Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Data / XmlNamespaceMappingCollection.cs / 1305600 / XmlNamespaceMappingCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMappingCollection object. // // Specs: http://avalon/connecteddata/M5%20Specs/XmlDataSource.mht // http://avalon/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.Collections.Generic; // ICollectionusing System.Xml; using System.Windows.Markup; using MS.Utility; namespace System.Windows.Data { /// /// XmlNamespaceMappingCollection Class /// Used to declare namespaces to be used in Xml data binding XPath queries /// [Localizability(LocalizationCategory.NeverLocalize)] public class XmlNamespaceMappingCollection : XmlNamespaceManager, ICollection, IAddChildInternal { /// /// Constructor /// public XmlNamespaceMappingCollection() : base(new NameTable()) {} #region IAddChild ////// IAddChild implementation /// /// void IAddChild.AddChild(object value) { AddChild(value); } ////// /// IAddChild implementation /// /// protected virtual void AddChild(object value) { XmlNamespaceMapping mapping = value as XmlNamespaceMapping; if (mapping == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMapping, value.GetType().FullName), "value"); Add(mapping); } ////// IAddChild implementation /// /// void IAddChild.AddText(string text) { AddText(text); } ////// IAddChild implementation /// /// protected virtual void AddText(string text) { if (text == null) throw new ArgumentNullException("text"); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion #region ICollection/// /// Add XmlNamespaceMapping /// ///mapping is null public void Add(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); // this.AddNamespace(mapping.Prefix, mapping.Uri.OriginalString); } ////// Remove all XmlNamespaceMappings /// ////// This is potentially an expensive operation. /// It may be cheaper to simply create a new XmlNamespaceMappingCollection. /// public void Clear() { int count = Count; XmlNamespaceMapping[] array = new XmlNamespaceMapping[count]; CopyTo(array, 0); for (int i = 0; i < count; ++i) { Remove(array[i]); } } ////// Add XmlNamespaceMapping /// ///mapping is null public bool Contains(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); return (this.LookupNamespace(mapping.Prefix) == mapping.Uri.OriginalString); } ////// Copy XmlNamespaceMappings to array /// public void CopyTo(XmlNamespaceMapping[] array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); int i = arrayIndex; int maxLength = array.Length; foreach (XmlNamespaceMapping mapping in this) { if (i >= maxLength) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); array[i] = mapping; ++ i; } } ////// Remove XmlNamespaceMapping /// ////// true if the mapping was removed /// ///mapping is null public bool Remove(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); if (Contains(mapping)) { this.RemoveNamespace(mapping.Prefix, mapping.Uri.OriginalString); return true; } return false; } ////// Count of the number of XmlNamespaceMappings /// public int Count { get { int count = 0; foreach (XmlNamespaceMapping mapping in this) { ++ count; } return count; } } ////// Value to indicate if this collection is read only /// public bool IsReadOnly { get { return false; } } ////// IEnumerable implementation. /// ////// This enables the serializer to serialize the contents of the XmlNamespaceMappingCollection. /// The default namespaces (xnm, xml, and string.Empty) are not included in this enumeration. /// public override IEnumerator GetEnumerator() { return ProtectedGetEnumerator(); } ////// IEnumerable (generic) implementation. /// IEnumeratorIEnumerable .GetEnumerator() { return ProtectedGetEnumerator(); } /// /// Protected member for use by IEnumerable implementations. /// protected IEnumeratorProtectedGetEnumerator() { IEnumerator enumerator = BaseEnumerator; while (enumerator.MoveNext()) { string prefix = (string) enumerator.Current; // ignore