Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmCustomContentSerializer.cs / 1305376 / EpmCustomContentSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Class used for serializing custom EntityPropertyMappingAttribute content // for non-syndication mappings // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System.Collections.Generic; using System.Diagnostics; #if !ASTORIA_CLIENT using System.ServiceModel.Syndication; using System.Data.Services.Providers; #else using System.Data.Services.Client; using System.Xml; #endif ////// Base visitor class for performing serialization of custom content in the feed entry whose mapping /// is provided through EntityPropertyMappingAttributes /// internal sealed class EpmCustomContentSerializer : EpmContentSerializerBase, IDisposable { ///IDisposable helper state private bool disposed; ////// Dictionary mapping visitor content and with target paths /// private DictionaryvisitorContent; #if ASTORIA_CLIENT /// /// Constructor initializes the base class be identifying itself as a custom content serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added internal EpmCustomContentSerializer(EpmTargetTree targetTree, object element, XmlWriter target) : base(targetTree, false, element, target) { this.InitializeVisitorContent(); } #else ////// Constructor initializes the base class be identifying itself as a custom content serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added /// Null valued properties found during serialization /// Data Service provider used for rights verification. internal EpmCustomContentSerializer(EpmTargetTree targetTree, object element, SyndicationItem target, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) : base(targetTree, false, element, target) { this.InitializeVisitorContent(nullValuedProperties, provider); } #endif ////// Closes all the XmlWriter and MemoryStream objects in the tree and adds them to the SyndicationItem /// as ElementExtensions. Invokes the NodeDataCleaner to dispose off any existing memory stream and /// XmlWriter objects /// public void Dispose() { if (!this.disposed) { foreach (EpmTargetPathSegment subSegmentOfRoot in this.Root.SubSegments) { EpmCustomContentWriterNodeData c = this.visitorContent[subSegmentOfRoot]; Debug.Assert(c != null, "Must have custom data for all the children of root"); if (this.Success) { c.AddContentToTarget(this.Target); } c.Dispose(); } this.disposed = true; } } #if ASTORIA_CLIENT ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) #else ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize /// Data Service provider used for rights verification. protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) #endif { if (targetSegment.IsAttribute) { this.WriteAttribute(targetSegment); } else { #if ASTORIA_CLIENT this.WriteElement(targetSegment); #else this.WriteElement(targetSegment, provider); #endif } } ////// Given a segment, writes the attribute to xml writer corresponding to it /// /// Segment being written private void WriteAttribute(EpmTargetPathSegment targetSegment) { // Content to be written in an attribute Debug.Assert(targetSegment.HasContent, "Must have content for attributes"); EpmCustomContentWriterNodeData currentContent = this.visitorContent[targetSegment]; currentContent.XmlContentWriter.WriteAttributeString( targetSegment.SegmentNamespacePrefix, targetSegment.SegmentName.Substring(1), targetSegment.SegmentNamespaceUri, currentContent.Data); } #if ASTORIA_CLIENT ////// Given a segment, writes the element to xml writer corresponding to it, works recursively to write child elements/attributes /// /// Segment being written private void WriteElement(EpmTargetPathSegment targetSegment) #else ////// Given a segment, writes the element to xml writer corresponding to it, works recursively to write child elements/attributes /// /// Segment being written /// Data Service provider used for rights verification. private void WriteElement(EpmTargetPathSegment targetSegment, DataServiceProviderWrapper provider) #endif { // Content to be written in an element EpmCustomContentWriterNodeData currentContent = this.visitorContent[targetSegment]; currentContent.XmlContentWriter.WriteStartElement( targetSegment.SegmentNamespacePrefix, targetSegment.SegmentName, targetSegment.SegmentNamespaceUri); #if ASTORIA_CLIENT // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Attributes); #else // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Attributes, provider); #endif if (targetSegment.HasContent) { Debug.Assert(currentContent.Data != null, "Must always have non-null data content value"); currentContent.XmlContentWriter.WriteString(currentContent.Data); } #if ASTORIA_CLIENT // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Elements); #else // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Elements, provider); #endif currentContent.XmlContentWriter.WriteEndElement(); } #if ASTORIA_CLIENT ///Initializes content for the serializer visitor private void InitializeVisitorContent() { this.visitorContent = new Dictionary(ReferenceEqualityComparer .Instance); // Initialize all the root's children's xml writers foreach (EpmTargetPathSegment subSegmentOfRoot in this.Root.SubSegments) { this.visitorContent.Add(subSegmentOfRoot, new EpmCustomContentWriterNodeData(subSegmentOfRoot, this.Element)); this.InitializeSubSegmentVisitorContent(subSegmentOfRoot); } } /// Initialize the visitor content for all of root's grandchildren and beyond /// One of root's children private void InitializeSubSegmentVisitorContent(EpmTargetPathSegment subSegment) { foreach (EpmTargetPathSegment segment in subSegment.SubSegments) { this.visitorContent.Add(segment, new EpmCustomContentWriterNodeData(this.visitorContent[subSegment], segment, this.Element)); this.InitializeSubSegmentVisitorContent(segment); } } #else ///Initializes content for the serializer visitor /// Null valued properties found during serialization /// Data Service provider used for rights verification. private void InitializeVisitorContent(EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) { this.visitorContent = new Dictionary(ReferenceEqualityComparer .Instance); // Initialize all the root's children's xml writers foreach (EpmTargetPathSegment subSegmentOfRoot in this.Root.SubSegments) { this.visitorContent.Add(subSegmentOfRoot, new EpmCustomContentWriterNodeData(subSegmentOfRoot, this.Element, nullValuedProperties, provider)); this.InitializeSubSegmentVisitorContent(subSegmentOfRoot, nullValuedProperties, provider); } } /// Initialize the visitor content for all of root's grandchildren and beyond /// One of root's children /// Null valued properties found during serialization /// Data Service provider used for rights verification. private void InitializeSubSegmentVisitorContent(EpmTargetPathSegment subSegment, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) { foreach (EpmTargetPathSegment segment in subSegment.SubSegments) { this.visitorContent.Add(segment, new EpmCustomContentWriterNodeData(this.visitorContent[subSegment], segment, this.Element, nullValuedProperties, provider)); this.InitializeSubSegmentVisitorContent(segment, nullValuedProperties, provider); } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Class used for serializing custom EntityPropertyMappingAttribute content // for non-syndication mappings // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System.Collections.Generic; using System.Diagnostics; #if !ASTORIA_CLIENT using System.ServiceModel.Syndication; using System.Data.Services.Providers; #else using System.Data.Services.Client; using System.Xml; #endif ////// Base visitor class for performing serialization of custom content in the feed entry whose mapping /// is provided through EntityPropertyMappingAttributes /// internal sealed class EpmCustomContentSerializer : EpmContentSerializerBase, IDisposable { ///IDisposable helper state private bool disposed; ////// Dictionary mapping visitor content and with target paths /// private DictionaryvisitorContent; #if ASTORIA_CLIENT /// /// Constructor initializes the base class be identifying itself as a custom content serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added internal EpmCustomContentSerializer(EpmTargetTree targetTree, object element, XmlWriter target) : base(targetTree, false, element, target) { this.InitializeVisitorContent(); } #else ////// Constructor initializes the base class