runtime; using System.ServiceModel; using System.Windows.Forms; using System.Workflow.ComponentModel; [ListItemView(typeof(ServiceContractViewControl))] [ListItemDetailView(typeof(ServiceContractDetailViewControl))] class ServiceContractListItem : object { ListBox container; Type contractType; bool isCustomContract; ServiceOperationListItem lastItemAdded; string name; ServiceOperationListItemList operations; public ServiceContractListItem(ListBox container) { if (container == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("container"); } this.operations = new ServiceOperationListItemList(); this.container = container; } public CancelEventHandler Validating; public Type ContractType { get { return contractType; } set { contractType = value; } } public bool IsCustomContract { get { return isCustomContract; } set { isCustomContract = value; } } public string Name { get { return name; } set { name = value; } } public IEnumerableOperations { get { return operations; } } public void AddOperation(ServiceOperationListItem operation) { if (operation == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("operation"); } // Dont add operation if operation.Name is broken if (String.IsNullOrEmpty(operation.Name)) { return; } ServiceOperationListItem cachedItem = this.operations.Find(operation.Name); if (cachedItem != null) { foreach (Activity activity in operation.ImplementingActivities) { if (!cachedItem.ImplementingActivities.Contains(activity)) { cachedItem.ImplementingActivities.Add(activity); } } } else { this.operations.Add(operation); int positionToAddAt = this.container.Items.IndexOf(this) + 1; if (this.operations.Count > 1) { positionToAddAt = this.container.Items.IndexOf(lastItemAdded) + 1; } lastItemAdded = operation; this.container.Items.Insert(positionToAddAt, operation); } } public WorkflowServiceOperationListItem CreateOperation() { WorkflowServiceOperationListItem result = (WorkflowServiceOperationListItem) this.operations.CreateWithUniqueName(); result.Operation.ContractName = this.Name; return result; } public ServiceOperationListItem Find(string operationName) { Fx.Assert(operationName != null, "operationName != null"); return this.operations.Find(operationName); } public void SelectionOperation(ServiceOperationListItem operation) { if (operation == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("operation"); } // Dont select if operation.Name is broken if (String.IsNullOrEmpty(operation.Name)) { return; } ServiceOperationListItem operationListItem = this.operations.Find(operation.Name); if (operationListItem != null) { this.container.SetSelected(container.Items.IndexOf(operationListItem), true); } } public override string ToString() { return Name; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
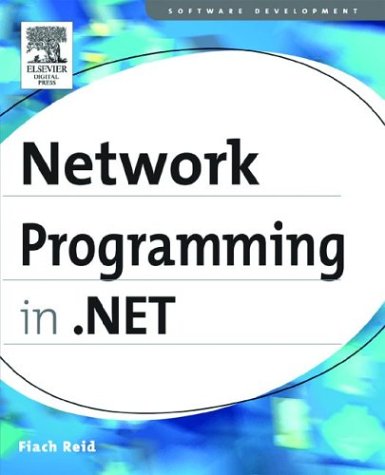
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- APCustomTypeDescriptor.cs
- PropertyEntry.cs
- EncryptedReference.cs
- XDRSchema.cs
- XMLSchema.cs
- ToolStripRendererSwitcher.cs
- CodeChecksumPragma.cs
- StorageModelBuildProvider.cs
- MSAAEventDispatcher.cs
- SystemColors.cs
- EdmRelationshipRoleAttribute.cs
- SqlBuilder.cs
- SoapCommonClasses.cs
- SqlTrackingWorkflowInstance.cs
- FilterException.cs
- EntityDataSourceSelectingEventArgs.cs
- TakeQueryOptionExpression.cs
- DefaultWorkflowLoaderService.cs
- Stackframe.cs
- SerializerDescriptor.cs
- HotCommands.cs
- SingleQueryOperator.cs
- BoundPropertyEntry.cs
- NamespaceInfo.cs
- PointHitTestResult.cs
- RoleGroupCollection.cs
- DataRowComparer.cs
- InvalidOleVariantTypeException.cs
- Native.cs
- PageSetupDialog.cs
- MainMenu.cs
- ComboBox.cs
- XmlIgnoreAttribute.cs
- HandleTable.cs
- SelectorAutomationPeer.cs
- MemberInfoSerializationHolder.cs
- SafeSerializationManager.cs
- FileSystemEventArgs.cs
- CheckBox.cs
- XslCompiledTransform.cs
- PathFigureCollectionConverter.cs
- LayoutDump.cs
- TraceSection.cs
- LoginView.cs
- HttpModuleCollection.cs
- MethodRental.cs
- WebPartZoneDesigner.cs
- XamlReaderHelper.cs
- SmiEventSink.cs
- BitmapInitialize.cs
- PointLight.cs
- NonSerializedAttribute.cs
- StorageSetMapping.cs
- ScrollBarRenderer.cs
- XmlArrayItemAttribute.cs
- ChameleonKey.cs
- StaticContext.cs
- ConversionValidationRule.cs
- StreamingContext.cs
- SmtpTransport.cs
- DataGridTable.cs
- CacheRequest.cs
- ExpressionDumper.cs
- EventsTab.cs
- ModifierKeysValueSerializer.cs
- CroppedBitmap.cs
- FileLevelControlBuilderAttribute.cs
- TdsParserHelperClasses.cs
- XmlSerializationWriter.cs
- KnownIds.cs
- ResourcePool.cs
- MulticastNotSupportedException.cs
- XmlUtil.cs
- WebPartCatalogAddVerb.cs
- WebServiceParameterData.cs
- CompressedStack.cs
- dataobject.cs
- BitmapEffectOutputConnector.cs
- EdmToObjectNamespaceMap.cs
- SuppressMergeCheckAttribute.cs
- GridViewSortEventArgs.cs
- SQLBinaryStorage.cs
- BindingWorker.cs
- Rotation3DKeyFrameCollection.cs
- SwitchDesigner.xaml.cs
- ScrollData.cs
- ForeignConstraint.cs
- WebControlAdapter.cs
- ThreadAbortException.cs
- Model3D.cs
- ErrorProvider.cs
- LeaseManager.cs
- InputLanguageEventArgs.cs
- EntityViewContainer.cs
- CompositeDuplexBindingElement.cs
- ObjectParameter.cs
- SspiHelper.cs
- ActiveXSite.cs
- TextTreeTextNode.cs
- GeometryGroup.cs