the default namespaces added automatically in the XmlNamespaceManager if (prefix == "xmlns" || prefix == "xml") continue; string ns = this.LookupNamespace(prefix); // ignore the empty prefix if the namespace has not been reassigned if ((prefix == string.Empty) && (ns == string.Empty)) continue; Uri uri = new Uri(ns, UriKind.RelativeOrAbsolute); XmlNamespaceMapping xnm = new XmlNamespaceMapping(prefix, uri); yield return xnm; } } // The iterator above cannot access base.GetEnumerator directly - this // causes build warning 1911, and makes MoveNext throw a security // exception under partial trust (bug 1785518). Accessing it indirectly // through this property fixes the problem. private IEnumerator BaseEnumerator { get { return base.GetEnumerator(); } } #endregion ICollection } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMappingCollection object. // // Specs: http://avalon/connecteddata/M5%20Specs/XmlDataSource.mht // http://avalon/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.Collections.Generic; // ICollectionusing System.Xml; using System.Windows.Markup; using MS.Utility; namespace System.Windows.Data { /// /// XmlNamespaceMappingCollection Class /// Used to declare namespaces to be used in Xml data binding XPath queries /// [Localizability(LocalizationCategory.NeverLocalize)] public class XmlNamespaceMappingCollection : XmlNamespaceManager, ICollection, IAddChildInternal { /// /// Constructor /// public XmlNamespaceMappingCollection() : base(new NameTable()) {} #region IAddChild ////// IAddChild implementation /// /// void IAddChild.AddChild(object value) { AddChild(value); } ////// /// IAddChild implementation /// /// protected virtual void AddChild(object value) { XmlNamespaceMapping mapping = value as XmlNamespaceMapping; if (mapping == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMapping, value.GetType().FullName), "value"); Add(mapping); } ////// IAddChild implementation /// /// void IAddChild.AddText(string text) { AddText(text); } ////// IAddChild implementation /// /// protected virtual void AddText(string text) { if (text == null) throw new ArgumentNullException("text"); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion #region ICollection/// /// Add XmlNamespaceMapping /// ///mapping is null public void Add(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); // this.AddNamespace(mapping.Prefix, mapping.Uri.OriginalString); } ////// Remove all XmlNamespaceMappings /// ////// This is potentially an expensive operation. /// It may be cheaper to simply create a new XmlNamespaceMappingCollection. /// public void Clear() { int count = Count; XmlNamespaceMapping[] array = new XmlNamespaceMapping[count]; CopyTo(array, 0); for (int i = 0; i < count; ++i) { Remove(array[i]); } } ////// Add XmlNamespaceMapping /// ///mapping is null public bool Contains(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); return (this.LookupNamespace(mapping.Prefix) == mapping.Uri.OriginalString); } ////// Copy XmlNamespaceMappings to array /// public void CopyTo(XmlNamespaceMapping[] array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); int i = arrayIndex; int maxLength = array.Length; foreach (XmlNamespaceMapping mapping in this) { if (i >= maxLength) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); array[i] = mapping; ++ i; } } ////// Remove XmlNamespaceMapping /// ////// true if the mapping was removed /// ///mapping is null public bool Remove(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); if (Contains(mapping)) { this.RemoveNamespace(mapping.Prefix, mapping.Uri.OriginalString); return true; } return false; } ////// Count of the number of XmlNamespaceMappings /// public int Count { get { int count = 0; foreach (XmlNamespaceMapping mapping in this) { ++ count; } return count; } } ////// Value to indicate if this collection is read only /// public bool IsReadOnly { get { return