Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmContentDeSerializer.cs / 1305376 / EpmContentDeSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Class used for deserializing EntityPropertyMappingAttribute content. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Collections.Generic; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq; using System.ServiceModel.Syndication; #endregion ///DeSerializer for reading EPM content internal sealed class EpmContentDeSerializer { ///private readonly ResourceType resourceType; /// for which this serializer works Object for which this serializer works private readonly object element; ////// Constructor creates contained serializers /// /// Resource type being serialized /// Instance ofinternal EpmContentDeSerializer(ResourceType resourceType, object element) { Debug.Assert(resourceType.HasEntityPropertyMappings == true, "Must have entity property mappings to instantiate EpmContentDeSerializer"); this.resourceType = resourceType; this.element = element; this.resourceType.EnsureEpmInfoAvailability(); } /// Delegates to each of custom and syndication deserializers for serializing content ///to deserialize /// State of the deserializer internal void DeSerialize(SyndicationItem item, EpmContentDeserializerState state) { if (this.resourceType.EpmTargetTree.SyndicationRoot.SubSegments.Count > 0) { new EpmSyndicationContentDeSerializer(item, state).DeSerialize(this.resourceType, this.element); } if (this.resourceType.EpmTargetTree.NonSyndicationRoot.SubSegments.Count > 0) { new EpmCustomContentDeSerializer(item, state).DeSerialize(this.resourceType, this.element); } } /// Representation of deserializer state internal sealed class EpmContentDeserializerState { ///Is current operation an update public bool IsUpdateOperation { get; set; } ///IUpdatable used for updating the object public UpdatableWrapper Updatable { get; set; } ///Service instance public IDataService Service { get; set; } ///Properties that have been applied public EpmAppliedPropertyInfo PropertiesApplied { get; set; } } ///Holder of information about properties applied during deserialization of an object internal sealed class EpmAppliedPropertyInfo { ///Properties already applied private Listproperties; /// Map from properties to their corresponding type names private ListpropertyToTypeNameMap; /// Propeties already applied private IListProperties { get { if (this.properties == null) { this.properties = new List (); } return this.properties; } } /// Propeties to type name mappings private IListPropertyToTypeNameMap { get { if (this.propertyToTypeNameMap == null) { this.propertyToTypeNameMap = new List (); } return this.propertyToTypeNameMap; } } /// Adds the given property to the collection of applied ones /// Path of property /// Does the path include all sub-properties internal void AddAppliedProperty(String propertyPath, bool wholePathCovered) { this.Properties.Add(new EpmAppliedProperty { PropertyPath = propertyPath, ApplyRecursive = wholePathCovered }); } ///Adds the given property to the collection of applied ones /// Path of property /// Type of the property internal void AddPropertyToTypeMapItem(String propertyPath, String typeName) { this.PropertyToTypeNameMap.Add(new EpmPropertyToTypeMappingElement { PropertyPath = propertyPath, TypeName = typeName }); } ///Checks if the given path is already applied /// Given property path ///true if the property has already been applied, false otherwise internal bool Lookup(String propertyPath) { return this.properties != null && this.Properties.Any(e => e.PropertyPath == propertyPath || (e.ApplyRecursive == true && e.PropertyPath.Length <= propertyPath.Length && e.PropertyPath == propertyPath.Substring(0, e.PropertyPath.Length))); } ///Looksup the type given a property path /// Given property path ///String containing mapped type name, null otherwise internal String MapPropertyToType(String propertyPath) { if (this.propertyToTypeNameMap != null) { EpmPropertyToTypeMappingElement mapping = this.PropertyToTypeNameMap.FirstOrDefault(e => e.PropertyPath == propertyPath); return mapping != null ? mapping.TypeName : null; } else { return null; } } ///Property that is applied private sealed class EpmAppliedProperty { ///Path of property public String PropertyPath { get; set; } ///Is the property application path considered recursive public bool ApplyRecursive { get; set; } } ///Maps a property path with the type private sealed class EpmPropertyToTypeMappingElement { ///Path of property public String PropertyPath { get; set; } ///Type of the property public String TypeName { get; set; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
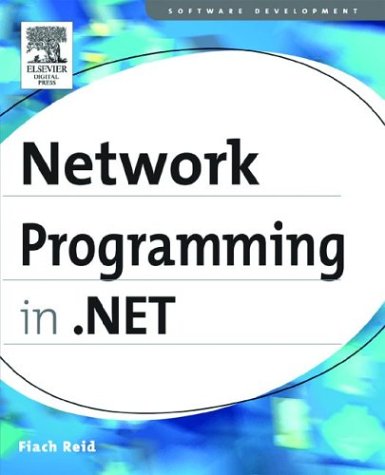
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WSFederationHttpBindingCollectionElement.cs
- PageAsyncTask.cs
- SafeNativeMethods.cs
- TraceSection.cs
- GroupBox.cs
- ValidatingPropertiesEventArgs.cs
- StorageInfo.cs
- TextEditorSpelling.cs
- EncodingTable.cs
- ImageSourceConverter.cs
- SessionSwitchEventArgs.cs
- XsdBuilder.cs
- WebRequestModuleElement.cs
- KeyboardDevice.cs
- CharEntityEncoderFallback.cs
- DataListCommandEventArgs.cs
- RtfNavigator.cs
- X509Certificate2.cs
- XPathExpr.cs
- CursorConverter.cs
- DocumentViewerBaseAutomationPeer.cs
- SystemPens.cs
- MessagePropertyFilter.cs
- PropertyBuilder.cs
- FocusManager.cs
- TextTrailingCharacterEllipsis.cs
- NameValueFileSectionHandler.cs
- DataGridViewToolTip.cs
- ListBoxItemWrapperAutomationPeer.cs
- CommandHelpers.cs
- DesignerView.Commands.cs
- CellCreator.cs
- DbSetClause.cs
- CodeMemberField.cs
- BlurBitmapEffect.cs
- GlyphRunDrawing.cs
- XmlDataProvider.cs
- XmlElementList.cs
- ObjRef.cs
- SessionPageStateSection.cs
- CompatibleComparer.cs
- DataGridViewCellLinkedList.cs
- TriggerActionCollection.cs
- WebReference.cs
- SubpageParagraph.cs
- SequenceNumber.cs
- ColorConvertedBitmap.cs
- SHA384Cng.cs
- MessageCredentialType.cs
- Package.cs
- PropertyChangingEventArgs.cs
- AccessControlList.cs
- XmlRootAttribute.cs
- DeclarationUpdate.cs
- AppAction.cs
- FormViewModeEventArgs.cs
- AuthenticationService.cs
- SweepDirectionValidation.cs
- TypeHelper.cs
- BaseCodePageEncoding.cs
- CellIdBoolean.cs
- FixedPageStructure.cs
- EntitySet.cs
- Divide.cs
- SqlDelegatedTransaction.cs
- SecurityDocument.cs
- SegmentInfo.cs
- PeerResolverBindingElement.cs
- GridViewRowCollection.cs
- StylusPointProperties.cs
- BitmapFrameDecode.cs
- AlternateView.cs
- OutputCacheModule.cs
- KnownBoxes.cs
- IPGlobalProperties.cs
- DuplicateWaitObjectException.cs
- FileLoadException.cs
- WebBrowsableAttribute.cs
- TranslateTransform3D.cs
- OSFeature.cs
- ControlBuilder.cs
- TimelineGroup.cs
- GraphicsContext.cs
- DelayedRegex.cs
- XsdBuilder.cs
- FilterEventArgs.cs
- BindingOperations.cs
- KnownTypesHelper.cs
- FlowDocumentPage.cs
- ThicknessKeyFrameCollection.cs
- ByeMessage11.cs
- DataControlFieldCollection.cs
- ProviderConnectionPointCollection.cs
- SqlColumnizer.cs
- AnimationStorage.cs
- RootProfilePropertySettingsCollection.cs
- Canvas.cs
- TextRunCacheImp.cs
- WebPartDisplayMode.cs
- UnicastIPAddressInformationCollection.cs