Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / CodeExpressionRuleDeclaration.cs / 1305376 / CodeExpressionRuleDeclaration.cs
namespace System.Workflow.Activities { using System; using System.Collections; using System.Collections.Generic; using System.CodeDom; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Workflow.ComponentModel; using System.Workflow.Runtime; using System.Workflow.ComponentModel.Compiler; using System.Workflow.ComponentModel.Serialization; using System.Workflow.Runtime.DebugEngine; [ToolboxItem(false)] [ActivityValidator(typeof(CodeConditionValidator))] [SRDisplayName(SR.CodeConditionDisplayName)] public class CodeCondition : ActivityCondition { public static readonly DependencyProperty ConditionEvent = DependencyProperty.Register("Condition", typeof(EventHandler), typeof(CodeCondition)); [SRDescription(SR.ExpressionDescr)] [SRCategory(SR.Handlers)] [MergableProperty(false)] public event EventHandler Condition { add { base.AddHandler(ConditionEvent, value); } remove { base.RemoveHandler(ConditionEvent, value); } } #region Bind resolution Support protected override object GetBoundValue(ActivityBind bind, Type targetType) { if (bind == null) throw new ArgumentNullException("bind"); if (targetType == null) throw new ArgumentNullException("targetType"); object returnVal = bind; Activity activity = this.ParentDependencyObject as Activity; if (activity != null) returnVal = bind.GetRuntimeValue(activity, targetType); return returnVal; } #endregion public override bool Evaluate(Activity ownerActivity, IServiceProvider provider) { if (provider == null) throw new ArgumentNullException("provider"); ConditionalEventArgs eventArgs = new ConditionalEventArgs(); EventHandler [] eventHandlers = base.GetInvocationList >(CodeCondition.ConditionEvent); IWorkflowDebuggerService workflowDebuggerService = provider.GetService(typeof(IWorkflowDebuggerService)) as IWorkflowDebuggerService; if (eventHandlers != null) { foreach (EventHandler eventHandler in eventHandlers) { if (workflowDebuggerService != null) workflowDebuggerService.NotifyHandlerInvoking(eventHandler); eventHandler(ownerActivity, eventArgs); if (workflowDebuggerService != null) workflowDebuggerService.NotifyHandlerInvoked(); } } return eventArgs.Result; } private class CodeConditionValidator : ConditionValidator { public override ValidationErrorCollection Validate(ValidationManager manager, object obj) { ValidationErrorCollection errors = new ValidationErrorCollection(); errors.AddRange(base.Validate(manager, obj)); CodeCondition codeCondition = obj as CodeCondition; if (codeCondition != null) { if (codeCondition.GetInvocationList >(CodeCondition.ConditionEvent).Length == 0 && codeCondition.GetBinding(CodeCondition.ConditionEvent) == null) { Hashtable hashtable = codeCondition.GetValue(WorkflowMarkupSerializer.EventsProperty) as Hashtable; if (hashtable == null || hashtable["Condition"] == null) errors.Add(ValidationError.GetNotSetValidationError(GetFullPropertyName(manager) + ".Condition")); } } return errors; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
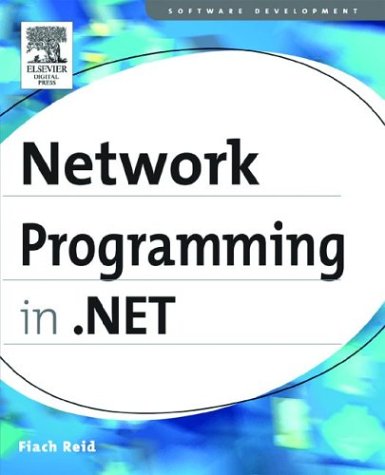
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PolicyManager.cs
- _Events.cs
- __ConsoleStream.cs
- EnumerableRowCollection.cs
- TabItemWrapperAutomationPeer.cs
- validation.cs
- SizeAnimationClockResource.cs
- SystemParameters.cs
- AnchoredBlock.cs
- NativeMethods.cs
- DataGridViewToolTip.cs
- __FastResourceComparer.cs
- OutputScope.cs
- ObjectViewQueryResultData.cs
- PageStatePersister.cs
- TextDecorationLocationValidation.cs
- CapabilitiesAssignment.cs
- HybridObjectCache.cs
- BitmapImage.cs
- WebUtil.cs
- OleDbWrapper.cs
- SafeNativeMethods.cs
- ExtendedProtectionPolicyTypeConverter.cs
- ParamArrayAttribute.cs
- HMACSHA384.cs
- MouseWheelEventArgs.cs
- RegisteredArrayDeclaration.cs
- DefaultPrintController.cs
- KeyToListMap.cs
- UInt32Storage.cs
- IntranetCredentialPolicy.cs
- ProjectionQueryOptionExpression.cs
- CharEntityEncoderFallback.cs
- MasterPageCodeDomTreeGenerator.cs
- DataSourceHelper.cs
- SecUtil.cs
- DrawingGroup.cs
- CacheMemory.cs
- CollectionsUtil.cs
- ItemList.cs
- Matrix.cs
- ChineseLunisolarCalendar.cs
- GZipStream.cs
- DetailsViewInsertedEventArgs.cs
- DispatcherObject.cs
- FillRuleValidation.cs
- HandlerBase.cs
- StringUtil.cs
- TypedRowGenerator.cs
- XmlException.cs
- DurableOperationAttribute.cs
- SocketSettings.cs
- login.cs
- IPAddressCollection.cs
- SafeFindHandle.cs
- MemberCollection.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ActiveXContainer.cs
- NameTable.cs
- FrameworkPropertyMetadata.cs
- StrokeSerializer.cs
- DataColumnMapping.cs
- FaultDesigner.cs
- HelloOperationAsyncResult.cs
- InsufficientMemoryException.cs
- ConditionalAttribute.cs
- RequestNavigateEventArgs.cs
- TaskFactory.cs
- ScriptControlDescriptor.cs
- WindowsContainer.cs
- UpdatePanelControlTrigger.cs
- CompoundFileStreamReference.cs
- UidPropertyAttribute.cs
- XmlAttributeOverrides.cs
- DesignTimeTemplateParser.cs
- KnownColorTable.cs
- ValueTable.cs
- XmlDataImplementation.cs
- AspNetPartialTrustHelpers.cs
- NumberFormatInfo.cs
- CodeRemoveEventStatement.cs
- ConfigurationPropertyAttribute.cs
- TextSpanModifier.cs
- FormViewModeEventArgs.cs
- TreePrinter.cs
- EventRecordWrittenEventArgs.cs
- GeneralTransform.cs
- DataGridViewCellFormattingEventArgs.cs
- ExpressionBindings.cs
- MergeLocalizationDirectives.cs
- ValidationError.cs
- StringToken.cs
- TableLayoutSettingsTypeConverter.cs
- XamlContextStack.cs
- DBSchemaRow.cs
- COM2AboutBoxPropertyDescriptor.cs
- EntityModelBuildProvider.cs
- AudioLevelUpdatedEventArgs.cs
- CodePrimitiveExpression.cs
- AttributeExtensions.cs