Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / SafeMILHandleMemoryPressure.cs / 1407647 / SafeMILHandleMemoryPressure.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Tracks the amount of native memory used by SafeMILHandle objects. //--------------------------------------------------------------------------- using System; using System.Security; using MS.Internal; using System.Threading; namespace System.Windows.Media { internal class SafeMILHandleMemoryPressure { ////// Critical: This code calls into AddMemoryPressure which has a link demand. /// [SecurityCritical] internal SafeMILHandleMemoryPressure(long gcPressure) { _gcPressure = gcPressure; _refCount = 0; if (_gcPressure > SMALL_BITMAP_MEM) { MemoryPressure.Add(_gcPressure); } else { GC.AddMemoryPressure(_gcPressure); } } internal void AddRef() { Interlocked.Increment(ref _refCount); } ////// Critical: This code calls into RemoveMemoryPressure which has a link demand. /// [SecurityCritical] internal void Release() { if (Interlocked.Decrement(ref _refCount) == 0) { if (_gcPressure > SMALL_BITMAP_MEM) { MemoryPressure.Remove(_gcPressure); } else { GC.RemoveMemoryPressure(_gcPressure); } _gcPressure = 0; } } // Estimated size in bytes of the unmanaged memory private long _gcPressure; // // SafeMILHandleMemoryPressure does its own ref counting in managed code, because the // associated memory pressure should be removed when there are no more managed // references to the unmanaged resource. There can still be references to it from // unmanaged code elsewhere, but that should not prevent the memory pressure from being // released. // private int _refCount; // // The memory usage for a "small" bitmap // // Small bitmaps will be handled by GC.AddMemoryPressure() rather than WPF's own // MemoryPressure algorithm. GC's algorithm is less aggressive than WPF's, and will // result in fewer expensive GC.Collect(2) operations. // // For now, a small bitmap is 32x32 or less. It's at 4 bytes per pixel, and the *2 is // to account for the doubled estimate in // BitmapSourceMILSafeHandle.ComputeEstimatedSize(). // private const long SMALL_BITMAP_MEM = 32 * 32 * 4 * 2; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Tracks the amount of native memory used by SafeMILHandle objects. //--------------------------------------------------------------------------- using System; using System.Security; using MS.Internal; using System.Threading; namespace System.Windows.Media { internal class SafeMILHandleMemoryPressure { ////// Critical: This code calls into AddMemoryPressure which has a link demand. /// [SecurityCritical] internal SafeMILHandleMemoryPressure(long gcPressure) { _gcPressure = gcPressure; _refCount = 0; if (_gcPressure > SMALL_BITMAP_MEM) { MemoryPressure.Add(_gcPressure); } else { GC.AddMemoryPressure(_gcPressure); } } internal void AddRef() { Interlocked.Increment(ref _refCount); } ////// Critical: This code calls into RemoveMemoryPressure which has a link demand. /// [SecurityCritical] internal void Release() { if (Interlocked.Decrement(ref _refCount) == 0) { if (_gcPressure > SMALL_BITMAP_MEM) { MemoryPressure.Remove(_gcPressure); } else { GC.RemoveMemoryPressure(_gcPressure); } _gcPressure = 0; } } // Estimated size in bytes of the unmanaged memory private long _gcPressure; // // SafeMILHandleMemoryPressure