Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / GridView.cs / 1305600 / GridView.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.ComponentModel; // DesignerSerializationVisibility using System.Windows.Automation.Peers; using System.Windows.Markup; using System.Diagnostics; // Debug using MS.Internal; // Helper using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ////// GridView is a built-in view of the ListView control. It is unique /// from other built-in views because of its table-like layout. Data in /// details view is shown in a table with each row corresponding to an /// entity in the data collection and each column being generated from a /// data-bound template, populated with values from the bound data entity. /// [StyleTypedProperty(Property = "ColumnHeaderContainerStyle", StyleTargetType = typeof(System.Windows.Controls.GridViewColumnHeader))] [ContentProperty("Columns")] public class GridView : ViewBase, IAddChild { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Add an object child to this control /// void IAddChild.AddChild(object column) { AddChild(column); } ////// Add an object child to this control /// protected virtual void AddChild(object column) { GridViewColumn c = column as GridViewColumn; if (c != null) { Columns.Add(c); } else { throw new InvalidOperationException(SR.Get(SRID.ListView_IllegalChildrenType)); } } ////// Add a text string to this control /// void IAddChild.AddText(string text) { AddText(text); } ////// Add a text string to this control /// protected virtual void AddText(string text) { AddChild(text); } ////// Returns a string representation of this object. /// ///public override string ToString() { return SR.Get(SRID.ToStringFormatString_GridView, this.GetType(), Columns.Count); } /// /// called when ListView creates its Automation peer /// GridView override this method to return a GridViewAutomationPeer /// /// listview reference ///GridView automation peer internal protected override IViewAutomationPeer GetAutomationPeer(ListView parent) { return new GridViewAutomationPeer(this, parent); } #endregion //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties // For all the DPs on GridView, null is treated as unset, // because it's impossible to distinguish null and unset. // Change a property between null and unset, PropertyChangedCallback will not be called. // --------------------------------------------------------------------------- // Defines the names of the resources to be consumed by the GridView style. // Used to restyle several roles of GridView without having to restyle // all of the control. // ---------------------------------------------------------------------------- #region StyleKeys ////// Key used to mark the template of ScrollViewer for use by GridView /// public static ResourceKey GridViewScrollViewerStyleKey { get { return SystemResourceKey.GridViewScrollViewerStyleKey; } } ////// Key used to mark the Style of GridView /// public static ResourceKey GridViewStyleKey { get { return SystemResourceKey.GridViewStyleKey; } } ////// Key used to mark the Style of ItemContainer /// public static ResourceKey GridViewItemContainerStyleKey { get { return SystemResourceKey.GridViewItemContainerStyleKey; } } #endregion StyleKeys #region GridViewColumnCollection Attached DP ////// Reads the attached property GridViewColumnCollection from the given element. /// /// The element from which to read the GridViewColumnCollection attached property. ///The property's value. public static GridViewColumnCollection GetColumnCollection(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (GridViewColumnCollection)element.GetValue(ColumnCollectionProperty); } ////// Writes the attached property GridViewColumnCollection to the given element. /// /// The element to which to write the GridViewColumnCollection attached property. /// The collection to set public static void SetColumnCollection(DependencyObject element, GridViewColumnCollection collection) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ColumnCollectionProperty, collection); } ////// This is the dependency property registered for the GridView' ColumnCollection attached property. /// public static readonly DependencyProperty ColumnCollectionProperty = DependencyProperty.RegisterAttached( "ColumnCollection", typeof(GridViewColumnCollection), typeof(GridView)); ////// Whether should serialize