Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / wmiutil.cs / 1305376 / wmiutil.cs
using System; using System.Runtime.InteropServices; using System.Runtime.Versioning; using WbemClient_v1; namespace System.Management { [ComImport, Guid("87A5AD68-A38A-43ef-ACA9-EFE910E5D24C"), InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] internal interface IWmiEventSource { [PreserveSig] void Indicate(IntPtr pIWbemClassObject); [PreserveSig] void SetStatus( int lFlags, int hResult, [MarshalAs(UnmanagedType.BStr)] string strParam , IntPtr pObjParam ); } #if USEIWOS // The following is a manually defined wrapper for IWbemObjectSink // since the size_is attribute cannot be dealt with by TlbImp. [Guid("7c857801-7381-11cf-884d-00aa004b2e24"), InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] public interface IWbemObjectSink { [PreserveSig] void Indicate( long lObjectCount, [MarshalAs(UnmanagedType.Interface, SizeParamIndex=0)] IWbemClassObject [] apObjArray ); [PreserveSig] void SetStatus( long lFlags, int hResult, [MarshalAs(UnmanagedType.BStr)] string strParam, [MarshalAs(UnmanagedType.Interface)] IWbemClassObject pObjParam ); }; #endif //Class for calling GetErrorInfo from managed code class WbemErrorInfo { public static IWbemClassObjectFreeThreaded GetErrorInfo() { IErrorInfo errorInfo = GetErrorInfo(0); if(null != errorInfo) { IntPtr pUnk = Marshal.GetIUnknownForObject(errorInfo); IntPtr pIWbemClassObject; Marshal.QueryInterface(pUnk, ref IWbemClassObjectFreeThreaded.IID_IWbemClassObject, out pIWbemClassObject); Marshal.Release(pUnk); // The IWbemClassObjectFreeThreaded instance will own reference count on pIWbemClassObject if(pIWbemClassObject != IntPtr.Zero) return new IWbemClassObjectFreeThreaded(pIWbemClassObject); } return null; } [ResourceExposure( ResourceScope.None),DllImport("oleaut32.dll", PreserveSig=false)] static extern IErrorInfo GetErrorInfo(int reserved); } //RCW for IErrorInfo [ComImport] [Guid("1CF2B120-547D-101B-8E65-08002B2BD119")] [InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] internal interface IErrorInfo { Guid GetGUID(); [return:MarshalAs(UnmanagedType.BStr)] string GetSource(); [return:MarshalAs(UnmanagedType.BStr)] string GetDescription(); [return:MarshalAs(UnmanagedType.BStr)] string GetHelpFile(); uint GetHelpContext(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Runtime.InteropServices; using System.Runtime.Versioning; using WbemClient_v1; namespace System.Management { [ComImport, Guid("87A5AD68-A38A-43ef-ACA9-EFE910E5D24C"), InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] internal interface IWmiEventSource { [PreserveSig] void Indicate(IntPtr pIWbemClassObject); [PreserveSig] void SetStatus( int lFlags, int hResult, [MarshalAs(UnmanagedType.BStr)] string strParam , IntPtr pObjParam ); } #if USEIWOS // The following is a manually defined wrapper for IWbemObjectSink // since the size_is attribute cannot be dealt with by TlbImp. [Guid("7c857801-7381-11cf-884d-00aa004b2e24"), InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] public interface IWbemObjectSink { [PreserveSig] void Indicate( long lObjectCount, [MarshalAs(UnmanagedType.Interface, SizeParamIndex=0)] IWbemClassObject [] apObjArray ); [PreserveSig] void SetStatus( long lFlags, int hResult, [MarshalAs(UnmanagedType.BStr)] string strParam, [MarshalAs(UnmanagedType.Interface)] IWbemClassObject pObjParam ); }; #endif //Class for calling GetErrorInfo from managed code class WbemErrorInfo { public static IWbemClassObjectFreeThreaded GetErrorInfo() { IErrorInfo errorInfo = GetErrorInfo(0); if(null != errorInfo) { IntPtr pUnk = Marshal.GetIUnknownForObject(errorInfo); IntPtr pIWbemClassObject; Marshal.QueryInterface(pUnk, ref IWbemClassObjectFreeThreaded.IID_IWbemClassObject, out pIWbemClassObject); Marshal.Release(pUnk); // The IWbemClassObjectFreeThreaded instance will own reference count on pIWbemClassObject if(pIWbemClassObject != IntPtr.Zero) return new IWbemClassObjectFreeThreaded(pIWbemClassObject); } return null; } [ResourceExposure( ResourceScope.None),DllImport("oleaut32.dll", PreserveSig=false)] static extern IErrorInfo GetErrorInfo(int reserved); } //RCW for IErrorInfo [ComImport] [Guid("1CF2B120-547D-101B-8E65-08002B2BD119")] [InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] internal interface IErrorInfo { Guid GetGUID(); [return:MarshalAs(UnmanagedType.BStr)] string GetSource(); [return:MarshalAs(UnmanagedType.BStr)] string GetDescription(); [return:MarshalAs(UnmanagedType.BStr)] string GetHelpFile(); uint GetHelpContext(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
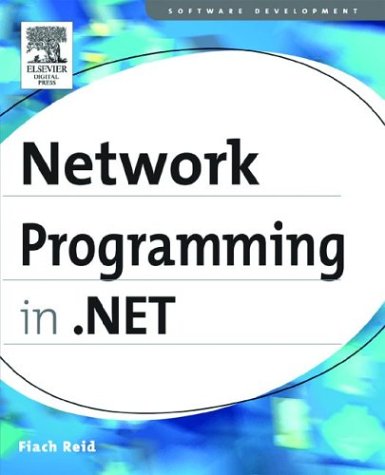
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SplitterCancelEvent.cs
- HttpRawResponse.cs
- CodeComment.cs
- keycontainerpermission.cs
- XsltQilFactory.cs
- ConstructorNeedsTagAttribute.cs
- DependencyPropertyHelper.cs
- PackageDigitalSignatureManager.cs
- SqlWriter.cs
- JoinGraph.cs
- MethodToken.cs
- DrawListViewColumnHeaderEventArgs.cs
- TableAdapterManagerGenerator.cs
- TreeNodeStyleCollectionEditor.cs
- ProviderConnectionPointCollection.cs
- MarshalByValueComponent.cs
- XmlJsonWriter.cs
- HostUtils.cs
- GroupPartitionExpr.cs
- RegisteredArrayDeclaration.cs
- ToolStripPanel.cs
- OdbcConnectionString.cs
- ExeConfigurationFileMap.cs
- PolyQuadraticBezierSegment.cs
- KeyedCollection.cs
- PixelFormat.cs
- ReadOnlyHierarchicalDataSourceView.cs
- EllipticalNodeOperations.cs
- XslVisitor.cs
- OrderingInfo.cs
- TypeSystem.cs
- BooleanExpr.cs
- ActivityBindForm.cs
- WindowAutomationPeer.cs
- CodeTypeParameter.cs
- Pair.cs
- BatchStream.cs
- TailPinnedEventArgs.cs
- ThemeableAttribute.cs
- AtomServiceDocumentSerializer.cs
- EditorZone.cs
- RequestCache.cs
- Matrix.cs
- SQLBinaryStorage.cs
- MasterPage.cs
- LinqDataSourceInsertEventArgs.cs
- ConfigLoader.cs
- XmlSchema.cs
- SerializationEventsCache.cs
- RTTrackingProfile.cs
- ListenerElementsCollection.cs
- BoundingRectTracker.cs
- Encoder.cs
- UnsafeNativeMethods.cs
- SqlDependency.cs
- WindowsIdentity.cs
- WebPartConnectionCollection.cs
- ResourceCodeDomSerializer.cs
- TextDecoration.cs
- DesignerAttribute.cs
- SQLString.cs
- HttpModulesSection.cs
- MatrixTransform3D.cs
- MergePropertyDescriptor.cs
- WebPartCancelEventArgs.cs
- PageContentCollection.cs
- SafeRightsManagementQueryHandle.cs
- DesignTable.cs
- DataSet.cs
- AutoGeneratedFieldProperties.cs
- HiddenFieldPageStatePersister.cs
- JsonQueryStringConverter.cs
- ColumnMapTranslator.cs
- PageAdapter.cs
- XamlGridLengthSerializer.cs
- PaginationProgressEventArgs.cs
- UnmanagedMemoryAccessor.cs
- WinCategoryAttribute.cs
- PerCallInstanceContextProvider.cs
- RoleBoolean.cs
- SqlMethodTransformer.cs
- ActivatedMessageQueue.cs
- OdbcDataAdapter.cs
- TextPatternIdentifiers.cs
- VarRemapper.cs
- DbModificationClause.cs
- MetadataCache.cs
- EpmContentDeSerializerBase.cs
- TdsParserSessionPool.cs
- base64Transforms.cs
- EmulateRecognizeCompletedEventArgs.cs
- WorkflowServiceHostFactory.cs
- Normalization.cs
- __TransparentProxy.cs
- HttpCookiesSection.cs
- PropertyEmitter.cs
- DynamicActionMessageFilter.cs
- CompositeControl.cs
- OleDbConnection.cs
- UniqueConstraint.cs