Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ProjectionPlan.cs / 1305376 / ProjectionPlan.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class that encapsulates how to materialize an ATOM // entry using a projection. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ///Use this class to store a materialization plan used with projections. internal class ProjectionPlan { #if DEBUG ///Source projection for this plan. internal System.Linq.Expressions.Expression SourceProjection { get; set; } ///Target projection for this plan. internal System.Linq.Expressions.Expression TargetProjection { get; set; } #endif ///Last segment type for query. ///This typically matches the expected element type at runtime. internal Type LastSegmentType { get; set; } ///Provides a method to materialize a payload. internal Func
Link Menu
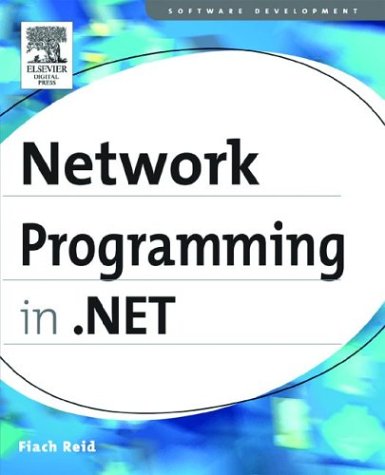
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLDateTimeStorage.cs
- ConditionBrowserDialog.cs
- StateChangeEvent.cs
- WebPartTracker.cs
- DateTimeFormatInfo.cs
- ActiveXHelper.cs
- HttpUnhandledOperationInvoker.cs
- WindowsUpDown.cs
- ExpressionVisitorHelpers.cs
- EllipseGeometry.cs
- DateTimeFormatInfo.cs
- DataGridViewCellParsingEventArgs.cs
- baseaxisquery.cs
- OrderablePartitioner.cs
- DeploymentExceptionMapper.cs
- DesignerForm.cs
- FixedBufferAttribute.cs
- CompoundFileIOPermission.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- SQLDateTimeStorage.cs
- LayoutEngine.cs
- SqlColumnizer.cs
- ICollection.cs
- CustomAttributeFormatException.cs
- PersonalizationEntry.cs
- EntryPointNotFoundException.cs
- RawStylusActions.cs
- WsatStrings.cs
- Validator.cs
- _AutoWebProxyScriptWrapper.cs
- ExpressionNormalizer.cs
- WinFormsUtils.cs
- ReliableRequestSessionChannel.cs
- DrawTreeNodeEventArgs.cs
- DataBinding.cs
- TextFormatterImp.cs
- EntityDataSourceValidationException.cs
- DataGridViewCellStateChangedEventArgs.cs
- PropertyCollection.cs
- X509AsymmetricSecurityKey.cs
- FixedSOMTableCell.cs
- IsolatedStorageFileStream.cs
- DbConnectionPoolIdentity.cs
- XmlSchemaCompilationSettings.cs
- ContextCorrelationInitializer.cs
- PlacementWorkspace.cs
- PrimitiveXmlSerializers.cs
- HandledEventArgs.cs
- TcpAppDomainProtocolHandler.cs
- TripleDES.cs
- OrderByBuilder.cs
- WebPartTransformer.cs
- OdbcConnection.cs
- TablePatternIdentifiers.cs
- FixedPageProcessor.cs
- LayoutEditorPart.cs
- BoundColumn.cs
- RC2.cs
- SocketAddress.cs
- ImageClickEventArgs.cs
- IsolatedStorageFileStream.cs
- Semaphore.cs
- DefaultWorkflowLoaderService.cs
- UnicastIPAddressInformationCollection.cs
- xdrvalidator.cs
- TreeIterator.cs
- DeviceOverridableAttribute.cs
- ObjectReferenceStack.cs
- SoapCodeExporter.cs
- KnownColorTable.cs
- ContainerCodeDomSerializer.cs
- LabelInfo.cs
- TimersDescriptionAttribute.cs
- RetriableClipboard.cs
- DBSchemaRow.cs
- SqlGatherProducedAliases.cs
- ButtonStandardAdapter.cs
- SerialPinChanges.cs
- SecurityVerifiedMessage.cs
- securestring.cs
- DataSourceExpression.cs
- FileVersion.cs
- TrustLevel.cs
- SystemIcmpV4Statistics.cs
- TargetParameterCountException.cs
- ExternalFile.cs
- ValidationHelper.cs
- RenderingBiasValidation.cs
- DataBindingList.cs
- PreviewPageInfo.cs
- BitmapEffectInput.cs
- BackgroundWorker.cs
- WebPartActionVerb.cs
- BrowserCapabilitiesCodeGenerator.cs
- MergeFilterQuery.cs
- SQLConvert.cs
- AnnotationStore.cs
- MediaScriptCommandRoutedEventArgs.cs
- JsonFormatWriterGenerator.cs
- PowerModeChangedEventArgs.cs