Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / Mobile / DeviceFilterDictionary.cs / 1305376 / DeviceFilterDictionary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Mobile { using System.Web; using System.Collections; using System.Reflection; using System.Diagnostics; using System.ComponentModel; [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal class DeviceFilterDictionary { internal class ComparisonEvaluator { internal readonly String capabilityName; internal readonly String capabilityArgument; internal ComparisonEvaluator(String name, String argument) { Debug.Assert(name != null); capabilityName = name; capabilityArgument = argument; } } private Hashtable _comparisonEvaluators = null; private Hashtable _delegateEvaluators = null; internal DeviceFilterDictionary() { _comparisonEvaluators = new Hashtable(); _delegateEvaluators = new Hashtable(); } internal DeviceFilterDictionary(DeviceFilterDictionary original) { _comparisonEvaluators = (Hashtable)original._comparisonEvaluators.Clone(); _delegateEvaluators = (Hashtable)original._delegateEvaluators.Clone(); } internal void AddCapabilityDelegate(String delegateName, MobileCapabilities.EvaluateCapabilitiesDelegate evaluator) { _delegateEvaluators[delegateName] = evaluator; } private void CheckForComparisonDelegateLoops(String delegateName) { String nextDelegateName = delegateName; Hashtable alreadyReferencedDelegates = new Hashtable(); while(true) { ComparisonEvaluator nextComparisonEvaluator = (ComparisonEvaluator)_comparisonEvaluators[nextDelegateName]; if(nextComparisonEvaluator == null) { break; } if(alreadyReferencedDelegates.Contains(nextDelegateName)) { String msg = SR.GetString(SR.DevFiltDict_FoundLoop, nextComparisonEvaluator.capabilityName, delegateName); throw new Exception(msg); } alreadyReferencedDelegates[nextDelegateName] = null; nextDelegateName = nextComparisonEvaluator.capabilityName; } } internal void AddComparisonDelegate(String delegateName, String comparisonName, String argument) { _comparisonEvaluators[delegateName] = new ComparisonEvaluator(comparisonName, argument); CheckForComparisonDelegateLoops(delegateName); } internal bool FindComparisonEvaluator(String evaluatorName, out String capabilityName, out String capabilityArgument) { capabilityName = null; capabilityArgument = null; ComparisonEvaluator evaluator = (ComparisonEvaluator)_comparisonEvaluators[evaluatorName]; if(evaluator == null) { return false; } capabilityName = evaluator.capabilityName; capabilityArgument = evaluator.capabilityArgument; return true; } internal bool FindDelegateEvaluator(String evaluatorName, out MobileCapabilities.EvaluateCapabilitiesDelegate evaluatorDelegate) { evaluatorDelegate = null; MobileCapabilities.EvaluateCapabilitiesDelegate evaluator; evaluator = (MobileCapabilities.EvaluateCapabilitiesDelegate) _delegateEvaluators[evaluatorName]; if(evaluator == null) { return false; } evaluatorDelegate = evaluator; return true; } internal bool IsComparisonEvaluator(String evaluatorName) { return _comparisonEvaluators.Contains(evaluatorName); } internal bool IsDelegateEvaluator(String evaluatorName) { return _delegateEvaluators.Contains(evaluatorName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
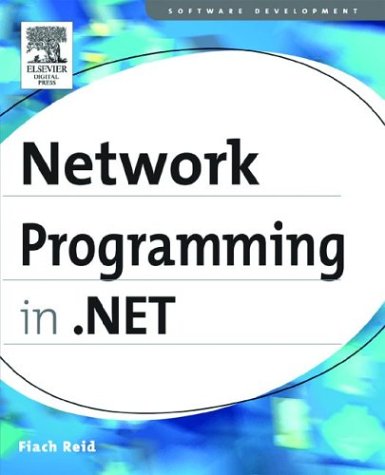
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateControl.cs
- Content.cs
- wmiutil.cs
- SmiTypedGetterSetter.cs
- DateBoldEvent.cs
- Span.cs
- ListControlConvertEventArgs.cs
- XmlSchemaSubstitutionGroup.cs
- XMLSchema.cs
- DataRecordInternal.cs
- ApplicationBuildProvider.cs
- SmtpDigestAuthenticationModule.cs
- MouseWheelEventArgs.cs
- StdValidatorsAndConverters.cs
- TransactionManagerProxy.cs
- TrailingSpaceComparer.cs
- Matrix.cs
- Win32.cs
- HandlerMappingMemo.cs
- PaginationProgressEventArgs.cs
- Literal.cs
- PropertyKey.cs
- CorrelationValidator.cs
- RijndaelManagedTransform.cs
- DataTableReaderListener.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- UndirectedGraph.cs
- EncodingConverter.cs
- CmsInterop.cs
- SchemaImporterExtensionsSection.cs
- SymbolEqualComparer.cs
- ToolStripProgressBar.cs
- LinkTarget.cs
- BrushValueSerializer.cs
- StrokeDescriptor.cs
- ValidationResult.cs
- OleDbRowUpdatingEvent.cs
- DataRelationPropertyDescriptor.cs
- FlowDocumentView.cs
- PointLight.cs
- PersonalizationProviderHelper.cs
- ImageMapEventArgs.cs
- SQLByte.cs
- ConfigXmlDocument.cs
- UserPreferenceChangedEventArgs.cs
- IWorkflowDebuggerService.cs
- XmlHierarchicalDataSourceView.cs
- AdapterDictionary.cs
- DragEventArgs.cs
- Win32SafeHandles.cs
- ClientConfigurationHost.cs
- XpsSerializationManagerAsync.cs
- StylusEditingBehavior.cs
- TCPClient.cs
- StandardOleMarshalObject.cs
- StringValidatorAttribute.cs
- StyleConverter.cs
- XPathNodeHelper.cs
- InternalPermissions.cs
- CodeTypeParameter.cs
- DataGridDetailsPresenterAutomationPeer.cs
- WizardPanel.cs
- InkPresenterAutomationPeer.cs
- LinqDataSourceStatusEventArgs.cs
- ToolStripDropDownMenu.cs
- ConcurrentBag.cs
- GridViewSelectEventArgs.cs
- SiteMapDataSourceView.cs
- BindingCollection.cs
- TextParaLineResult.cs
- MemberJoinTreeNode.cs
- HashSetDebugView.cs
- MessageSecurityOverHttp.cs
- ContentDesigner.cs
- XmlFormatWriterGenerator.cs
- FamilyMap.cs
- CodeTypeOfExpression.cs
- DockingAttribute.cs
- DataControlButton.cs
- ApplicationCommands.cs
- TreeSet.cs
- DBSchemaRow.cs
- FixedTextPointer.cs
- AtomPub10ServiceDocumentFormatter.cs
- MessageAction.cs
- NamespaceInfo.cs
- PaperSize.cs
- Metafile.cs
- ErrorFormatter.cs
- SqlServer2KCompatibilityAnnotation.cs
- AutomationPropertyInfo.cs
- RuleSettingsCollection.cs
- EventDescriptor.cs
- CompositeTypefaceMetrics.cs
- PropertyGridCommands.cs
- UIElementPropertyUndoUnit.cs
- FileLoadException.cs
- keycontainerpermission.cs
- SchemaReference.cs
- PrintingPermission.cs