Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / ContainerCodeDomSerializer.cs / 1 / ContainerCodeDomSerializer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Reflection; ////// This class is used to serialize things of type "IContainer". We route all containers /// to the designer host's container. /// internal class ContainerCodeDomSerializer : CodeDomSerializer { private const string _containerName = "components"; private static ContainerCodeDomSerializer _defaultSerializer; ////// Retrieves a default static instance of this serializer. /// internal new static ContainerCodeDomSerializer Default { get { if (_defaultSerializer == null) { _defaultSerializer = new ContainerCodeDomSerializer(); } return _defaultSerializer; } } ////// We override this so we can always provide the correct container as a reference. /// protected override object DeserializeInstance(IDesignerSerializationManager manager, Type type, object[] parameters, string name, bool addToContainer) { if (typeof(IContainer).IsAssignableFrom(type)) { object obj = manager.GetService(typeof(IContainer)); if (obj != null) { Trace("Returning IContainer service as container"); manager.SetName(obj, name); return obj; } } Trace("No IContainer service, creating default container."); return base.DeserializeInstance(manager, type, parameters, name, addToContainer); } ////// Serializes the given object into a CodeDom object. We serialize an IContainer by /// declaring an IContainer member variable and then assigning a Container into it. /// public override object Serialize(IDesignerSerializationManager manager, object value) { // See if there is a type declaration on the stack. If there is, create a field representing // the container member variable. CodeTypeDeclaration typeDecl = manager.Context[typeof(CodeTypeDeclaration)] as CodeTypeDeclaration; RootContext rootCxt = manager.Context[typeof(RootContext)] as RootContext; CodeStatementCollection statements = new CodeStatementCollection(); CodeExpression lhs; if (typeDecl != null && rootCxt != null) { CodeMemberField field = new CodeMemberField(typeof(IContainer), _containerName); field.Attributes = MemberAttributes.Private; typeDecl.Members.Add(field); lhs = new CodeFieldReferenceExpression(rootCxt.Expression, _containerName); } else { CodeVariableDeclarationStatement var = new CodeVariableDeclarationStatement(typeof(IContainer), _containerName); statements.Add(var); lhs = new CodeVariableReferenceExpression(_containerName); } // Now create the container SetExpression(manager, value, lhs); CodeObjectCreateExpression objCreate = new CodeObjectCreateExpression(typeof(Container)); CodeAssignStatement assign = new CodeAssignStatement(lhs, objCreate); assign.UserData["IContainer"] = "IContainer"; statements.Add(assign); return statements; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
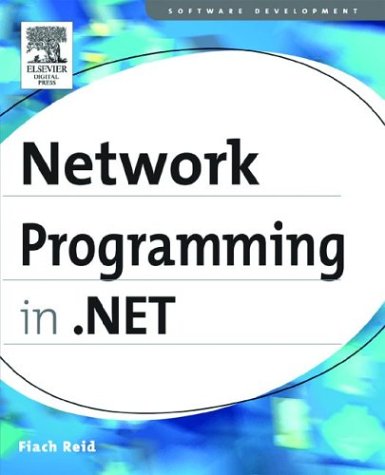
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Task.cs
- MenuItem.cs
- SiteMapDataSource.cs
- Confirm.cs
- PartialArray.cs
- TextViewBase.cs
- SQLRoleProvider.cs
- DesigntimeLicenseContext.cs
- LinearKeyFrames.cs
- Calendar.cs
- DockAndAnchorLayout.cs
- ListBindableAttribute.cs
- List.cs
- DependencyObjectValidator.cs
- _UncName.cs
- IntersectQueryOperator.cs
- HwndSourceKeyboardInputSite.cs
- WebPartMovingEventArgs.cs
- ToolStripPanelRenderEventArgs.cs
- ChannelBinding.cs
- AtomServiceDocumentSerializer.cs
- AutoGeneratedField.cs
- SchemaComplexType.cs
- TokenizerHelper.cs
- RoutedEventConverter.cs
- SchemeSettingElement.cs
- ISFClipboardData.cs
- XmlObjectSerializerReadContextComplex.cs
- Size3D.cs
- SecurityPermission.cs
- FragmentNavigationEventArgs.cs
- TraceSection.cs
- HttpCachePolicyElement.cs
- SoapCommonClasses.cs
- CodeMemberEvent.cs
- ResXDataNode.cs
- Header.cs
- NegotiateStream.cs
- X509Utils.cs
- TableAutomationPeer.cs
- CompilerGlobalScopeAttribute.cs
- ConnectionOrientedTransportElement.cs
- XsltLoader.cs
- InputLanguageEventArgs.cs
- DmlSqlGenerator.cs
- SqlDataSourceEnumerator.cs
- SymDocumentType.cs
- BackgroundWorker.cs
- NoPersistScope.cs
- PathGradientBrush.cs
- BindValidator.cs
- WebExceptionStatus.cs
- CellIdBoolean.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- ControlPropertyNameConverter.cs
- FolderBrowserDialog.cs
- OleDbDataReader.cs
- xml.cs
- MultipartContentParser.cs
- DataControlFieldsEditor.cs
- FlowPosition.cs
- FacetChecker.cs
- ObjectListFieldCollection.cs
- ModulesEntry.cs
- PartManifestEntry.cs
- ContentPosition.cs
- MouseOverProperty.cs
- PerformanceCounter.cs
- ZipFileInfoCollection.cs
- ProfilePropertyNameValidator.cs
- RecognizerStateChangedEventArgs.cs
- Update.cs
- WebSysDescriptionAttribute.cs
- OdbcCommand.cs
- LineSegment.cs
- ParameterCollection.cs
- SmtpAuthenticationManager.cs
- SqlDataSourceView.cs
- InstanceHandle.cs
- Splitter.cs
- SecUtil.cs
- ColumnWidthChangedEvent.cs
- DataGridAddNewRow.cs
- DataMemberConverter.cs
- MsmqNonTransactedPoisonHandler.cs
- SchemaObjectWriter.cs
- QilStrConcat.cs
- AnnotationDocumentPaginator.cs
- AddInDeploymentState.cs
- DoubleAnimationClockResource.cs
- IntegerValidator.cs
- DocumentReferenceCollection.cs
- StringToken.cs
- SafeProcessHandle.cs
- ObjectDataSourceSelectingEventArgs.cs
- ECDiffieHellmanPublicKey.cs
- ToolStripManager.cs
- ConnectionPoint.cs
- StandardCommands.cs
- TemplateControlCodeDomTreeGenerator.cs