Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / StaticResourceExtension.cs / 1586720 / StaticResourceExtension.cs
/****************************************************************************\ * * File: StaticResourceExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Reflection; using MS.Internal; using System.Xaml; using System.Collections.Generic; namespace System.Windows { ////// Class for Xaml markup extension for static resource references. /// [MarkupExtensionReturnType(typeof(object))] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public class StaticResourceExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticResourceExtension() { } ////// Constructor that takes the resource key that this is a static reference to. /// public StaticResourceExtension( object resourceKey) { if (resourceKey == null) { throw new ArgumentNullException("resourceKey"); } _resourceKey = resourceKey; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For StaticResourceExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (ResourceKey is SystemResourceKey) { return (ResourceKey as SystemResourceKey).Resource; } return ProvideValueInternal(serviceProvider, false); } ////// The key in a Resource Dictionary used to find the object refered to by this /// Markup Extension. /// [ConstructorArgument("resourceKey")] public object ResourceKey { get { return _resourceKey; } set { if (value == null) { throw new ArgumentNullException("value"); } _resourceKey = value; } } ////// This value is used to resolved StaticResourceIds /// internal virtual DeferredResourceReference PrefetchedValue { get { return null; } } internal object ProvideValueInternal(IServiceProvider serviceProvider, bool allowDeferredReference) { object value = TryProvideValueInternal(serviceProvider, allowDeferredReference, false /* mustReturnDeferredResourceReference */); if (value == DependencyProperty.UnsetValue) { throw new Exception(SR.Get(SRID.ParserNoResource, ResourceKey.ToString())); } return value; } internal object TryProvideValueInternal(IServiceProvider serviceProvider, bool allowDeferredReference, bool mustReturnDeferredResourceReference) { // Get prefetchedValue DeferredResourceReference prefetchedValue = PrefetchedValue; object value; if (prefetchedValue == null) { // Do a normal look up. value = FindResourceInEnviroment(serviceProvider, allowDeferredReference, mustReturnDeferredResourceReference); } else { // If we have a Deferred Value, first check the current parse stack for a better (nearer) // value. This happens when this is a parse of deferred content and there is another // Resource Dictionary availible above this, yet still part of this deferred content. // This searches up to the outer most enclosing resource dictionary. value = FindResourceInDeferredContent(serviceProvider, allowDeferredReference, mustReturnDeferredResourceReference); // If we didn't find a new value in this part of deferred content // then use the existing prefetchedValue (DeferredResourceReference) if (value == DependencyProperty.UnsetValue) { value = allowDeferredReference ? prefetchedValue : prefetchedValue.GetValue(BaseValueSourceInternal.Unknown); } } return value; } private ResourceDictionary FindTheResourceDictionary(IServiceProvider serviceProvider, bool isDeferredContentSearch) { var schemaContextProvider = serviceProvider.GetService(typeof(IXamlSchemaContextProvider)) as IXamlSchemaContextProvider; if (schemaContextProvider == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IXamlSchemaContextProvider")); } var ambientProvider = serviceProvider.GetService(typeof(IAmbientProvider)) as IAmbientProvider; if (ambientProvider == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IAmbientProvider")); } XamlSchemaContext schemaContext = schemaContextProvider.SchemaContext; // This seems like a lot of work to do on every Provide Value // but that types and properties are cached in the schema. // XamlType