Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / ModelProviders / DLinqDataModelProvider.cs / 1305376 / DLinqDataModelProvider.cs
using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Linq; using System.Linq; using System.Reflection; using LinqMetaTable = System.Data.Linq.Mapping.MetaTable; using LinqMetaType = System.Data.Linq.Mapping.MetaType; namespace System.Web.DynamicData.ModelProviders { internal sealed class DLinqDataModelProvider : DataModelProvider { private ReadOnlyCollection_roTables; private Dictionary _columnLookup = new Dictionary (); private Func
Link Menu
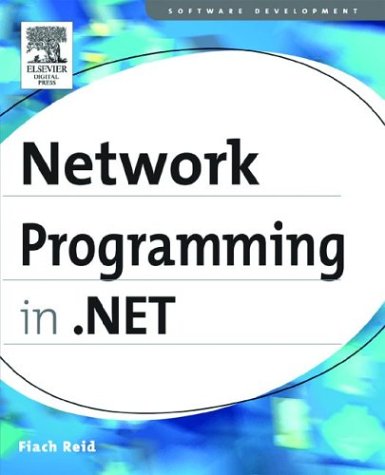
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedOutputStream.cs
- StylusPointPropertyId.cs
- FormatPage.cs
- HttpFileCollection.cs
- DataGridViewLinkColumn.cs
- NonParentingControl.cs
- BrowserInteropHelper.cs
- SmiConnection.cs
- DetectEofStream.cs
- LinkTarget.cs
- UnsafeNativeMethodsCLR.cs
- Event.cs
- Processor.cs
- SQlBooleanStorage.cs
- MSAANativeProvider.cs
- SecurityContextSecurityTokenAuthenticator.cs
- FormViewCommandEventArgs.cs
- StreamWithDictionary.cs
- SQLMoney.cs
- DefaultCommandConverter.cs
- RC2CryptoServiceProvider.cs
- CultureTable.cs
- PartialCachingAttribute.cs
- figurelength.cs
- Utils.cs
- SqlUserDefinedAggregateAttribute.cs
- EndOfStreamException.cs
- FrameworkContentElementAutomationPeer.cs
- ApplicationServiceManager.cs
- DynamicVirtualDiscoSearcher.cs
- OrderedEnumerableRowCollection.cs
- BitmapVisualManager.cs
- Rss20ItemFormatter.cs
- NonDualMessageSecurityOverHttp.cs
- NumericUpDownAcceleration.cs
- ColorConvertedBitmap.cs
- BasicHttpBindingElement.cs
- ApplicationTrust.cs
- HttpInputStream.cs
- BitmapPalette.cs
- BreadCrumbTextConverter.cs
- SchemeSettingElement.cs
- MenuItemStyleCollection.cs
- ConcurrencyMode.cs
- BlurBitmapEffect.cs
- Soap.cs
- VisualStateManager.cs
- ReaderOutput.cs
- TextTabProperties.cs
- DataGridViewRowCollection.cs
- PropertyGeneratedEventArgs.cs
- PagerStyle.cs
- RuleSettingsCollection.cs
- InvalidPropValue.cs
- DataGridViewCellEventArgs.cs
- PropertyFilterAttribute.cs
- SubpageParagraph.cs
- SamlAttributeStatement.cs
- CommandSet.cs
- KeyTimeConverter.cs
- SymbolMethod.cs
- PortCache.cs
- PointF.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- DataServices.cs
- Matrix3D.cs
- NumberFormatter.cs
- SqlClientWrapperSmiStreamChars.cs
- FamilyMap.cs
- DropShadowBitmapEffect.cs
- AuditLevel.cs
- WindowsListViewItemCheckBox.cs
- EntitySqlQueryState.cs
- SynchronizationHandlesCodeDomSerializer.cs
- CodeSnippetTypeMember.cs
- LineServices.cs
- TextInfo.cs
- HMACSHA512.cs
- WindowsGrip.cs
- HtmlElement.cs
- ConfigurationElementCollection.cs
- SafeNativeMethods.cs
- CancelEventArgs.cs
- EventLogPermissionHolder.cs
- XmlSchemaSimpleType.cs
- CuspData.cs
- ConfigurationSettings.cs
- WindowsGraphics.cs
- ControlPaint.cs
- PersonalizationProvider.cs
- SevenBitStream.cs
- XmlElement.cs
- MsmqInputChannelBase.cs
- ScriptDescriptor.cs
- HelpProvider.cs
- ExpressionBindingCollection.cs
- FSWPathEditor.cs
- Line.cs
- XmlElementAttribute.cs
- ExplicitDiscriminatorMap.cs