ColumnCollection attach DP /// /// Object on which this DP is set [EditorBrowsable(EditorBrowsableState.Never)] public static bool ShouldSerializeColumnCollection(DependencyObject obj) { ListViewItem listViewItem = obj as ListViewItem; if (listViewItem != null) { ListView listView = listViewItem.ParentSelector as ListView; if (listView != null) { GridView gridView = listView.View as GridView; if (gridView != null) { // if GridViewColumnCollection attached on ListViewItem is Details.Columns, it should't be serialized. GridViewColumnCollection localValue = listViewItem.ReadLocalValue(ColumnCollectionProperty) as GridViewColumnCollection; return (localValue != gridView.Columns); } } } return true; } #endregion ///GridViewColumn List [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public GridViewColumnCollection Columns { get { if (_columns == null) { _columns = new GridViewColumnCollection(); // Give the collection a back-link, this is used for the inheritance context _columns.Owner = this; _columns.InViewMode = true; } return _columns; } } #region ColumnHeaderContainerStyle ////// ColumnHeaderContainerStyleProperty DependencyProperty /// public static readonly DependencyProperty ColumnHeaderContainerStyleProperty = DependencyProperty.Register( "ColumnHeaderContainerStyle", typeof(Style), typeof(GridView) ); ////// header container's style /// public Style ColumnHeaderContainerStyle { get { return (Style)GetValue(ColumnHeaderContainerStyleProperty); } set { SetValue(ColumnHeaderContainerStyleProperty, value); } } #endregion // ColumnHeaderContainerStyle #region ColumnHeaderTemplate ////// ColumnHeaderTemplate DependencyProperty /// public static readonly DependencyProperty ColumnHeaderTemplateProperty = DependencyProperty.Register( "ColumnHeaderTemplate", typeof(DataTemplate), typeof(GridView), new FrameworkPropertyMetadata( new PropertyChangedCallback(OnColumnHeaderTemplateChanged)) ); ////// column header template /// public DataTemplate ColumnHeaderTemplate { get { return (DataTemplate)GetValue(ColumnHeaderTemplateProperty); } set { SetValue(ColumnHeaderTemplateProperty, value); } } private static void OnColumnHeaderTemplateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GridView dv = (GridView)d; // Check to prevent Template and TemplateSelector at the same time Helper.CheckTemplateAndTemplateSelector("GridViewColumnHeader", ColumnHeaderTemplateProperty, ColumnHeaderTemplateSelectorProperty, dv); } #endregion ColumnHeaderTemplate #region ColumnHeaderTemplateSelector ////// ColumnHeaderTemplateSelector DependencyProperty /// public static readonly DependencyProperty ColumnHeaderTemplateSelectorProperty = DependencyProperty.Register( "ColumnHeaderTemplateSelector", typeof(DataTemplateSelector), typeof(GridView), new FrameworkPropertyMetadata( new PropertyChangedCallback(OnColumnHeaderTemplateSelectorChanged)) ); ////// header template selector /// ////// This property is ignored if [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public DataTemplateSelector ColumnHeaderTemplateSelector { get { return (DataTemplateSelector)GetValue(ColumnHeaderTemplateSelectorProperty); } set { SetValue(ColumnHeaderTemplateSelectorProperty, value); } } private static void OnColumnHeaderTemplateSelectorChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GridView dv = (GridView)d; // Check to prevent Template and TemplateSelector at the same time Helper.CheckTemplateAndTemplateSelector("GridViewColumnHeader", ColumnHeaderTemplateProperty, ColumnHeaderTemplateSelectorProperty, dv); } #endregion ColumnHeaderTemplateSelector #region ColumnHeaderStringFormat ///is set. /// /// ColumnHeaderStringFormat DependencyProperty /// public static readonly DependencyProperty ColumnHeaderStringFormatProperty = DependencyProperty.Register( "ColumnHeaderStringFormat", typeof(String), typeof(GridView) ); ////// column header string format /// public String ColumnHeaderStringFormat { get { return (String)GetValue(ColumnHeaderStringFormatProperty); } set { SetValue(ColumnHeaderStringFormatProperty, value); } } #endregion ColumnHeaderStringFormat #region AllowsColumnReorder ////// AllowsColumnReorderProperty DependencyProperty /// public static readonly DependencyProperty AllowsColumnReorderProperty = DependencyProperty.Register( "AllowsColumnReorder", typeof(bool), typeof(GridView), new FrameworkPropertyMetadata(BooleanBoxes.TrueBox /* default