feXType = schemaContext.GetXamlType(typeof(FrameworkElement)); XamlType styleXType = schemaContext.GetXamlType(typeof(Style)); XamlType templateXType = schemaContext.GetXamlType(typeof(FrameworkTemplate)); XamlType appXType = schemaContext.GetXamlType(typeof(Application)); XamlType fceXType = schemaContext.GetXamlType(typeof(FrameworkContentElement)); XamlMember fceResourcesProperty = fceXType.GetMember("Resources"); XamlMember feResourcesProperty = feXType.GetMember("Resources"); XamlMember styleResourcesProperty = styleXType.GetMember("Resources"); XamlMember styleBasedOnProperty = styleXType.GetMember("BasedOn"); XamlMember templateResourcesProperty = templateXType.GetMember("Resources"); XamlMember appResourcesProperty = appXType.GetMember("Resources"); XamlType[] types = new XamlType[1] { schemaContext.GetXamlType(typeof(ResourceDictionary)) }; IEnumerableambientValues = null; ambientValues = ambientProvider.GetAllAmbientValues(null, // ceilingTypes isDeferredContentSearch, types, fceResourcesProperty, feResourcesProperty, styleResourcesProperty, styleBasedOnProperty, templateResourcesProperty, appResourcesProperty); List ambientList; ambientList = ambientValues as List ; Debug.Assert(ambientList != null, "IAmbientProvider.GetAllAmbientValues no longer returns List<>, please copy the list"); for(int i=0; i /// Search just the App and Theme Resources. /// /// /// /// /// private object FindResourceInAppOrSystem(IServiceProvider serviceProvider, bool allowDeferredReference, bool mustReturnDeferredResourceReference) { object val; if (!SystemResources.IsSystemResourcesParsing) { object source; val = FrameworkElement.FindResourceFromAppOrSystem(ResourceKey, out source, false, // disableThrowOnResourceFailure allowDeferredReference, mustReturnDeferredResourceReference); } else { val = SystemResources.FindResourceInternal(ResourceKey, allowDeferredReference, mustReturnDeferredResourceReference); } return val; } private object FindResourceInEnviroment(IServiceProvider serviceProvider, bool allowDeferredReference, bool mustReturnDeferredResourceReference) { ResourceDictionary dictionaryWithKey = FindTheResourceDictionary(serviceProvider, /* isDeferredContentSearch */ false); if (dictionaryWithKey != null) { object value = dictionaryWithKey.Lookup(ResourceKey, allowDeferredReference, mustReturnDeferredResourceReference); return value; } // Look at app or themes object val = FindResourceInAppOrSystem(serviceProvider, allowDeferredReference, mustReturnDeferredResourceReference); if (val == null) { val = DependencyProperty.UnsetValue; } if (mustReturnDeferredResourceReference) { if (!(val is DeferredResourceReference)) { val = new DeferredResourceReferenceHolder(ResourceKey, val); } } return val; } private object _resourceKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: StaticResourceExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Reflection; using MS.Internal; using System.Xaml; using System.Collections.Generic; namespace System.Windows { /// /// Class for Xaml markup extension for static resource references. /// [MarkupExtensionReturnType(typeof(object))] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public class StaticResourceExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticResourceExtension() { } ////// Constructor that takes the resource key that this is a static reference to. /// public StaticResourceExtension( object resourceKey) { if (resourceKey == null) { throw new ArgumentNullException("resourceKey"); } _resourceKey = resourceKey; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For StaticResourceExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (ResourceKey is SystemResourceKey) { return (ResourceKey as SystemResourceKey).Resource; } return ProvideValueInternal(serviceProvider, false); } ////// The key in a Resource Dictionary used to find the object refered to by this /// Markup Extension. /// [ConstructorArgument("resourceKey")] public