Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / ResponseBodyWriter.cs / 1305376 / ResponseBodyWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a base class for DataWeb services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections; using System.Data.Services.Providers; using System.Data.Services.Serializers; using System.Diagnostics; using System.IO; using System.Text; ////// Use this class to encapsulate writing the body of the outgoing response /// for a data request. /// internal class ResponseBodyWriter { ///Encoding, if available. private readonly Encoding encoding; ///Whether private readonly bool hasMoved; ///has already moved. Host for the request being processed. private readonly IDataService service; ///Enumerator for results. private readonly IEnumerator queryResults; ///Description of request made to the system. private readonly RequestDescription requestDescription; ///Content format for response. private readonly ContentFormat responseFormat; ///If the target is a Media Resource, this holds the read stream for the Media Resource. private Stream mediaResourceStream; ///Initializes a new /// Encoding, if available. /// Whetherthat can write the body of a response. has already moved. /// Service for the request being processed. /// Enumerator for results. /// Description of request made to the system. /// Content format for response. internal ResponseBodyWriter( Encoding encoding, bool hasMoved, IDataService service, IEnumerator queryResults, RequestDescription requestDescription, ContentFormat responseFormat) { Debug.Assert(responseFormat != ContentFormat.Unknown, "responseFormat != ContentFormat.Unknown"); this.encoding = encoding; this.hasMoved = hasMoved; this.service = service; this.queryResults = queryResults; this.requestDescription = requestDescription; this.responseFormat = responseFormat; if (this.requestDescription.TargetKind == RequestTargetKind.MediaResource) { // Note that GetReadStream will set the ResponseETag before it returns this.mediaResourceStream = service.StreamProvider.GetReadStream(this.queryResults.Current, this.service.OperationContext); } } /// Gets the absolute URI to the service. internal Uri AbsoluteServiceUri { get { return this.service.OperationContext.AbsoluteServiceUri; } } ///Gets the internal DataServiceHostWrapper Host { get { return this.service.OperationContext.Host; } } ///for this response. Gets the internal DataServiceProviderWrapper Provider { get { return this.service.Provider; } } ///for this response. Writes the request body to the specified /// Stream to write to. internal void Write(Stream stream) { Debug.Assert(stream != null, "stream != null"); IExceptionWriter exceptionWriter = null; try { switch (this.responseFormat) { case ContentFormat.Binary: Debug.Assert( this.requestDescription.TargetKind == RequestTargetKind.OpenPropertyValue || this.requestDescription.TargetKind == RequestTargetKind.PrimitiveValue || this.requestDescription.TargetKind == RequestTargetKind.MediaResource, this.requestDescription.TargetKind + " is PrimitiveValue or OpenPropertyValue or StreamPropertyValue"); BinarySerializer binarySerializer = new BinarySerializer(stream); exceptionWriter = binarySerializer; if (this.requestDescription.TargetKind == RequestTargetKind.MediaResource) { Debug.Assert(this.mediaResourceStream != null, "this.mediaResourceStream != null"); binarySerializer.WriteRequest(this.mediaResourceStream, this.service.StreamProvider.StreamBufferSize); } else { binarySerializer.WriteRequest(this.queryResults.Current); } break; case ContentFormat.Text: Debug.Assert( this.requestDescription.TargetKind == RequestTargetKind.OpenPropertyValue || this.requestDescription.TargetKind == RequestTargetKind.PrimitiveValue, this.requestDescription.TargetKind + " is PrimitiveValue or OpenPropertyValue"); TextSerializer textSerializer = new TextSerializer(stream, this.encoding); exceptionWriter = textSerializer; textSerializer.WriteRequest(this.queryResults.Current); break; case ContentFormat.Atom: case ContentFormat.Json: case ContentFormat.PlainXml: Debug.Assert(this.requestDescription.TargetKind != RequestTargetKind.PrimitiveValue, "this.requestDescription.TargetKind != RequestTargetKind.PrimitiveValue"); Debug.Assert(this.requestDescription.TargetKind != RequestTargetKind.OpenPropertyValue, "this.requestDescription.TargetKind != RequestTargetKind.OpenPropertyValue"); Debug.Assert(this.requestDescription.TargetKind != RequestTargetKind.Metadata, "this.requestDescription.TargetKind != RequestTargetKind.Metadata"); if (this.requestDescription.TargetKind == RequestTargetKind.ServiceDirectory) { if (this.responseFormat == ContentFormat.Json) { JsonServiceDocumentSerializer serviceSerializer = new JsonServiceDocumentSerializer(stream, this.Provider, this.encoding); exceptionWriter = serviceSerializer; serviceSerializer.WriteRequest(); break; } else { AtomServiceDocumentSerializer serviceSerializer = new AtomServiceDocumentSerializer(stream, this.AbsoluteServiceUri, this.Provider, this.encoding); exceptionWriter = serviceSerializer; serviceSerializer.WriteRequest(this.service); } } else { Serializer serializer; if (ContentFormat.Json == this.responseFormat) { serializer = new JsonSerializer(this.requestDescription, stream, this.AbsoluteServiceUri, this.service, this.encoding, this.Host.ResponseETag); } else if (ContentFormat.PlainXml == this.responseFormat) { serializer = new PlainXmlSerializer(this.requestDescription, this.AbsoluteServiceUri, this.service, stream, this.encoding); } else { Debug.Assert( this.requestDescription.TargetKind == RequestTargetKind.OpenProperty || this.requestDescription.TargetKind == RequestTargetKind.Resource, "TargetKind " + this.requestDescription.TargetKind + " == Resource || OpenProperty -- POX should have handled it otherwise."); serializer = new SyndicationSerializer( this.requestDescription, this.AbsoluteServiceUri, this.service, stream, this.encoding, this.Host.ResponseETag, new Atom10FormatterFactory()); } exceptionWriter = serializer; Debug.Assert(exceptionWriter != null, "this.exceptionWriter != null"); serializer.WriteRequest(this.queryResults, this.hasMoved); } break; default: Debug.Assert( this.responseFormat == ContentFormat.MetadataDocument, "responseFormat(" + this.responseFormat + ") == ContentFormat.MetadataDocument -- otherwise exception should have been thrown before"); Debug.Assert(this.requestDescription.TargetKind == RequestTargetKind.Metadata, "this.requestDescription.TargetKind == RequestTargetKind.Metadata"); MetadataSerializer metadataSerializer = new MetadataSerializer(stream, this.AbsoluteServiceUri, this.Provider, this.encoding); exceptionWriter = metadataSerializer; metadataSerializer.WriteRequest(this.service); break; } } catch (Exception exception) { if (!WebUtil.IsCatchableExceptionType(exception)) { throw; } // Only JSON and XML are supported. string contentType = (this.responseFormat == ContentFormat.Json) ? XmlConstants.MimeApplicationJson : XmlConstants.MimeApplicationXml; ErrorHandler.HandleDuringWritingException(exception, this.service, contentType, exceptionWriter); } finally { WebUtil.Dispose(this.queryResults); WebUtil.Dispose(this.mediaResourceStream); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007..
