Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / Instrumentation / Attributes.cs / 1305376 / Attributes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Management.Instrumentation { using System; using System.Reflection; using System.Collections; using System.Text.RegularExpressions; using System.Management; using System.Globalization; ////// ///Specifies that this assembly provides management instrumentation. This attribute should appear one time per assembly. ////// [AttributeUsage(AttributeTargets.Assembly)] public class InstrumentedAttribute : Attribute { string namespaceName; string securityDescriptor; ///For more information about using attributes, see ///. /// Initializes a new instance /// of the ///class. /// /// public InstrumentedAttribute() : this(null, null) {} ///Initializes a new instance of the ////// class that is set for the root\default namespace. This is the default constructor. /// /// The namespace for instrumentation instances and events. public InstrumentedAttribute(string namespaceName) : this(namespaceName, null) {} ///Initializes a new instance of the ///class that is set to the specified namespace for instrumentation within this assembly. /// /// The namespace for instrumentation instances and events. /// A security descriptor that allows only the specified users or groups to run applications that provide the instrumentation supported by this assembly. public InstrumentedAttribute(string namespaceName, string securityDescriptor) { // if(namespaceName != null) namespaceName = namespaceName.Replace('/', '\\'); if(namespaceName == null || namespaceName.Length == 0) namespaceName = "root\\default"; // bug#60933 Use a default namespace if null bool once = true; foreach(string namespacePart in namespaceName.Split('\\')) { if( namespacePart.Length == 0 || (once && String.Compare(namespacePart, "root", StringComparison.OrdinalIgnoreCase) != 0) // Must start with 'root' || !Regex.Match(namespacePart, @"^[a-z,A-Z]").Success // All parts must start with letter || Regex.Match(namespacePart, @"_$").Success // Must not end with an underscore || Regex.Match(namespacePart, @"[^a-z,A-Z,0-9,_,\u0080-\uFFFF]").Success) // Only letters, digits, or underscores { ManagementException.ThrowWithExtendedInfo(ManagementStatus.InvalidNamespace); } once = false; } this.namespaceName = namespaceName; this.securityDescriptor = securityDescriptor; } ///Initializes a new instance of the ///class that is set to the specified namespace and security settings for instrumentation within this assembly. /// ///Gets or sets the namespace for instrumentation instances and events in this assembly. ////// ///If not specified, the default namespace will be set as "\\.\root\default". /// Otherwise, a string indicating the name of the namespace for instrumentation /// instances and events in this assembly. ////// It is highly recommended that the namespace name be specified by the /// assembly, and that it should be a unique namespace per assembly, or per /// application. Having a specific namespace for each assembly or /// application instrumentation allows more granularity for securing access to /// instrumentation provided by different assemblies or applications. /// public string NamespaceName { get { return namespaceName == null ? string.Empty : namespaceName; } } ////// ///Gets or sets a security descriptor that allows only the specified users or groups to run /// applications that provide the instrumentation supported by this assembly. ////// ////// If null, the default value is defined to include the /// following security groups : ///, , , and . This will only allow /// members of these security groups /// to publish data and fire events from this assembly. Otherwise, this is a string in SDDL format representing the security /// descriptor that defines which users and groups can provide instrumentation data /// and events from this application. ////// ///Users or groups not specified in this /// security descriptor may still run the application, but cannot provide /// instrumentation from this assembly. ////// public string SecurityDescriptor { get { // This will never return an empty string. Instead, it will // return null, or a