object ResourceKey { get { return _resourceKey; } set { if (value == null) { throw new ArgumentNullException("value"); } _resourceKey = value; } } ////// This value is used to resolved StaticResourceIds /// internal virtual DeferredResourceReference PrefetchedValue { get { return null; } } internal object ProvideValueInternal(IServiceProvider serviceProvider, bool allowDeferredReference) { object value = TryProvideValueInternal(serviceProvider, allowDeferredReference, false /* mustReturnDeferredResourceReference */); if (value == DependencyProperty.UnsetValue) { throw new Exception(SR.Get(SRID.ParserNoResource, ResourceKey.ToString())); } return value; } internal object TryProvideValueInternal(IServiceProvider serviceProvider, bool allowDeferredReference, bool mustReturnDeferredResourceReference) { // Get prefetchedValue DeferredResourceReference prefetchedValue = PrefetchedValue; object value; if (prefetchedValue == null) { // Do a normal look up. value = FindResourceInEnviroment(serviceProvider, allowDeferredReference, mustReturnDeferredResourceReference); } else { // If we have a Deferred Value, first check the current parse stack for a better (nearer) // value. This happens when this is a parse of deferred content and there is another // Resource Dictionary availible above this, yet still part of this deferred content. // This searches up to the outer most enclosing resource dictionary. value = FindResourceInDeferredContent(serviceProvider, allowDeferredReference, mustReturnDeferredResourceReference); // If we didn't find a new value in this part of deferred content // then use the existing prefetchedValue (DeferredResourceReference) if (value == DependencyProperty.UnsetValue) { value = allowDeferredReference ? prefetchedValue : prefetchedValue.GetValue(BaseValueSourceInternal.Unknown); } } return value; } private ResourceDictionary FindTheResourceDictionary(IServiceProvider serviceProvider, bool isDeferredContentSearch) { var schemaContextProvider = serviceProvider.GetService(typeof(IXamlSchemaContextProvider)) as IXamlSchemaContextProvider; if (schemaContextProvider == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IXamlSchemaContextProvider")); } var ambientProvider = serviceProvider.GetService(typeof(IAmbientProvider)) as IAmbientProvider; if (ambientProvider == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IAmbientProvider")); } XamlSchemaContext schemaContext = schemaContextProvider.SchemaContext; // This seems like a lot of work to do on every Provide Value // but that types and properties are cached in the schema. // XamlType feXType = schemaContext.GetXamlType(typeof(FrameworkElement)); XamlType styleXType = schemaContext.GetXamlType(typeof(Style)); XamlType templateXType = schemaContext.GetXamlType(typeof(FrameworkTemplate)); XamlType appXType = schemaContext.GetXamlType(typeof(Application)); XamlType fceXType = schemaContext.GetXamlType(typeof(FrameworkContentElement)); XamlMember fceResourcesProperty = fceXType.GetMember("Resources"); XamlMember feResourcesProperty = feXType.GetMember("Resources"); XamlMember styleResourcesProperty = styleXType.GetMember("Resources"); XamlMember styleBasedOnProperty = styleXType.GetMember("BasedOn"); XamlMember templateResourcesProperty = templateXType.GetMember("Resources"); XamlMember appResourcesProperty = appXType.GetMember("Resources"); XamlType[] types = new XamlType[1] { schemaContext.GetXamlType(typeof(ResourceDictionary)) }; IEnumerableambientValues = null; ambientValues = ambientProvider.GetAllAmbientValues(null, // ceilingTypes isDeferredContentSearch, types, fceResourcesProperty, feResourcesProperty, styleResourcesProperty, styleBasedOnProperty, templateResourcesProperty, appResourcesProperty); List ambientList; ambientList = ambientValues as List ; Debug.Assert(ambientList != null, "IAmbientProvider.GetAllAmbientValues no longer returns List<>, please copy the