Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Discovery / System / ServiceModel / Discovery / DefaultDiscoveryService.cs / 1305376 / DefaultDiscoveryService.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Discovery { using System.Collections.ObjectModel; using System.Runtime; class DefaultDiscoveryService : DiscoveryService { readonly ReadOnlyCollectionpublishedEndpoints; public DefaultDiscoveryService( DiscoveryServiceExtension discoveryServiceExtension, DiscoveryMessageSequenceGenerator discoveryMessageSequenceGenerator, int duplicateMessageHistoryLength) : base(discoveryMessageSequenceGenerator, duplicateMessageHistoryLength) { Fx.Assert(discoveryServiceExtension != null, "The discoveryServiceExtension must be non null."); this.publishedEndpoints = discoveryServiceExtension.PublishedEndpoints; } protected override IAsyncResult OnBeginFind( FindRequestContext findRequestContext, AsyncCallback callback, object state) { this.Match(findRequestContext); return new CompletedAsyncResult(callback, state); } protected override void OnEndFind(IAsyncResult result) { CompletedAsyncResult.End(result); } protected override IAsyncResult OnBeginResolve(ResolveCriteria resolveCriteria, AsyncCallback callback, object state) { return new CompletedAsyncResult ( this.Match(resolveCriteria), callback, state); } protected override EndpointDiscoveryMetadata OnEndResolve(IAsyncResult result) { return CompletedAsyncResult .End(result); } EndpointDiscoveryMetadata Match(ResolveCriteria criteria) { for (int i = 0; i < this.publishedEndpoints.Count; i++) { if (this.publishedEndpoints[i].Address.Equals(criteria.Address)) { return this.publishedEndpoints[i]; } } return null; } void Match(FindRequestContext findRequestContext) { FindCriteria criteria = findRequestContext.Criteria; if (!ScopeCompiler.IsSupportedMatchingRule(criteria.ScopeMatchBy)) { return; } CompiledScopeCriteria[] compiledScopeCriterias = ScopeCompiler.CompileMatchCriteria( criteria.InternalScopes, criteria.ScopeMatchBy); int matchingEndpointCount = 0; for (int i = 0; i < this.publishedEndpoints.Count; i++) { if (criteria.IsMatch(this.publishedEndpoints[i], compiledScopeCriterias)) { findRequestContext.AddMatchingEndpoint(this.publishedEndpoints[i]); matchingEndpointCount++; if (matchingEndpointCount == criteria.MaxResults) { break; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
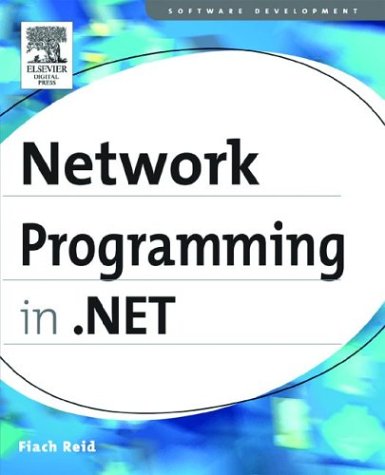
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- remotingproxy.cs
- BaseParaClient.cs
- DataGridViewCellStateChangedEventArgs.cs
- DocumentGrid.cs
- WebServiceAttribute.cs
- ParseNumbers.cs
- ActiveXContainer.cs
- SmtpReplyReaderFactory.cs
- FontFamilyConverter.cs
- XmlNodeComparer.cs
- SByteConverter.cs
- TrackingProfileCache.cs
- DragDrop.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- EventHandlers.cs
- XD.cs
- TlsSspiNegotiation.cs
- DataControlFieldCell.cs
- SqlDependency.cs
- MemberRestriction.cs
- PropertySet.cs
- ComplexTypeEmitter.cs
- Stylus.cs
- Pair.cs
- SlipBehavior.cs
- SerialPort.cs
- CacheMode.cs
- PartitionResolver.cs
- DmlSqlGenerator.cs
- WebBrowserBase.cs
- MetadataPropertyAttribute.cs
- VisualStyleInformation.cs
- ItemCollection.cs
- DelegatingHeader.cs
- DataBindingCollectionEditor.cs
- GifBitmapDecoder.cs
- ConstraintCollection.cs
- HtmlInputImage.cs
- ContextMenu.cs
- StaticResourceExtension.cs
- SoapWriter.cs
- PropertyItem.cs
- ClaimTypes.cs
- DBConcurrencyException.cs
- CalendarSelectionChangedEventArgs.cs
- ConsoleKeyInfo.cs
- HttpRequestCacheValidator.cs
- FileSystemEventArgs.cs
- WebControlsSection.cs
- Base64Decoder.cs
- NaturalLanguageHyphenator.cs
- OleDbConnection.cs
- DesignerAttribute.cs
- DataGrid.cs
- SqlOuterApplyReducer.cs
- DrawingServices.cs
- KnownTypesHelper.cs
- DataBoundLiteralControl.cs
- CollectionViewSource.cs
- PaintValueEventArgs.cs
- CultureInfoConverter.cs
- HttpProtocolReflector.cs
- MsmqQueue.cs
- KeyConverter.cs
- printdlgexmarshaler.cs
- VectorAnimationBase.cs
- ListBoxChrome.cs
- WebPartTransformerCollection.cs
- TemplatePartAttribute.cs
- MarshalByRefObject.cs
- SyntaxCheck.cs
- EntityKey.cs
- InputManager.cs
- SqlDataSourceView.cs
- HTTPNotFoundHandler.cs
- DESCryptoServiceProvider.cs
- ObjectFullSpanRewriter.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- IdentitySection.cs
- TreeViewBindingsEditor.cs
- XXXOnTypeBuilderInstantiation.cs
- FlagsAttribute.cs
- SqlTypeConverter.cs
- COM2IDispatchConverter.cs
- ObjectDataSource.cs
- PatternMatcher.cs
- LineServicesCallbacks.cs
- RadialGradientBrush.cs
- KeyPullup.cs
- Char.cs
- IItemProperties.cs
- PreservationFileReader.cs
- NativeMethods.cs
- CFStream.cs
- ObjectStateFormatter.cs
- TrackingMemoryStreamFactory.cs
- Stackframe.cs
- Vector3DAnimationBase.cs
- RubberbandSelector.cs
- ParserExtension.cs