does its own ref counting in managed code, because the // associated memory pressure should be removed when there are no more managed // references to the unmanaged resource. There can still be references to it from // unmanaged code elsewhere, but that should not prevent the memory pressure from being // released. // private int _refCount; // // The memory usage for a "small" bitmap // // Small bitmaps will be handled by GC.AddMemoryPressure() rather than WPF's own // MemoryPressure algorithm. GC's algorithm is less aggressive than WPF's, and will // result in fewer expensive GC.Collect(2) operations. // // For now, a small bitmap is 32x32 or less. It's at 4 bytes per pixel, and the *2 is // to account for the doubled estimate in // BitmapSourceMILSafeHandle.ComputeEstimatedSize(). // private const long SMALL_BITMAP_MEM = 32 * 32 * 4 * 2; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
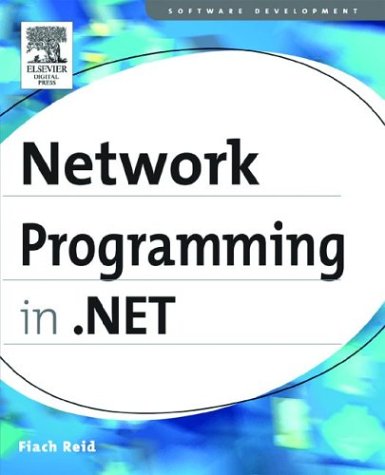
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TripleDES.cs
- StorageAssociationTypeMapping.cs
- LinkedResource.cs
- DataTableMapping.cs
- BitmapImage.cs
- XmlImplementation.cs
- StreamAsIStream.cs
- JsonReader.cs
- FontCacheLogic.cs
- BitmapImage.cs
- Line.cs
- ModulesEntry.cs
- CompiledRegexRunnerFactory.cs
- UrlParameterReader.cs
- sqlpipe.cs
- Int32.cs
- VisualTreeHelper.cs
- StyleCollectionEditor.cs
- EditorPartChrome.cs
- XmlNodeReader.cs
- AutomationElementIdentifiers.cs
- MetaColumn.cs
- ConfigXmlElement.cs
- sitestring.cs
- METAHEADER.cs
- StringBlob.cs
- LiteralTextContainerControlBuilder.cs
- ListDesigner.cs
- ReceiveMessageRecord.cs
- Byte.cs
- OdbcParameter.cs
- ControlsConfig.cs
- CursorConverter.cs
- SuppressedPackageProperties.cs
- HtmlElementErrorEventArgs.cs
- LoadedOrUnloadedOperation.cs
- BaseCollection.cs
- ToolstripProfessionalRenderer.cs
- DocobjHost.cs
- Timeline.cs
- Control.cs
- SortExpressionBuilder.cs
- SystemIPAddressInformation.cs
- Encoder.cs
- DataGridLinkButton.cs
- DocumentCollection.cs
- XmlTextEncoder.cs
- ReachIDocumentPaginatorSerializer.cs
- RequestResizeEvent.cs
- DataGridColumnCollectionEditor.cs
- AnnouncementDispatcherAsyncResult.cs
- QilXmlWriter.cs
- ValidationErrorEventArgs.cs
- BitmapEffectDrawing.cs
- SoapConverter.cs
- TimeSpanConverter.cs
- FactoryGenerator.cs
- UnrecognizedPolicyAssertionElement.cs
- EDesignUtil.cs
- DataSetFieldSchema.cs
- ControlParameter.cs
- XComponentModel.cs
- BrowsableAttribute.cs
- XmlDataSourceNodeDescriptor.cs
- SQLCharsStorage.cs
- Double.cs
- StringValueSerializer.cs
- filewebresponse.cs
- ActiveDocumentEvent.cs
- Label.cs
- VsPropertyGrid.cs
- CommandDevice.cs
- IPAddress.cs
- ScriptServiceAttribute.cs
- UpdatePanelTriggerCollection.cs
- CharacterHit.cs
- XmlSchemaComplexContent.cs
- DBDataPermissionAttribute.cs
- UIServiceHelper.cs
- SessionEndingCancelEventArgs.cs
- ControlAdapter.cs
- AuthenticationConfig.cs
- InternalCache.cs
- ServicePointManager.cs
- Solver.cs
- ContentElement.cs
- WindowsToolbar.cs
- SiteMembershipCondition.cs
- ProxyAssemblyNotLoadedException.cs
- UidManager.cs
- ImageList.cs
- CodeStatementCollection.cs
- DiagnosticsConfigurationHandler.cs
- SqlFacetAttribute.cs
- GridSplitterAutomationPeer.cs
- LinkLabelLinkClickedEvent.cs
- DeleteBookmarkScope.cs
- SettingsPropertyCollection.cs
- XmlNodeComparer.cs
- ColorBlend.cs