non-zero length string if(null == securityDescriptor || securityDescriptor.Length == 0) return null; return securityDescriptor; } } internal static InstrumentedAttribute GetAttribute(Assembly assembly) { Object [] rg = assembly.GetCustomAttributes(typeof(InstrumentedAttribute), false); if(rg.Length > 0) return ((InstrumentedAttribute)rg[0]); return new InstrumentedAttribute(); } internal static Type[] GetInstrumentedTypes(Assembly assembly) { ArrayList types = new ArrayList(); // // The recursion has been moved to the parent level to avoid ineffiency of wading through all types // at each stage of recursion. Also, the recursive method has been replaced with the more correct: // GetInstrumentedParentTypes method (see comments on header). // foreach (Type type in assembly.GetTypes()) { if (IsInstrumentationClass(type)) { GetInstrumentedParentTypes(types, type); } } return (Type[])types.ToArray(typeof(Type)); } // // Recursive function that adds the type to the array and recurses on the parent type. The end condition // is either no parent type or a parent type which is not marked as instrumented. // static void GetInstrumentedParentTypes(ArrayList types, Type childType) { if (types.Contains(childType) == false) { Type parentType = InstrumentationClassAttribute.GetBaseInstrumentationType(childType) ; // // If we have a instrumented base type and it has not already // been included in the list of instrumented types // traverse the inheritance hierarchy. // if (parentType != null) { GetInstrumentedParentTypes(types, parentType); } types.Add(childType); } } static bool IsInstrumentationClass(Type type) { return (null != InstrumentationClassAttribute.GetAttribute(type)); } } ///The SDDL representing the default set of security groups defined above is as /// follows : ///O:BAG:BAD:(A;;0x10000001;;;BA)(A;;0x10000001;;;SY)(A;;0x10000001;;;LA)(A;;0x10000001;;;S-1-5-20)(A;;0x10000001;;;S-1-5-19) ///To add the ///group to the users allowed to fire events or publish /// instances from this assembly, the attribute should be specificed as /// follows : [Instrumented("root\\MyApplication", "O:BAG:BAD:(A;;0x10000001;;;BA)(A;;0x10000001;;;SY)(A;;0x10000001;;;LA)(A;;0x10000001;;;S-1-5-20)(A;;0x10000001;;;S-1-5-19)(A;;0x10000001;;;PU)")] ////// ///Specifies the type of instrumentation provided by a class. ////// public enum InstrumentationType { ///using System; /// using System.Management; /// using System.Configuration.Install; /// using System.Management.Instrumentation; /// /// // This example demonstrates how to create a Management Event class by using /// // the InstrumentationClass attribute and to fire a Management Event from /// // managed code. /// /// // Specify which namespace the Management Event class is created in /// [assembly:Instrumented("Root/Default")] /// /// // Let the system know you will run InstallUtil.exe utility against /// // this assembly /// [System.ComponentModel.RunInstaller(true)] /// public class MyInstaller : DefaultManagementProjectInstaller {} /// /// // Create a Management Instrumentation Event class /// [InstrumentationClass(InstrumentationType.Event)] /// public class MyEvent /// { /// public string EventName; /// } /// /// public class WMI_InstrumentedEvent_Example /// { /// public static void Main() { /// MyEvent e = new MyEvent(); /// e.EventName = "Hello"; /// /// // Fire a Management Event /// Instrumentation.Fire(e); /// /// return; /// } /// } ///
///Imports System /// Imports System.Management /// Imports System.Configuration.Install /// Imports System.Management.Instrumentation /// /// ' This sample demonstrates how to create a Management Event class by using /// ' the InstrumentationClass attribute and to fire a Management Event from /// ' managed code. /// /// ' Specify which namespace the Manaegment Event class is created in /// <assembly: Instrumented("Root/Default")> /// /// ' Let the system know InstallUtil.exe utility will be run against /// ' this assembly /// <System.ComponentModel.RunInstaller(True)> _ /// Public Class MyInstaller /// Inherits DefaultManagementProjectInstaller /// End Class 'MyInstaller /// /// ' Create a Management Instrumentation Event class /// <InstrumentationClass(InstrumentationType.Event)> _ /// Public Class MyEvent /// Public EventName As String /// End Class /// /// Public Class Sample_EventProvider /// Public Shared Function Main(args() As String) As Integer /// Dim e As New MyEvent() /// e.EventName = "Hello" /// /// ' Fire a Management Event /// Instrumentation.Fire(e) /// /// Return 0 /// End Function /// End Class ///
