Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / IndexerHelper.cs / 1305376 / IndexerHelper.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Expressions { using System.Activities.Validation; using System.Collections.Generic; using System.Linq.Expressions; using System.Runtime; using System.Collections.ObjectModel; using System.Reflection; static class IndexerHelper { public static void OnGetArguments(Collection indices, OutArgument > result, CodeActivityMetadata metadata) { for (int i = 0; i < indices.Count; i++) { RuntimeArgument indexArgument = new RuntimeArgument("Index" + i, indices[i].ArgumentType, ArgumentDirection.In, true); metadata.Bind(indices[i], indexArgument); metadata.AddArgument(indexArgument); } RuntimeArgument resultArgument = new RuntimeArgument("Result", typeof(Location ), ArgumentDirection.Out); metadata.Bind(result, resultArgument); metadata.AddArgument(resultArgument); } public static void CacheMethod (Collection indices, ref MethodInfo getMethod, ref MethodInfo setMethod) { Type[] getTypes = new Type[indices.Count]; for (int i = 0; i < indices.Count; i++) { getTypes[i] = indices[i].ArgumentType; } getMethod = typeof(TOperand).GetMethod("get_Item", getTypes); if (getMethod != null && !getMethod.IsSpecialName) { getMethod = null; } Type[] setTypes = new Type[indices.Count + 1]; for (int i = 0; i < indices.Count; i++) { setTypes[i] = indices[i].ArgumentType; } setTypes[setTypes.Length - 1] = typeof(TItem); setMethod = typeof(TOperand).GetMethod("set_Item", setTypes); if (setMethod != null && !setMethod.IsSpecialName) { setMethod = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
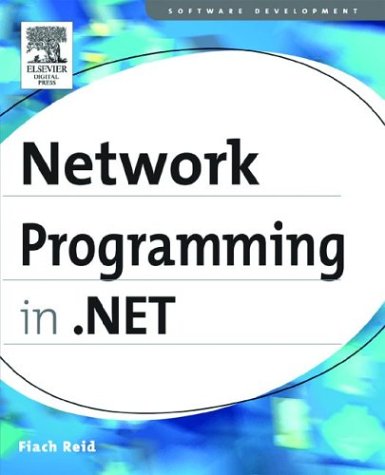
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- followingsibling.cs
- RowBinding.cs
- WindowsStartMenu.cs
- DefaultDiscoveryService.cs
- DbgCompiler.cs
- DynamicDataRouteHandler.cs
- DesignerActionPanel.cs
- KeyPressEvent.cs
- WsdlServiceChannelBuilder.cs
- Parsers.cs
- TextChangedEventArgs.cs
- DifferencingCollection.cs
- ObjectDataSourceChooseTypePanel.cs
- XmlIncludeAttribute.cs
- Pair.cs
- WebPartHeaderCloseVerb.cs
- DebugView.cs
- MutexSecurity.cs
- ErasingStroke.cs
- DiscoveryEndpointElement.cs
- InterleavedZipPartStream.cs
- WebPartEditorApplyVerb.cs
- ActivityTrace.cs
- XmlSchemaChoice.cs
- MobileUserControlDesigner.cs
- OdbcConnectionOpen.cs
- PageContentCollection.cs
- NoClickablePointException.cs
- IisTraceListener.cs
- XPathDocumentNavigator.cs
- xmlsaver.cs
- DataServiceClientException.cs
- TdsParserStaticMethods.cs
- Size3DValueSerializer.cs
- FileLoadException.cs
- ExpanderAutomationPeer.cs
- PrinterUnitConvert.cs
- SamlSecurityTokenAuthenticator.cs
- BufferAllocator.cs
- GraphicsPathIterator.cs
- SR.cs
- AssemblyUtil.cs
- BamlStream.cs
- IMembershipProvider.cs
- Rotation3D.cs
- FamilyMapCollection.cs
- SdlChannelSink.cs
- MetadataItem_Static.cs
- ZoneLinkButton.cs
- SqlBuffer.cs
- DataSourceCacheDurationConverter.cs
- Setter.cs
- CommandCollectionEditor.cs
- OptimizerPatterns.cs
- ImageConverter.cs
- DataTableCollection.cs
- CodeDirectoryCompiler.cs
- FrameworkName.cs
- SqlMultiplexer.cs
- CodeCommentStatementCollection.cs
- PrintPreviewGraphics.cs
- BinaryObjectWriter.cs
- XmlQueryTypeFactory.cs
- RSAProtectedConfigurationProvider.cs
- EndOfStreamException.cs
- CookieProtection.cs
- XPathNavigatorKeyComparer.cs
- SapiInterop.cs
- SslStream.cs
- QuaternionAnimation.cs
- MenuCommand.cs
- Bitmap.cs
- GlyphCache.cs
- AncillaryOps.cs
- XmlNamespaceManager.cs
- DocumentSequenceHighlightLayer.cs
- ValidationError.cs
- Int64.cs
- BlockExpression.cs
- WebSysDefaultValueAttribute.cs
- DataControlPagerLinkButton.cs
- TypeContext.cs
- WindowsProgressbar.cs
- LazyLoadBehavior.cs
- Error.cs
- DBParameter.cs
- TemplateControlCodeDomTreeGenerator.cs
- HostedAspNetEnvironment.cs
- IncrementalCompileAnalyzer.cs
- DataGridViewBand.cs
- ClrProviderManifest.cs
- PackageDigitalSignature.cs
- PreloadedPackages.cs
- ClientSideProviderDescription.cs
- COM2ExtendedTypeConverter.cs
- WsdlInspector.cs
- SafeReversePInvokeHandle.cs
- OleDbParameterCollection.cs
- MarkupExtensionSerializer.cs
- ResourcePart.cs