Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewToolTip.cs / 1305376 / DataGridViewToolTip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; namespace System.Windows.Forms { public partial class DataGridView { private class DataGridViewToolTip { DataGridView dataGridView = null; ToolTip toolTip = null; private bool toolTipActivated = false; public DataGridViewToolTip(DataGridView dataGridView) { this.dataGridView = dataGridView; } public bool Activated { get { return this.toolTipActivated; } } public ToolTip ToolTip { get { return this.toolTip; } } public void Activate(bool activate) { if (this.dataGridView.DesignMode) { return; } // Create the tool tip handle on demand. if (activate && this.toolTip == null) { this.toolTip = new ToolTip(); this.toolTip.ShowAlways = true; this.toolTip.InitialDelay = 0; this.toolTip.UseFading = false; this.toolTip.UseAnimation = false; this.toolTip.AutoPopDelay = 0; } if (this.dataGridView.IsRestricted) { IntSecurity.AllWindows.Assert(); } try { if (activate) { this.toolTip.Active = true; this.toolTip.Show(this.dataGridView.ToolTipPrivate, this.dataGridView); } else if (this.toolTip != null) { this.toolTip.Hide(this.dataGridView); this.toolTip.Active = false; } } finally { if (this.dataGridView.IsRestricted) { CodeAccessPermission.RevertAssert(); } } this.toolTipActivated = activate; } public void Dispose() { if (this.toolTip != null) { this.toolTip.Dispose(); this.toolTip = null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; namespace System.Windows.Forms { public partial class DataGridView { private class DataGridViewToolTip { DataGridView dataGridView = null; ToolTip toolTip = null; private bool toolTipActivated = false; public DataGridViewToolTip(DataGridView dataGridView) { this.dataGridView = dataGridView; } public bool Activated { get { return this.toolTipActivated; } } public ToolTip ToolTip { get { return this.toolTip; } } public void Activate(bool activate) { if (this.dataGridView.DesignMode) { return; } // Create the tool tip handle on demand. if (activate && this.toolTip == null) { this.toolTip = new ToolTip(); this.toolTip.ShowAlways = true; this.toolTip.InitialDelay = 0; this.toolTip.UseFading = false; this.toolTip.UseAnimation = false; this.toolTip.AutoPopDelay = 0; } if (this.dataGridView.IsRestricted) { IntSecurity.AllWindows.Assert(); } try { if (activate) { this.toolTip.Active = true; this.toolTip.Show(this.dataGridView.ToolTipPrivate, this.dataGridView); } else if (this.toolTip != null) { this.toolTip.Hide(this.dataGridView); this.toolTip.Active = false; } } finally { if (this.dataGridView.IsRestricted) { CodeAccessPermission.RevertAssert(); } } this.toolTipActivated = activate; } public void Dispose() { if (this.toolTip != null) { this.toolTip.Dispose(); this.toolTip = null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
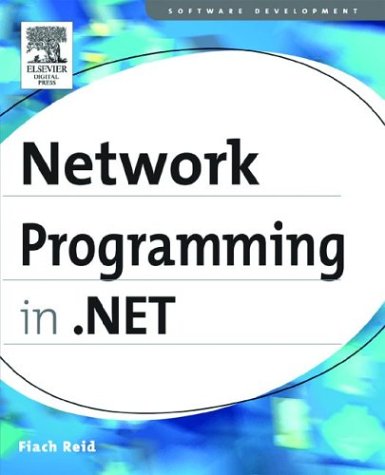
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GorillaCodec.cs
- WebContext.cs
- loginstatus.cs
- BamlReader.cs
- ModelServiceImpl.cs
- PathGradientBrush.cs
- AvTrace.cs
- BinaryMethodMessage.cs
- ToolStripOverflow.cs
- ListControlStringCollectionEditor.cs
- Cursor.cs
- StorageEntityContainerMapping.cs
- UpdateTranslator.cs
- EditingScope.cs
- cookiecollection.cs
- BinaryObjectReader.cs
- LinqDataSourceInsertEventArgs.cs
- VersionPair.cs
- ToolZone.cs
- QueryAsyncResult.cs
- VirtualizingStackPanel.cs
- safePerfProviderHandle.cs
- GridLength.cs
- RedirectionProxy.cs
- Symbol.cs
- TypeNameParser.cs
- StringKeyFrameCollection.cs
- GenericAuthenticationEventArgs.cs
- SqlTriggerAttribute.cs
- CodeMemberEvent.cs
- WindowsButton.cs
- XamlReader.cs
- ControlValuePropertyAttribute.cs
- OdbcConnectionString.cs
- QuaternionAnimation.cs
- WorkflowApplicationAbortedException.cs
- KnownBoxes.cs
- TrimSurroundingWhitespaceAttribute.cs
- DropShadowEffect.cs
- AsyncStreamReader.cs
- Rule.cs
- Span.cs
- TextTreeRootTextBlock.cs
- OutputCacheSettingsSection.cs
- RectKeyFrameCollection.cs
- XmlAttributes.cs
- XmlResolver.cs
- ObjectViewListener.cs
- RNGCryptoServiceProvider.cs
- XmlSerializableWriter.cs
- CalendarDateRange.cs
- SR.cs
- FlowDocument.cs
- OracleRowUpdatedEventArgs.cs
- WindowsAuthenticationModule.cs
- ParseChildrenAsPropertiesAttribute.cs
- CheckBox.cs
- DbDeleteCommandTree.cs
- ObjectViewEntityCollectionData.cs
- HtmlAnchor.cs
- DbSourceCommand.cs
- StackBuilderSink.cs
- StrokeCollection2.cs
- TypeRestriction.cs
- XamlTypeWithExplicitNamespace.cs
- BooleanSwitch.cs
- TimeManager.cs
- FixedLineResult.cs
- ValueHandle.cs
- ContractTypeNameCollection.cs
- DataGridViewCellStateChangedEventArgs.cs
- CompositeDataBoundControl.cs
- EntityUtil.cs
- AutomationPeer.cs
- CryptoApi.cs
- RIPEMD160.cs
- OracleCommand.cs
- ListItemParagraph.cs
- CodeTryCatchFinallyStatement.cs
- ImageCreator.cs
- WindowClosedEventArgs.cs
- ChannelBinding.cs
- ToolboxComponentsCreatedEventArgs.cs
- GridSplitterAutomationPeer.cs
- DataServiceQueryException.cs
- MachineKeySection.cs
- InternalConfigSettingsFactory.cs
- ObjectDataSource.cs
- WebPartUtil.cs
- BitmapCacheBrush.cs
- SocketInformation.cs
- DbDataSourceEnumerator.cs
- ZoneMembershipCondition.cs
- Mapping.cs
- ClientType.cs
- CancelEventArgs.cs
- ImagingCache.cs
- UndoUnit.cs
- AlternateViewCollection.cs
- CodeArgumentReferenceExpression.cs