////// Instance, ///Specifies that the class provides instances for management instrumentation. ////// Event, ///Specifies that the class provides events for management instrumentation. ////// Abstract } ///Specifies that the class defines an abstract class for management instrumentation. ////// Specifies that a class provides event or instance instrumentation. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Struct)] public class InstrumentationClassAttribute : Attribute { InstrumentationType instrumentationType; string managedBaseClassName; ////// Initializes a new instance /// of the ///class. /// /// /// The type of instrumentation provided by this class. public InstrumentationClassAttribute(InstrumentationType instrumentationType) { this.instrumentationType = instrumentationType; } ///Initializes a new instance of the ///class that is used if this type is derived from another type that has the attribute, or if this is a /// top-level instrumentation class (for example, an instance or abstract class /// without a base class, or an event derived from ). /// /// The type of instrumentation provided by this class. /// The name of the base class. public InstrumentationClassAttribute(InstrumentationType instrumentationType, string managedBaseClassName) { this.instrumentationType = instrumentationType; this.managedBaseClassName = managedBaseClassName; } ///Initializes a new instance of the ///class that /// has schema for an existing base class. The class must contain /// proper member definitions for the properties of the existing /// WMI base class. /// ///Gets or sets the type of instrumentation provided by this class. ////// Contains an public InstrumentationType InstrumentationType { get { return instrumentationType; } } ///value that /// indicates whether this is an instrumented event, instance or abstract class. /// /// ///Gets or sets the name of the base class of this instrumentation class. ////// public string ManagedBaseClassName { get { // This will never return an empty string. Instead, it will // return null, or a non-zero length string if(null == managedBaseClassName || managedBaseClassName.Length == 0) return null; return managedBaseClassName; } } internal static InstrumentationClassAttribute GetAttribute(Type type) { // We don't want BaseEvent or Instance to look like that have an 'InstrumentedClass' attribute if(type == typeof(BaseEvent) || type == typeof(Instance)) return null; // We will inherit the 'InstrumentedClass' attribute from a base class Object [] rg = type.GetCustomAttributes(typeof(InstrumentationClassAttribute), true); if(rg.Length > 0) return ((InstrumentationClassAttribute)rg[0]); return null; } ///If not null, this string indicates the WMI baseclass that this class inherits /// from in the CIM schema. ////// /// ///Displays the ///of the base class. /// internal static Type GetBaseInstrumentationType(Type type) { // If the BaseType has a InstrumentationClass attribute, // we return the BaseType if(GetAttribute(type.BaseType) != null) return type.BaseType; return null; } } ///The ///of the base class, if this class is derived from another /// instrumentation class; otherwise, null. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Field | AttributeTargets.Property | AttributeTargets.Method)] public class ManagedNameAttribute : Attribute { string name; ///Allows an instrumented class, or member of an instrumented class, /// to present an alternate name through management instrumentation. ////// ///Gets the name of the managed entity. ////// Contains the name of the managed entity. /// public string Name { get { return name ; } } ////// /// The alternate name for the type, field, property, method, or parameter to which this attribute is applied. public ManagedNameAttribute(string name) { this.name = name; } internal static string GetMemberName(MemberInfo