false; } } ////// IEnumerable implementation. /// ////// This enables the serializer to serialize the contents of the XmlNamespaceMappingCollection. /// The default namespaces (xnm, xml, and string.Empty) are not included in this enumeration. /// public override IEnumerator GetEnumerator() { return ProtectedGetEnumerator(); } ////// IEnumerable (generic) implementation. /// IEnumeratorIEnumerable .GetEnumerator() { return ProtectedGetEnumerator(); } /// /// Protected member for use by IEnumerable implementations. /// protected IEnumeratorProtectedGetEnumerator() { IEnumerator enumerator = BaseEnumerator; while (enumerator.MoveNext()) { string prefix = (string) enumerator.Current; // ignore the default namespaces added automatically in the XmlNamespaceManager if (prefix == "xmlns" || prefix == "xml") continue; string ns = this.LookupNamespace(prefix); // ignore the empty prefix if the namespace has not been reassigned if ((prefix == string.Empty) && (ns == string.Empty)) continue; Uri uri = new Uri(ns, UriKind.RelativeOrAbsolute); XmlNamespaceMapping xnm = new XmlNamespaceMapping(prefix, uri); yield return xnm; } } // The iterator above cannot access base.GetEnumerator directly - this // causes build warning 1911, and makes MoveNext throw a security // exception under partial trust (bug 1785518). Accessing it indirectly // through this property fixes the problem. private IEnumerator BaseEnumerator { get { return base.GetEnumerator(); } } #endregion ICollection } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
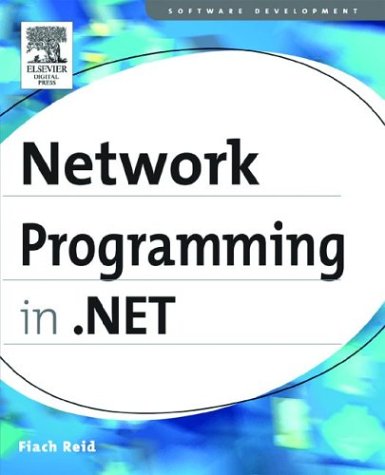
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WCFServiceClientProxyGenerator.cs
- AssociationSetMetadata.cs
- UriGenerator.cs
- TextEditorContextMenu.cs
- EntitySetDataBindingList.cs
- DataGridRowDetailsEventArgs.cs
- COM2PropertyDescriptor.cs
- ListViewDataItem.cs
- SimpleRecyclingCache.cs
- CustomPopupPlacement.cs
- LocatorBase.cs
- RsaSecurityToken.cs
- OracleFactory.cs
- WindowsRegion.cs
- SqlDataSource.cs
- AvTraceDetails.cs
- XmlSchemaSimpleContentRestriction.cs
- TextMetrics.cs
- basevalidator.cs
- ListBox.cs
- SoundPlayerAction.cs
- State.cs
- AccessorTable.cs
- PageContent.cs
- ArraySet.cs
- Aes.cs
- ScaleTransform3D.cs
- Condition.cs
- ScriptingProfileServiceSection.cs
- CompiledAction.cs
- XhtmlConformanceSection.cs
- SqlError.cs
- Error.cs
- CollaborationHelperFunctions.cs
- DataBindEngine.cs
- XmlNamespaceMapping.cs
- RepeaterItemEventArgs.cs
- X509KeyIdentifierClauseType.cs
- InternalException.cs
- Scanner.cs
- SmiEventSink.cs
- RadioButton.cs
- Label.cs
- ReachPageContentSerializer.cs
- EqualityComparer.cs
- ActivityTypeResolver.xaml.cs
- Latin1Encoding.cs
- BitSet.cs
- WorkerRequest.cs
- SolidColorBrush.cs
- WebServiceFault.cs
- InstanceNotReadyException.cs
- _CommandStream.cs
- XPathExpr.cs
- ComponentResourceManager.cs
- DriveNotFoundException.cs
- IisTraceListener.cs
- WebControl.cs
- FixedSOMPageConstructor.cs
- EntityProviderFactory.cs
- IisTraceWebEventProvider.cs
- FixedSOMTableCell.cs
- path.cs
- ManifestSignatureInformation.cs
- WebPartConnectionsEventArgs.cs
- TextProperties.cs
- ChannelServices.cs
- BitmapEffectRenderDataResource.cs
- Sentence.cs
- ProviderConnectionPointCollection.cs
- PerspectiveCamera.cs
- StandardCommands.cs
- ContextStaticAttribute.cs
- ReadOnlyDataSourceView.cs
- XmlNavigatorStack.cs
- RtType.cs
- EllipseGeometry.cs
- cache.cs
- DataReaderContainer.cs
- LeaseManager.cs
- CultureSpecificCharacterBufferRange.cs
- XmlSchemas.cs
- TempFiles.cs
- CalendarDataBindingHandler.cs
- FragmentQueryKB.cs
- PenLineCapValidation.cs
- FrameAutomationPeer.cs
- GeometryCollection.cs
- WpfWebRequestHelper.cs
- CodeIdentifiers.cs
- UnsafeNativeMethods.cs
- DataGridViewCheckBoxColumn.cs
- Point3D.cs
- ScriptingJsonSerializationSection.cs
- GPPOINTF.cs
- BaseInfoTable.cs
- IdnMapping.cs
- EpmAttributeNameBuilder.cs
- AppearanceEditorPart.cs
- DataRelationPropertyDescriptor.cs