be identifying itself as a custom content serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added /// Null valued properties found during serialization /// Data Service provider used for rights verification. internal EpmCustomContentSerializer(EpmTargetTree targetTree, object element, SyndicationItem target, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) : base(targetTree, false, element, target) { this.InitializeVisitorContent(nullValuedProperties, provider); } #endif ////// Closes all the XmlWriter and MemoryStream objects in the tree and adds them to the SyndicationItem /// as ElementExtensions. Invokes the NodeDataCleaner to dispose off any existing memory stream and /// XmlWriter objects /// public void Dispose() { if (!this.disposed) { foreach (EpmTargetPathSegment subSegmentOfRoot in this.Root.SubSegments) { EpmCustomContentWriterNodeData c = this.visitorContent[subSegmentOfRoot]; Debug.Assert(c != null, "Must have custom data for all the children of root"); if (this.Success) { c.AddContentToTarget(this.Target); } c.Dispose(); } this.disposed = true; } } #if ASTORIA_CLIENT ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) #else ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize /// Data Service provider used for rights verification. protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) #endif { if (targetSegment.IsAttribute) { this.WriteAttribute(targetSegment); } else { #if ASTORIA_CLIENT this.WriteElement(targetSegment); #else this.WriteElement(targetSegment, provider); #endif } } ////// Given a segment, writes the attribute to xml writer corresponding to it /// /// Segment being written private void WriteAttribute(EpmTargetPathSegment targetSegment) { // Content to be written in an attribute Debug.Assert(targetSegment.HasContent, "Must have content for attributes"); EpmCustomContentWriterNodeData currentContent = this.visitorContent[targetSegment]; currentContent.XmlContentWriter.WriteAttributeString( targetSegment.SegmentNamespacePrefix, targetSegment.SegmentName.Substring(1), targetSegment.SegmentNamespaceUri, currentContent.Data); } #if ASTORIA_CLIENT ////// Given a segment, writes the element to xml writer corresponding to it, works recursively to write child elements/attributes /// /// Segment being written private void WriteElement(EpmTargetPathSegment targetSegment) #else ////// Given a segment, writes the element to xml writer corresponding to it, works recursively to write child elements/attributes /// /// Segment being written /// Data Service provider used for rights verification. private void WriteElement(EpmTargetPathSegment targetSegment, DataServiceProviderWrapper provider) #endif { // Content to be written in an element EpmCustomContentWriterNodeData currentContent = this.visitorContent[targetSegment]; currentContent.XmlContentWriter.WriteStartElement( targetSegment.SegmentNamespacePrefix, targetSegment.SegmentName, targetSegment.SegmentNamespaceUri); #if ASTORIA_CLIENT // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Attributes); #else // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Attributes, provider); #endif if (targetSegment.HasContent) { Debug.Assert(currentContent.Data != null, "Must always have non-null data content value"); currentContent.XmlContentWriter.WriteString(currentContent.Data); } #if ASTORIA_CLIENT // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Elements); #else // Serialize the attributes and children before serializing the data itself base.Serialize(targetSegment, EpmSerializationKind.Elements, provider); #endif currentContent.XmlContentWriter.WriteEndElement(); } #if ASTORIA_CLIENT ///Initializes content for the serializer visitor private void InitializeVisitorContent() { this.visitorContent = new Dictionary(ReferenceEqualityComparer .Instance); // Initialize all the root's children's xml writers foreach (EpmTargetPathSegment subSegmentOfRoot in this.Root.SubSegments) { this.visitorContent.Add(subSegmentOfRoot, new EpmCustomContentWriterNodeData(subSegmentOfRoot, this.Element)); this.InitializeSubSegmentVisitorContent(subSegmentOfRoot); } } /// Initialize the visitor content for all of root's grandchildren and beyond /// One of root's