member) { // This works for all sorts of things: Type, MethodInfo, PropertyInfo, FieldInfo Object [] rg = member.GetCustomAttributes(typeof(ManagedNameAttribute), false); if(rg.Length > 0) { // bug#69115 - if null or empty string are passed, we just ignore this attribute ManagedNameAttribute attr = (ManagedNameAttribute)rg[0]; if(attr.name != null && attr.name.Length != 0) return attr.name; } return member.Name; } internal static string GetBaseClassName(Type type) { InstrumentationClassAttribute attr = InstrumentationClassAttribute.GetAttribute(type); string name = attr.ManagedBaseClassName; if(name != null) return name; // Get managed base type's attribute InstrumentationClassAttribute attrParent = InstrumentationClassAttribute.GetAttribute(type.BaseType); // If the base type does not have a InstrumentationClass attribute, // return a base type based on the InstrumentationType if(null == attrParent) { switch(attr.InstrumentationType) { case InstrumentationType.Abstract: return null; case InstrumentationType.Instance: return null; case InstrumentationType.Event: return "__ExtrinsicEvent"; default: break; } } // Our parent was also a managed provider type. Use it's managed name. return GetMemberName(type.BaseType); } } ///Initializes a new instance of the ///class that allows the alternate name to be specified /// for the type, field, property, method, or parameter to which this attribute is applied. /// [AttributeUsage(AttributeTargets.Field | AttributeTargets.Property | AttributeTargets.Method)] public class IgnoreMemberAttribute : Attribute { } #if REQUIRES_EXPLICIT_DECLARATION_OF_INHERITED_PROPERTIES ///Allows a particular member of an instrumented class to be ignored /// by management instrumentation ////// [AttributeUsage(AttributeTargets.Field)] public class InheritedPropertyAttribute : Attribute { internal static InheritedPropertyAttribute GetAttribute(FieldInfo field) { Object [] rg = field.GetCustomAttributes(typeof(InheritedPropertyAttribute), false); if(rg.Length > 0) return ((InheritedPropertyAttribute)rg[0]); return null; } } #endif #if SUPPORTS_WMI_DEFAULT_VAULES [AttributeUsage(AttributeTargets.Field)] internal class ManagedDefaultValueAttribute : Attribute { Object defaultValue; public ManagedDefaultValueAttribute(Object defaultValue) { this.defaultValue = defaultValue; } public static Object GetManagedDefaultValue(FieldInfo field) { Object [] rg = field.GetCustomAttributes(typeof(ManagedDefaultValueAttribute), false); if(rg.Length > 0) return ((ManagedDefaultValueAttribute)rg[0]).defaultValue; return null; } } #endif #if SUPPORTS_ALTERNATE_WMI_PROPERTY_TYPE [AttributeUsage(AttributeTargets.Field)] internal class ManagedTypeAttribute : Attribute { Type type; public ManagedTypeAttribute(Type type) { this.type = type; } public static Type GetManagedType(FieldInfo field) { Object [] rg = field.GetCustomAttributes(typeof(ManagedTypeAttribute), false); if(rg.Length > 0) return ((ManagedTypeAttribute)rg[0]).type; return field.FieldType; } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Management.Instrumentation { using System; using System.Reflection; using System.Collections; using System.Text.RegularExpressions; using System.Management; using System.Globalization; ////// ///Specifies that this assembly provides management instrumentation. This attribute should appear one time per assembly. ////// [AttributeUsage(AttributeTargets.Assembly)] public class InstrumentedAttribute : Attribute { string namespaceName; string securityDescriptor; ///For more information about using attributes, see ///. /// Initializes a new instance /// of the ///class. /// /// public InstrumentedAttribute() : this(null, null) {} ///Initializes a new instance of the ////// class that is set for the root\default namespace. This is the default constructor. /// /// The namespace for instrumentation instances and events. public InstrumentedAttribute(string namespaceName) : this(namespaceName, null) {} ///Initializes a new instance of the ///class that is set to the specified namespace for instrumentation within this assembly. /// /// The namespace for instrumentation instances and events. /// A security descriptor that allows only the