Link Menu
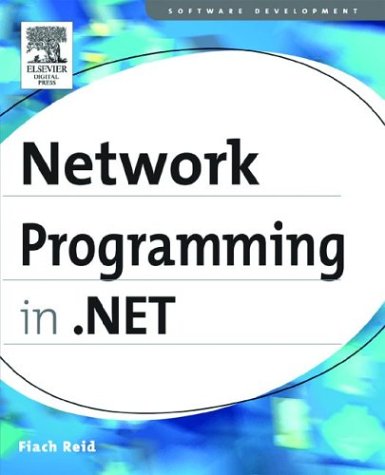
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Paragraph.cs
- NotFiniteNumberException.cs
- PackUriHelper.cs
- DateTimeConstantAttribute.cs
- ColorContextHelper.cs
- RenderTargetBitmap.cs
- ListView.cs
- HtmlGenericControl.cs
- FilterException.cs
- ProxySimple.cs
- ContainerParagraph.cs
- Function.cs
- GeneratedCodeAttribute.cs
- HttpModule.cs
- StretchValidation.cs
- ExpressionsCollectionConverter.cs
- TileBrush.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- DataServiceHostFactory.cs
- TextRenderer.cs
- WorkflowEventArgs.cs
- FontSourceCollection.cs
- EventKeyword.cs
- WebPartHelpVerb.cs
- VariableQuery.cs
- StackOverflowException.cs
- ListViewItem.cs
- SmiMetaData.cs
- EdgeModeValidation.cs
- SimpleHandlerBuildProvider.cs
- PackageRelationshipSelector.cs
- MissingManifestResourceException.cs
- PathGeometry.cs
- NamedPipeProcessProtocolHandler.cs
- FigureParagraph.cs
- NotFiniteNumberException.cs
- QuadraticEase.cs
- PathSegmentCollection.cs
- PointLight.cs
- EncoderExceptionFallback.cs
- AppDomain.cs
- LocatorGroup.cs
- ISAPIApplicationHost.cs
- WebPartVerb.cs
- CommonDialog.cs
- XmlDocumentFieldSchema.cs
- adornercollection.cs
- WebResourceAttribute.cs
- RenderContext.cs
- SessionSwitchEventArgs.cs
- CompModSwitches.cs
- C14NUtil.cs
- WebPartManager.cs
- ContentPlaceHolder.cs
- ClassHandlersStore.cs
- ResourcePool.cs
- SingleTagSectionHandler.cs
- SecurityKeyUsage.cs
- Schema.cs
- TailCallAnalyzer.cs
- SimpleBitVector32.cs
- ValueQuery.cs
- ScopelessEnumAttribute.cs
- InputLangChangeRequestEvent.cs
- NativeCppClassAttribute.cs
- AspNetRouteServiceHttpHandler.cs
- PathFigureCollectionConverter.cs
- ModelTreeEnumerator.cs
- ToolStripPanelRenderEventArgs.cs
- TemporaryBitmapFile.cs
- Event.cs
- ScriptControl.cs
- SourceLocationProvider.cs
- VectorAnimationBase.cs
- Vector3DCollection.cs
- ServerValidateEventArgs.cs
- SecurityElementBase.cs
- ToolStripLocationCancelEventArgs.cs
- BamlRecordReader.cs
- BaseComponentEditor.cs
- TemplateAction.cs
- SvcMapFile.cs
- DataControlLinkButton.cs
- RoleExceptions.cs
- ZeroOpNode.cs
- ViewLoader.cs
- ControlCollection.cs
- AppSecurityManager.cs
- ApplicationActivator.cs
- PropertyCondition.cs
- CredentialCache.cs
- KeyEventArgs.cs
- SimplePropertyEntry.cs
- DiagnosticTraceSource.cs
- HtmlInputRadioButton.cs
- Configuration.cs
- StickyNote.cs
- BinaryConverter.cs
- LinqDataSourceSelectEventArgs.cs
- Int32Converter.cs