value */ ) ); ////// AllowsColumnReorder /// public bool AllowsColumnReorder { get { return (bool)GetValue(AllowsColumnReorderProperty); } set { SetValue(AllowsColumnReorderProperty, value); } } #endregion AllowsColumnReorder #region ColumnHeaderContextMenu ////// ColumnHeaderContextMenuProperty DependencyProperty /// public static readonly DependencyProperty ColumnHeaderContextMenuProperty = DependencyProperty.Register( "ColumnHeaderContextMenu", typeof(ContextMenu), typeof(GridView) ); ////// ColumnHeaderContextMenu /// public ContextMenu ColumnHeaderContextMenu { get { return (ContextMenu)GetValue(ColumnHeaderContextMenuProperty); } set { SetValue(ColumnHeaderContextMenuProperty, value); } } #endregion ColumnHeaderContextMenu #region ColumnHeaderToolTip ////// ColumnHeaderToolTipProperty DependencyProperty /// public static readonly DependencyProperty ColumnHeaderToolTipProperty = DependencyProperty.Register( "ColumnHeaderToolTip", typeof(object), typeof(GridView) ); ////// ColumnHeaderToolTip /// public object ColumnHeaderToolTip { get { return GetValue(ColumnHeaderToolTipProperty); } set { SetValue(ColumnHeaderToolTipProperty, value); } } #endregion ColumnHeaderToolTip #endregion // Public Properties //------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods ////// called when ListView is prepare container for item. /// GridView override this method to attache the column collection /// /// the container protected internal override void PrepareItem(ListViewItem item) { base.PrepareItem(item); // attach GridViewColumnCollection to ListViewItem. SetColumnCollection(item, _columns); } ////// called when ListView is clear container for item. /// GridView override this method to clear the column collection /// /// the container protected internal override void ClearItem(ListViewItem item) { item.ClearValue(ColumnCollectionProperty); base.ClearItem(item); } #endregion //------------------------------------------------------------------- // // Protected Properties // //-------------------------------------------------------------------- #region Protected Properties ////// override with style key of GridView. /// protected internal override object DefaultStyleKey { get { return GridViewStyleKey; } } ////// override with style key using GridViewRowPresenter. /// protected internal override object ItemContainerDefaultStyleKey { get { return GridViewItemContainerStyleKey; } } #endregion //------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal override void OnInheritanceContextChangedCore(EventArgs args) { base.OnInheritanceContextChangedCore(args); if (_columns != null) { foreach (GridViewColumn column in _columns) { column.OnInheritanceContextChanged(args); } } } // Propagate theme changes to contained headers internal override void OnThemeChanged() { if (_columns != null) { for (int i=0; i<_columns.Count; i++) { _columns[i].OnThemeChanged(); } } } #endregion //-------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields private GridViewColumnCollection _columns; #endregion // Private Fields internal GridViewHeaderRowPresenter HeaderRowPresenter { get { return _gvheaderRP; } set { _gvheaderRP = value; } } private GridViewHeaderRowPresenter _gvheaderRP; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.ComponentModel; // DesignerSerializationVisibility using System.Windows.Automation.Peers; using System.Windows.Markup; using System.Diagnostics; // Debug using MS.Internal; // Helper using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ////// GridView is a built-in view of the ListView control. It is unique /// from other built-in views because of its table-like layout. Data in /// details view is shown in a table with each row corresponding to an /// entity in the data collection and each column being generated from a /// data-bound template, populated with values from the bound data entity. /// [StyleTypedProperty(Property = "ColumnHeaderContainerStyle", StyleTargetType = typeof(System.Windows.Controls.GridViewColumnHeader))] [ContentProperty("Columns")] public class GridView : ViewBase, IAddChild { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Add an object child to this control /// void IAddChild.AddChild(object column) { AddChild(column); } ////// Add an object child to this control /// protected virtual void AddChild(object column) { GridViewColumn c = column as GridViewColumn; if (c != null) { Columns.Add(c); } else { throw new InvalidOperationException(SR.Get(SRID.ListView_IllegalChildrenType)); } } ////// Add a text string to this control /// void IAddChild.AddText(string text) { AddText(text); } ////// Add a text string to this control /// protected virtual void AddText(string text) { AddChild(text); } ////// Returns a string representation of this object. /// ///public override string ToString() { return SR.Get(SRID.ToStringFormatString_GridView, this.GetType(), Columns.Count); } /// /// called when ListView creates its Automation peer /// GridView override this method to return a GridViewAutomationPeer /// /// listview reference ///GridView automation peer internal protected override IViewAutomationPeer GetAutomationPeer(ListView parent) { return new GridViewAutomationPeer(this, parent); } #endregion //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties // For all the DPs on GridView, null is treated as unset, // because it's impossible to distinguish null and unset. // Change a property between null and unset, PropertyChangedCallback will not be called. // --------------------------------------------------------------------------- // Defines the names of the resources to be consumed by the GridView style. // Used to restyle several roles of GridView without having to restyle // all of the control. // ---------------------------------------------------------------------------- #region StyleKeys ////// Key used to mark the template of ScrollViewer for use by GridView /// public static ResourceKey GridViewScrollViewerStyleKey { get { return SystemResourceKey.GridViewScrollViewerStyleKey; } } ////// Key used to mark the Style of GridView /// public static ResourceKey GridViewStyleKey { get { return SystemResourceKey.GridViewStyleKey; } } ////// Key used to mark the Style of ItemContainer /// public static ResourceKey GridViewItemContainerStyleKey { get { return SystemResourceKey.GridViewItemContainerStyleKey; } } #endregion StyleKeys #region GridViewColumnCollection Attached DP ////// Reads the attached property GridViewColumnCollection from the given element. /// /// The element from which to read the GridViewColumnCollection attached property. ///The property's value. public static GridViewColumnCollection GetColumnCollection(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (GridViewColumnCollection)element.GetValue(ColumnCollectionProperty); } ////// Writes the attached property GridViewColumnCollection to the given element. /// /// The element to which to write the GridViewColumnCollection attached property. /// The collection to set public static void SetColumnCollection(DependencyObject element, GridViewColumnCollection collection) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ColumnCollectionProperty, collection); } ////// This is the dependency property registered for the GridView' ColumnCollection attached property. /// public static readonly DependencyProperty ColumnCollectionProperty = DependencyProperty.RegisterAttached( "ColumnCollection", typeof(GridViewColumnCollection), typeof(GridView)); ////// Whether should serialize ColumnCollection attach DP /// /// Object on which this DP is set [EditorBrowsable(EditorBrowsableState.Never)] public static bool ShouldSerializeColumnCollection(DependencyObject obj) { ListViewItem listViewItem = obj as ListViewItem; if (listViewItem != null) { ListView listView = listViewItem.ParentSelector as ListView; if (listView != null) { GridView gridView = listView.View as GridView; if (gridView != null) { // if GridViewColumnCollection attached on ListViewItem is Details.Columns, it should't be serialized. GridViewColumnCollection localValue = listViewItem.ReadLocalValue(ColumnCollectionProperty) as GridViewColumnCollection; return (localValue != gridView.Columns); } } } return true; } #endregion ///GridViewColumn List [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public GridViewColumnCollection Columns { get { if (_columns == null) { _columns = new GridViewColumnCollection(); // Give the collection a back-link, this is used for the inheritance context _columns.Owner = this; _columns.InViewMode = true; } return _columns; } } #region ColumnHeaderContainerStyle ////// ColumnHeaderContainerStyleProperty DependencyProperty /// public static readonly DependencyProperty ColumnHeaderContainerStyleProperty = DependencyProperty.Register( "ColumnHeaderContainerStyle", typeof(Style), typeof(GridView) ); ////// header container's style /// public Style ColumnHeaderContainerStyle { get { return (Style)GetValue(ColumnHeaderContainerStyleProperty); } set { SetValue(ColumnHeaderContainerStyleProperty, value); } } #endregion // ColumnHeaderContainerStyle #region ColumnHeaderTemplate ////// ColumnHeaderTemplate DependencyProperty /// public static readonly DependencyProperty ColumnHeaderTemplateProperty = DependencyProperty.Register( "ColumnHeaderTemplate", typeof(DataTemplate), typeof(GridView), new FrameworkPropertyMetadata( new PropertyChangedCallback(OnColumnHeaderTemplateChanged)) ); ////// column header template /// public DataTemplate ColumnHeaderTemplate { get { return (DataTemplate)GetValue(ColumnHeaderTemplateProperty); } set { SetValue(ColumnHeaderTemplateProperty, value); } } private static void OnColumnHeaderTemplateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GridView dv = (GridView)d; // Check to prevent Template and TemplateSelector at the same time Helper.CheckTemplateAndTemplateSelector("GridViewColumnHeader", ColumnHeaderTemplateProperty, ColumnHeaderTemplateSelectorProperty, dv); } #endregion ColumnHeaderTemplate #region ColumnHeaderTemplateSelector ////// ColumnHeaderTemplateSelector DependencyProperty /// public static readonly DependencyProperty ColumnHeaderTemplateSelectorProperty = DependencyProperty.Register( "ColumnHeaderTemplateSelector", typeof(DataTemplateSelector), typeof(GridView), new FrameworkPropertyMetadata( new PropertyChangedCallback(OnColumnHeaderTemplateSelectorChanged)) ); ////// header template selector /// ////// This property is ignored if [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public DataTemplateSelector ColumnHeaderTemplateSelector { get { return (DataTemplateSelector)GetValue(ColumnHeaderTemplateSelectorProperty); } set { SetValue(ColumnHeaderTemplateSelectorProperty, value); } } private static void OnColumnHeaderTemplateSelectorChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GridView dv = (GridView)d; // Check to prevent Template and TemplateSelector at the same time Helper.CheckTemplateAndTemplateSelector("GridViewColumnHeader", ColumnHeaderTemplateProperty, ColumnHeaderTemplateSelectorProperty, dv); } #endregion ColumnHeaderTemplateSelector #region ColumnHeaderStringFormat ///is set. /// /// ColumnHeaderStringFormat DependencyProperty /// public static readonly DependencyProperty ColumnHeaderStringFormatProperty = DependencyProperty.Register( "ColumnHeaderStringFormat", typeof(String), typeof(GridView) ); ////// column header string format /// public String ColumnHeaderStringFormat { get { return (String)GetValue(ColumnHeaderStringFormatProperty); } set { SetValue(ColumnHeaderStringFormatProperty, value); } } #endregion ColumnHeaderStringFormat #region AllowsColumnReorder ////// AllowsColumnReorderProperty DependencyProperty /// public static readonly DependencyProperty AllowsColumnReorderProperty = DependencyProperty.Register( "AllowsColumnReorder", typeof(bool), typeof(GridView), new FrameworkPropertyMetadata(BooleanBoxes.TrueBox /* default value */ ) ); ////// AllowsColumnReorder /// public bool AllowsColumnReorder { get { return (bool)GetValue(AllowsColumnReorderProperty); } set { SetValue(AllowsColumnReorderProperty, value); } } #endregion AllowsColumnReorder #region ColumnHeaderContextMenu ////// ColumnHeaderContextMenuProperty DependencyProperty /// public static readonly DependencyProperty ColumnHeaderContextMenuProperty = DependencyProperty.Register( "ColumnHeaderContextMenu", typeof(ContextMenu), typeof(GridView) ); ////// ColumnHeaderContextMenu /// public ContextMenu ColumnHeaderContextMenu { get { return (ContextMenu)GetValue(ColumnHeaderContextMenuProperty); } set { SetValue(ColumnHeaderContextMenuProperty, value); } } #endregion ColumnHeaderContextMenu #region ColumnHeaderToolTip ////// ColumnHeaderToolTipProperty DependencyProperty /// public static readonly DependencyProperty ColumnHeaderToolTipProperty = DependencyProperty.Register( "ColumnHeaderToolTip", typeof(object), typeof(GridView) ); ////// ColumnHeaderToolTip /// public object ColumnHeaderToolTip { get { return GetValue(ColumnHeaderToolTipProperty); } set { SetValue(ColumnHeaderToolTipProperty, value); } } #endregion ColumnHeaderToolTip #endregion // Public Properties //------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods ////// called when ListView is prepare container for item. /// GridView override this method to attache the column collection /// /// the container protected internal override void PrepareItem(ListViewItem item) { base.PrepareItem(item); // attach GridViewColumnCollection to ListViewItem. SetColumnCollection(item, _columns); } ////// called when ListView is clear container for item. /// GridView override this method to clear the column collection /// /// the container protected internal override void ClearItem(ListViewItem item) { item.ClearValue(ColumnCollectionProperty); base.ClearItem(item); } #endregion //------------------------------------------------------------------- // // Protected Properties // //-------------------------------------------------------------------- #region Protected Properties ////// override with style key of GridView. /// protected internal override object DefaultStyleKey { get { return GridViewStyleKey; } } ////// override with style key using GridViewRowPresenter. /// protected internal override object ItemContainerDefaultStyleKey { get { return GridViewItemContainerStyleKey; } } #endregion //------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal override void OnInheritanceContextChangedCore(EventArgs args) { base.OnInheritanceContextChangedCore(args); if (_columns != null) { foreach (GridViewColumn column in _columns) { column.OnInheritanceContextChanged(args); } } } // Propagate theme changes to contained headers internal override void OnThemeChanged() { if (_columns != null) { for (int i=0; i<_columns.Count; i++) { _columns[i].OnThemeChanged(); } } } #endregion //-------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields private GridViewColumnCollection _columns; #endregion // Private Fields internal GridViewHeaderRowPresenter HeaderRowPresenter { get { return _gvheaderRP; } set { _gvheaderRP = value; } } private GridViewHeaderRowPresenter _gvheaderRP; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
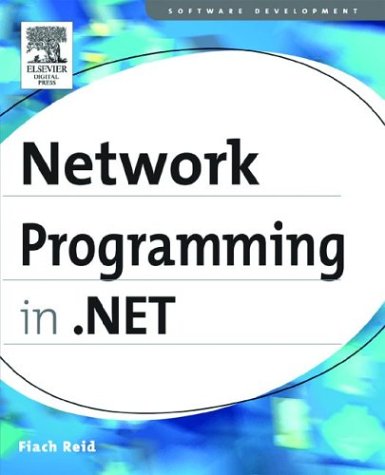
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileIOPermission.cs
- Label.cs
- EqualityComparer.cs
- XmlSerializerFactory.cs
- ThreadStateException.cs
- DesignerDataColumn.cs
- IndexedString.cs
- _ListenerRequestStream.cs
- SynchronizedCollection.cs
- SqlProvider.cs
- SmtpClient.cs
- SmtpSection.cs
- DataService.cs
- ManualResetEventSlim.cs
- XmlChildEnumerator.cs
- ListDictionaryInternal.cs
- RowsCopiedEventArgs.cs
- Translator.cs
- X509InitiatorCertificateServiceElement.cs
- TextEffectResolver.cs
- ProfileSettings.cs
- HtmlFormParameterReader.cs
- PointCollection.cs
- CompiledXpathExpr.cs
- UnsafeNativeMethodsMilCoreApi.cs
- Rule.cs
- ComponentResourceManager.cs
- OrderedDictionary.cs
- BitmapMetadata.cs
- ThousandthOfEmRealPoints.cs
- ShapingWorkspace.cs
- XsltArgumentList.cs
- PropertyGridCommands.cs
- GenericIdentity.cs
- DiscoveryOperationContextExtension.cs
- HttpVersion.cs
- SuppressIldasmAttribute.cs
- AttachInfo.cs
- CodeGenerationManager.cs
- TreeNodeClickEventArgs.cs
- ResizeGrip.cs
- WindowsImpersonationContext.cs
- XmlReflectionImporter.cs
- FixedFindEngine.cs
- ProxyManager.cs
- WebDisplayNameAttribute.cs
- ConvertersCollection.cs
- DrawingGroup.cs
- XmlSchemaSequence.cs
- RedistVersionInfo.cs
- HandlerFactoryCache.cs
- TransportContext.cs
- Transactions.cs
- LayoutEditorPart.cs
- SecurityKeyUsage.cs
- XmlConvert.cs
- ConfigurationFileMap.cs
- GroupBox.cs
- PTUtility.cs
- PathStreamGeometryContext.cs
- SerializationHelper.cs
- TextBox.cs
- StateManagedCollection.cs
- XPathDocumentIterator.cs
- ConfigurationElementCollection.cs
- AssociationSetMetadata.cs
- Point3DValueSerializer.cs
- RootBuilder.cs
- VerificationException.cs
- MetaModel.cs
- ObjectQueryState.cs
- AttributeData.cs
- DirectoryInfo.cs
- MetadataStore.cs
- GenericEnumConverter.cs
- ProgressBarAutomationPeer.cs
- DynamicResourceExtension.cs
- HttpListenerRequest.cs
- BufferedResponseStream.cs
- BitmapImage.cs
- RadialGradientBrush.cs
- ThousandthOfEmRealDoubles.cs
- DictionarySectionHandler.cs
- TraceInternal.cs
- graph.cs
- IteratorFilter.cs
- HostSecurityManager.cs
- QueryExpr.cs
- SafePointer.cs
- UIElement.cs
- WindowsToolbarItemAsMenuItem.cs
- EventTrigger.cs
- NamedPermissionSet.cs
- ReflectionUtil.cs
- ScalarOps.cs
- SetterBase.cs
- ThreadSafeList.cs
- ToolStripLabel.cs
- DateTimePicker.cs
- DesignTimeTemplateParser.cs