specified users or groups to run applications that provide the instrumentation supported by this assembly. public InstrumentedAttribute(string namespaceName, string securityDescriptor) { // if(namespaceName != null) namespaceName = namespaceName.Replace('/', '\\'); if(namespaceName == null || namespaceName.Length == 0) namespaceName = "root\\default"; // bug#60933 Use a default namespace if null bool once = true; foreach(string namespacePart in namespaceName.Split('\\')) { if( namespacePart.Length == 0 || (once && String.Compare(namespacePart, "root", StringComparison.OrdinalIgnoreCase) != 0) // Must start with 'root' || !Regex.Match(namespacePart, @"^[a-z,A-Z]").Success // All parts must start with letter || Regex.Match(namespacePart, @"_$").Success // Must not end with an underscore || Regex.Match(namespacePart, @"[^a-z,A-Z,0-9,_,\u0080-\uFFFF]").Success) // Only letters, digits, or underscores { ManagementException.ThrowWithExtendedInfo(ManagementStatus.InvalidNamespace); } once = false; } this.namespaceName = namespaceName; this.securityDescriptor = securityDescriptor; } ///Initializes a new instance of the ///class that is set to the specified namespace and security settings for instrumentation within this assembly. /// ///Gets or sets the namespace for instrumentation instances and events in this assembly. ////// ///If not specified, the default namespace will be set as "\\.\root\default". /// Otherwise, a string indicating the name of the namespace for instrumentation /// instances and events in this assembly. ////// It is highly recommended that the namespace name be specified by the /// assembly, and that it should be a unique namespace per assembly, or per /// application. Having a specific namespace for each assembly or /// application instrumentation allows more granularity for securing access to /// instrumentation provided by different assemblies or applications. /// public string NamespaceName { get { return namespaceName == null ? string.Empty : namespaceName; } } ////// ///Gets or sets a security descriptor that allows only the specified users or groups to run /// applications that provide the instrumentation supported by this assembly. ////// ////// If null, the default value is defined to include the /// following security groups : ///, , , and . This will only allow /// members of these security groups /// to publish data and fire events from this assembly. Otherwise, this is a string in SDDL format representing the security /// descriptor that defines which users and groups can provide instrumentation data /// and events from this application. ////// ///Users or groups not specified in this /// security descriptor may still run the application, but cannot provide /// instrumentation from this assembly. ////// public string SecurityDescriptor { get { // This will never return an empty string. Instead, it will // return null, or a non-zero length string if(null == securityDescriptor || securityDescriptor.Length == 0) return null; return securityDescriptor; } } internal static InstrumentedAttribute GetAttribute(Assembly assembly) { Object [] rg = assembly.GetCustomAttributes(typeof(InstrumentedAttribute), false); if(rg.Length > 0) return ((InstrumentedAttribute)rg[0]); return new InstrumentedAttribute(); } internal static Type[] GetInstrumentedTypes(Assembly assembly) { ArrayList types = new ArrayList(); // // The recursion has been moved to the parent level to avoid ineffiency of wading through all types // at each stage of recursion. Also, the recursive method has been replaced with the more correct: // GetInstrumentedParentTypes method (see comments on header). // foreach (Type type in assembly.GetTypes()) { if (IsInstrumentationClass(type)) { GetInstrumentedParentTypes(types, type); } } return (Type[])types.ToArray(typeof(Type)); } // // Recursive function that adds the type to the array and recurses on the parent type. The end condition // is either no parent type or a parent type which is not marked as instrumented. // static void GetInstrumentedParentTypes(ArrayList types, Type childType) { if (types.Contains(childType) == false) { Type parentType = InstrumentationClassAttribute.GetBaseInstrumentationType(childType) ; // // If