Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Debugger / State.cs / 1305376 / State.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Debugger { using System; using System.Collections.Generic; using System.Diagnostics; using System.Reflection; using System.Runtime; using System.Diagnostics.CodeAnalysis; // Describes a "state" in the interpretter. A state is any source location that // a breakpoint could be set on or that could be stepped to. [DebuggerNonUserCode] [Fx.Tag.XamlVisible(false)] public class State { SourceLocation location; string name; IEnumerableearlyLocals; int numberOfEarlyLocals; // Calling Type.GetMethod() is slow (10,000 calls can take ~1 minute). // So we stash extra fields to be able to make the call lazily (as we Enter the state). // this.type.GetMethod Type type; string methodName; internal State(SourceLocation location, string name, IEnumerable earlyLocals, int numberOfEarlyLocals) { this.location = location; this.name = name; this.earlyLocals = earlyLocals; Fx.Assert(earlyLocals != null || numberOfEarlyLocals == 0, "If earlyLocals is null then numberOfEarlyLocals should be 0"); // Ignore the passed numberOfEarlyLocals if earlyLocal is null. this.numberOfEarlyLocals = (earlyLocals == null) ? 0 : numberOfEarlyLocals; } // Location in source file associated with this state. internal SourceLocation Location { get { return this.location; } } // Friendly name of the state. May be null if state is not named. // States need unique names. internal string Name { get { return this.name; } } // Type definitions for early bound locals. This list is ordered. // Names should be unique. internal IEnumerable EarlyLocals { get { return this.earlyLocals; } } internal int NumberOfEarlyLocals { get { return this.numberOfEarlyLocals; } } internal void CacheMethodInfo(Type type, string methodName) { this.type = type; this.methodName = methodName; } // Helper to lazily get the MethodInfo. This is expensive, so caller should cache it. internal MethodInfo GetMethodInfo(bool withPriming) { MethodInfo methodInfo = this.type.GetMethod(withPriming ? StateManager.MethodWithPrimingPrefix + this.methodName : this.methodName); return methodInfo; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
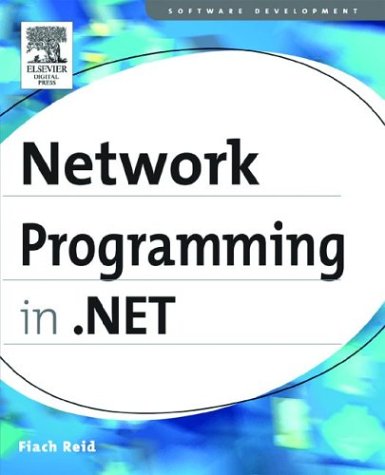
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadStateException.cs
- UIAgentAsyncEndRequest.cs
- FilterableAttribute.cs
- InkCanvasSelection.cs
- InvalidComObjectException.cs
- DataError.cs
- BaseTemplateBuildProvider.cs
- StyleCollection.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- DragEventArgs.cs
- XmlHierarchicalDataSourceView.cs
- BindToObject.cs
- CornerRadiusConverter.cs
- Selection.cs
- SliderAutomationPeer.cs
- PrimitiveOperationFormatter.cs
- PartManifestEntry.cs
- CalendarKeyboardHelper.cs
- LineServicesRun.cs
- RootProfilePropertySettingsCollection.cs
- control.ime.cs
- AnimationClockResource.cs
- MediaElement.cs
- OdbcConnectionPoolProviderInfo.cs
- ServerValidateEventArgs.cs
- AssociationEndMember.cs
- GPRECT.cs
- MailMessage.cs
- TextSelectionProcessor.cs
- EncodingTable.cs
- CheckBoxRenderer.cs
- ManagementQuery.cs
- FontWeights.cs
- OleDbConnection.cs
- Vector3DKeyFrameCollection.cs
- CheckBoxAutomationPeer.cs
- CustomError.cs
- CodeSnippetStatement.cs
- XmlConverter.cs
- COM2Properties.cs
- NamespaceCollection.cs
- IconConverter.cs
- RangeValuePattern.cs
- XmlUTF8TextReader.cs
- SettingsProviderCollection.cs
- SchemaComplexType.cs
- ResourceAttributes.cs
- ContainsSearchOperator.cs
- DrawToolTipEventArgs.cs
- DataGridViewAutoSizeModeEventArgs.cs
- SpellerStatusTable.cs
- GridViewColumnCollectionChangedEventArgs.cs
- ContractListAdapter.cs
- WebPartDisplayModeCollection.cs
- XmlEncodedRawTextWriter.cs
- OracleParameterBinding.cs
- DependencyPropertyAttribute.cs
- ParseHttpDate.cs
- XmlWhitespace.cs
- BindingMAnagerBase.cs
- Util.cs
- PageContent.cs
- HandleScope.cs
- HwndSource.cs
- DCSafeHandle.cs
- MailWebEventProvider.cs
- DataTable.cs
- FixUpCollection.cs
- EntityConnectionStringBuilder.cs
- TcpClientSocketManager.cs
- ChangeTracker.cs
- Image.cs
- Base64Stream.cs
- CursorInteropHelper.cs
- M3DUtil.cs
- InvalidBodyAccessException.cs
- BreakRecordTable.cs
- RenderDataDrawingContext.cs
- Int32CollectionConverter.cs
- xmlfixedPageInfo.cs
- Ref.cs
- StaticFileHandler.cs
- FrameworkElementFactory.cs
- NotificationContext.cs
- XamlSerializerUtil.cs
- TimeManager.cs
- SHA384.cs
- ChineseLunisolarCalendar.cs
- DrawListViewColumnHeaderEventArgs.cs
- DataChangedEventManager.cs
- SessionStateItemCollection.cs
- CachedTypeface.cs
- Perspective.cs
- InternalConfigHost.cs
- FileLoadException.cs
- WhiteSpaceTrimStringConverter.cs
- BuildDependencySet.cs
- SecurityNegotiationException.cs
- InvocationExpression.cs
- ElementHostPropertyMap.cs