Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities.DurableInstancing / System / Activities / DurableInstancing / SerializationUtilities.cs / 1305376 / SerializationUtilities.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.DurableInstancing { using System.Collections.Generic; using System.Globalization; using System.IO; using System.IO.Compression; using System.Runtime; using System.Runtime.DurableInstancing; using System.Runtime.Serialization; using System.Linq; using System.Xml.Linq; using System.Text; using System.Xml; using System.Xml.Serialization; static class SerializationUtilities { public static byte[] CreateKeyBinaryBlob(ListcorrelationKeys) { long memoryRequired = correlationKeys.Sum(i => i.BinaryData.Count); byte[] concatenatedBlob = null; if (memoryRequired > 0) { concatenatedBlob = new byte[memoryRequired]; long insertLocation = 0; foreach (CorrelationKey correlationKey in correlationKeys) { Buffer.BlockCopy(correlationKey.BinaryData.Array, 0, concatenatedBlob, Convert.ToInt32(insertLocation), Convert.ToInt32(correlationKey.BinaryData.Count)); correlationKey.StartPosition = insertLocation; insertLocation += correlationKey.BinaryData.Count; } } return concatenatedBlob; } public static object CreateCorrelationKeyXmlBlob(List correlationKeys) { if (correlationKeys == null || correlationKeys.Count == 0) { return DBNull.Value; } StringBuilder stringBuilder = new StringBuilder(SqlWorkflowInstanceStoreConstants.DefaultStringBuilderCapacity); using (XmlWriter xmlWriter = XmlWriter.Create(stringBuilder)) { xmlWriter.WriteStartElement("CorrelationKeys"); foreach (CorrelationKey correlationKey in correlationKeys) { correlationKey.SerializeToXmlElement(xmlWriter); } xmlWriter.WriteEndElement(); } return stringBuilder.ToString(); } public static bool IsPropertyTypeSqlVariantCompatible(InstanceValue value) { if ((value.IsDeletedValue) || (value.Value == null) || (value.Value is string && ((string)value.Value).Length <= 4000) || (value.Value is Guid) || (value.Value is DateTime) || (value.Value is int) || (value.Value is double) || (value.Value is float) || (value.Value is long) || (value.Value is short) || (value.Value is byte) || (value.Value is decimal && CanDecimalBeStoredAsSqlVariant((decimal)value.Value))) { return true; } else { return false; } } public static Dictionary DeserializeMetadataPropertyBag(byte[] serializedMetadataProperties, InstanceEncodingOption instanceEncodingOption) { Dictionary metadataProperties = new Dictionary (); if (serializedMetadataProperties != null) { IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(instanceEncodingOption); Dictionary propertyBag = serializer.DeserializePropertyBag(serializedMetadataProperties); foreach (KeyValuePair property in propertyBag) { metadataProperties.Add(property.Key, new InstanceValue(property.Value)); } } return metadataProperties; } public static ArraySegment SerializeMetadataPropertyBag(SaveWorkflowCommand saveWorkflowCommand, InstancePersistenceContext context, InstanceEncodingOption instanceEncodingOption) { IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(instanceEncodingOption); Dictionary propertyBagToSerialize = new Dictionary (); if (context.InstanceView.InstanceMetadataConsistency == InstanceValueConsistency.None) { foreach (KeyValuePair metadataProperty in context.InstanceView.InstanceMetadata) { if ((metadataProperty.Value.Options & InstanceValueOptions.WriteOnly) == 0) { propertyBagToSerialize.Add(metadataProperty.Key, metadataProperty.Value.Value); } } } foreach (KeyValuePair metadataChange in