Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Runtime / MappableObjectManager.cs / 1305376 / MappableObjectManager.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Runtime { using System.Activities.Hosting; using System.Collections.Generic; using System.Globalization; using System.Runtime; using System.Runtime.Serialization; using System.Xml.Linq; [DataContract] class MappableObjectManager { [DataMember(EmitDefaultValue = false)] ListmappableLocations; public MappableObjectManager() { } public int Count { get { int result = 0; if (this.mappableLocations != null) { result += this.mappableLocations.Count; } return result; } } public IDictionary GatherMappableVariables() { Dictionary result = null; if (this.mappableLocations != null && this.mappableLocations.Count > 0) { result = new Dictionary (this.mappableLocations.Count); for (int locationIndex = 0; locationIndex < this.mappableLocations.Count; locationIndex++) { MappableLocation mappableLocation = this.mappableLocations[locationIndex]; result.Add(mappableLocation.MappingKeyName, new LocationInfo(mappableLocation.Name, mappableLocation.OwnerDisplayName, mappableLocation.Location.Value)); } } return result; } public void Register(Location location, Activity activity, LocationReference locationOwner, ActivityInstance activityInstance) { Fx.Assert(location.CanBeMapped, "should only register mappable locations"); if (this.mappableLocations == null) { this.mappableLocations = new List (); } this.mappableLocations.Add(new MappableLocation(locationOwner, activity, activityInstance, location)); } public void Unregister(Location location) { Fx.Assert(location.CanBeMapped, "should only register mappable locations"); int mappedLocationsCount = this.mappableLocations.Count; for (int i = 0; i < mappedLocationsCount; i++) { if (object.ReferenceEquals(this.mappableLocations[i].Location, location)) { this.mappableLocations.RemoveAt(i); break; } } Fx.Assert(this.mappableLocations.Count == mappedLocationsCount - 1, "can only unregister locations that have been registered"); } [DataContract] class MappableLocation { public MappableLocation(LocationReference locationOwner, Activity activity, ActivityInstance activityInstance, Location location) { this.Name = locationOwner.Name; this.OwnerDisplayName = activity.DisplayName; this.Location = location; this.MappingKeyName = string.Format(CultureInfo.InvariantCulture, "activity.{0}-{1}_{2}", activity.Id, locationOwner.Id, activityInstance.Id); } [DataMember] internal string MappingKeyName { get; private set; } [DataMember] public string Name { get; private set; } [DataMember(EmitDefaultValue = false)] public string OwnerDisplayName { get; private set; } [DataMember] internal Location Location { get; private set; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
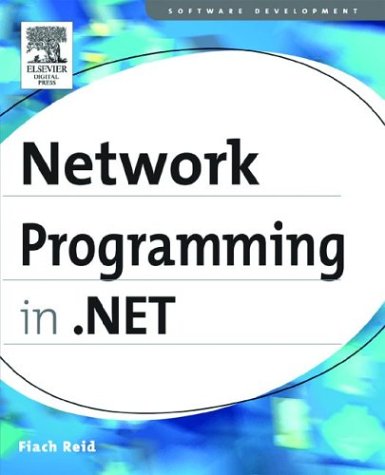
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NavigatingCancelEventArgs.cs
- AvTraceFormat.cs
- BypassElementCollection.cs
- TabletDeviceInfo.cs
- securitymgrsite.cs
- HttpContext.cs
- ZipIOLocalFileBlock.cs
- coordinatorscratchpad.cs
- XmlSchemaComplexContentExtension.cs
- GlyphingCache.cs
- DictionaryContent.cs
- WinHttpWebProxyFinder.cs
- GridViewColumnCollection.cs
- HGlobalSafeHandle.cs
- _HeaderInfoTable.cs
- HtmlGenericControl.cs
- StorageScalarPropertyMapping.cs
- ProtocolsConfigurationEntry.cs
- TemplateEditingFrame.cs
- ProfilePropertyNameValidator.cs
- MarkupObject.cs
- ObjectListField.cs
- ThreadSafeList.cs
- TraceSection.cs
- TextServicesCompartment.cs
- FontFamily.cs
- RegisteredExpandoAttribute.cs
- CombinedHttpChannel.cs
- BinaryUtilClasses.cs
- TimerEventSubscriptionCollection.cs
- ComplexType.cs
- EmptyStringExpandableObjectConverter.cs
- XPathItem.cs
- TypeUtil.cs
- CollectionMarkupSerializer.cs
- Command.cs
- datacache.cs
- XPathExpr.cs
- Parser.cs
- DbException.cs
- Buffer.cs
- CodeGenerationManager.cs
- ReferenceConverter.cs
- BevelBitmapEffect.cs
- XmlJsonWriter.cs
- ComPlusAuthorization.cs
- VisualStyleElement.cs
- AlternateView.cs
- MarkupCompiler.cs
- AutoResizedEvent.cs
- TreeView.cs
- CancelEventArgs.cs
- GridSplitter.cs
- ListItemDetailViewAttribute.cs
- ReadOnlyDictionary.cs
- SqlException.cs
- DataGridSortCommandEventArgs.cs
- RichTextBoxConstants.cs
- MenuItemCollection.cs
- SqlAliasesReferenced.cs
- PlanCompilerUtil.cs
- MarginsConverter.cs
- RuntimeHelpers.cs
- MediaScriptCommandRoutedEventArgs.cs
- FrameworkTemplate.cs
- WinInetCache.cs
- DtdParser.cs
- WmlValidatorAdapter.cs
- InterleavedZipPartStream.cs
- ReturnValue.cs
- ToolStripContainer.cs
- _AcceptOverlappedAsyncResult.cs
- LazyTextWriterCreator.cs
- HandlerBase.cs
- PeerTransportListenAddressValidatorAttribute.cs
- ButtonBaseDesigner.cs
- EntityDataSourceContainerNameConverter.cs
- ProcessModelSection.cs
- RemoteWebConfigurationHostServer.cs
- Error.cs
- SqlClientFactory.cs
- UIElementHelper.cs
- ReadWriteControlDesigner.cs
- ResourceContainer.cs
- JsonEncodingStreamWrapper.cs
- WebScriptServiceHost.cs
- IdentityValidationException.cs
- ProxyFragment.cs
- Model3DGroup.cs
- ExpressionEditorAttribute.cs
- LassoSelectionBehavior.cs
- Point3DCollectionConverter.cs
- ZoneLinkButton.cs
- Brush.cs
- XmlAttributeAttribute.cs
- WebReferencesBuildProvider.cs
- AlternateViewCollection.cs
- Messages.cs
- SqlDataSourceCommandEventArgs.cs
- TraceUtility.cs