Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Description / DescriptionCreator.cs / 1305376 / DescriptionCreator.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Description { using System.Collections.Generic; using System.Workflow.ComponentModel; using System.Workflow.Runtime; class DescriptionCreator { WorkflowDefinitionContext workflowDefinitionContext; public DescriptionCreator(WorkflowDefinitionContext workflowDefinitionContext) { if (workflowDefinitionContext == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("workflowDefinitionContext"); } this.workflowDefinitionContext = workflowDefinitionContext; } public ServiceDescription BuildServiceDescription(out IDictionaryimplementedContracts, out IList reflectedContracts) { ServiceDescriptionContext context = new ServiceDescriptionContext(); ServiceDescription description = new ServiceDescription(); ApplyBehaviors(description); context.ServiceDescription = description; Walker walker = new Walker(true); walker.FoundActivity += delegate(Walker w, WalkerEventArgs args) { IServiceDescriptionBuilder activity = args.CurrentActivity as IServiceDescriptionBuilder; if (activity == null) { return; } activity.BuildServiceDescription(context); }; walker.Walk(this.workflowDefinitionContext.GetWorkflowDefinition()); if (context.Contracts == null || context.Contracts.Count == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.NoContract))); } implementedContracts = context.Contracts; reflectedContracts = context.ReflectedContracts; return description; } void ApplyBehaviors(ServiceDescription serviceDescription) { WorkflowServiceBehavior wsb = new WorkflowServiceBehavior(workflowDefinitionContext); serviceDescription.Behaviors.Add(wsb); if (wsb.Name != null) { serviceDescription.Name = wsb.Name; } if (wsb.Namespace != null) { serviceDescription.Namespace = wsb.Namespace; } if (wsb.ConfigurationName != null) { serviceDescription.ConfigurationName = wsb.ConfigurationName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
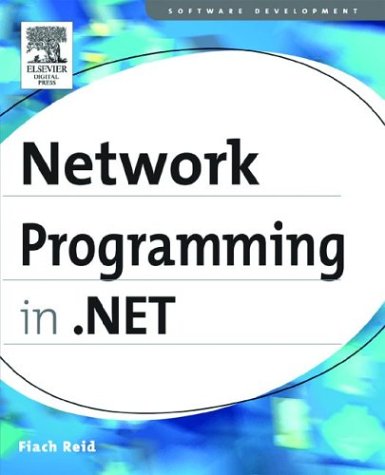
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImageUrlEditor.cs
- FixedPage.cs
- ActivationWorker.cs
- BinaryFormatterWriter.cs
- BitConverter.cs
- BufferModesCollection.cs
- IntegerValidator.cs
- FixedSOMGroup.cs
- XamlParser.cs
- AuthenticationModuleElementCollection.cs
- CounterSampleCalculator.cs
- SchemaImporterExtensionsSection.cs
- shaper.cs
- HTTPNotFoundHandler.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- TextBoxRenderer.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- SEHException.cs
- BitmapFrameDecode.cs
- ThrowHelper.cs
- AppDomain.cs
- DesignBindingEditor.cs
- IndexerNameAttribute.cs
- FixedDocument.cs
- NameNode.cs
- HashCodeCombiner.cs
- OdbcConnectionString.cs
- DataGridViewTopRowAccessibleObject.cs
- SystemWebExtensionsSectionGroup.cs
- QueryableDataSourceView.cs
- UriWriter.cs
- ListChangedEventArgs.cs
- DataViewSetting.cs
- CacheChildrenQuery.cs
- HostedTransportConfigurationBase.cs
- InfoCardProofToken.cs
- FileDialog_Vista_Interop.cs
- ComponentDispatcherThread.cs
- SafeIUnknown.cs
- StylusLogic.cs
- CompoundFileDeflateTransform.cs
- ListGeneralPage.cs
- DataBoundControlHelper.cs
- invalidudtexception.cs
- CompleteWizardStep.cs
- BmpBitmapEncoder.cs
- WebPartConnectionsDisconnectVerb.cs
- TemplateXamlParser.cs
- ContractListAdapter.cs
- MenuAdapter.cs
- PaginationProgressEventArgs.cs
- QueueProcessor.cs
- ThemeDirectoryCompiler.cs
- Duration.cs
- SemanticResultValue.cs
- ListControl.cs
- SafeNativeMethodsCLR.cs
- IsolatedStorageFilePermission.cs
- LiteralLink.cs
- TcpAppDomainProtocolHandler.cs
- FontClient.cs
- ActivityExecutionContextCollection.cs
- Compiler.cs
- IntellisenseTextBox.designer.cs
- ObjectQueryExecutionPlan.cs
- TextDecorationCollection.cs
- PingOptions.cs
- Vector3DCollectionConverter.cs
- XmlSchemaValidator.cs
- Vector3DConverter.cs
- ParserHooks.cs
- HttpApplication.cs
- MatrixValueSerializer.cs
- SharedPersonalizationStateInfo.cs
- _UriTypeConverter.cs
- NodeInfo.cs
- BindingMemberInfo.cs
- StylusPointDescription.cs
- StringTraceRecord.cs
- ChangeBlockUndoRecord.cs
- WebException.cs
- DateTimeUtil.cs
- BitmapEffect.cs
- LazyTextWriterCreator.cs
- TableAutomationPeer.cs
- WinFormsSecurity.cs
- ScriptingSectionGroup.cs
- AnnotationStore.cs
- XmlSchemaValidationException.cs
- DrawingContextWalker.cs
- PointHitTestParameters.cs
- DataGridViewImageColumn.cs
- DataBindingsDialog.cs
- TextDecorationCollection.cs
- EventSetter.cs
- DataGridPagingPage.cs
- Serializer.cs
- UnsupportedPolicyOptionsException.cs
- ImageList.cs
- CrossSiteScriptingValidation.cs