Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Endpoint.cs / 1305376 / Endpoint.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel { using System; using System.Collections.ObjectModel; using System.ComponentModel; using System.Runtime; using System.ServiceModel.Activities; using System.ServiceModel.Channels; using System.ServiceModel.XamlIntegration; using System.Xml.Linq; using SR2 = System.ServiceModel.Activities.SR; public class Endpoint { Collectionheaders; [DefaultValue(null)] public string BehaviorConfigurationName { get; set; } [Fx.Tag.KnownXamlExternal] [DefaultValue(null)] public Binding Binding { get; set; } [DefaultValue(null)] [TypeConverter(typeof(ServiceXNameTypeConverter))] public XName ServiceContractName { get; set; } // concrete AddressHeader descendants aren't currently XAMLable, they are not initialized until runtime // If user adds an address header, this object will fail to xamlize. [Fx.Tag.KnownXamlExternal] public Collection Headers { get { if (this.headers == null) { this.headers = new Collection (); } return this.headers; } } [DefaultValue(null)] [TypeConverter(typeof(EndpointIdentityConverter))] public EndpointIdentity Identity { get; set; } [DefaultValue(null)] public Uri ListenUri { get; set; } [DefaultValue(null)] public string Name { get; set; } [DefaultValue(null)] public Uri AddressUri { get; set; } public EndpointAddress GetAddress() { return GetAddress(null); } public EndpointAddress GetAddress(ServiceHostBase host) { if (this.AddressUri == null) { string endpointName = ContractValidationHelper.GetErrorMessageEndpointName(this.Name); string contractName = ContractValidationHelper.GetErrorMessageEndpointServiceContractName(this.ServiceContractName); throw FxTrace.Exception.AsError(new InvalidOperationException( SR2.MissingUriInEndpoint(endpointName, contractName))); } Uri address = null; if (this.AddressUri.IsAbsoluteUri) { address = this.AddressUri; } else { if (this.Binding == null) { string endpointName = ContractValidationHelper.GetErrorMessageEndpointName(this.Name); string contractName = ContractValidationHelper.GetErrorMessageEndpointServiceContractName(this.ServiceContractName); throw FxTrace.Exception.AsError(new InvalidOperationException( SR2.RelativeUriRequiresBinding(endpointName, contractName, this.AddressUri))); } if (host == null) { string endpointName = ContractValidationHelper.GetErrorMessageEndpointName(this.Name); string contractName = ContractValidationHelper.GetErrorMessageEndpointServiceContractName(this.ServiceContractName); throw FxTrace.Exception.AsError(new InvalidOperationException( SR2.RelativeUriRequiresHost(endpointName, contractName, this.AddressUri))); } address = host.MakeAbsoluteUri(this.AddressUri, this.Binding); } return new EndpointAddress(address, this.Identity, new AddressHeaderCollection(this.Headers)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
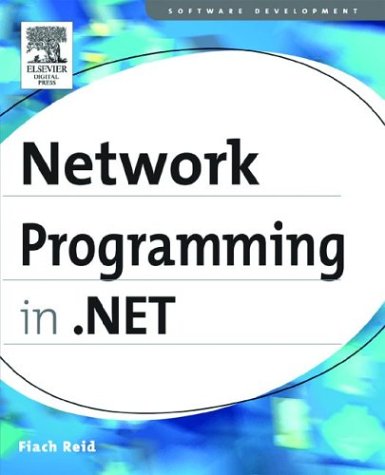
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WsatAdminException.cs
- SoapObjectInfo.cs
- exports.cs
- CatalogZoneBase.cs
- CompressEmulationStream.cs
- MediaSystem.cs
- WorkflowInstanceRecord.cs
- QilReplaceVisitor.cs
- DataException.cs
- SplitterPanel.cs
- DataGridViewCellStyleConverter.cs
- ShaperBuffers.cs
- initElementDictionary.cs
- RegularExpressionValidator.cs
- BinHexEncoder.cs
- COM2Properties.cs
- GorillaCodec.cs
- CollectionViewGroupInternal.cs
- Quad.cs
- CharUnicodeInfo.cs
- InheritablePropertyChangeInfo.cs
- TypeUtils.cs
- CollectionChangedEventManager.cs
- PositiveTimeSpanValidatorAttribute.cs
- InProcStateClientManager.cs
- PropertyPath.cs
- DoubleAnimationClockResource.cs
- HScrollProperties.cs
- FileUtil.cs
- LoaderAllocator.cs
- OverflowException.cs
- DataGridViewEditingControlShowingEventArgs.cs
- MulticastNotSupportedException.cs
- PolyBezierSegment.cs
- Pointer.cs
- BridgeDataReader.cs
- KeyFrames.cs
- AutomationPropertyInfo.cs
- ContractComponent.cs
- GroupByQueryOperator.cs
- ClassHandlersStore.cs
- WebPartCatalogCloseVerb.cs
- GeometryHitTestResult.cs
- DataGridTableCollection.cs
- InlineCollection.cs
- ViewBase.cs
- PropertyGrid.cs
- WebServicesInteroperability.cs
- WeakReferenceList.cs
- BindingCompleteEventArgs.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- DbInsertCommandTree.cs
- SQLByte.cs
- DataTemplateKey.cs
- WmlSelectionListAdapter.cs
- UDPClient.cs
- XpsS0ValidatingLoader.cs
- TextTreeRootTextBlock.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- LocalFileSettingsProvider.cs
- FontInfo.cs
- __Error.cs
- SymbolPair.cs
- NavigationCommands.cs
- FloaterBaseParagraph.cs
- ListBoxItemAutomationPeer.cs
- TCPClient.cs
- ExtentKey.cs
- TextProperties.cs
- IpcServerChannel.cs
- ExpressionReplacer.cs
- Transform3DCollection.cs
- BehaviorDragDropEventArgs.cs
- EventMappingSettingsCollection.cs
- CodeIndexerExpression.cs
- SetStoryboardSpeedRatio.cs
- WebBrowserNavigatedEventHandler.cs
- AssemblyCacheEntry.cs
- ColorComboBox.cs
- PersonalizationProvider.cs
- EnumValAlphaComparer.cs
- Visual3D.cs
- RecommendedAsConfigurableAttribute.cs
- ActivatedMessageQueue.cs
- ToolStripContainer.cs
- BindingManagerDataErrorEventArgs.cs
- EventLog.cs
- PeerOutputChannel.cs
- Menu.cs
- ValueSerializerAttribute.cs
- VisualBasic.cs
- MultiSelectRootGridEntry.cs
- ProxyGenerationError.cs
- TextComposition.cs
- EventKeyword.cs
- CancellationHandler.cs
- Decorator.cs
- AdornerHitTestResult.cs
- TreeNodeConverter.cs
- TemplateBindingExtensionConverter.cs