Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmSourceTree.cs / 1305376 / EpmSourceTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Tree for managing SourceNames on EntityPropertyMappingAttributes // for a ResourceType. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System.Collections.Generic; using System.Diagnostics; using System.Linq; #if ASTORIA_CLIENT using System.Data.Services.Client; #else using System.Data.Services; #endif ////// Tree representing the sourceName properties in all the EntityPropertyMappingAttributes for a resource type /// internal sealed class EpmSourceTree { #region Fields ///Root of the tree private readonly EpmSourcePathSegment root; ///private readonly EpmTargetTree epmTargetTree; #endregion /// corresponding to this tree Default constructor creates a null root /// Target xml tree internal EpmSourceTree(EpmTargetTree epmTargetTree) { this.root = new EpmSourcePathSegment(""); this.epmTargetTree = epmTargetTree; } #region Properties ////// Root of the tree /// internal EpmSourcePathSegment Root { get { return this.root; } } #endregion ////// Adds a path to the source and target tree which is obtained by looking at the EntityPropertyMappingAttribute in the /// EnitityPropertyMappingInfo holding the source path internal void Add(EntityPropertyMappingInfo epmInfo) { String sourceName = epmInfo.Attribute.SourcePath; EpmSourcePathSegment currentProperty = this.Root; IList/// activeSubProperties = currentProperty.SubProperties; EpmSourcePathSegment foundProperty = null; Debug.Assert(!String.IsNullOrEmpty(sourceName), "Must have been validated during EntityPropertyMappingAttribute construction"); foreach (String propertyName in sourceName.Split('/')) { if (propertyName.Length == 0) { throw new InvalidOperationException(Strings.EpmSourceTree_InvalidSourcePath(epmInfo.DefiningType.Name, sourceName)); } foundProperty = activeSubProperties.SingleOrDefault(e => e.PropertyName == propertyName); if (foundProperty != null) { currentProperty = foundProperty; } else { currentProperty = new EpmSourcePathSegment(propertyName); activeSubProperties.Add(currentProperty); } activeSubProperties = currentProperty.SubProperties; } // Two EpmAttributes with same PropertyName in the same ResourceType, this could be a result of inheritance if (foundProperty != null) { Debug.Assert(Object.ReferenceEquals(foundProperty, currentProperty), "currentProperty variable should have been updated already to foundProperty"); // Check for duplicates on the same entity type #if !ASTORIA_CLIENT Debug.Assert(foundProperty.SubProperties.Count == 0, "If non-leaf, it means we allowed complex type to be a leaf node"); if (foundProperty.EpmInfo.DefiningType == epmInfo.DefiningType) { throw new InvalidOperationException(Strings.EpmSourceTree_DuplicateEpmAttrsWithSameSourceName(epmInfo.Attribute.SourcePath, epmInfo.DefiningType.Name)); } #else if (foundProperty.EpmInfo.DefiningType.Name == epmInfo.DefiningType.Name) { throw new InvalidOperationException(Strings.EpmSourceTree_DuplicateEpmAttrsWithSameSourceName(epmInfo.Attribute.SourcePath, epmInfo.DefiningType.Name)); } #endif // In case of inheritance, we need to remove the node from target tree which was mapped to base type property this.epmTargetTree.Remove(foundProperty.EpmInfo); } currentProperty.EpmInfo = epmInfo; this.epmTargetTree.Add(epmInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
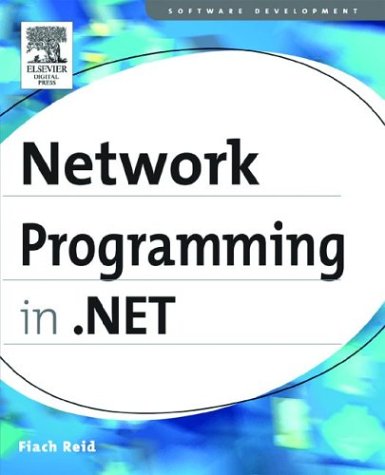
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceLockQueryResult.cs
- CodeAttributeDeclarationCollection.cs
- ValidationError.cs
- RuntimeCompatibilityAttribute.cs
- EventLogPermissionHolder.cs
- SafeFindHandle.cs
- SubpageParagraph.cs
- TreeNodeCollection.cs
- SmtpReplyReaderFactory.cs
- ServiceObjectContainer.cs
- QuotedPrintableStream.cs
- EndOfStreamException.cs
- MemberDescriptor.cs
- XmlRootAttribute.cs
- XmlILCommand.cs
- PagedControl.cs
- NativeMethods.cs
- DataTableTypeConverter.cs
- CaseKeyBox.ViewModel.cs
- StringPropertyBuilder.cs
- SymbolTable.cs
- GroupByExpressionRewriter.cs
- GrabHandleGlyph.cs
- AsyncOperationLifetimeManager.cs
- Canonicalizers.cs
- MsmqMessage.cs
- SHA384Managed.cs
- VirtualizedItemPattern.cs
- MdiWindowListStrip.cs
- EntityKey.cs
- ArgumentOutOfRangeException.cs
- AuthenticationService.cs
- SqlVersion.cs
- FolderLevelBuildProviderCollection.cs
- SapiRecoInterop.cs
- AuthenticationConfig.cs
- SAPIEngineTypes.cs
- DirectoryObjectSecurity.cs
- LogicalTreeHelper.cs
- StylusPoint.cs
- TextControl.cs
- ToolConsole.cs
- DrawingVisual.cs
- AnimatedTypeHelpers.cs
- Matrix3DStack.cs
- EdmSchemaAttribute.cs
- DrawingContextDrawingContextWalker.cs
- SoapAttributeAttribute.cs
- StartFileNameEditor.cs
- StringToken.cs
- ScrollContentPresenter.cs
- UriExt.cs
- _PooledStream.cs
- LOSFormatter.cs
- AnchoredBlock.cs
- StyleCollection.cs
- HighlightComponent.cs
- FormViewInsertedEventArgs.cs
- AppDomainAttributes.cs
- Hashtable.cs
- EntityModelBuildProvider.cs
- TemplateAction.cs
- EventLogException.cs
- ClickablePoint.cs
- Trace.cs
- ToolboxDataAttribute.cs
- RewritingProcessor.cs
- GrammarBuilderDictation.cs
- HttpWrapper.cs
- MetadataUtilsSmi.cs
- RenderCapability.cs
- PropertyEntry.cs
- TripleDESCryptoServiceProvider.cs
- Pair.cs
- ListItemParagraph.cs
- PkcsUtils.cs
- GlyphInfoList.cs
- OletxDependentTransaction.cs
- InstallHelper.cs
- GridViewHeaderRowPresenter.cs
- CellParaClient.cs
- securitycriticaldataformultiplegetandset.cs
- RichTextBoxDesigner.cs
- ImageListImage.cs
- SoapSchemaImporter.cs
- MenuDesigner.cs
- PeerTransportCredentialType.cs
- PolyBezierSegment.cs
- IgnoreDataMemberAttribute.cs
- DataGridViewCellValidatingEventArgs.cs
- WebPartDisplayModeCancelEventArgs.cs
- LinkedResourceCollection.cs
- ResolvePPIDRequest.cs
- SqlDataSourceStatusEventArgs.cs
- TdsValueSetter.cs
- ItemChangedEventArgs.cs
- ConfigurationValues.cs
- FontDriver.cs
- BaseCodeDomTreeGenerator.cs
- ReceiveSecurityHeaderEntry.cs