Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / Microsoft / VisualBasic / Activities / VisualBasicValue.cs / 1305376 / VisualBasicValue.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.VisualBasic.Activities { using System; using System.Activities; using System.Activities.ExpressionParser; using System.Activities.XamlIntegration; using System.Linq.Expressions; using System.Windows.Markup; public sealed class VisualBasicValue: CodeActivity , IValueSerializableExpression, IExpressionContainer, IVisualBasicExpression { Expression > expressionTree; Func compiledExpression; public VisualBasicValue() : base() { this.SkipArgumentResolution = true; } public VisualBasicValue(string expressionText) : this() { this.ExpressionText = expressionText; } public string ExpressionText { get; set; } Expression IExpressionContainer.Expression { get { return this.expressionTree; } } protected override TResult Execute(CodeActivityContext context) { if (this.expressionTree != null) { return GetValueCore(context); } else { return default(TResult); } } internal override bool TryGetValue(ActivityContext context, out TResult value) { if (!this.SkipArgumentResolution && this.RuntimeArguments.Count > 1) { // We can't fast path because we have arguments other than the result // and we haven't obtained inlined references value = default(TResult); return false; } value = GetValueCore(context); return true; } TResult GetValueCore(ActivityContext context) { if (this.compiledExpression == null) { if (this.expressionTree == null) { return default(TResult); } this.compiledExpression = this.expressionTree.Compile(); } return this.compiledExpression(context); } protected override void CacheMetadata(CodeActivityMetadata metadata) { this.expressionTree = null; try { this.expressionTree = VisualBasicHelper.Compile (this.ExpressionText, metadata); } catch (SourceExpressionException e) { metadata.AddValidationError(e.Message); } } public bool CanConvertToString(IValueSerializerContext context) { // we can always convert to a string return true; } public string ConvertToString(IValueSerializerContext context) { // Return our bracket-escaped text return "[" + this.ExpressionText + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
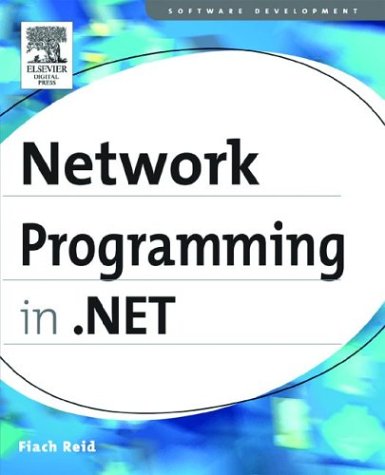
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostingEnvironment.cs
- ComboBoxHelper.cs
- EncoderNLS.cs
- PersonalizableTypeEntry.cs
- TextElementCollection.cs
- AtomEntry.cs
- DataServiceException.cs
- LookupNode.cs
- SignalGate.cs
- ProtectedConfigurationSection.cs
- Validator.cs
- XmlSerializerSection.cs
- PagesSection.cs
- CharAnimationUsingKeyFrames.cs
- ToolboxSnapDragDropEventArgs.cs
- HotSpot.cs
- TextContainerChangedEventArgs.cs
- SymbolPair.cs
- SQLStringStorage.cs
- StreamGeometry.cs
- XmlElementList.cs
- RulePatternOps.cs
- FixedPage.cs
- UncommonField.cs
- ProcessHost.cs
- DelimitedListTraceListener.cs
- TreeWalkHelper.cs
- DataGridLinkButton.cs
- LocalBuilder.cs
- CodeTypeConstructor.cs
- PersonalizationStateQuery.cs
- TextLineBreak.cs
- RowCache.cs
- OutputCacheSettingsSection.cs
- XPathPatternParser.cs
- SqlCacheDependencySection.cs
- OletxDependentTransaction.cs
- ModelPerspective.cs
- DynamicValueConverter.cs
- GetCardDetailsRequest.cs
- ToolTip.cs
- SrgsElementFactory.cs
- ElementAction.cs
- CodeCommentStatementCollection.cs
- precedingsibling.cs
- HostedImpersonationContext.cs
- CompiledQuery.cs
- GeometryGroup.cs
- PropertyEmitter.cs
- StrokeDescriptor.cs
- NetworkInformationPermission.cs
- HttpPostLocalhostServerProtocol.cs
- SafeRightsManagementPubHandle.cs
- InvokePattern.cs
- ColumnResult.cs
- PageRequestManager.cs
- AssemblyAssociatedContentFileAttribute.cs
- FontWeight.cs
- XsdValidatingReader.cs
- SendKeys.cs
- ToolStripArrowRenderEventArgs.cs
- IisTraceListener.cs
- ApplicationCommands.cs
- MatrixValueSerializer.cs
- WindowsPen.cs
- WindowsServiceCredential.cs
- ZipIOLocalFileDataDescriptor.cs
- EventsTab.cs
- CounterCreationDataConverter.cs
- ActiveXHost.cs
- CodePropertyReferenceExpression.cs
- XsltConvert.cs
- CodeObject.cs
- CodeSnippetTypeMember.cs
- MultiSelectRootGridEntry.cs
- MatrixIndependentAnimationStorage.cs
- xmlsaver.cs
- GlyphRunDrawing.cs
- ExtendLockCommand.cs
- EntityModelBuildProvider.cs
- XPathException.cs
- UpdatePanel.cs
- BrushMappingModeValidation.cs
- UnsafeNativeMethods.cs
- CapabilitiesAssignment.cs
- GenericXmlSecurityToken.cs
- TransportDefaults.cs
- GradientBrush.cs
- COAUTHINFO.cs
- MemoryStream.cs
- AtlasWeb.Designer.cs
- DefaultAuthorizationContext.cs
- BezierSegment.cs
- DataTableTypeConverter.cs
- SmiMetaData.cs
- DataGridSortCommandEventArgs.cs
- FilterableAttribute.cs
- ViewBase.cs
- FontDriver.cs
- HtmlValidationSummaryAdapter.cs