we have a instrumented base type and it has not already // been included in the list of instrumented types // traverse the inheritance hierarchy. // if (parentType != null) { GetInstrumentedParentTypes(types, parentType); } types.Add(childType); } } static bool IsInstrumentationClass(Type type) { return (null != InstrumentationClassAttribute.GetAttribute(type)); } } ///The SDDL representing the default set of security groups defined above is as /// follows : ///O:BAG:BAD:(A;;0x10000001;;;BA)(A;;0x10000001;;;SY)(A;;0x10000001;;;LA)(A;;0x10000001;;;S-1-5-20)(A;;0x10000001;;;S-1-5-19) ///To add the ///group to the users allowed to fire events or publish /// instances from this assembly, the attribute should be specificed as /// follows : [Instrumented("root\\MyApplication", "O:BAG:BAD:(A;;0x10000001;;;BA)(A;;0x10000001;;;SY)(A;;0x10000001;;;LA)(A;;0x10000001;;;S-1-5-20)(A;;0x10000001;;;S-1-5-19)(A;;0x10000001;;;PU)")] ////// ///Specifies the type of instrumentation provided by a class. ////// public enum InstrumentationType { ///using System; /// using System.Management; /// using System.Configuration.Install; /// using System.Management.Instrumentation; /// /// // This example demonstrates how to create a Management Event class by using /// // the InstrumentationClass attribute and to fire a Management Event from /// // managed code. /// /// // Specify which namespace the Management Event class is created in /// [assembly:Instrumented("Root/Default")] /// /// // Let the system know you will run InstallUtil.exe utility against /// // this assembly /// [System.ComponentModel.RunInstaller(true)] /// public class MyInstaller : DefaultManagementProjectInstaller {} /// /// // Create a Management Instrumentation Event class /// [InstrumentationClass(InstrumentationType.Event)] /// public class MyEvent /// { /// public string EventName; /// } /// /// public class WMI_InstrumentedEvent_Example /// { /// public static void Main() { /// MyEvent e = new MyEvent(); /// e.EventName = "Hello"; /// /// // Fire a Management Event /// Instrumentation.Fire(e); /// /// return; /// } /// } ///
///Imports System /// Imports System.Management /// Imports System.Configuration.Install /// Imports System.Management.Instrumentation /// /// ' This sample demonstrates how to create a Management Event class by using /// ' the InstrumentationClass attribute and to fire a Management Event from /// ' managed code. /// /// ' Specify which namespace the Manaegment Event class is created in /// <assembly: Instrumented("Root/Default")> /// /// ' Let the system know InstallUtil.exe utility will be run against /// ' this assembly /// <System.ComponentModel.RunInstaller(True)> _ /// Public Class MyInstaller /// Inherits DefaultManagementProjectInstaller /// End Class 'MyInstaller /// /// ' Create a Management Instrumentation Event class /// <InstrumentationClass(InstrumentationType.Event)> _ /// Public Class MyEvent /// Public EventName As String /// End Class /// /// Public Class Sample_EventProvider /// Public Shared Function Main(args() As String) As Integer /// Dim e As New MyEvent() /// e.EventName = "Hello" /// /// ' Fire a Management Event /// Instrumentation.Fire(e) /// /// Return 0 /// End Function /// End Class ///
////// Instance, ///Specifies that the class provides instances for management instrumentation. ////// Event, ///Specifies that the class provides events for management instrumentation. ////// Abstract } ///Specifies that the class defines an abstract class for management instrumentation. ////// Specifies that a class provides event or instance instrumentation. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Struct)] public class InstrumentationClassAttribute : Attribute { InstrumentationType instrumentationType; string managedBaseClassName; ////// Initializes a new instance /// of the ///class. /// /// /// The type of instrumentation provided by this class. public InstrumentationClassAttribute(InstrumentationType instrumentationType) { this.instrumentationType = instrumentationType; } ///Initializes a new instance of the ///class that is used if this type is derived from another type that has the attribute, or if this is a /// top-level instrumentation class (for example, an instance or abstract class /// without a base class, or an event derived from ). /// /// The type of instrumentation provided by this class. /// The name of the base class. public InstrumentationClassAttribute(InstrumentationType instrumentationType, string managedBaseClassName) { this.instrumentationType = instrumentationType; this.managedBaseClassName = managedBaseClassName; } ///Initializes a new instance of the ///class that /// has schema for an existing base class. The class must contain /// proper member definitions for the properties of the existing /// WMI base class. /// ///Gets or sets the type of instrumentation provided by this class. ////// Contains an public InstrumentationType InstrumentationType { get { return instrumentationType; } } ///value that /// indicates whether this is an instrumented event, instance or abstract class. /// /// ///Gets or sets the name of the base class of this instrumentation class. ////// public string ManagedBaseClassName { get { // This will never return an empty string. Instead, it will // return null, or a non-zero length string if(null == managedBaseClassName || managedBaseClassName.Length == 0) return null; return managedBaseClassName; } } internal static InstrumentationClassAttribute GetAttribute(Type type) { // We don't want BaseEvent or Instance to look like that have an 'InstrumentedClass' attribute if(type == typeof(BaseEvent) || type == typeof(Instance)) return null; // We will inherit the 'InstrumentedClass' attribute from a base class Object [] rg = type.GetCustomAttributes(typeof(InstrumentationClassAttribute), true); if(rg.Length > 0) return ((InstrumentationClassAttribute)rg[0]); return null; } ///If not null, this string indicates the WMI baseclass that this class inherits /// from in the CIM schema. ////// /// ///Displays the ///of the base class. /// internal static Type GetBaseInstrumentationType(Type type) { // If the BaseType has a InstrumentationClass attribute, // we return the BaseType if(GetAttribute(type.BaseType) != null) return type.BaseType; return null; } } ///The ///of the base class, if this class is derived from another /// instrumentation class; otherwise, null. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Field | AttributeTargets.Property | AttributeTargets.Method)] public class ManagedNameAttribute : Attribute { string name; ///Allows an instrumented class, or member of an instrumented class, /// to present an alternate name through management instrumentation. ////// ///Gets the name of the managed entity. ////// Contains the name of the managed entity. /// public string Name { get { return name ; } } ////// /// The alternate name for the type, field, property, method, or parameter to which this attribute is applied. public ManagedNameAttribute(string name) { this.name = name; } internal static string GetMemberName(MemberInfo member) { // This works for all sorts of things: Type, MethodInfo, PropertyInfo, FieldInfo Object [] rg = member.GetCustomAttributes(typeof(ManagedNameAttribute), false); if(rg.Length > 0) { // bug#69115 - if null or empty string are passed, we just ignore this attribute ManagedNameAttribute attr = (ManagedNameAttribute)rg[0]; if(attr.name != null && attr.name.Length != 0) return attr.name; } return member.Name; } internal static string GetBaseClassName(Type type) { InstrumentationClassAttribute attr = InstrumentationClassAttribute.GetAttribute(type); string name = attr.ManagedBaseClassName; if(name != null) return name; // Get managed base type's attribute InstrumentationClassAttribute attrParent = InstrumentationClassAttribute.GetAttribute(type.BaseType); // If the base type does not have a InstrumentationClass attribute, // return a base type based on the InstrumentationType if(null == attrParent) { switch(attr.InstrumentationType) { case InstrumentationType.Abstract: return null; case InstrumentationType.Instance: return null; case InstrumentationType.Event: return "__ExtrinsicEvent"; default: break; } } // Our parent was also a managed provider type. Use it's managed name. return GetMemberName(type.BaseType); } } ///Initializes a new instance of the ///class that