Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / DataGridItemAttachedStorage.cs / 1305600 / DataGridItemAttachedStorage.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows; namespace System.Windows.Controls { ////// Holds all of the information that we need to attach to row items so that we can restore rows when they're devirtualized. /// internal class DataGridItemAttachedStorage { public void SetValue(object item, DependencyProperty property, object value) { var map = EnsureItem(item); map[property] = value; } public bool TryGetValue(object item, DependencyProperty property, out object value) { value = null; Dictionarymap; EnsureItemStorageMap(); if (_itemStorageMap.TryGetValue(item, out map)) { return map.TryGetValue(property, out value); } return false; } public void ClearValue(object item, DependencyProperty property) { Dictionary map; EnsureItemStorageMap(); if (_itemStorageMap.TryGetValue(item, out map)) { map.Remove(property); } } public void ClearItem(object item) { EnsureItemStorageMap(); _itemStorageMap.Remove(item); } public void Clear() { _itemStorageMap = null; } private void EnsureItemStorageMap() { if (_itemStorageMap == null) { _itemStorageMap = new Dictionary
Link Menu
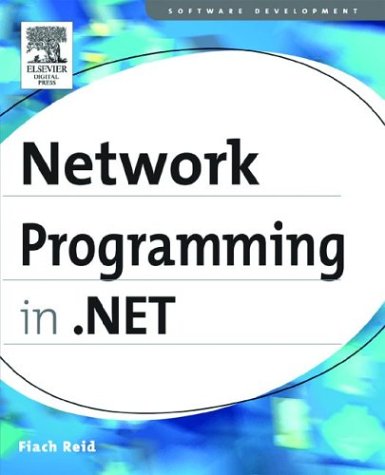
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlAuthFailedErrorFormatter.cs
- Effect.cs
- Ops.cs
- DbDataAdapter.cs
- FontDifferentiator.cs
- Win32SafeHandles.cs
- Table.cs
- MatrixCamera.cs
- Rect.cs
- IndexOutOfRangeException.cs
- TdsParserStaticMethods.cs
- SmtpMail.cs
- WebPart.cs
- PageContentAsyncResult.cs
- XmlSchemaChoice.cs
- GenericsNotImplementedException.cs
- WeakEventManager.cs
- Encoder.cs
- CopyAttributesAction.cs
- ButtonBase.cs
- OracleEncoding.cs
- ClusterUtils.cs
- RawStylusInputReport.cs
- nulltextcontainer.cs
- ContextMenuService.cs
- ColorConvertedBitmap.cs
- ClientSettingsSection.cs
- MethodBody.cs
- DebugView.cs
- SmtpNtlmAuthenticationModule.cs
- XmlSchemaAppInfo.cs
- IisTraceWebEventProvider.cs
- TableRowGroup.cs
- ToolStripRendererSwitcher.cs
- PriorityChain.cs
- XmlNamespaceDeclarationsAttribute.cs
- SingleStorage.cs
- WebBrowserContainer.cs
- SizeFConverter.cs
- ProjectionPlan.cs
- DrawingGroupDrawingContext.cs
- DiscoveryReference.cs
- TextServicesCompartmentContext.cs
- Subtract.cs
- ConstructorNeedsTagAttribute.cs
- EntityAdapter.cs
- XmlSchemaSimpleContentRestriction.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- ImageUrlEditor.cs
- MouseActionValueSerializer.cs
- ExpressionBinding.cs
- DecimalStorage.cs
- SizeChangedInfo.cs
- Listbox.cs
- CrossContextChannel.cs
- DBSchemaTable.cs
- CustomCategoryAttribute.cs
- OleDbDataAdapter.cs
- OLEDB_Enum.cs
- MailWebEventProvider.cs
- EntityProviderFactory.cs
- HttpHandlerActionCollection.cs
- SystemIcmpV6Statistics.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- BaseConfigurationRecord.cs
- RequiredFieldValidator.cs
- TemplateXamlParser.cs
- Parser.cs
- AsyncCompletedEventArgs.cs
- LicenseProviderAttribute.cs
- ArrayElementGridEntry.cs
- StateMachine.cs
- FormatConvertedBitmap.cs
- DesignTimeXamlWriter.cs
- ImmutableObjectAttribute.cs
- ControlBindingsCollection.cs
- GradientSpreadMethodValidation.cs
- BuildManager.cs
- FrameworkPropertyMetadata.cs
- ConnectionConsumerAttribute.cs
- InkSerializer.cs
- BaseInfoTable.cs
- SQLBytesStorage.cs
- UInt16Storage.cs
- WsdlInspector.cs
- ExceptionHandlersDesigner.cs
- storagemappingitemcollection.viewdictionary.cs
- TextTrailingWordEllipsis.cs
- RenderData.cs
- ObjectIDGenerator.cs
- ColumnResizeAdorner.cs
- MaterialGroup.cs
- ReaderOutput.cs
- EventLogSession.cs
- RelationshipConverter.cs
- ColorConvertedBitmapExtension.cs
- DetailsViewInsertedEventArgs.cs
- EventSinkActivityDesigner.cs
- IxmlLineInfo.cs
- AnnotationResourceCollection.cs