Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Routing / ContentPathSegment.cs / 1305376 / ContentPathSegment.cs
namespace System.Web.Routing { using System.Collections.Generic; using System.Linq; // Represents a segment of a URL that is not a separator. It contains subsegments such as literals and parameters. internal sealed class ContentPathSegment : PathSegment { public ContentPathSegment(IListsubsegments) { Subsegments = subsegments; } public bool IsCatchAll { get { // return Subsegments.Any (seg => (seg is ParameterSubsegment) && (((ParameterSubsegment)seg).IsCatchAll)); } } public IList Subsegments { get; private set; } #if ROUTE_DEBUGGING public override string LiteralText { get { List s = new List (); foreach (PathSubsegment subsegment in Subsegments) { s.Add(subsegment.LiteralText); } return String.Join(String.Empty, s.ToArray()); } } public override string ToString() { List s = new List (); foreach (PathSubsegment subsegment in Subsegments) { s.Add(subsegment.ToString()); } return "[ " + String.Join(", ", s.ToArray()) + " ]"; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web.Routing { using System.Collections.Generic; using System.Linq; // Represents a segment of a URL that is not a separator. It contains subsegments such as literals and parameters. internal sealed class ContentPathSegment : PathSegment { public ContentPathSegment(IList subsegments) { Subsegments = subsegments; } public bool IsCatchAll { get { // return Subsegments.Any (seg => (seg is ParameterSubsegment) && (((ParameterSubsegment)seg).IsCatchAll)); } } public IList Subsegments { get; private set; } #if ROUTE_DEBUGGING public override string LiteralText { get { List s = new List (); foreach (PathSubsegment subsegment in Subsegments) { s.Add(subsegment.LiteralText); } return String.Join(String.Empty, s.ToArray()); } } public override string ToString() { List s = new List (); foreach (PathSubsegment subsegment in Subsegments) { s.Add(subsegment.ToString()); } return "[ " + String.Join(", ", s.ToArray()) + " ]"; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
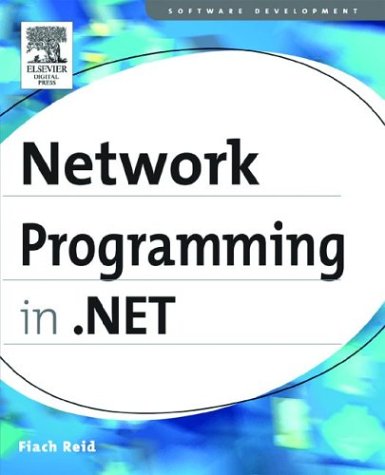
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnionExpr.cs
- TraceHwndHost.cs
- ZipIOExtraField.cs
- Vector3D.cs
- HttpRuntimeSection.cs
- ZipIOLocalFileBlock.cs
- ObfuscateAssemblyAttribute.cs
- BinaryObjectWriter.cs
- BaseAddressElement.cs
- RegionData.cs
- PointHitTestResult.cs
- AnnotationAuthorChangedEventArgs.cs
- RestHandlerFactory.cs
- PolicyStatement.cs
- SqlCacheDependencyDatabaseCollection.cs
- _UriTypeConverter.cs
- SqlDataSourceStatusEventArgs.cs
- AxHost.cs
- NavigationWindowAutomationPeer.cs
- ComponentDispatcherThread.cs
- XPathAncestorQuery.cs
- CodeTypeConstructor.cs
- Row.cs
- ValidationError.cs
- ErrorsHelper.cs
- TagPrefixInfo.cs
- TextDecoration.cs
- BmpBitmapEncoder.cs
- GZipStream.cs
- ColumnHeaderCollectionEditor.cs
- LocalizableAttribute.cs
- TemplatedAdorner.cs
- NumberFormatInfo.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- PageFunction.cs
- FormView.cs
- ScaleTransform3D.cs
- GridLengthConverter.cs
- XmlAnyElementAttribute.cs
- InternalPermissions.cs
- TemplatedWizardStep.cs
- CodeDOMUtility.cs
- DrawingContextWalker.cs
- Encoder.cs
- CaseInsensitiveHashCodeProvider.cs
- AllMembershipCondition.cs
- KerberosRequestorSecurityTokenAuthenticator.cs
- XmlSchemaComplexType.cs
- PresentationTraceSources.cs
- EntityContainerEmitter.cs
- CodeConstructor.cs
- TextEditorTables.cs
- EpmContentDeSerializer.cs
- _BaseOverlappedAsyncResult.cs
- DataShape.cs
- ObjectDataSourceFilteringEventArgs.cs
- CaseCqlBlock.cs
- HttpRuntime.cs
- AnimationClock.cs
- ValidationRuleCollection.cs
- SystemIPv6InterfaceProperties.cs
- IssuedSecurityTokenProvider.cs
- LinkedList.cs
- ListViewItem.cs
- ListViewAutomationPeer.cs
- _NetworkingPerfCounters.cs
- SqlAliasesReferenced.cs
- PrivilegeNotHeldException.cs
- URLIdentityPermission.cs
- ValidationPropertyAttribute.cs
- ImageKeyConverter.cs
- CodeCastExpression.cs
- PipelineModuleStepContainer.cs
- EventMappingSettingsCollection.cs
- Accessors.cs
- Literal.cs
- DataSourceViewSchemaConverter.cs
- CodeTypeReferenceExpression.cs
- BuilderPropertyEntry.cs
- Site.cs
- SqlDataSourceWizardForm.cs
- XXXOnTypeBuilderInstantiation.cs
- DocumentReference.cs
- Token.cs
- TextProviderWrapper.cs
- KnownTypesProvider.cs
- DoubleAverageAggregationOperator.cs
- StringCollectionMarkupSerializer.cs
- XslVisitor.cs
- Timeline.cs
- DWriteFactory.cs
- DiscoveryCallbackBehavior.cs
- UncommonField.cs
- XmlSchemaProviderAttribute.cs
- DoubleSumAggregationOperator.cs
- RegexReplacement.cs
- AliasedExpr.cs
- CultureTableRecord.cs
- WindowsListViewGroupSubsetLink.cs
- SqlTypeConverter.cs