children private void InitializeSubSegmentVisitorContent(EpmTargetPathSegment subSegment) { foreach (EpmTargetPathSegment segment in subSegment.SubSegments) { this.visitorContent.Add(segment, new EpmCustomContentWriterNodeData(this.visitorContent[subSegment], segment, this.Element)); this.InitializeSubSegmentVisitorContent(segment); } } #else ///Initializes content for the serializer visitor /// Null valued properties found during serialization /// Data Service provider used for rights verification. private void InitializeVisitorContent(EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) { this.visitorContent = new Dictionary(ReferenceEqualityComparer .Instance); // Initialize all the root's children's xml writers foreach (EpmTargetPathSegment subSegmentOfRoot in this.Root.SubSegments) { this.visitorContent.Add(subSegmentOfRoot, new EpmCustomContentWriterNodeData(subSegmentOfRoot, this.Element, nullValuedProperties, provider)); this.InitializeSubSegmentVisitorContent(subSegmentOfRoot, nullValuedProperties, provider); } } /// Initialize the visitor content for all of root's grandchildren and beyond /// One of root's children /// Null valued properties found during serialization /// Data Service provider used for rights verification. private void InitializeSubSegmentVisitorContent(EpmTargetPathSegment subSegment, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) { foreach (EpmTargetPathSegment segment in subSegment.SubSegments) { this.visitorContent.Add(segment, new EpmCustomContentWriterNodeData(this.visitorContent[subSegment], segment, this.Element, nullValuedProperties, provider)); this.InitializeSubSegmentVisitorContent(segment, nullValuedProperties, provider); } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
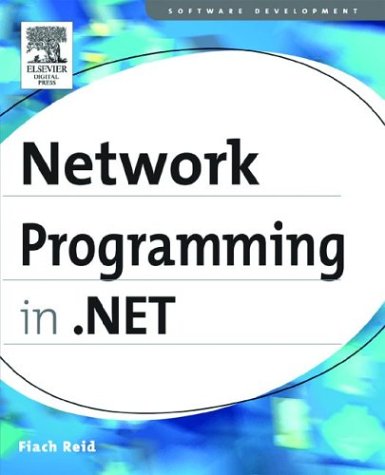
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RMEnrollmentPage3.cs
- CompoundFileStreamReference.cs
- ActivityDesignerAccessibleObject.cs
- OleDbPermission.cs
- Config.cs
- SemanticAnalyzer.cs
- ValidatingCollection.cs
- ListViewSelectEventArgs.cs
- DurableMessageDispatchInspector.cs
- PropertyEntry.cs
- SoapHeaders.cs
- RegexFCD.cs
- ServiceNameElement.cs
- FormatSettings.cs
- AuthenticateEventArgs.cs
- RegexReplacement.cs
- Size3D.cs
- SelectorAutomationPeer.cs
- TextDocumentView.cs
- SystemSounds.cs
- PublisherIdentityPermission.cs
- TextStore.cs
- IriParsingElement.cs
- TrackingMemoryStream.cs
- ErrorFormatterPage.cs
- ToolboxDataAttribute.cs
- CacheHelper.cs
- GridViewColumnCollection.cs
- CodeIdentifiers.cs
- Win32Native.cs
- StopStoryboard.cs
- _DigestClient.cs
- AVElementHelper.cs
- RequestChannel.cs
- HelpProvider.cs
- IPEndPointCollection.cs
- SQLUtility.cs
- ColumnReorderedEventArgs.cs
- WorkflowViewManager.cs
- Thumb.cs
- returneventsaver.cs
- CreateRefExpr.cs
- HttpCachePolicyElement.cs
- CurrentTimeZone.cs
- DataTemplateSelector.cs
- CheckBoxFlatAdapter.cs
- ThicknessKeyFrameCollection.cs
- Pen.cs
- ParserHooks.cs
- FigureParaClient.cs
- _SecureChannel.cs
- PatternMatchRules.cs
- _TimerThread.cs
- DictionaryEntry.cs
- ButtonBase.cs
- PropertyTabChangedEvent.cs
- WindowPatternIdentifiers.cs
- SmiMetaData.cs
- RelationshipType.cs
- CompilerScopeManager.cs
- List.cs
- SoapServerMethod.cs
- BindingExpressionUncommonField.cs
- StaticSiteMapProvider.cs
- Panel.cs
- Simplifier.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- RefreshEventArgs.cs
- ColumnPropertiesGroup.cs
- Permission.cs
- ItemAutomationPeer.cs
- SqlUserDefinedAggregateAttribute.cs
- ClrProviderManifest.cs
- Columns.cs
- AssemblyAttributes.cs
- ArrayElementGridEntry.cs
- OverrideMode.cs
- SecurityTimestamp.cs
- GZipDecoder.cs
- BufferedStream.cs
- TypeAccessException.cs
- FacetEnabledSchemaElement.cs
- TextContainerHelper.cs
- RSAOAEPKeyExchangeFormatter.cs
- SpeechRecognitionEngine.cs
- DataFieldConverter.cs
- MetabaseServerConfig.cs
- AuthenticationSection.cs
- ValidationErrorInfo.cs
- DataGridViewColumnHeaderCell.cs
- ItemContainerGenerator.cs
- NotifyCollectionChangedEventArgs.cs
- XmlIlGenerator.cs
- BigInt.cs
- TextParagraphCache.cs
- ApplicationProxyInternal.cs
- PolyBezierSegmentFigureLogic.cs
- EntityClientCacheKey.cs
- IncrementalReadDecoders.cs
- UserNameSecurityTokenProvider.cs