list"); for(int i=0; i /// Search just the App and Theme Resources. /// /// /// /// /// private object FindResourceInAppOrSystem(IServiceProvider serviceProvider, bool allowDeferredReference, bool mustReturnDeferredResourceReference) { object val; if (!SystemResources.IsSystemResourcesParsing) { object source; val = FrameworkElement.FindResourceFromAppOrSystem(ResourceKey, out source, false, // disableThrowOnResourceFailure allowDeferredReference, mustReturnDeferredResourceReference); } else { val = SystemResources.FindResourceInternal(ResourceKey, allowDeferredReference, mustReturnDeferredResourceReference); } return val; } private object FindResourceInEnviroment(IServiceProvider serviceProvider, bool allowDeferredReference, bool mustReturnDeferredResourceReference) { ResourceDictionary dictionaryWithKey = FindTheResourceDictionary(serviceProvider, /* isDeferredContentSearch */ false); if (dictionaryWithKey != null) { object value = dictionaryWithKey.Lookup(ResourceKey, allowDeferredReference, mustReturnDeferredResourceReference); return value; } // Look at app or themes object val = FindResourceInAppOrSystem(serviceProvider, allowDeferredReference, mustReturnDeferredResourceReference); if (val == null) { val = DependencyProperty.UnsetValue; } if (mustReturnDeferredResourceReference) { if (!(val is DeferredResourceReference)) { val = new DeferredResourceReferenceHolder(ResourceKey, val); } } return val; } private object _resourceKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
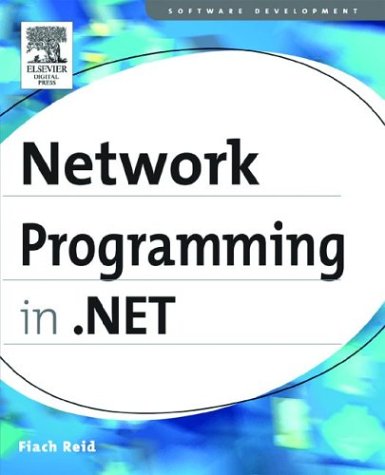
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CloseSequence.cs
- StandardOleMarshalObject.cs
- NotCondition.cs
- SqlUtil.cs
- DateTimeStorage.cs
- MetaModel.cs
- DataGridViewCellValueEventArgs.cs
- XamlSerializer.cs
- Keyboard.cs
- MaskedTextBoxDesignerActionList.cs
- CommandBindingCollection.cs
- ProfileModule.cs
- Duration.cs
- SpanIndex.cs
- PrePrepareMethodAttribute.cs
- DependencyPropertyAttribute.cs
- LiteralControl.cs
- SizeAnimationClockResource.cs
- PrinterSettings.cs
- DictionaryEntry.cs
- MetadataHelper.cs
- SafeRightsManagementPubHandle.cs
- AssemblyHash.cs
- PrintingPermission.cs
- VisualCollection.cs
- DataGridCell.cs
- PrinterResolution.cs
- AttributeCollection.cs
- SmiGettersStream.cs
- DataGridViewSelectedCellCollection.cs
- HostedTcpTransportManager.cs
- RegexGroup.cs
- SafeEventLogWriteHandle.cs
- Cursors.cs
- SqlNodeTypeOperators.cs
- SafeFileMappingHandle.cs
- Matrix3DConverter.cs
- BrowserInteropHelper.cs
- ObjectListItemCollection.cs
- SettingsPropertyValue.cs
- ToolStripItem.cs
- FileDialog_Vista.cs
- UnauthorizedWebPart.cs
- Material.cs
- BamlResourceSerializer.cs
- SamlAuthorizationDecisionClaimResource.cs
- MaterialCollection.cs
- BitmapFrame.cs
- NGCSerializer.cs
- CharAnimationUsingKeyFrames.cs
- CodeGen.cs
- ImplicitInputBrush.cs
- StatusBarPanel.cs
- HelpInfo.cs
- NavigationWindowAutomationPeer.cs
- EraserBehavior.cs
- SortKey.cs
- IdnElement.cs
- WindowsEditBoxRange.cs
- SourceSwitch.cs
- SecurityManager.cs
- ColorBlend.cs
- XmlSchemaInfo.cs
- OverlappedAsyncResult.cs
- TextTreeObjectNode.cs
- DataMemberAttribute.cs
- Certificate.cs
- CultureSpecificCharacterBufferRange.cs
- PanelStyle.cs
- XhtmlConformanceSection.cs
- StringAnimationUsingKeyFrames.cs
- CookielessHelper.cs
- TextChange.cs
- HttpsHostedTransportConfiguration.cs
- BindingRestrictions.cs
- DataConnectionHelper.cs
- UidPropertyAttribute.cs
- ReverseQueryOperator.cs
- CancellationScope.cs
- HWStack.cs
- ScriptHandlerFactory.cs
- DataSetMappper.cs
- FontWeights.cs
- SRDisplayNameAttribute.cs
- IPEndPoint.cs
- PlaceHolder.cs
- MimeParameter.cs
- GridErrorDlg.cs
- IEnumerable.cs
- EffectiveValueEntry.cs
- ExpandedProjectionNode.cs
- FastEncoder.cs
- NullRuntimeConfig.cs
- ConfigurationPropertyCollection.cs
- StaticFileHandler.cs
- UiaCoreApi.cs
- BamlLocalizer.cs
- SQLMoneyStorage.cs
- EntityDataSourceStatementEditorForm.cs
- ScriptModule.cs