saveWorkflowCommand.InstanceMetadataChanges) { if (metadataChange.Value.IsDeletedValue) { if (context.InstanceView.InstanceMetadataConsistency == InstanceValueConsistency.None) { propertyBagToSerialize.Remove(metadataChange.Key); } else { propertyBagToSerialize[metadataChange.Key] = new DeletedMetadataValue(); } } else if ((metadataChange.Value.Options & InstanceValueOptions.WriteOnly) == 0) { propertyBagToSerialize[metadataChange.Key] = metadataChange.Value.Value; } } if (propertyBagToSerialize.Count > 0) { return serializer.SerializePropertyBag(propertyBagToSerialize); } return new ArraySegment (); } public static ArraySegment [] SerializePropertyBag(IDictionary properties, InstanceEncodingOption encodingOption) { ArraySegment [] dataArrays = new ArraySegment [4]; if (properties.Count > 0) { IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(encodingOption); XmlPropertyBag primitiveProperties = new XmlPropertyBag(); XmlPropertyBag primitiveWriteOnlyProperties = new XmlPropertyBag(); Dictionary complexProperties = new Dictionary (); Dictionary complexWriteOnlyProperties = new Dictionary (); Dictionary [] propertyBags = new Dictionary [] { primitiveProperties, complexProperties, primitiveWriteOnlyProperties, complexWriteOnlyProperties }; foreach (KeyValuePair property in properties) { bool isComplex = (XmlPropertyBag.GetPrimitiveType(property.Value.Value) == PrimitiveType.Unavailable); bool isWriteOnly = (property.Value.Options & InstanceValueOptions.WriteOnly) == InstanceValueOptions.WriteOnly; int index = (isWriteOnly ? 2 : 0) + (isComplex ? 1 : 0); propertyBags[index].Add(property.Key, property.Value.Value); } // Remove the properties that are already stored as individual columns from the serialized blob primitiveWriteOnlyProperties.Remove(SqlWorkflowInstanceStoreConstants.StatusPropertyName); primitiveWriteOnlyProperties.Remove(SqlWorkflowInstanceStoreConstants.LastUpdatePropertyName); primitiveWriteOnlyProperties.Remove(SqlWorkflowInstanceStoreConstants.PendingTimerExpirationPropertyName); complexWriteOnlyProperties.Remove(SqlWorkflowInstanceStoreConstants.BinaryBlockingBookmarksPropertyName); for (int i = 0; i < propertyBags.Length; i++) { if (propertyBags[i].Count > 0) { if (propertyBags[i] is XmlPropertyBag) { dataArrays[i] = serializer.SerializeValue(propertyBags[i]); } else { dataArrays[i] = serializer.SerializePropertyBag(propertyBags[i]); } } } } return dataArrays; } public static ArraySegment SerializeKeyMetadata(IDictionary metadataProperties, InstanceEncodingOption encodingOption) { if (metadataProperties != null && metadataProperties.Count > 0) { Dictionary propertyBag = new Dictionary (); foreach (KeyValuePair property in metadataProperties) { if ((property.Value.Options & InstanceValueOptions.WriteOnly) != InstanceValueOptions.WriteOnly) { propertyBag.Add(property.Key, property.Value.Value); } } IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(encodingOption); return serializer.SerializePropertyBag(propertyBag); } return new ArraySegment (); } public static Dictionary DeserializeKeyMetadata(byte[] serializedKeyMetadata, InstanceEncodingOption encodingOption) { return DeserializeMetadataPropertyBag(serializedKeyMetadata, encodingOption); } public static Dictionary DeserializePropertyBag(byte[] primitiveDataProperties, byte[] complexDataProperties, InstanceEncodingOption encodingOption) { IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(encodingOption); Dictionary properties = new Dictionary (); Dictionary [] propertyBags = new Dictionary [2]; if (primitiveDataProperties != null) { propertyBags[0] = (Dictionary )serializer.DeserializeValue(primitiveDataProperties); } if (complexDataProperties != null) { propertyBags[1] = serializer.DeserializePropertyBag(complexDataProperties); } foreach (Dictionary propertyBag in propertyBags) { if (propertyBag != null) { foreach (KeyValuePair property in propertyBag) { properties.Add(property.Key, new InstanceValue(property.Value)); } } } return properties; } static bool CanDecimalBeStoredAsSqlVariant(decimal value) { string decimalAsString = value.ToString("G", CultureInfo.InvariantCulture); return ((decimalAsString.Length - decimalAsString.IndexOf(".", StringComparison.Ordinal)) - 1 <= 18); } public static object DeserializeObject(byte[] serializedObject, InstanceEncodingOption encodingOption) { IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(encodingOption); return serializer.DeserializeValue(serializedObject); } public static ArraySegment SerializeObject(object objectToSerialize, InstanceEncodingOption encodingOption) { IObjectSerializer serializer = ObjectSerializerFactory.GetObjectSerializer(encodingOption); return serializer.SerializeValue(objectToSerialize); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
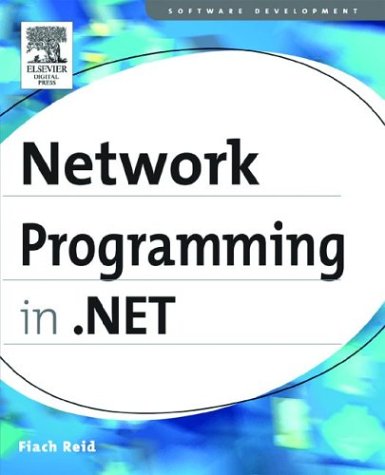
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NetworkStream.cs
- StylusShape.cs
- ReferencedType.cs
- Serializer.cs
- WebPartEditorApplyVerb.cs
- SignedInfo.cs
- AuthenticationException.cs
- PolyLineSegmentFigureLogic.cs
- ConfigurationPermission.cs
- DbConnectionPoolCounters.cs
- SiteMembershipCondition.cs
- GenericTypeParameterBuilder.cs
- SessionStateUtil.cs
- DesignerView.cs
- ReliableMessagingVersion.cs
- DSACryptoServiceProvider.cs
- LateBoundBitmapDecoder.cs
- DBDataPermission.cs
- HtmlControl.cs
- FrameworkObject.cs
- Shape.cs
- UserNameSecurityTokenAuthenticator.cs
- RuntimeWrappedException.cs
- MetadataItemEmitter.cs
- IdnMapping.cs
- XmlHierarchyData.cs
- TemplateLookupAction.cs
- BamlTreeUpdater.cs
- WeakHashtable.cs
- UserMapPath.cs
- DataDesignUtil.cs
- ProcessHostConfigUtils.cs
- mansign.cs
- TextFormatterImp.cs
- HandleCollector.cs
- PngBitmapEncoder.cs
- TimeoutValidationAttribute.cs
- ButtonChrome.cs
- StoreContentChangedEventArgs.cs
- WeakRefEnumerator.cs
- _AutoWebProxyScriptHelper.cs
- Error.cs
- Helper.cs
- BasicHttpSecurityMode.cs
- BufferedGraphics.cs
- CodeTypeOfExpression.cs
- SuppressMergeCheckAttribute.cs
- CapabilitiesSection.cs
- EdmType.cs
- ContainerControl.cs
- CollectionsUtil.cs
- StringConverter.cs
- odbcmetadatafactory.cs
- StringUtil.cs
- EntityKey.cs
- ContextStack.cs
- PointAnimationClockResource.cs
- AnimatedTypeHelpers.cs
- OLEDB_Util.cs
- AppSettingsSection.cs
- MultipartContentParser.cs
- RegistryPermission.cs
- TableRow.cs
- RegexCapture.cs
- XmlSchemaAttributeGroupRef.cs
- AsymmetricSignatureDeformatter.cs
- DataServiceQueryException.cs
- DbConnectionPoolOptions.cs
- EncryptedKey.cs
- DiscoveryDocumentLinksPattern.cs
- StringValueConverter.cs
- DataView.cs
- InstancePersistenceContext.cs
- SendContent.cs
- LinqDataSourceView.cs
- MailMessageEventArgs.cs
- CssTextWriter.cs
- XamlStream.cs
- UriSchemeKeyedCollection.cs
- InputScopeNameConverter.cs
- BuildProvider.cs
- EntityAdapter.cs
- ICspAsymmetricAlgorithm.cs
- SoapHeaderAttribute.cs
- GeneralTransform3D.cs
- MenuAdapter.cs
- ToolStripCustomTypeDescriptor.cs
- PopupEventArgs.cs
- SymLanguageVendor.cs
- UdpDiscoveryEndpoint.cs
- CodeCastExpression.cs
- Message.cs
- SqlProfileProvider.cs
- DnsPermission.cs
- WindowsHyperlink.cs
- CompilerState.cs
- ServerIdentity.cs
- DBSchemaTable.cs
- MenuRendererClassic.cs
- NotCondition.cs