allows the alternate name to be specified /// for the type, field, property, method, or parameter to which this attribute is applied. /// [AttributeUsage(AttributeTargets.Field | AttributeTargets.Property | AttributeTargets.Method)] public class IgnoreMemberAttribute : Attribute { } #if REQUIRES_EXPLICIT_DECLARATION_OF_INHERITED_PROPERTIES ///Allows a particular member of an instrumented class to be ignored /// by management instrumentation ////// [AttributeUsage(AttributeTargets.Field)] public class InheritedPropertyAttribute : Attribute { internal static InheritedPropertyAttribute GetAttribute(FieldInfo field) { Object [] rg = field.GetCustomAttributes(typeof(InheritedPropertyAttribute), false); if(rg.Length > 0) return ((InheritedPropertyAttribute)rg[0]); return null; } } #endif #if SUPPORTS_WMI_DEFAULT_VAULES [AttributeUsage(AttributeTargets.Field)] internal class ManagedDefaultValueAttribute : Attribute { Object defaultValue; public ManagedDefaultValueAttribute(Object defaultValue) { this.defaultValue = defaultValue; } public static Object GetManagedDefaultValue(FieldInfo field) { Object [] rg = field.GetCustomAttributes(typeof(ManagedDefaultValueAttribute), false); if(rg.Length > 0) return ((ManagedDefaultValueAttribute)rg[0]).defaultValue; return null; } } #endif #if SUPPORTS_ALTERNATE_WMI_PROPERTY_TYPE [AttributeUsage(AttributeTargets.Field)] internal class ManagedTypeAttribute : Attribute { Type type; public ManagedTypeAttribute(Type type) { this.type = type; } public static Type GetManagedType(FieldInfo field) { Object [] rg = field.GetCustomAttributes(typeof(ManagedTypeAttribute), false); if(rg.Length > 0) return ((ManagedTypeAttribute)rg[0]).type; return field.FieldType; } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
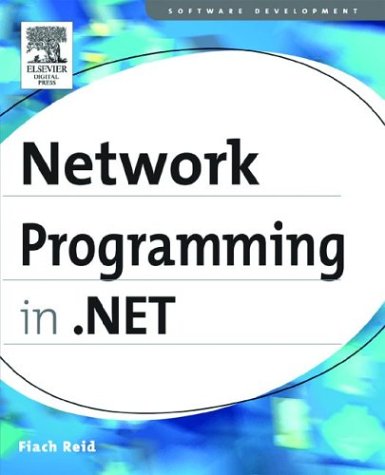
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SamlAttribute.cs
- MaskedTextProvider.cs
- ProtectedConfiguration.cs
- DynamicRendererThreadManager.cs
- NamespaceEmitter.cs
- ArrangedElementCollection.cs
- Rectangle.cs
- CryptoKeySecurity.cs
- EditingMode.cs
- Vector3DCollectionValueSerializer.cs
- GridViewCommandEventArgs.cs
- CardSpacePolicyElement.cs
- Function.cs
- ConfigUtil.cs
- Proxy.cs
- HandlerBase.cs
- FreezableOperations.cs
- XPathDocumentIterator.cs
- CompilerLocalReference.cs
- CustomErrorCollection.cs
- FormView.cs
- ConfigurationManagerInternalFactory.cs
- Win32PrintDialog.cs
- DataGridViewComboBoxColumn.cs
- GetPageNumberCompletedEventArgs.cs
- ThreadAbortException.cs
- ToolboxComponentsCreatingEventArgs.cs
- NativeMethods.cs
- AccessibleObject.cs
- OdbcParameter.cs
- MimeFormReflector.cs
- ToggleProviderWrapper.cs
- AsnEncodedData.cs
- UserNameSecurityTokenAuthenticator.cs
- wmiprovider.cs
- DataGridViewToolTip.cs
- PeerNode.cs
- JournalEntry.cs
- ServiceBuildProvider.cs
- QilTargetType.cs
- ObjectDataSourceEventArgs.cs
- UnitySerializationHolder.cs
- StringFreezingAttribute.cs
- MethodBody.cs
- PrinterSettings.cs
- EntityViewGenerationAttribute.cs
- WorkingDirectoryEditor.cs
- XmlDownloadManager.cs
- RoutedEvent.cs
- SelectionRangeConverter.cs
- UIPermission.cs
- SourceFileBuildProvider.cs
- InternalTypeHelper.cs
- FilteredDataSetHelper.cs
- UnsafeNativeMethods.cs
- CollectionViewSource.cs
- ToolboxItemAttribute.cs
- NumberAction.cs
- DirectoryObjectSecurity.cs
- PermissionAttributes.cs
- UnmanagedMemoryStream.cs
- ISAPIApplicationHost.cs
- AssemblyAttributes.cs
- StrokeRenderer.cs
- rsa.cs
- ShaderEffect.cs
- BaseValidatorDesigner.cs
- DtdParser.cs
- ArraySubsetEnumerator.cs
- SqlTrackingWorkflowInstance.cs
- NamedPermissionSet.cs
- EncryptedData.cs
- ErrorWrapper.cs
- DynamicActivity.cs
- ClrPerspective.cs
- SynchronizationLockException.cs
- BitmapMetadataBlob.cs
- RangeBase.cs
- LexicalChunk.cs
- SelectionWordBreaker.cs
- ManagedFilter.cs
- TTSEngineTypes.cs
- SqlError.cs
- EventDescriptor.cs
- WorkItem.cs
- AdornedElementPlaceholder.cs
- Hex.cs
- LingerOption.cs
- SimpleFileLog.cs
- StringComparer.cs
- DerivedKeySecurityTokenStub.cs
- LogLogRecordEnumerator.cs
- TraceContextEventArgs.cs
- ChangeProcessor.cs
- EventRecordWrittenEventArgs.cs
- DataGridTable.cs
- SignHashRequest.cs
- BitmapDecoder.cs
- DocumentStream.cs
- WeakHashtable.cs