Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Dispatcher / DurableRuntimeValidator.cs / 1305376 / DurableRuntimeValidator.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System.ServiceModel.Description; using System.Workflow.Runtime; using System.Runtime; class DurableRuntimeValidator { ConcurrencyMode concurrencyMode; UnknownExceptionAction exceptionAction; bool saveStateInOperationTransaction; bool validated; public DurableRuntimeValidator(bool saveStateInOperationTransaction, UnknownExceptionAction exceptionAction) { this.saveStateInOperationTransaction = saveStateInOperationTransaction; this.exceptionAction = exceptionAction; this.validated = false; } public ConcurrencyMode ConcurrencyMode { get { if (!this.validated) { ValidateRuntime(); } return concurrencyMode; } } public void ValidateRuntime() { if (!this.validated) { Fx.Assert( OperationContext.Current != null && OperationContext.Current.EndpointDispatcher != null && OperationContext.Current.EndpointDispatcher.DispatchRuntime != null, "There shouldn't have been a null value in " + "OperationContext.Current.EndpointDispatcher.DispatchRuntime."); this.concurrencyMode = OperationContext.Current.EndpointDispatcher.DispatchRuntime.ConcurrencyMode; if (this.concurrencyMode == ConcurrencyMode.Multiple) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException( SR2.GetString(SR2.ConcurrencyMultipleNotSupported))); } if (this.saveStateInOperationTransaction && this.concurrencyMode != ConcurrencyMode.Single) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException( SR2.GetString(SR2.SaveStateInTransactionRequiresSingle))); } if (this.concurrencyMode == ConcurrencyMode.Reentrant && this.exceptionAction == UnknownExceptionAction.AbortInstance) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException( SR2.GetString(SR2.ConcurrencyReentrantAndAbortNotSupported))); } this.validated = true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
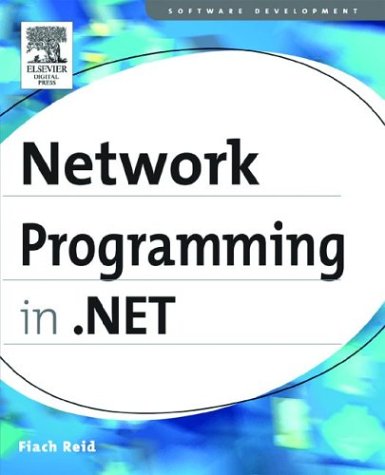
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Transform.cs
- SecurityResources.cs
- TdsParameterSetter.cs
- RsaSecurityTokenAuthenticator.cs
- CommandValueSerializer.cs
- FieldNameLookup.cs
- Assert.cs
- XmlSchemaExternal.cs
- SchemaImporterExtension.cs
- CreateUserWizard.cs
- GlyphRunDrawing.cs
- SelectingProviderEventArgs.cs
- BrowserCapabilitiesFactory.cs
- FormsAuthenticationModule.cs
- AutomationIdentifierGuids.cs
- HScrollBar.cs
- WindowsListViewItemStartMenu.cs
- DbSetClause.cs
- DateBoldEvent.cs
- ErrorLog.cs
- FileSystemWatcher.cs
- MenuItemStyle.cs
- UnconditionalPolicy.cs
- ComponentEvent.cs
- TextDecoration.cs
- InternalBufferManager.cs
- DbCommandTree.cs
- DataServiceBuildProvider.cs
- Section.cs
- AsymmetricSignatureFormatter.cs
- ThrowHelper.cs
- StateManagedCollection.cs
- MetadataException.cs
- FileUpload.cs
- FileAuthorizationModule.cs
- SettingsContext.cs
- ResolveMatchesMessageCD1.cs
- MaterialCollection.cs
- OdbcConnectionStringbuilder.cs
- Expression.cs
- DefaultParameterValueAttribute.cs
- UnmanagedBitmapWrapper.cs
- HeaderLabel.cs
- DesignerImageAdapter.cs
- ScrollViewer.cs
- WebControl.cs
- _UriTypeConverter.cs
- WebPartVerbCollection.cs
- Stackframe.cs
- RemoteWebConfigurationHostStream.cs
- LayoutInformation.cs
- MobilePage.cs
- InternalReceiveMessage.cs
- TracedNativeMethods.cs
- Style.cs
- CompilerParameters.cs
- FontDialog.cs
- HMACRIPEMD160.cs
- ApplicationGesture.cs
- PopOutPanel.cs
- ExpressionVisitor.cs
- TdsParserSessionPool.cs
- FreezableOperations.cs
- x509store.cs
- Transform.cs
- Helper.cs
- TextDecorationUnitValidation.cs
- SmtpSection.cs
- ByteArrayHelperWithString.cs
- App.cs
- GeneralTransform3DCollection.cs
- OleDbWrapper.cs
- log.cs
- ControlCachePolicy.cs
- Quaternion.cs
- CustomErrorsSectionWrapper.cs
- NameValueConfigurationCollection.cs
- HostUtils.cs
- NativeMethods.cs
- WSHttpBindingBase.cs
- TextTreeRootTextBlock.cs
- DataSourceConverter.cs
- LineServices.cs
- BaseWebProxyFinder.cs
- TraceEventCache.cs
- ArrayListCollectionBase.cs
- DesignRelationCollection.cs
- BlockUIContainer.cs
- XmlBindingWorker.cs
- SslStream.cs
- XmlWriterTraceListener.cs
- CollectionChangedEventManager.cs
- ConfigurationStrings.cs
- TextChange.cs
- ClientUrlResolverWrapper.cs
- XmlTypeMapping.cs
- NamespaceList.cs
- ArcSegment.cs
- PortCache.cs
- WebPartDescriptionCollection.cs